How to Use If, Else, and Switch Statements in JavaScript
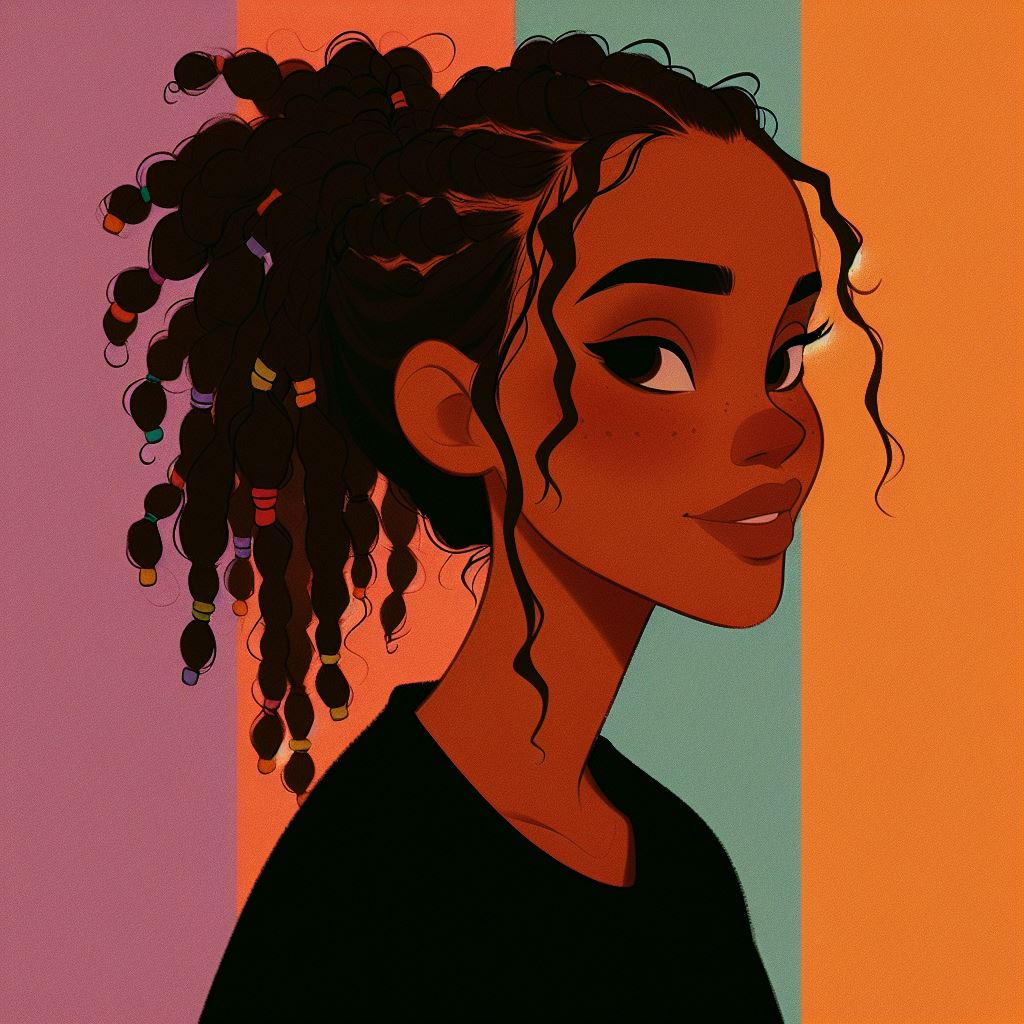
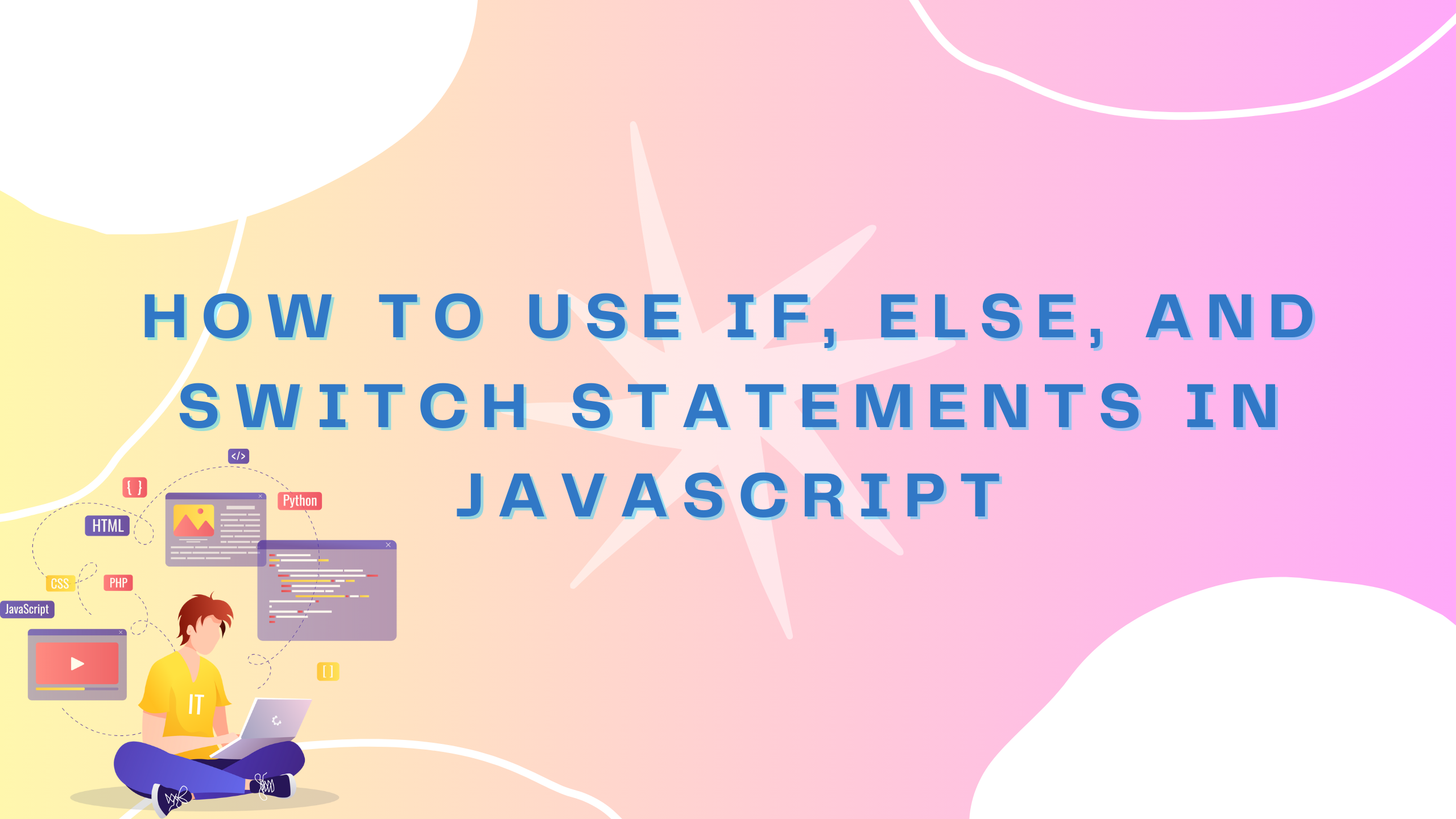
Introduction
In this tutorial, I will show you how to use two control structures in JavaScript: the if/else statement and the switch statement. ๐
By the end of this article, you'll be able to use both in your projects and understand control flow in JavaScript.
Prerequisites
- Nothing but a willingness to learn ๐
If Statement
By using the if statement, you can evaluate the value in the parentheses. If the value is true, the code block will run. If not, it does not.
For example, if you have a variable that holds a boolean value, let's use a dark mode toggle as an example.
let darkModeState = true;
You want to check if your darkModeState variable is true or false to make the right decision with your code.
This is what the if statement can do. It can answer the question and you can write code based on the answer (true/false) you get returned to you.
Let's continue and explore the syntax of the if statement.
if(darkModeState) {
console.log("Dark mode is active")
}
The syntax is:
if() {}
the parentheses () hold the condition you're checking.
the {} code block holds the code action to be run if the condition evaluates to true
You now have a simple conditional statement. The statement you have currently checks if ==darkModeState== is true, and if it is, will console.log a string "Dark mode is active".
You can also check if something is not true. You can do this by using the bang operator (!) which means "not equal to".
What this will do for your if statement is ask "Is darkModeState not equal to true".
Your question then becomes "Is darkModeState false".
if(!darkModeState) {
console.log("Light mode is active")
}
Your if statement only ever evaluates to true or false.
In the two code examples, I taught you how to use the if statement with a bang operator and without one.
Now I will teach you about the else statement, which adds an extra layer to your if statement and allows you to have more than one action if the condition evaluates to something other than what you expect.
Else statement
The else
statement functions alongside the if
statement. If the previous if statement evaluates as false, the else statement serves as a default case.
Let's say you have a conditional statement to verify if a user is signed in/registered for your service. You may have a piece of code that looks something like this:
let userLoggedIn = false;
const user = "Jake!";
if (loggedIn) {
console.log(`Hey, welcome back ${user}`);
} else {
console.log(`Hey stranger, good to meet you!`);
}
Your code has the userLoggedIn
variable as false. The if
statement failed to evaluate as true, so the default else
runs.
If you change the value of userLoggedIn
to true, then the else
statement will never run, because the execution of the conditional statement will end when it reads true.
This naturally brings us to the next option you have when using if/else
statements.
Imagine you have more than one conditional to check. You would use an if
and an else
as you just learned.
However, what if you had four conditions to check before considering a default case running?
That would be a job for the else if
statement.
Else If statement
The if else
statement allows you to check multiple conditions before you get to a final default with else
.
Let us look at an example. You have a birthday party coming up, and you have multiple venue options. For each option, you will wear something different.
Using this example, you can go through an example using if else
with this code:
let venue = "Paris"; //Change this to anything you like.
if (venue === "Paris") {
console.log("Yay! I will wear a sunny summer dress!");
} else if (venue === "Moscow") {
console.log("Chilly! I will wear a faux fur coat!");
} else {
console.log("I will wear jeans and a t-shirt!");
}
For this example, we would get: Yay! I will wear a sunny summer dress!
If you change the venue variable to Moscow, the correct console will run.
Now, in the event neither of the preceding statements evaluates to true, for example, if the venue was "Tokyo" then the else would run.
This makes your code very powerful. You now cannot only check if something is true and run a specific code. You can also check to see if a secondary option is true, and if you have a third, a third as well.
๐ก It is important to note that once your code evaluates to "true", it stops and does not execute any additional code.
This makes your code very powerful. It provides flexibility and allows you to have more than two simple conditional branches in your code.
Now you know how to use if/else
and else if
statements with JavaScript.
I would like to show you a second control structure.
The switch
statement.
The Switch statement
The switch
statement functions the same as the if/else
statement. It checks a condition, evaluates it to either true or false, and then runs some code.
The difference is the syntax. You will see in the code example that the switch statement looks better when you have multiple conditions to check.
//Switch statement
const day = "Thursday";
switch (day) {
case "Monday":
alert("Monday Funday, rise and shine! A new week begins");
break;
case "Tuesday":
alert("Tuesday fools day! Go take a walk!");
break;
case "Wednesday":
alert("Wednesday! Take a half day?");
break;
case "Thursday":
alert("ITS THURSDAY I SHOULD NOT BE THIS HAPPY!");
break;
case "Friday":
alert("Ah! Friday my sweet. I need a nap!");
break;
default:
alert("Its a weekend, nobody cares which day! Bye");
break;
}
In this example, the condition in parentheses is used to check the case. If the value is true, the code after the case will run. If not, the code will move on to the next case.
In the event of a true value, the code will execute and the code will then break and end the conditional checks.
The other code will cease to be checked upon finding a true value.
The switch
syntax is:
switch(condition) {
case "condition":
break;
default:
break;
}
The switch statement checks against the condition using cases and the break keyword is necessary to exit the code block after the case is evaluated.
๐ก Remember: The break keyword is not optional. If you omit the break keyword, your switch will never break out of the case being checked and will continue to run and, in the end, run the final case/default case.
You can now see that the switch
is a powerful control structure that allows you to check multiple possible cases and run different codes depending on which is true.
This structure can sometimes be a neater and more readable way to write conditional statements.
With that, you have learned multiple ways to run conditional logic in your code. ๐๐
Conclusion
In this article, you learned about the if
, if/else
, else
and switch
statements.
You learned how to use these conditional statements to run different code based on certain conditions.
This will allow you to create more flexible code in the future.
I hope this was helpful. โจ
Subscribe to my newsletter
Read articles from Kirsty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
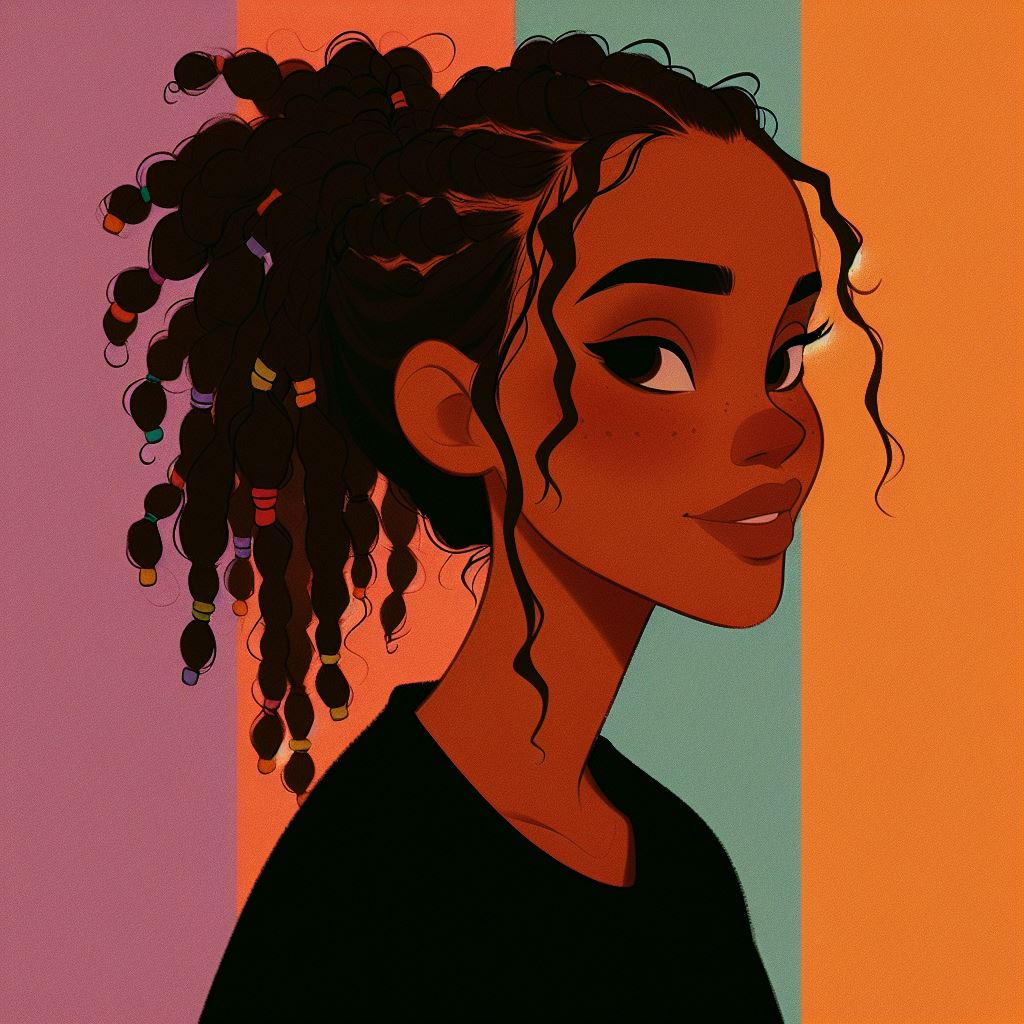
Kirsty
Kirsty
Hi, Iโm Kirsten, a passionate web developer. Iโm using JavaScript, React, and more to build impactful projects. After 2 years of learning through trial and error, Iโm ready to share my journey and create work that solves problems. ๐ฌ Connect with me: Twitter: twitter.com/km_fsdev Blog: kirsty.hashnode.dev GitHub: github.com/ofthewildire