Mastering JavaScript Interview Essentials
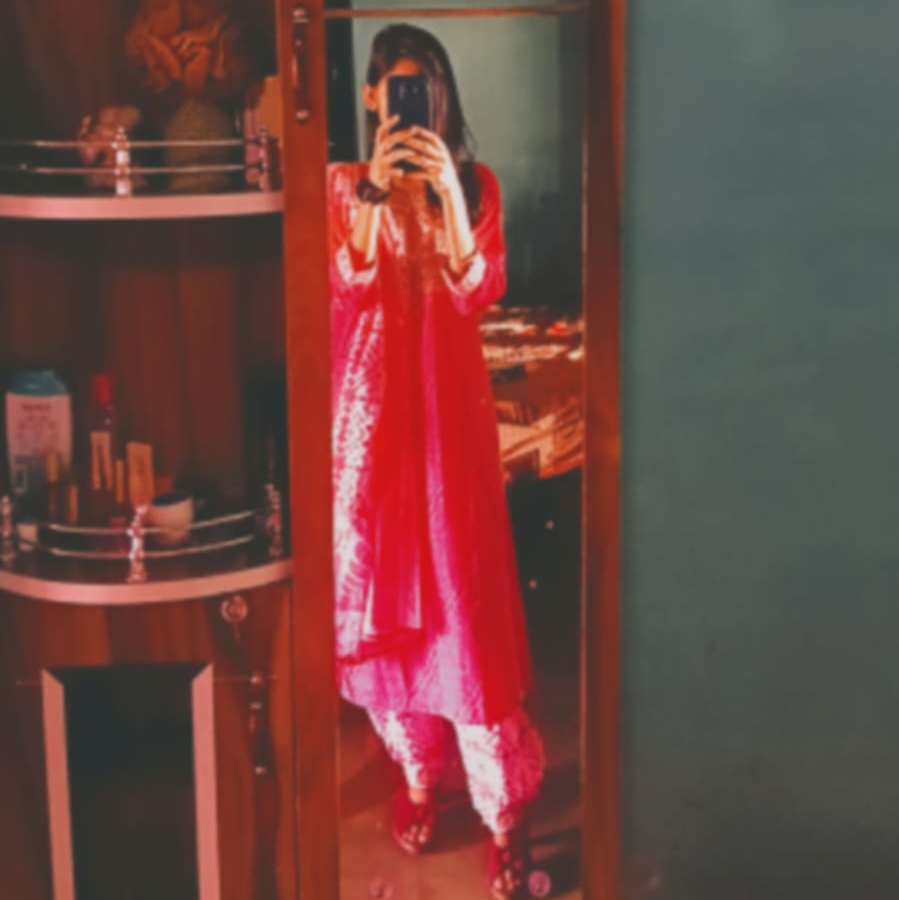
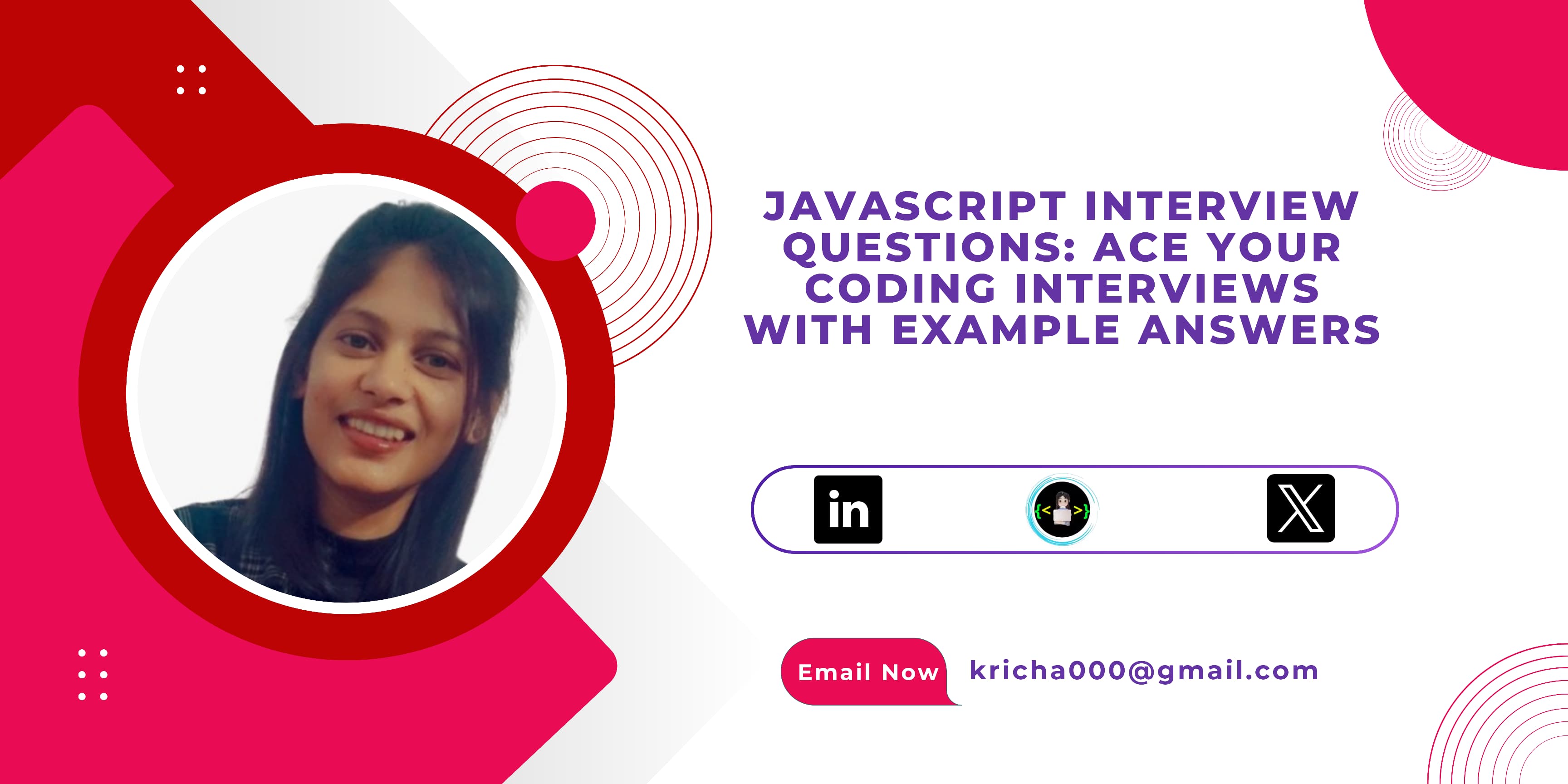
Hi Coders👩💻👨💻, I'm here to share my interview experiences. I've participated in numerous interviews where I've encountered a wide range of JavaScript questions—from the basics, like explaining different data types in JavaScript, to more complex concepts such as generator functions and async/await. This blog post aims to cover all these questions, whether you're interviewing with a large MNC or a startup. Each question comes with detailed answers and examples to help you prepare effectively for your next interview. So, let's dive in! Are you all excited? Because I definitely am!
What is JavaScript?
JavaScript is a high-level, interpreted programming language used to create interactive web pages and dynamic web applications. It is often referred to as the universal language of the web and is essential for both front-end and back-end development.
What are the different data types in JavaScript?
JavaScript has six primitive data types:
Number: Represents numeric values. Example:
let num = 10;
String: Represents textual data enclosed within quotes. Example:
let name = 'John';
Boolean: Represents true or false values. Example:
let isStudent = true;
Null: Represents an intentional absence of any object value. Example:
let data = null;
Undefined: Represents a variable that has been declared but not assigned a value. Example:
let x;
Symbol: Represents unique identifiers. Example:
const sym = Symbol('key');
JavaScript also has two compound or reference data types:
Object: Represents a collection of key-value pairs (properties).
Example:
let person = { name: 'Richa', age: 25 };
Array: Represents a list-like collection of elements.
Example:
let numbers = [1, 2, 3, 4, 5];
What is hoisting in JavaScript? How does it work?
Hoisting in JavaScript is a behavior where functions and variables can be used before their declaration.
Example:
console.log(test)
var test;
Variable Hoisting
Example: Variable declarations are hoisted to the top of their scope, but not their assignments:
console.log(name); // Output: undefined
var name = 'Richa';
console.log(name); // Output: Richa
In this example, var name;
is hoisted to the top, which means console.log(name);
doesn't throw an error. However, name
is initialized later with 'Richa'
.
var is Hosting.
let and const does not allow Hosting.
Function Hoisting
Example: Function declarations are fully hoisted, allowing you to call the function before it's declared:
codesayHello(); // Output: Hello, World!
function sayHello() {
console.log('Hello, World!');
}
In this case, sayHello();
can be called before the actual function declaration due to hoisting.
Differences between var
, let
, and const
in JavaScript:
var
:Function-scoped or globally scoped.
Variables declared with
var
are hoisted to the top of their scope and initialized withundefined
.
console.log(age); // Output: undefined
var age = 30;
console.log(age); // Output: 30
let
:Block-scoped.
Variables declared with
let
are hoisted to the top of their block but are not initialized.
console.log(name); // Output: ReferenceError: Cannot access 'name' before initialization
let name = 'John';
console.log(name); // Output: John
const
:Block-scoped.
Constants declared with
const
behave likelet
, but their value cannot be reassigned once set.
const PI = 3.14;
PI = 3.14159; // Error: Assignment to constant variable
Re-assignment:
var
:- Allows re-assignment of values.
javascriptCopy codevar a = 10;
a = 20; // Re-assignment is allowed
console.log(a); // Output: 20
let
:- Allows re-assignment of values.
javascriptCopy codelet b = 30;
b = 40; // Re-assignment is allowed
console.log(b); // Output: 40
const
:Does not allow re-assignment of the variable itself.
For objects and arrays, allows mutation of properties or elements.
javascriptCopy codeconst c = 50;
c = 60; // Error: Assignment to constant variable
const person = { name: 'Alice' };
person.name = 'Bob'; // Mutation is allowed for objects
console.log(person); // Output: { name: 'Bob' }
Temporal Dead Zone (TDZ):
let
andconst
:- Variables declared with
let
andconst
are in a "temporal dead zone" from the start of the block until the declaration is processed.
- Variables declared with
console.log(name); // Output: ReferenceError: Cannot access 'name' before initialization
let name = 'Alice';
Global Object Property:
var
:- Creates a property on the global object (
window
in browsers) when declared globally.
- Creates a property on the global object (
var globalVar = 10;
console.log(window.globalVar); // Output: 10 (in browser environment)
let
andconst
:- Do not create properties on the global object when declared globally.
let globalLet = 20;
console.log(window.globalLet); // Output: undefined
Why can't we reassign a const variable in JavaScript, but we can modify properties of a const object?
Primitive Data Types:
const
prevents reassignment because the variable directly holds the primitive value. Reassigning would mean changing this value, which is not allowed.Non-Primitive Data Types:
const
prevents reassignment of the variable itself (the reference), but it does not prevent modification of the object that the variable references. This is because the reference (memory address) remains constant, while the object's properties can be freely modified.
Primitive Data Types:
When you declare a const
variable with a primitive data type (like numbers, strings, booleans, etc.), JavaScript stores the actual value directly in the variable's memory space. Here’s an example:
const x = 5;
In this case, x
holds the value 5
. The const
keyword ensures that you cannot reassign x
to point to a different value:
// This will cause an error
x = 10;
The reason for this behavior is that x
directly contains the primitive value 5
. When you try to reassign x
, you're attempting to change the value itself, which contradicts the const
declaration.
Non-Primitive Data Types (Objects, Arrays, Functions):
Non-primitive data types (objects, arrays, functions) behave differently because they are stored and accessed via references. When you declare a const
variable that references a non-primitive data type, such as an object:
const person = { name: 'Richa', age: 25 };
Here, person
is a constant that holds a reference to the object { name: 'Richa', age: 25 }
. The reference itself (the memory address where the object is stored) is what is constant, not the contents of the object. Therefore, you can modify properties of the object:
// This is allowed
person.age = 20;
person.city = 'Bangalore';
You can also mutate the object itself:
// This is allowed
person.name = 'Reone';
However, you cannot reassign person
to point to a different object:
// This will cause an error
person = { name: 'Jane', age: 25 };
The reason modifying properties of a const
object is allowed is because you are not changing the reference stored in person
; you are merely changing the state or content of the object that person
refers to.
What is the difference between null and undefined?
null
is an assignment value that represents no valueor an empty value, while unde
fined
is a variable that has been declared but not assigned a value.
examples:
let a;
console.log(a); // Output: undefined
let person = null;
console.log(person); // Output: null
In this example, person
is explicitly set to null
, indicating that there is no meaningful object assigned to it at this time.
What is the difference between == and === operators in JavaScript?
The equality ==
operator is a comparison operator that compares two values and returns true if they are equal. The strict equality ===
operator is also a comparison operator, but it compares two values and returns true only if they are equal and of the same type.
(Equality Operator):
console.log(5 == '5'); // Output: true
console.log(1 == true); // Output: true
console.log(0 == false); // Output: true
(Strict Equality Operator):
console.log(5 === '5'); // Output: false
console.log(1 === true); // Output: false
console.log(0 === false); // Output: false
What are closures in JavaScript?
Closure is a feature in JavaScript where an inner function has access to the outer function's variables.
For example:
function hello() {
var a = 10;
var b = 20;
function print() {
var c = a + b;
console.log(c);
}
print();
}
hello(); // Output: 30
The example demonstrates how an inner function (print
) has access to variables (a
and b
) from its outer function (hello
) due to closures in JavaScript.
What is implicit type coercion in JavaScript?
Implicit type coercion is a JavaScript concept that refers to the automatic conversion of a value from one type to another. In JavaScript, this conversion follows a priority order that typically begins with strings, then numbers, and finally booleans. If you try to add a string to a number, JavaScript will implicitly coerce the number to a string before performing the addition operation because strings have the highest priority in type coercion.
For example, when you combine the number 5
with the string '10'
using the addition operator, the result is the string '510'
. This occurs because JavaScript will implicitly convert the number 5
to a string following the priority of coercion, and then concatenate it to the string '10'
.
let x = 10; let y = '5'; console.log(x + y); // Output: "105"
Explain the concept of prototypes in JavaScript.
In JavaScript, prototypes are a fundamental part of how objects inherit properties and methods from one another.
Example of Prototypes:
// Parent object constructor
function Animal(name) {
this.name = name;
}
// Adding a method to the prototype of Animal
Animal.prototype.makeSound = function() {
console.log('Animal sound');
};
// Creating instances of Animal
let animal1 = new Animal('Lion');
let animal2 = new Animal('Elephant');
// Accessing properties and methods
console.log(animal1.name); // Output: "Lion"
console.log(animal2.name); // Output: "Elephant"
animal1.makeSound(); // Output: "Animal sound"
Explain how to clone an object in JavaScript. Differentiate between shallow copy and deep copy with examples. Provide all possible methods to clone an object in JavaScript.
Cloning an object in JavaScript involves creating a duplicate that retains the original's structure and values. There are two primary methods of cloning: shallow copy and deep copy.
1. Shallow Copy:
Definition: Shallow copy creates a new object and copies all top-level properties of the original object. However, it does not recursively clone nested objects; instead, it copies references to them.
Example:
// Original object let original = { a: 1, b: { c: 2 } }; // Shallow copy using spread operator let shallowCopy = { ...original }; // Modifying the shallow copy shallowCopy.a = 5; shallowCopy.b.c = 10; console.log(original); // Output: { a: 1, b: { c: 10 } } console.log(shallowCopy); // Output: { a: 5, b: { c: 10 } }
Explanation: Changes to
shallowCopy.a
do not affectoriginal.a
, but changes toshallowCopy.b.c
affectoriginal.b.c
.
2. Deep Copy:
Definition: Deep copy creates a new object and recursively copies all properties of nested objects, ensuring the cloned object is entirely independent of the original.
Example (using JSON methods):
// Original object let original = { a: 1, b: { c: 2 } }; // Deep copy using JSON methods let deepCopy = JSON.parse(JSON.stringify(original)); // Modifying the deep copy deepCopy.a = 5; deepCopy.b.c = 10; console.log(original); // Output: { a: 1, b: { c: 2 } } console.log(deepCopy); // Output: { a: 5, b: { c: 10 } }
Explanation: Changes to
deepCopy.a
anddeepCopy.b.c
do not affectoriginal.a
andoriginal.b.c
.
Other Methods to Clone Objects:
Using
Object.assign
: Copies enumerable own properties from one or more source objects to a target object.let clone = Object.assign({}, original);
UsingObject.create
: Creates a new object with the specified prototype object and properties.
let clone = Object.create(Object.getPrototypeOf(original), Object.getOwnPropertyDescriptors(original));
What are higher-order functions in JavaScript?
Higher order functions are functions that can accept other functions as arguments or return functions as their results. They enable powerful functional programming patterns in JavaScript.
Example of Higher-Order Function:
Consider a scenario where we have an array of numbers and we want to perform various operations on them using higher-order functions.
// Example array of numbers
let numbers = [1, 2, 3, 4, 5];
// Example higher-order function: map
// 'map' applies a function to each element in an array and returns a new array with the results
let squaredNumbers = numbers.map(function(x) {
return x * x;
});
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
What is the difference between function declarations and function expressions?
Function declarations are defined using the function keyword, while function expressions are defined by assigning a function to a variable. Function declarations are hoisted, while function expressions are not.
Example of Function Declaration:
// Function Declaration
function greet(name) {
console.log(`Hello, ${name}!`);
}
// Calling the function
greet('Alice'); // Output: Hello, Alice!
Example of Function Expression:
// Function Expression
let greet = function(name) {
console.log(`Hello, ${name}!`);
};
// Calling the function
greet('Bob'); // Output: Hello, Bob!
What are the different types of errors in JavaScript?
JavaScript can throw a variety of errors, including:
Syntax errors: These errors occur when the JavaScript code is not syntactically correct.
Runtime errors: These errors occur when the JavaScript code is executed and there is a problem.
Logical errors: These errors occur when the JavaScript code does not do what it is supposed to do.
What is memoization in JavaScript?
Memoization is a technique that can be used to improve the performance of JavaScript code. Memoization works by storing the results of expensive calculations in a cache. This allows the JavaScript code to avoid re-performing the expensive calculations if the same input is provided again.
For example, the following code calculates the factorial of a number. The factorial of a number is the product of all the positive integers from one to the number.
Naive Approach (Without Memoization):
// Function to calculate factorial without memoization
function factorial(n) {
if (n === 0 || n === 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
// Calculating factorial of 5
console.log(factorial(5)); // Output: 120
In this example:
The
factorial
function calculates the factorial of a numbern
.It uses recursion to compute the factorial, which means it recalculates factorial values for the same number multiple times.
Using Memoization for Factorial Calculation:
Now, let's optimize the factorial
function using memoization. We'll store computed results in a cache to avoid redundant calculations.
// Function to calculate factorial with memoization
function memoizedFactorial() {
let cache = {};
return function factorial(n) {
if (n === 0 || n === 1) {
return 1;
} else if (cache[n]) {
return cache[n];
} else {
cache[n] = n * factorial(n - 1);
return cache[n];
}
};
}
const factorial = memoizedFactorial();
// Calculating factorial of 5
console.log(factorial(5)); // Output: 120
// Calculating factorial of 3 (should use memoized result)
console.log(factorial(3)); // Output: 6
// Calculating factorial of 5 again (should use memoized result)
console.log(factorial(5)); // Output: 120
Explanation:
Memoized Function (
memoizedFactorial
):memoizedFactorial
is a higher-order function that initializes a cache (cache
) to store factorial results.It returns an inner function (
factorial
) that calculates the factorial using recursion.Before computing the factorial, it checks if the result for
n
is already stored incache
.If
n
is found incache
, it returns the cached result; otherwise, it calculates the factorial, stores it incache
, and returns the result.
Usage:
- When
factorial
is called multiple times with the same argument, the memoization ensures that the factorial computation is performed only once for each unique input. Subsequent calls with the same input fetch the result from the cache, improving performance significantly for repeated calculations.
- When
Benefits of Memoization:
Performance Improvement: Memoization optimizes performance by reducing redundant calculations.
Space Complexity: It trades memory space for execution speed by storing computed results.
Simplicity: Once implemented, the memoized function can be used like any other function without needing to manage caching externally.
Considerations:
Pure Functions: Memoization works best with pure functions (functions that depend only on their input and produce the same output for the same input), ensuring the cache remains valid.
Cache Management: Depending on the use case, you may need to consider cache expiration or clearing mechanisms to prevent memory leaks or stale data.
Memoization is a powerful technique in JavaScript (and other programming languages) for optimizing recursive or repetitive computations, especially in scenarios where function results depend only on their arguments.
What are some key features introduced in ECMAScript 2015 (ES6)?
ECMAScript 2015 (ES6) introduced several new features and improvements to JavaScript, enhancing its capabilities and syntax. Here are some of the notable features:
let and const Declarations:
let
allows declaring block-scoped variables, replacingvar
.const
allows declaring constants whose values cannot be re-assigned.
let x = 10;
const PI = 3.14;
Arrow Functions:
Concise syntax for writing anonymous functions.
Lexical
this
binding (does not have its ownthis
,arguments
,super
, ornew.target
).
// Regular function
function add(a, b) {
return a + b;
}
// Arrow function
const add = (a, b) => a + b;
Template Literals:
- Allow embedding expressions inside string literals using backticks (``).
let name = 'Alice';
console.log(`Hello, ${name}!`);
Enhanced Object Literals:
Shorthand for initializing properties from variables with the same name.
Method definition syntax.
let x = 10, y = 20;
let obj = {
x,
y,
greet() {
return 'Hello!';
}
};
Destructuring Assignment:
- Extract values from arrays or objects into variables in a concise syntax.
let [a, b] = [1, 2];
let { x, y } = { x: 10, y: 20 };
Classes:
Syntactical sugar over JavaScript's existing prototype-based inheritance.
Allows defining class-based objects with constructors, methods, and inheritance.
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise.`);
}
}
class Dog extends Animal {
speak() {
console.log(`${this.name} barks.`);
}
}
let dog = new Dog('Buddy');
dog.speak(); // Output: Buddy barks.
Modules:
- Enable modular programming with
import
andexport
statements, allowing better organization and reuse of code.
- Enable modular programming with
// math.js
export function sum(a, b) {
return a + b;
}
// app.js
import { sum } from './math.js';
console.log(sum(5, 10)); // Output: 15
Promises:
- Provide a cleaner and more flexible alternative to callbacks for handling asynchronous operations.
function fetchData() {
return new Promise((resolve, reject) => {
// Asynchronous operation (e.g., fetching data)
if (/* success */) {
resolve(data);
} else {
reject(error);
}
});
}
fetchData().then(data => console.log(data))
.catch(error => console.error(error));
Iterators and Generators:
- Allow custom iteration behavior in JavaScript using
Symbol.iterator
andfunction*
.
let iterable = {
[Symbol.iterator]() {
let count = 0;
return {
next() {
return count < 3 ? { value: count++, done: false } : { done: true };
}
};
}
};
for (let value of iterable) {
console.log(value); // Output: 0, 1, 2
}
Default Parameters for Function Arguments:
Default parameters allow you to initialize parameters with default values if no value or undefined
is passed. This simplifies function definitions by reducing the need for explicit checks and default assignments.
Example:
// Function with default parameter
function greet(name = 'Guest') {
console.log(`Hello, ${name}!`);
}
greet('Alice'); // Output: Hello, Alice!
greet(); // Output: Hello, Guest!
In this example:
The
greet
function has a default parametername
set to'Guest'
.When
greet('Alice')
is called with an argument, it uses the provided value ('Alice'
).When
greet()
is called without an argument, it uses the default value ('Guest'
).
11. Rest Parameters (...args):
Rest parameters allow a function to accept an indefinite number of arguments as an array. This is useful when you want to work with a variable number of arguments without explicitly naming them.
Example:
// Function with rest parameters
function sum(...args) {
let total = 0;
for (let num of args) {
total += num;
}
return total;
}
console.log(sum(1, 2, 3)); // Output: 6
console.log(sum(1, 2, 3, 4, 5)); // Output: 15
In this example:
The
sum
function uses rest parameters (...args
) to collect all provided arguments into an array namedargs
.It then iterates over
args
to compute the total sum of all numbers passed to the function.
12 . Spread Syntax (...) for Arrays and Function Calls:
Spread syntax allows an iterable (like an array) to be expanded into individual elements. It is useful for array literals, function arguments, and object literals.
Example with Arrays:
// Array example
let numbers = [1, 2, 3];
let moreNumbers = [4, 5, 6];
// Combining arrays using spread syntax
let combined = [...numbers, ...moreNumbers];
console.log(combined); // Output: [1, 2, 3, 4, 5, 6]
Example with Function Calls:
// Function example
function multiply(x, y, z) {
return x * y * z;
}
let args = [2, 3, 4];
// Calling function with spread syntax
console.log(multiply(...args)); // Output: 24
In these examples:
The first example uses spread syntax (
...numbers
and...moreNumbers
) to concatenate arrays.The second example uses spread syntax (
...args
) to pass elements of an array as individual arguments to themultiply
function.
What is recursion in JavaScript?
Recursion is a programming technique that allows a function to call itself. Recursion can be used to solve a variety of problems, such as finding the factorial of a number or calculating the Fibonacci sequence.
Factorial Using Recursion
The factorial of a non-negative integer n
is the product of all positive integers less than or equal to n
. It's denoted as n!
.
// Recursive function to compute factorial
function factorial(n) {
// Base case: factorial of 0 or 1 is 1
if (n === 0 || n === 1) {
return 1;
}
// Recursive case: factorial of n is n * factorial(n - 1)
return n * factorial(n - 1);
}
// Example usage:
console.log(factorial(5)); // Output: 120 (5! = 5 * 4 * 3 * 2 * 1)
console.log(factorial(0)); // Output: 1 (0! and 1! are both 1)
What is the use of a constructor function in JavaScript?
A constructor function is a special type of function that is used to create objects. Constructor functions are used to define the properties and methods of an object.
Example of a Constructor Function
Let's create a Person
constructor function that will define a blueprint for creating person objects with name
and age
properties:
// Constructor function for Person objects
function Person(name, age) {
this.name = name;
this.age = age;
// Example method
this.sayHello = function() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
};
}
What is a callback function in JavaScript?
A callback
function is a function passed as an argument to another function, which is then invoked inside the outer function. It allows asynchronous or event-driven programming.
Example of a Callback Function
Let's define a function doHomework
which takes two parameters: subject
(a string) and callback
(a function). The callback
function will be invoked after a simulated homework completion process.
// Example of a callback function
function doHomework(subject, callback) {
console.log(`Starting my ${subject} homework.`);
callback();
}
// Callback function definition
function finishedHomework() {
console.log('Finished my homework!');
}
// Using the callback function
doHomework('Math', finishedHomework);
What are promises in JavaScript? Benefits of promise Promise
A Promise in JavaScript is an object that connects the producing code and consuming code. It has three states: pending, fulfilled, and rejected. Promises enhance code readability and improve handling of asynchronous operations and errors.
Key Aspects of Promises:
States:
Pending: Initial state, neither fulfilled nor rejected.
Fulfilled: The operation completed successfully.
Rejected: The operation failed.
Handlers:
.then()
: Handles fulfillment of the promise..catch()
: Handles rejection of the promise..finally()
: Executes regardless of the promise's outcome (optional).
Benefits of Promises:
Improved Readability:
- Promises make asynchronous code more readable compared to traditional callback-based code, especially for handling multiple asynchronous operations sequentially or in parallel.
Better Error Handling:
- Errors in promises can be handled using
.catch()
at the end of the promise chain, which consolidates error handling logic and avoids nested error callbacks.
- Errors in promises can be handled using
Chaining:
- Promises can be chained using
.then()
to sequence asynchronous operations in a more natural and synchronous-looking way.
- Promises can be chained using
Composition:
- Promises can be composed using utilities like
Promise.all()
andPromise.race()
to handle multiple asynchronous operations concurrently or conditionally.
// Example: Simulating a delayed operation using a Promise
// Creating a Promise
const myPromise = new Promise((resolve, reject) => {
// Simulating an asynchronous operation (e.g., fetching data)
setTimeout(() => {
const success = true; // Simulating success or failure
if (success) {
resolve("Data successfully fetched!"); // Resolve the promise
} else {
reject(new Error("Failed to fetch data.")); // Reject the promise
}
}, 2000); // Simulating a 2-second delay
});
// Consuming the Promise
myPromise.then((result) => {
console.log(result); // Output: Data successfully fetched!
}).catch((error) => {
console.error(error.message); // Output: Failed to fetch data.
});
Why do we need Promise?
Promises are crucial in JavaScript to effectively manage asynchronous operations, avoid callback hell, handle errors uniformly, support compositional operations, benefit from standardization, and ensure compatibility with future JavaScript enhancements like async/await syntax.
Why do we need Callback?
Callbacks are essential in JavaScript for handling asynchronous operations, allowing functions to be executed asynchronously when an operation completes, ensuring non-blocking behavior, and facilitating event-driven programming. They enable developers to manage control flow and handle responses or errors from asynchronous tasks effectively.
What is Callback Hell and Promise Chaining?
Callback Hell:
Callback hell refers to the situation where multiple nested callbacks are used to handle asynchronous operations in JavaScript. This nesting can become deep and unreadable, making the code difficult to manage, debug, and maintain. It typically arises when handling multiple asynchronous tasks
Promise Chaining:
Promises offer a solution to callback hell by providing a more structured way to handle asynchronous operations. Instead of nesting callbacks, promises allow chaining using .then()
and .catch()
methods.
What is the difference between synchronous and asynchronous programming?
Synchronous Programming:
In synchronous programming, tasks are executed sequentially, one after the other.
Each statement or function call blocks the execution of the program until it completes.
The program waits for each operation to finish before moving on to the next one.
This blocking behavior can lead to inefficiencies, especially when dealing with tasks that involve waiting for I/O operations (like fetching data from a server or reading a file).
Asynchronous Programming:
In asynchronous programming, tasks can be executed concurrently or in parallel.
The program initiates an operation and continues to execute other tasks without waiting for the operation to complete.
Callbacks, Promises, or async/await syntax are commonly used to handle asynchronous operations.
Asynchronous programming is well-suited for tasks that involve waiting for I/O operations or handling events, as it allows the program to remain responsive and efficient.
Synchronous Example:
// Synchronous function
function syncFunction() {
console.log("Synchronous task 1");
console.log("Synchronous task 2");
console.log("Synchronous task 3");
}
// Calling synchronous function
console.log("Start synchronous execution");
syncFunction();
console.log("End synchronous execution");
Asynchronous Example:
// Asynchronous function with setTimeout
function asyncFunction() {
console.log("Async task 1");
setTimeout(() => {
console.log("Async task 2 (after 2 seconds)");
}, 2000);
console.log("Async task 3");
}
// Calling asynchronous function
console.log("Start asynchronous execution");
asyncFunction();
console.log("End asynchronous execution");
How do you handle errors in JavaScript?
Errors in JavaScript can be handled using try-catch blocks. The try block contains the code that may throw an error, and the catch block handles the error and provides an alternative execution path.
try {
// Code that may throw an error
throw new Error('Something went wrong!');
} catch (error) {
console.error('Error caught:', error.message);
}
Explain the concept of event bubbling in JavaScript.
Event bubbling is the propagation of an event from a target element up through its ancestor elements in the DOM tree.
<div id="parent">
<button id="child">Click Me</button>
</div>
<script>
document.getElementById('child').addEventListener('click', function() {
console.log('Child clicked');
});
document.getElementById('parent').addEventListener('click', function() {
console.log('Parent clicked');
});
</script>
What are arrow functions in JavaScript?
Arrow functions provide a concise syntax for writing functions and inherit this
from their surrounding scope.
const add = (a, b) => a + b;
console.log(add(2, 3)); // Output: 5
What is the difference between querySelector and getElementById in JavaScript?
querySelector
is a more versatile method that allows you to select elements using CSS-like selectors, while getElementById
specifically selects an element with the specified ID.
const element1 = document.querySelector('.example'); // Selects by class
const element2 = document.getElementById('example'); // Selects by ID
What is the purpose of the setTimeout() function in JavaScript?
The setTimeout()
function is used to delay the execution of a function or the evaluation of an expression after a specified amount of time in milliseconds.
console.log('Start');
setTimeout(() => {
console.log('Delayed execution');
}, 2000);
console.log('End');
What is event delegation and why is it useful?
Event delegation attaches a single event listener to a parent element to handle events for its child elements.
Event delegation is a technique where you attach a single event listener to a parent element to handle events occurring on its child elements. It’s useful for dynamically created elements or when you have a large number of elements.
<ul id="parentList">
<li>Item 1</li>
<li>Item 2</li>
</ul>
<script>
document.getElementById('parentList').addEventListener('click', function(event) {
if (event.target.tagName === 'LI') {
console.log('Clicked on:', event.target.textContent);
}
});
</script>
How can you prevent the default behavior of an event in JavaScript?
You can use the preventDefault()
method on the event object within an event handler to prevent the default behavior associated with that event.
<a href="#" onclick="event.preventDefault();">Click me</a>
What is the difference between localStorage and sessionStorage in JavaScript?
Both localStorage
and sessionStorage
are web storage objects in JavaScript, but they have different scopes and lifetimes.
localStorage
persists data even after the browser window is closed and is accessible across different browser tabs/windows of the same origin.sessionStorage
stores data for a single browser session and is accessible only within the same tab or window.
How can you convert a string to lowercase in JavaScript?
You can use the toLowerCase()
method to convert a string to lowercase in JavaScript.
const str = 'Hello World';
console.log(str.toLowerCase()); // Output: 'hello world'
What is the purpose of the map() function in JavaScript?
The map()
function is used to iterate over an arrayand apply a transformation or computation on each element. It returns a new array with the results of the transformation.
const numbers = [1, 2, 3];
const doubled = numbers.map(num => num * 2);
console.log(doubled); // Output: [2, 4, 6]
What is the difference between splice() and slice() in JavaScript?
splice()
is used to modify an array by adding, removing, or replacing elements at a specific position.slice()
is used to create a new array that contains a portion of an existing array, specified by the starting and ending indices.
const fruits = ['Apple', 'Banana', 'Cherry'];
const removed = fruits.splice(1, 1, 'Orange'); // Modifies array
const sliced = fruits.slice(1, 2); // Returns new array
What is the purpose of the reduce() function in JavaScript?
The reduce() functionis used to reduce an array to a single value by applying a function to each element and accumulating the result.
const numbers = [1, 2, 3, 4];
const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
console.log(sum); // Output: 10
How can you check if an array includes a certain value in JavaScript?
You can use the includes()
method to check if an array includes a specific value. It returns true if the value is found, and false otherwise.
const numbers = [1, 2, 3];
console.log(numbers.includes(2)); // Output: true
What is the difference between prototype and instance properties in JavaScript?
Prototype properties are shared among all instances of an object, while instance properties are unique to each instance.
function Person(name) {
this.name = name; // Instance property
}
Person.prototype.age = 30; // Prototype property
What is the difference between an array and an object in JavaScript?
An array is a data structure that can store a collection of values. An object is a data structure that can store a collection of properties.
Arrays are indexed by numbers. Objects are indexed by strings. Arrays can only store primitive data types and objects. Objects can store primitive data types, objects and arrays.
const fruitsArray = ['Apple', 'Banana'];
const fruitsObject = { fruit1: 'Apple', fruit2: 'Banana' };
How can you remove duplicates from an array in JavaScript?
Use a Set or filter()
method with indexOf()
to remove duplicates from an array.
const numbers = [1, 2, 2, 3, 3];
const uniqueNumbers =new Set([...numbers]);
// Or using filter and indexOf
const uniqueNumbersFiltered = numbers.filter((value, index) => numbers.indexOf(value) === index);
What is the purpose of the fetch() function in JavaScript?
fetch()
is used to make asynchronous HTTP requests and handle responses using Promises.javascriptCopy codefetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
What is a generator function in JavaScript?
A generator function in JavaScript allows pausing and resuming execution to generate a sequence of values using yield
.
javascriptCopy codefunction* generatorFunction() {
yield 1;
yield 2;
yield 3;
}
const gen = generatorFunction();
console.log(gen.next().value); // Output: 1
What are the different events in JavaScript?
There are many different events in JavaScript, but some of the most common events include:
Click: The click event occurs when a user clicks on an HTML element.
Mouseover: The mouseover event occurs when a user's mouse pointer moves over an HTML element.
Keydown: The keydown event occurs when a user presses a key on the keyboard.
Keyup: The keyup event occurs when a user releases a key on the keyboard.
Change: The change event occurs when a user changes the value of an HTML input element.
<button onclick="console.log('Clicked')">Click Me</button>
What are the different ways to access an HTML element in JavaScript?
There are three main ways to access an HTMLelement in JavaScript:
Using the
getElementById()
method: ThegetEl
emen
tById()
method takes a string as an argument and returns the HTML element with the specified ID.Using the
getElementsByTagName()
method: Theget
ElementsByTagName()
method takes a string as an argument and returns an array of all the HTML elements with the specified tag name.Using the
querySelector()
method: ThequeryS
elec
tor()
method takes a CSS selector as an argument and returns the first HTML element that matches the selector.
const elementById = document.getElementById('example');
const elementsByTagName = document.getElementsByTagName('div');
const elementByQuerySelector = document.querySelector('.example');
What is the scope of a variable in JavaScript?
Variable scope in JavaScript defines where a variable can be accessed within the code.
function exampleScope() {
if (true) {
var localVar = 'Local'; // Function-scoped with var
let blockVar = 'Block'; // Block-scoped with let
const constVar = 'Constant'; // Block-scoped with const
}
console.log(localVar); // 'Local'
console.log(blockVar); // Error: blockVar is not defined
}
What are the different ways to create objects in JavaScript?
Objects can be created using object literals, constructor functions, Object.create()
, or the ES6 class
syntax.
// Using object literals
const person = {
name: 'John',
age: 30
};
What is the purpose of the window object in JavaScript?
he window
object represents the browser window. The window
object can be used to access the browser’s features, such as the location bar, the status bar and the bookmarks bar.
window.location.href = 'https://www.example.com';
What is the purpose of the async and await keywords in JavaScript?
The async
and await
keywords are used for handling asynchronous operations in a more synchronous-like manner. The async
keyword is used to define an asynchronous function, and the await
keyword is used to pause the execution of an async function until a promise is fulfilled or rejected.
async function fetchData() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
}
Tips for Success
To stand out in your JavaScript interview:
Practice Explanation: Practice explaining concepts clearly and concisely, focusing on both theoretical understanding and practical application.
Code Practice: Regularly practice coding exercises to reinforce your problem-solving skills.
Stay Updated: Keep abreast of the latest JavaScript updates and best practices.
🎯 Wrap Up!!
Preparing comprehensively for a JavaScript interview involves mastering core concepts, practicing coding challenges, and being able to communicate effectively about your experience and knowledge. Remember, while technical skills are crucial, your ability to articulate and demonstrate your understanding will set you apart. Embrace the learning journey, and good luck with your interview preparation!
If you found this guide helpful, like, share, and leave a comment. Let me know if there are any topics you'd like me to cover in my next blog post. Your feedback drives the content, ensuring it’s both informative and engaging for JavaScript enthusiasts like you.
Subscribe to my newsletter
Read articles from Richa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
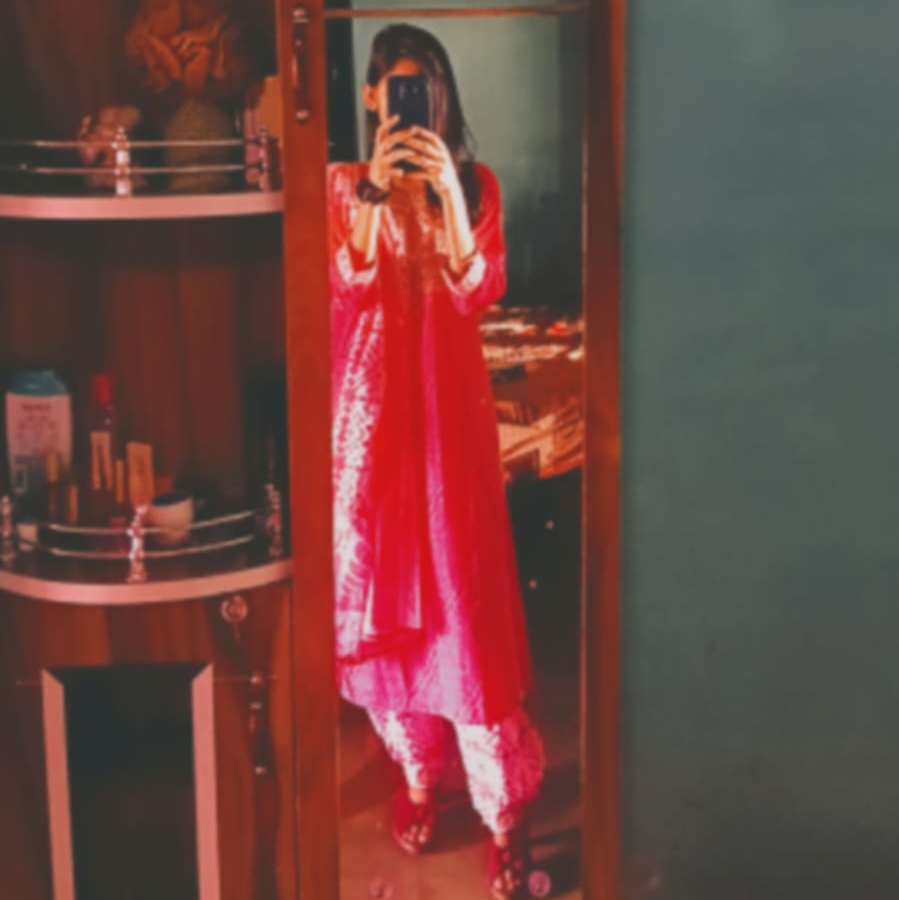
Richa
Richa
Hello Coders!! 👋 I'm Richa, and I'm passionate about web development. 🚀🧿 Join me on this exciting journey if you're eager to learn! Remember, "If you want to excel in any field, ask questions about things you're not clear about." 🙌 Let's grow together!