Implementing Governance and Access Control in Smart Contracts with OpenZeppelin v5
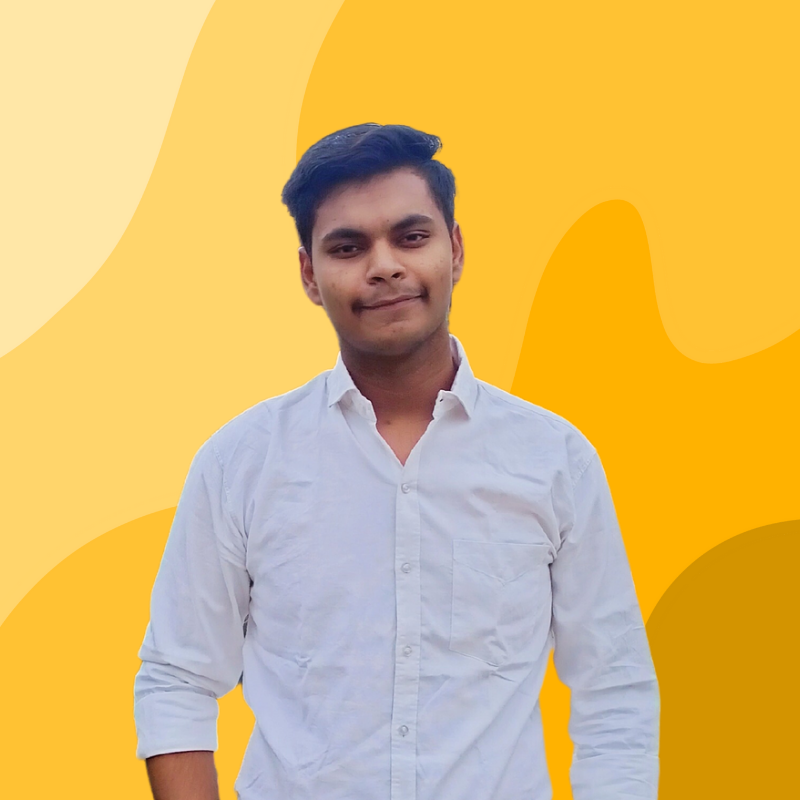
Introduction
In the world of decentralized applications and smart contracts, governance and access control are crucial components that ensure the security, flexibility, and longevity of your project. This article will dive deep into how to implement these features using OpenZeppelin v5, a library of reusable and secure smart contract components.
Understanding Governance in Smart Contracts
Governance in smart contracts refers to the mechanisms that allow token holders to participate in the decision-making process of a protocol. It typically involves proposing, voting on, and implementing changes to the protocol.
Key Components of Governance
Proposal Mechanism: Allows users to suggest changes or actions.
Voting System: Enables token holders to cast votes on proposals.
Execution Process: Implements approved proposals automatically.
Access Control in Smart Contracts
Access control is the practice of restricting system access to authorized users. In smart contracts, this often means limiting certain functions to specific roles or addresses.
Types of Access Control
Role-Based Access Control (RBAC): Assigns permissions to roles, and roles to users.
Ownership: A simple form where a single address (the owner) has special privileges.
Implementing Governance with OpenZeppelin v5
OpenZeppelin provides a suite of contracts to implement governance. Let's walk through the key components:
1. The Governor Contract
The Governor
contract is the core of the governance system. Here's a basic implementation:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
import "@openzeppelin/contracts/governance/Governor.sol";
import "@openzeppelin/contracts/governance/extensions/GovernorSettings.sol";
import "@openzeppelin/contracts/governance/extensions/GovernorCountingSimple.sol";
import "@openzeppelin/contracts/governance/extensions/GovernorVotes.sol";
import "@openzeppelin/contracts/governance/extensions/GovernorVotesQuorumFraction.sol";
contract MyGovernor is Governor, GovernorSettings, GovernorCountingSimple, GovernorVotes, GovernorVotesQuorumFraction {
constructor(IVotes _token)
Governor("MyGovernor")
GovernorSettings(1 /* 1 block */, 50400 /* 1 week */, 0)
GovernorVotes(_token)
GovernorVotesQuorumFraction(4)
{}
// The following functions are overrides required by Solidity.
function votingDelay()
public
view
override(IGovernor, GovernorSettings)
returns (uint256)
{
return super.votingDelay();
}
function votingPeriod()
public
view
override(IGovernor, GovernorSettings)
returns (uint256)
{
return super.votingPeriod();
}
function quorum(uint256 blockNumber)
public
view
override(IGovernor, GovernorVotesQuorumFraction)
returns (uint256)
{
return super.quorum(blockNumber);
}
function proposalThreshold()
public
view
override(Governor, GovernorSettings)
returns (uint256)
{
return super.proposalThreshold();
}
}
This contract sets up a basic governance system with:
A voting delay of 1 block
A voting period of 1 week
A quorum of 4% of total supply
2. The Token Contract
For governance to work, you need a token that supports voting. OpenZeppelin provides the ERC20Votes
extension:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
import "@openzeppelin/contracts/token/ERC20/extensions/ERC20Permit.sol";
import "@openzeppelin/contracts/token/ERC20/extensions/ERC20Votes.sol";
contract MyToken is ERC20, ERC20Permit, ERC20Votes {
constructor() ERC20("MyToken", "MTK") ERC20Permit("MyToken") {
_mint(msg.sender, 1000000 * 10 ** decimals());
}
// The following functions are overrides required by Solidity.
function _update(address from, address to, uint256 amount)
internal
override(ERC20, ERC20Votes)
{
super._update(from, to, amount);
}
function _mint(address to, uint256 amount)
internal
override(ERC20, ERC20Votes)
{
super._mint(to, amount);
}
function _burn(address account, uint256 amount)
internal
override(ERC20, ERC20Votes)
{
super._burn(account, amount);
}
}
This token contract includes voting capabilities, which are necessary for governance.
Implementing Access Control with OpenZeppelin v5
OpenZeppelin provides robust tools for implementing access control. Let's look at two common approaches:
1. Role-Based Access Control (RBAC)
RBAC is implemented using the AccessControl
contract:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
import "@openzeppelin/contracts/access/AccessControl.sol";
contract MyContract is AccessControl {
bytes32 public constant MINTER_ROLE = keccak256("MINTER_ROLE");
bytes32 public constant BURNER_ROLE = keccak256("BURNER_ROLE");
constructor() {
_grantRole(DEFAULT_ADMIN_ROLE, msg.sender);
_grantRole(MINTER_ROLE, msg.sender);
_grantRole(BURNER_ROLE, msg.sender);
}
function mint(address to, uint256 amount) public onlyRole(MINTER_ROLE) {
// Minting logic here
}
function burn(address from, uint256 amount) public onlyRole(BURNER_ROLE) {
// Burning logic here
}
}
This contract defines two roles (MINTER_ROLE and BURNER_ROLE) and restricts certain functions to addresses with these roles.
2. Ownable Pattern
For simpler access control, OpenZeppelin provides the Ownable
contract:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
import "@openzeppelin/contracts/access/Ownable.sol";
contract MyContract is Ownable {
constructor() Ownable(msg.sender) {}
function sensitiveFunction() public onlyOwner {
// Only the owner can call this function
}
}
This pattern is useful when you only need to restrict access to a single privileged account.
Combining Governance and Access Control
In many cases, you'll want to combine governance and access control. Here's an example of how you might do that:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
import "@openzeppelin/contracts/governance/Governor.sol";
import "@openzeppelin/contracts/governance/extensions/GovernorSettings.sol";
import "@openzeppelin/contracts/governance/extensions/GovernorCountingSimple.sol";
import "@openzeppelin/contracts/governance/extensions/GovernorVotes.sol";
import "@openzeppelin/contracts/governance/extensions/GovernorVotesQuorumFraction.sol";
import "@openzeppelin/contracts/governance/extensions/GovernorTimelockControl.sol";
import "@openzeppelin/contracts/access/AccessControl.sol";
contract MyGovernor is Governor, GovernorSettings, GovernorCountingSimple, GovernorVotes, GovernorVotesQuorumFraction, GovernorTimelockControl, AccessControl {
constructor(IVotes _token, TimelockController _timelock)
Governor("MyGovernor")
GovernorSettings(1 /* 1 block */, 50400 /* 1 week */, 0)
GovernorVotes(_token)
GovernorVotesQuorumFraction(4)
GovernorTimelockControl(_timelock)
{
_grantRole(DEFAULT_ADMIN_ROLE, msg.sender);
}
function propose(address[] memory targets, uint256[] memory values, bytes[] memory calldatas, string memory description)
public
override(Governor, IGovernor)
onlyRole(DEFAULT_ADMIN_ROLE)
returns (uint256)
{
return super.propose(targets, values, calldatas, description);
}
// ... (other overrides as in previous examples)
}
In this example, we've combined the Governor
contract with AccessControl
, allowing us to restrict who can create proposals.
Conclusion
Implementing governance and access control in your smart contracts is crucial for creating secure, flexible, and community-driven protocols. OpenZeppelin v5 provides powerful tools to help you achieve this. By understanding and properly implementing these concepts, you can create more robust and adaptable smart contracts.
Remember, the exact implementation will depend on your specific use case. Always ensure your contracts are thoroughly tested and audited before deploying to mainnet.
Subscribe to my newsletter
Read articles from Yash Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
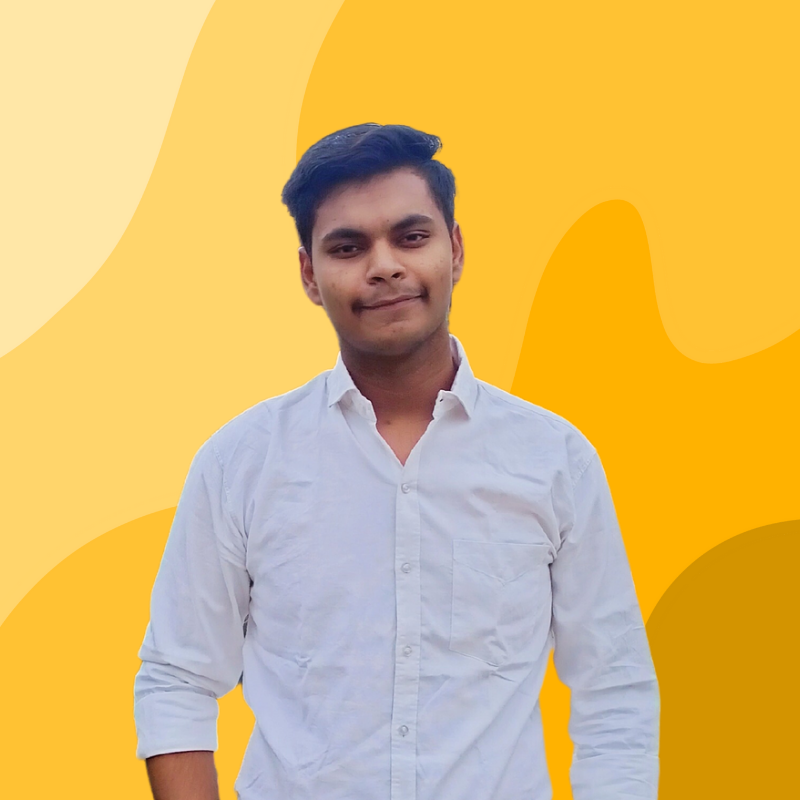
Yash Jain
Yash Jain
Blockchain Developer