Node.js Basics: A Beginner's Step-by-Step Guide
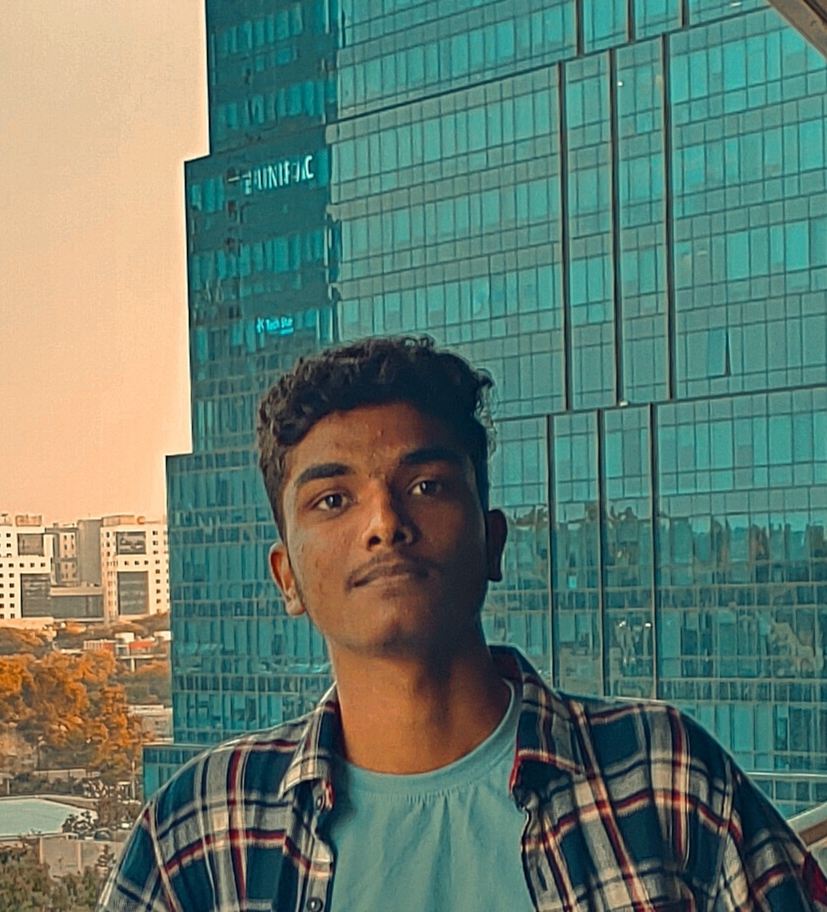
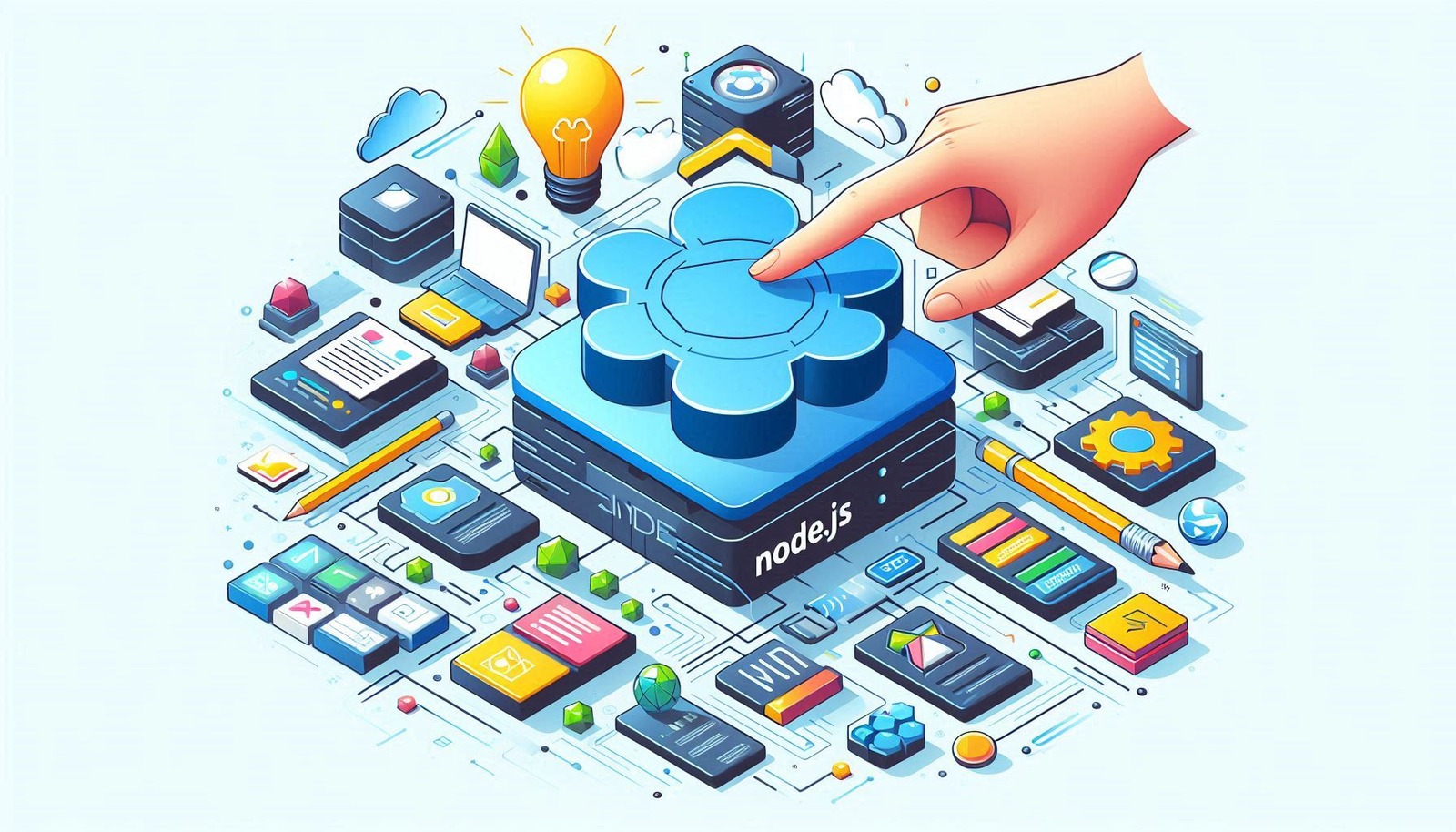
Introduction
Node.js is an open-source, cross-platform runtime environment that allows developers to use JavaScript for server-side scripting. This innovation has fundamentally transformed web development, enabling the creation of fast, scalable network applications. In this blog, we'll delve into what makes Node.js unique, explore its core features, and walk you through building your first Node.js application.
What is Node.js?
Node.js was created by Ryan Dahl in 2009 and is built on Chrome's V8 JavaScript engine. It enables JavaScript to be run on the server side, outside of the browser. This breakthrough means that developers can use a single programming language across both client and server environments, leading to more efficient and cohesive development processes.
Key Features of Node.js
Asynchronous and Event-Driven: Node.js operates on an event-driven, non-blocking I/O model, making it ideal for real-time applications that require high throughput, such as chat applications, online gaming, and live streaming.
Single Programming Language: With Node.js, you can write both server-side and client-side scripts in JavaScript, facilitating code reusability and a smoother development workflow.
High Performance: Node.js is built on the V8 JavaScript engine, which compiles JavaScript into native machine code before execution, resulting in faster performance.
Large Ecosystem: The Node Package Manager (npm) is the largest ecosystem of open-source libraries in the world, providing numerous modules and packages that can be easily integrated into your projects.
Community Support: Node.js has a vast and active community that contributes to its continuous improvement. This means a wealth of resources, tutorials, and support is available for developers at all levels.
Setting Up Your Node.js Environment
To start developing with Node.js, you need to install Node.js and npm on your computer.
Installation Steps
Download Node.js: Visit the Node.js website and download the latest stable version for your operating system.
Install Node.js: Run the installer and follow the instructions. This will also install npm, the package manager for Node.js.
Verify Installation: Open your terminal or command prompt and enter the following commands to verify the installation:
node -v npm -v
These commands should display the installed versions of Node.js and npm.
Building Your First Node.js Application
Let’s create a basic web server with Node.js.
Step 1: Initialize a New Project
Open your terminal and create a new directory for your project:
mkdir my-node-app cd my-node-app
Initialize a new Node.js project:
npm init -y
This command creates a
package.json
file with default settings.
Step 2: Create a Simple Server
Create a file named
app.js
in your project directory and add the following code:const http = require('http'); const hostname = '127.0.0.1'; const port = 3000; const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello, World!\n'); }); server.listen(port, hostname, () => { console.log(`Server running at http://${hostname}:${port}/`); });
Save the file and start your server:
node app.js
Open your web browser and navigate to
http://127.0.0.1:3000
. You should see the message "Hello, World!".
Conclusion
Node.js is a versatile and powerful runtime environment that opens up new possibilities for JavaScript developers. Its non-blocking, event-driven architecture makes it ideal for building scalable and high-performance applications. Whether you're creating a simple web server or a complex real-time application, Node.js provides the tools and community support you need to succeed.
Happy coding!
Subscribe to my newsletter
Read articles from Gondi Bharadhwaj Reddy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
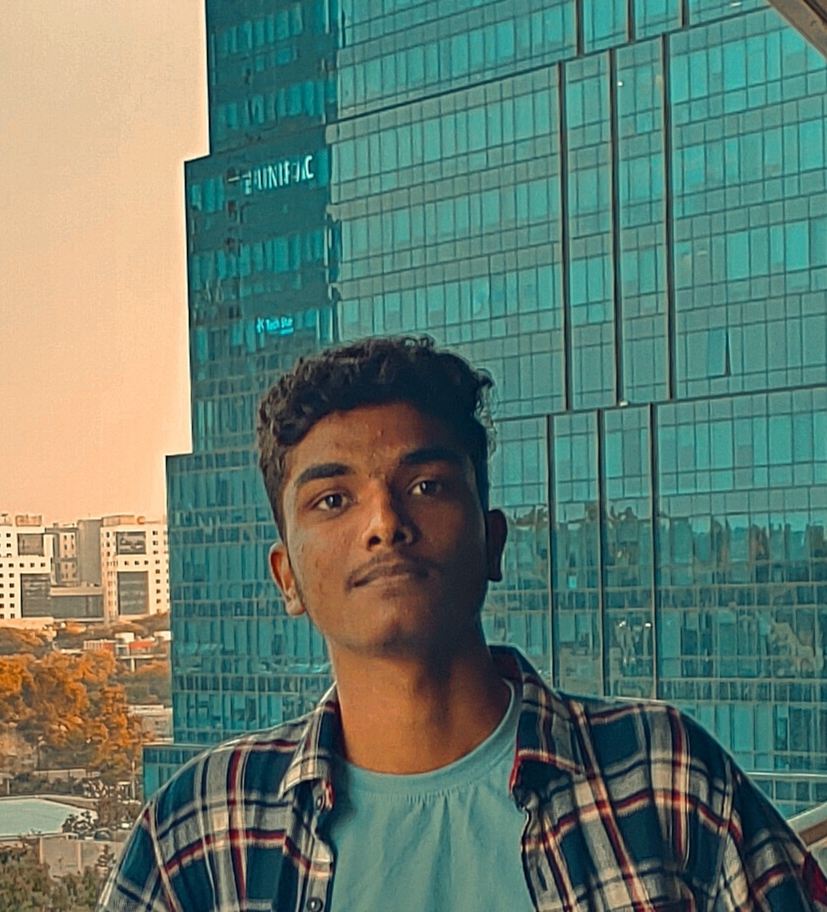
Gondi Bharadhwaj Reddy
Gondi Bharadhwaj Reddy
I am a dedicated undergraduate student passionate about exploring and learning new things. My interests span across various fields, from technology and web development. Currently focused on projects that enhance learning experiences and make meaningful contributions to communities.