The Importance of HTTP Server Knowledge

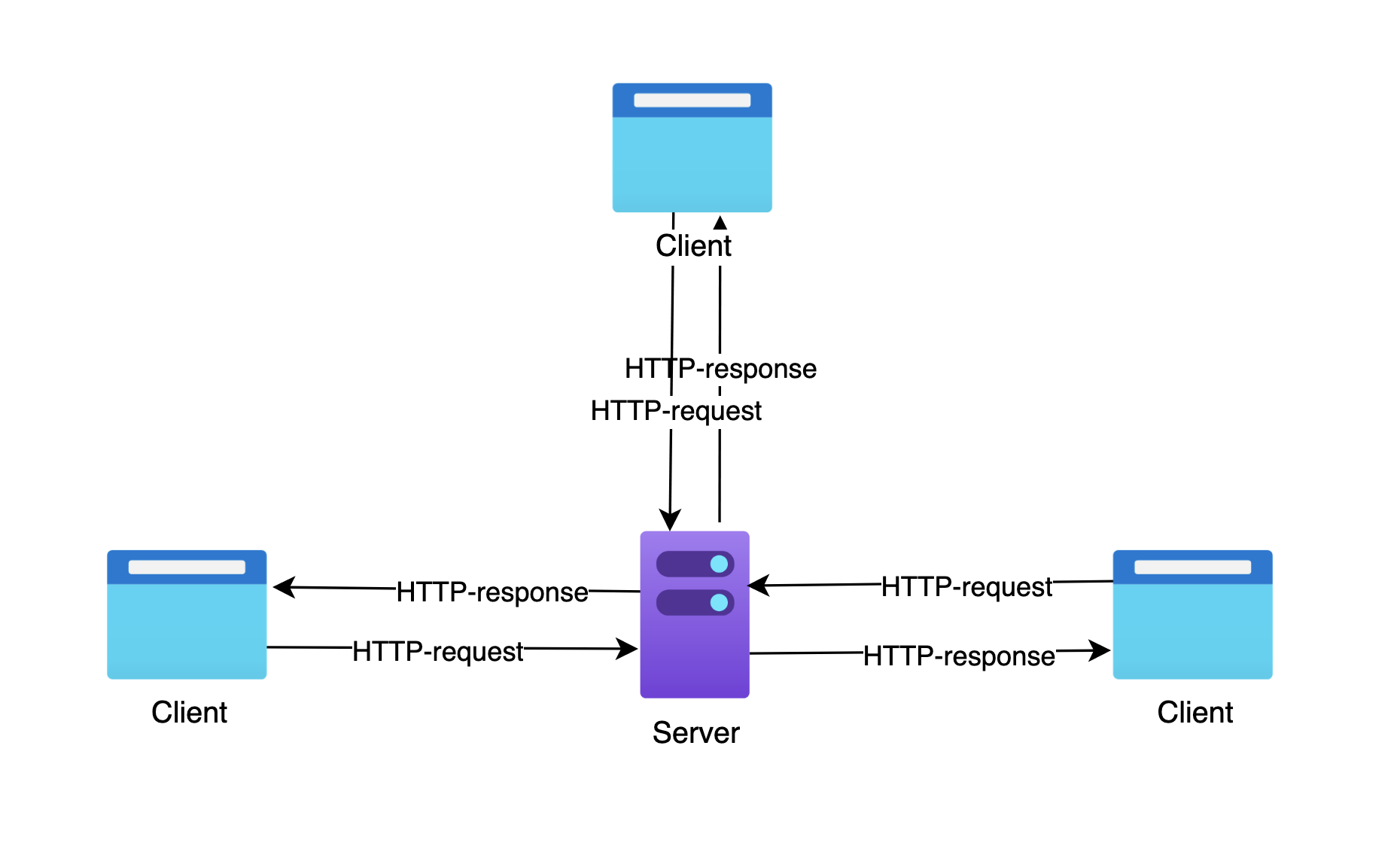
Understanding HTTP servers is fundamental for web developers and IT professionals. An HTTP server is the backbone of the web, facilitating communication between clients and servers. This blog post delves into the importance of HTTP server knowledge, how an HTTP server works, how to create one, and how it handles requests.
Why HTTP Server Knowledge is Important
Foundation of the Web: HTTP (Hypertext Transfer Protocol) is the protocol that underpins the World Wide Web. Knowing how HTTP servers work is crucial for building, maintaining, and troubleshooting websites and web applications.
Performance Optimization: Knowledge of HTTP servers can help optimize the performance of web applications by understanding server configurations, load balancing, caching, and more.
Security: Understanding HTTP servers is essential for implementing security measures such as SSL/TLS, preventing common vulnerabilities like SQL injection, and ensuring data protection.
Troubleshooting: When things go wrong, knowing how an HTTP server works allows you to diagnose and fix issues efficiently, minimizing downtime and improving user experience.
How an HTTP Server Works
An HTTP server is a software program that processes requests from clients (usually web browsers) and returns responses, typically web pages, to those clients. Here’s a simplified flow of how an HTTP server works:
Listening for Requests: The server listens on a specific port (usually port 80 for HTTP and port 443 for HTTPS) for incoming client requests.
Processing Requests: When a request arrives, the server parses the HTTP request, which includes the method (GET, POST, etc.), headers, and possibly a body.
Generating Responses: The server processes the request, which might involve retrieving data from a database, performing business logic, or simply serving static files. It then generates an HTTP response, which includes status codes, headers, and a body.
Sending Responses: The server sends the HTTP response back to the client, completing the request-response cycle.
How to Create an HTTP Server
Creating a basic HTTP server can be done using various programming languages. Here, we’ll use Node.js, a popular choice for its simplicity and efficiency.
Step-by-Step: Creating an HTTP Server in Node.js
Install Node.js: Ensure you have Node.js installed. You can download it from nodejs.org.
Create a Server: Use the built-in
http
module in Node.js to create a simple HTTP server.
// server.js
const http = require('http');
// Create an HTTP server
const server = http.createServer((req, res) => {
// Set the response header
res.writeHead(200, { 'Content-Type': 'text/plain' });
// Send response body
res.end('Hello, World!\n');
});
// Server listens on port 3000
server.listen(3000, '127.0.0.1', () => {
console.log('Server is running at http://127.0.0.1:3000/');
});
- Run the Server: Execute the server script using Node.js.
node server.js
Open your browser and navigate to http://127.0.0.1:3000/
to see your server in action.
How an HTTP Server Handles Requests
An HTTP server handles requests through the following steps:
Receiving a Request: When a client sends a request, the server receives and parses it. This includes the request line (method, URL, HTTP version), headers, and body (if present).
Routing: The server determines how to handle the request based on the URL and method. This is often managed by routing mechanisms that map URLs to specific handlers or controllers.
Processing the Request: The server executes the necessary code to process the request. This might involve querying a database, performing calculations, or fetching resources.
Generating the Response: The server constructs an HTTP response, setting the appropriate status code, headers, and body content.
Sending the Response: The server sends the response back to the client. Depending on the server configuration, this can involve compression (e.g., gzip) and other optimizations.
Example: Handling Different Request Methods
const http = require('http');
const server = http.createServer((req, res) => {
if (req.method === 'GET') {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Received a GET request\n');
} else if (req.method === 'POST') {
res.writeHead(200, { 'Content-Type': 'application/json' });
let body = '';
req.on('data', chunk => {
body += chunk;
});
req.on('end', () => {
res.end(`Received a POST request with data: ${body}\n`);
});
} else {
res.writeHead(405, { 'Content-Type': 'text/plain' });
res.end('Method not allowed\n');
}
});
server.listen(3000, '127.0.0.1', () => {
console.log('Server is running at http://127.0.0.1:3000/');
});
In this example, the server responds differently based on the request method (GET or POST).
Conclusion
Having a solid understanding of HTTP servers is crucial for anyone involved in web development. It enables you to build efficient, secure, and scalable web applications. By knowing how to create an HTTP server and understanding how it handles requests, you gain the foundational knowledge needed to troubleshoot issues, optimize performance, and enhance the overall user experience. Start exploring HTTP servers today and see how this knowledge can transform your web development skills.
Subscribe to my newsletter
Read articles from Aniket Dhaygude directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Aniket Dhaygude
Aniket Dhaygude
👨💻 Freelance Developer | UI/UX Enthusiast | Tech Blogger Transforming ideas into engaging digital experiences. I specialize in front-end development, UI/UX design, and creating dynamic web apps. Let's build something amazing together! 🚀