Understanding Enums in Rust: A Beginner's Guide
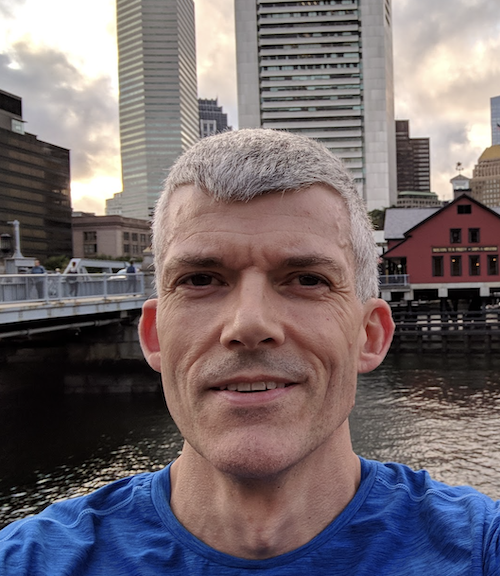
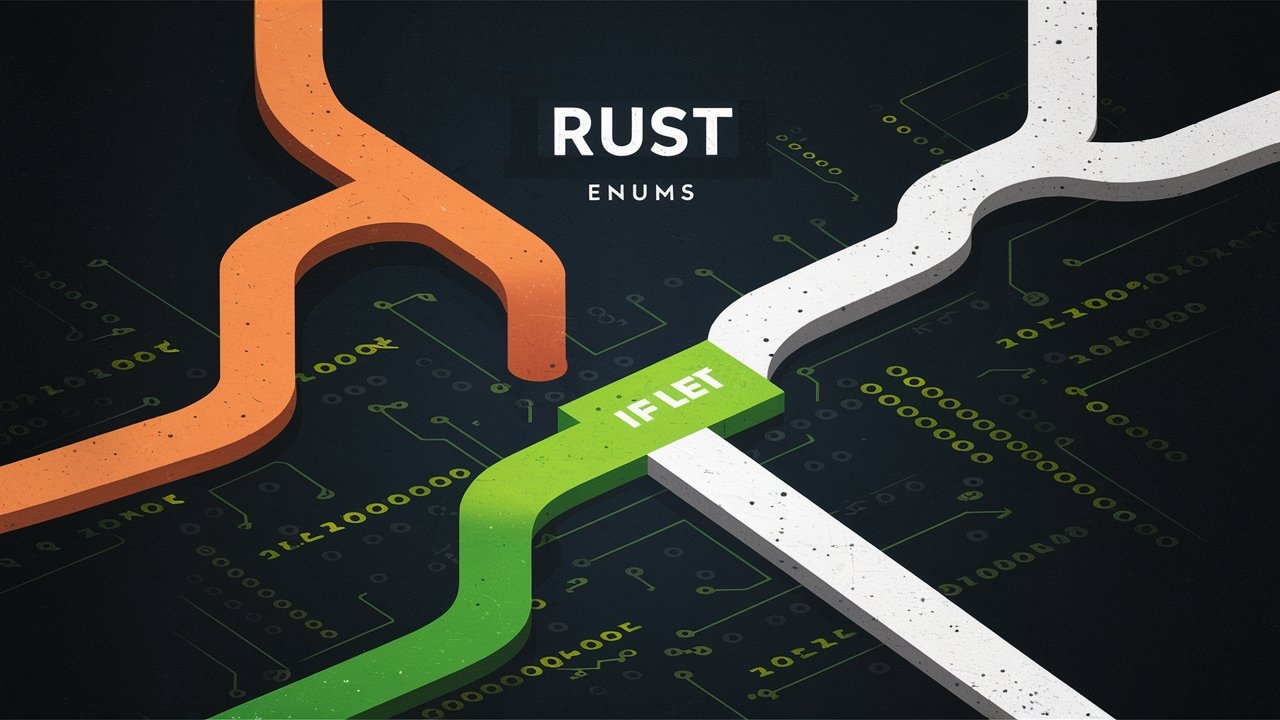
If you're new to Rust, you might have encountered enums and wondered what they're all about. In this post, we'll explore Rust enums, their uses, and how they can make your code more expressive and safer. We'll cover basic enums, enums with associated data, and some common patterns for using enums effectively.
What are Enums?
In Rust, an enum (short for enumeration) is a type that can have a fixed set of variants. Each variant represents a possible value for that type. Enums are particularly useful when you have a value that can be one of a few different things.
Let's start with a simple example:
enum Direction {
North,
South,
East,
West,
}
Here, we've defined an enum called Direction
with four variants. A value of type Direction
can only be one of these four variants at any given time.
Using Basic Enums
You can create a value of an enum type by using the enum name followed by two colons and the variant name:
fn describe_direction(direction: Direction) {
match direction {
Direction::North => println!("Heading north"),
Direction::South => println!("Heading south"),
Direction::East => println!("Heading east"),
Direction::West => println!("Heading west"),
}
}
describe_direction(Direction::East); // Outputs: Heading east
To illustrate the benefits of using enums, let's consider how we might represent directions without them:
// Representing direction without enums
const NORTH: u8 = 0;
const SOUTH: u8 = 1;
const EAST: u8 = 2;
const WEST: u8 = 3;
fn describe_direction_without_enum(direction: u8) {
match direction {
NORTH => println!("Heading north"),
SOUTH => println!("Heading south"),
EAST => println!("Heading east"),
WEST => println!("Heading west"),
_ => println!("Invalid direction"),
}
}
describe_direction_without_enum(EAST); // Outputs: Heading east
describe_direction_without_enum(5); // Outputs: Invalid direction
While this approach works, it has several drawbacks that enums solve:
Type Safety: The non-enum version uses
u8
to represent directions, allowing anyu8
value as input, even invalid ones. Enums ensure only valid Direction variants can be used.Readability: Enums make the code's intent clearer.
Direction::East
is more descriptive than a magic number like2
.Maintainability: Adding a new direction (like
NorthEast
) is simpler with enums— add a new variant, the compiler will inform you of allmatch
statements which need updating. Without enums, you'd need to add a new constant and remember to update all relevantmatch
statements.Namespace: Enums create their own namespace (
Direction::North
), avoiding potential naming conflicts with other constants.
By using enums, we leverage Rust's type system to create more robust, readable, and maintainable code. This example demonstrates why enums are often a superior choice for representing a fixed set of variants in Rust.
Enums with Associated Data
One of the powerful features of Rust enums is that variants can hold associated data. This allows you to attach additional information to each variant, making enums far more versatile than their counterparts in many other languages. Let's look at an example:
enum WebEvent {
PageLoad,
KeyPress(char),
Click { x: i64, y: i64 },
}
In this WebEvent
enum:
PageLoad
is a simple variant without any associated data, similar to our basicDirection
enum.KeyPress
holds a singlechar
value, representing which key was pressed.Click
holds a struct-like set of named fields, representing the coordinates of the click.
This is a significant step up from our basic Direction
enum. Here's how it differs:
Varied Data Types: Each variant can hold different types and amounts of data. This allows a single enum to represent a variety of related but distinct concepts.
Rich Information: Unlike the basic enum where each variant was just a marker, these variants carry meaningful data. A
KeyPress
tells us which key, and aClick
tells us where.Compact Representation: Instead of needing separate structs for each event type and an enum to distinguish between them, we combine everything into a single, expressive enum.
You can create values of these variants like this:
let event1 = WebEvent::PageLoad;
let event2 = WebEvent::KeyPress('c');
let event3 = WebEvent::Click { x: 10, y: 20 };
Working with these richer enums often involves pattern matching to extract the associated data:
fn handle_event(event: WebEvent) {
match event {
WebEvent::PageLoad => println!("Page loaded"),
WebEvent::KeyPress(c) => println!("Pressed key '{}'", c),
WebEvent::Click { x, y } => println!("Clicked at x={}, y={}", x, y),
}
}
handle_event(WebEvent::KeyPress('a')); // Outputs: Pressed key 'a'
handle_event(WebEvent::Click { x: 100, y: 200 }); // Outputs: Clicked at x=100, y=200
This pattern matching allows us to easily handle each variant differently and access its associated data.
The power of enums with associated data becomes even more apparent in real-world scenarios. For example, in a game, you might represent different power-ups:
enum PowerUp {
Health(u32),
Strength(f64),
Armor { defense: u32, weight: f64 },
}
fn apply_power_up(player: &mut Player, power_up: PowerUp) {
match power_up {
PowerUp::Health(amount) => player.heal(amount),
PowerUp::Strength(factor) => player.increase_strength(factor),
PowerUp::Armor { defense, weight } => {
player.increase_defense(defense);
player.decrease_speed(weight);
}
}
}
This approach allows for a flexible, type-safe way to represent and handle a variety of related concepts within a single type. It combines the benefits of enums (mutual exclusivity of variants) with the expressiveness of structs (ability to carry data), making it a powerful tool in Rust programming.
The if let
Syntax; a shortcut for a common pattern
While match
statements are powerful and require us to account for every variant of a given enum, there are times when we only care about one specific case and want to ignore all others. For these situations, Rust provides the if let
syntax. This concise alternative allows us to handle a single pattern matching case without the need to explicitly handle other cases. Let's explore how if let
can make our code more readable and focused.
Here's an example using match
as we have above.
/// given maybe_value is an Option<i32>
/// ex: let maybe_value: Option<i32> = Some(42);
// Equivalent match statement
match maybe_value {
Some(value) => println!("The value is: {}", value),
None => (), // We must handle this case, even if we don't care about it
}
Notice that in this context, we are only interested in the Some
variant of the Option
enum, but the compiler requires us to hand all variants, thus we essentially code a no-op for the None
variant (None => ()
).
using the if let
syntax we can make our code more concise:
// Using if let
if let Some(value) = maybe_value {
println!("The value is: {}", value);
}
In this example, the if let
syntax allows us to focus solely on the Some
case of our Option<i32>
. We don't need to provide any code for the None
case, which we do not care about in this context. The if let
syntax allows us to avoid writing boilerplate code for cases we're not interested in, which would be necessary with a match
statement.
Now, let's apply this pattern to our WebEvent
enum:
enum WebEvent {
PageLoad,
KeyPress(char),
Click { x: i64, y: i64 },
}
fn handle_input(event: WebEvent) {
if let WebEvent::KeyPress(c) = event {
if c.is_alphabetic() {
println!("Alphabetic key press: {}", c);
} else {
println!("Non-alphabetic key press: {}", c);
}
} else if let WebEvent::Click { x, y } = event {
if x < 100 && y < 100 {
println!("Click in top-left corner: ({}, {})", x, y);
} else {
println!("Click outside top-left corner: ({}, {})", x, y);
}
} else {
println!("Other event");
}
}
In this example, we're using if let
to handle specific cases of our WebEvent
enum:
We first check if the event is a
KeyPress
. If it is, we extract the character and perform further processing.If it's not a
KeyPress
, we then check if it's aClick
event. If it is, we extract the x and y coordinates and perform specific actions based on the click location.If it's neither a
KeyPress
nor aClick
, we handle it as "Other event".
This approach allows us to easily handle specific cases without needing to write out a full match
expression, which can be particularly useful when we're only interested in a subset of the possible enum variants.
Here's how we might use this in a real-world scenario:
fn process_user_input(events: Vec<WebEvent>) {
for event in events {
handle_input(event);
}
}
// Usage
let events = vec![
WebEvent::PageLoad,
WebEvent::KeyPress('a'),
WebEvent::Click { x: 20, y: 80 },
WebEvent::KeyPress('5'),
WebEvent::Click { x: 150, y: 200 },
];
process_user_input(events);
This would output:
Other event
Alphabetic key press: a
Click in top-left corner: (20, 80)
Non-alphabetic key press: 5
Click outside top-left corner: (150, 200)
The if let
syntax shines in scenarios like this where we want to handle specific enum variants differently, without the verbosity of a full match
expression. It's particularly useful in event handling systems, parsers, or any situation where you're working with enums that have many variants, but you only need to handle a few specific cases in a given context.
Conclusion
Why Use Enums?
Enums in Rust offer several benefits:
Type Safety: Enums help you express the idea that a value must be one of a fixed set of possibilities, which the compiler can check.
Expressiveness: Enums with associated data allow you to represent complex ideas in a clear, type-safe manner.
Pattern Matching: Rust's
match
expression works beautifully with enums, ensuring you handle all cases.
Enums in Rust are a powerful feature that allows you to express ideas clearly and safely. From simple sets of variants to complex associated data, enums provide a flexible way to model your domain. As you continue your Rust journey, you'll find enums becoming an essential tool in your programming toolkit.
Subscribe to my newsletter
Read articles from Sam Keen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
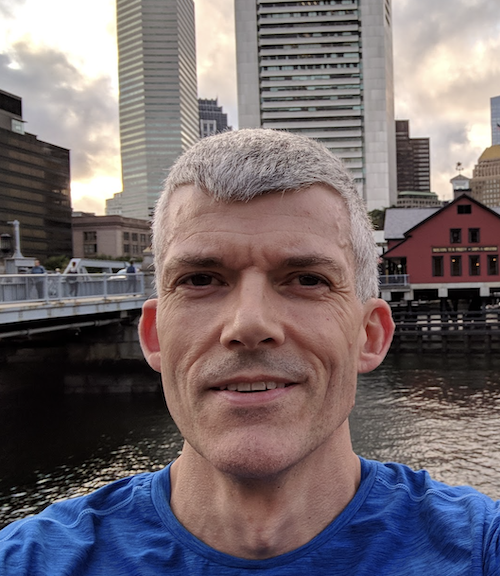
Sam Keen
Sam Keen
Experienced Software Development Leader | Expertise in Cloud Architecture | Spearheaded Projects at AWS, Lululemon, & Nike