Greedy Algorithm - Assign Cookies
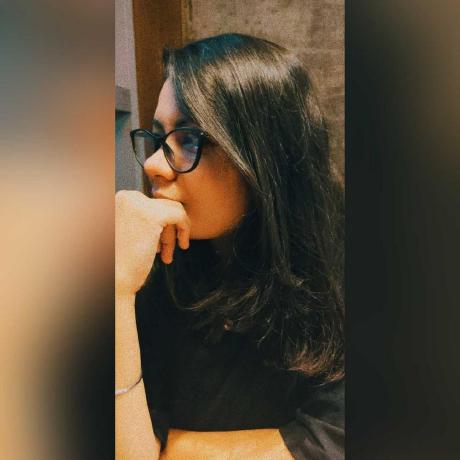
Assume you are an awesome parent and want to give your children some cookies. But, you should give each child at most one cookie.
Each child i
has a greed factor g[i]
, which is the minimum size of a cookie that the child will be content with; and each cookie j
has a size s[j]
. If s[j] >= g[i]
, we can assign the cookie j
to the child i
, and the child i
will be content. Your goal is to maximise the number of your content children and output the maximum number.
Example 1:
Input: g = [1,2,3], s = [1,1]
Output: 1
Explanation: You have 3 children and 2 cookies. The greed factors of 3 children are 1, 2, 3.
And even though you have 2 cookies, since their size is both 1, you could only make the child whose greed factor is 1 content.
You need to output 1.
Example 2:
Input: g = [1,2], s = [1,2,3]
Output: 2
Explanation: You have 2 children and 3 cookies. The greed factors of 2 children are 1, 2.
You have 3 cookies and their sizes are big enough to gratify all of the children,
You need to output 2.
Solution:
/**
* @param {number[]} g - Greed factors of children
* @param {number[]} s - Sizes of cookies
* @return {number} - Maximum number of content children
*/
var findContentChildren = function(g, s) {
// Sort greed factors and cookie sizes
g.sort((a, b) => a - b);
s.sort((a, b) => a - b);
// Initialize indices for greed factors and cookie sizes
let i = 0; // Index for greed factors
let j = 0; // Index for cookie sizes
// Iterate over both arrays
while (i < g.length && j < s.length) {
if (s[j] >= g[i]) { // If the cookie can satisfy the child
i++; // Move to the next child
}
j++; // Move to the next cookie
}
return i; // The number of content children
};
Time Complexity: O(N log N + M log M + M) - 2 sort functions and a while loop
Space Complexity: O(1) because we are not occupying and auxiliary space
Note: Try to greedily assign the smallest possible value to maximise the output.
Hope this helps!
Subscribe to my newsletter
Read articles from Palak Bansal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
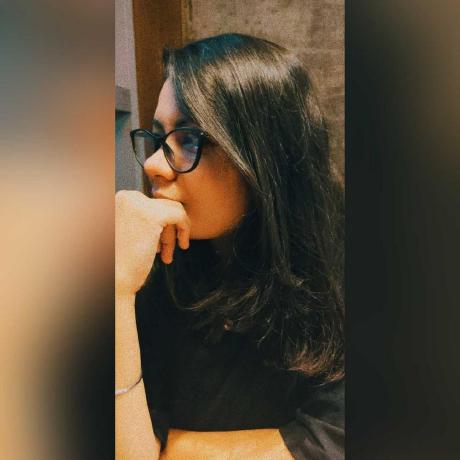
Palak Bansal
Palak Bansal
Experienced Frontend Developer with a demonstrated history of working in the financial services industry along with having 5+ years of hands on professional experience efficiently coding websites and applications using modern HTML, CSS and Javascript along with their respective frameworks viz.Vue.JS, React.JS. Instituting new technologies and building easy to use and user-friendly websites is truly a passion of mine. I actively seek out new libraries and stay up-to-date on industry trends and advancements. Translated designs to frontend code along with streamlining existing code to improve site performance. Collaborated with UX designers and Back End Developers to ensure coherence between all parties. Also tested some feature prototypes for bugs and user experience.