[Rust] Variables part 4

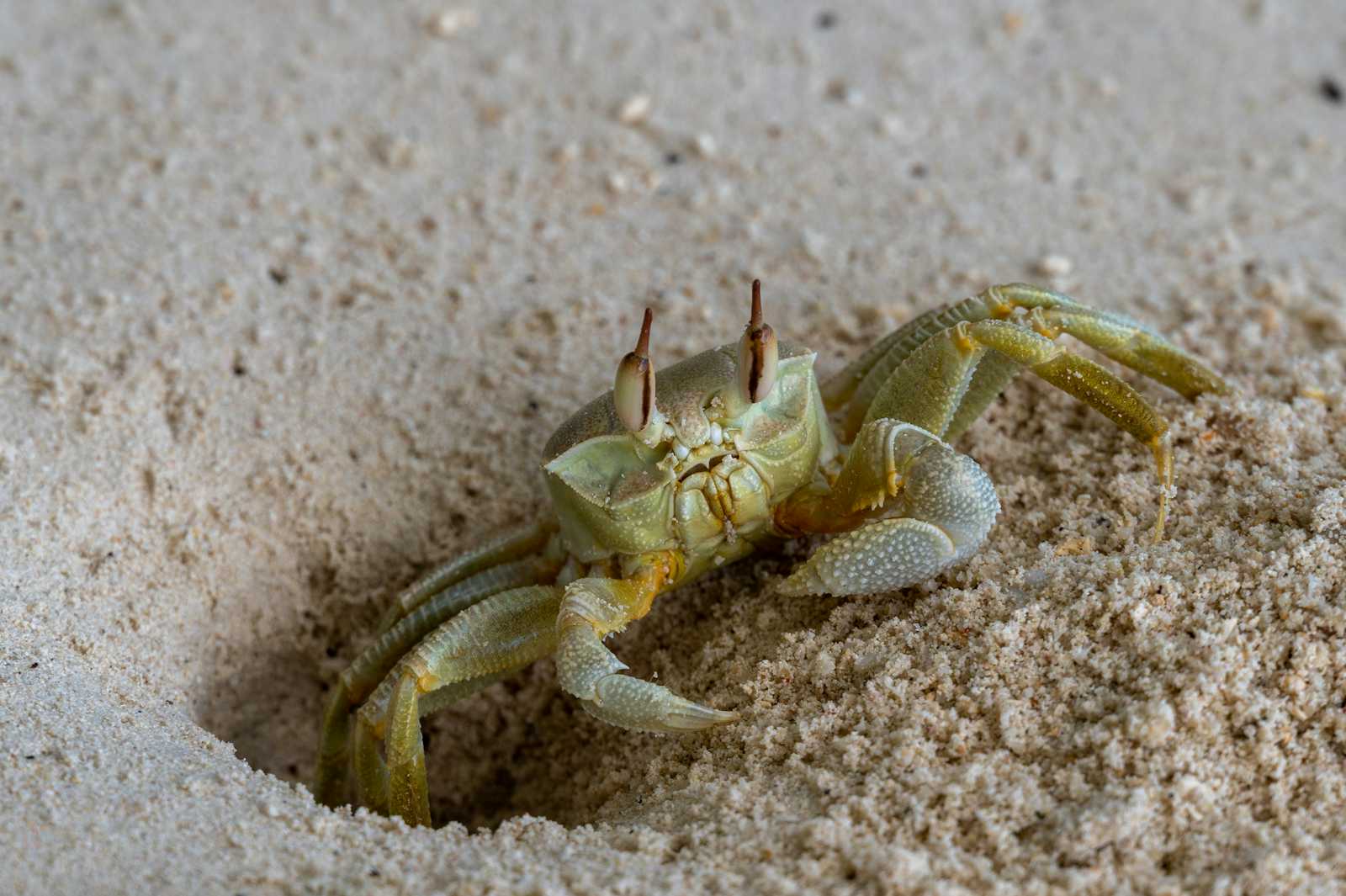
A variable is a named storage that a program can manipulate.Simply put, variables help programs store values.Variables in Rust are associated with a specific data type.The data type determines the size and layout of the variable's memory, the range of values that can be stored in that memory, and the set of operations that can be performed on the variable.
Variable naming conventions
Variable names consist of letters, numbers, and underscore characters
Must start with a letter or underscore
Case-sensitive
Syntax
The data type is optional while declaring a variable.
By default, the data type is inferred from the value assigned to the variable.
let variable_name = value; // no type specified
let variable_name:dataType = value; //type specified
fn main() {
let fees = 25_000;
let salary:f64 = 35_000.00;
println!("fees is {} and salary is {}",fees,salary);
}
Immutable
Rust에서는 변수는 기본적으로 읽기 전용입니다.
즉, 값이 변수 이름에 바인딩되면 변수의 값을 변경할 수 없습니다.
Rust가 프로그래머가 코드를 작성하고 안전하고 쉬운 동시성을 활용할 수 있도록 하는 많은 방법 중 하나입니다.
fn main() {
let fees = 25_000;
println!("fees is {} ",fees);
fees = 35_000;
println!("fees changed is {}",fees);
}
Mutable
Variable names can be mutable by appending the mut keyword to them
fn main() {
let mut fees: i32 = 25_000;
println!("fees is {} ", fees);
fees = 35_000;
println!("fees changed is {}", fees);
}
Subscribe to my newsletter
Read articles from Harold Lippin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Harold Lippin
Harold Lippin
Hansei Cyber Security High Schoool (2022/3/2 ~ 2025/2/10)