Understanding the UUID Replacement and Configuration File Generator Script
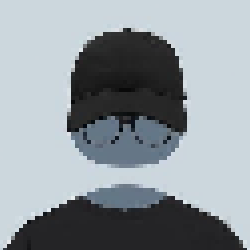
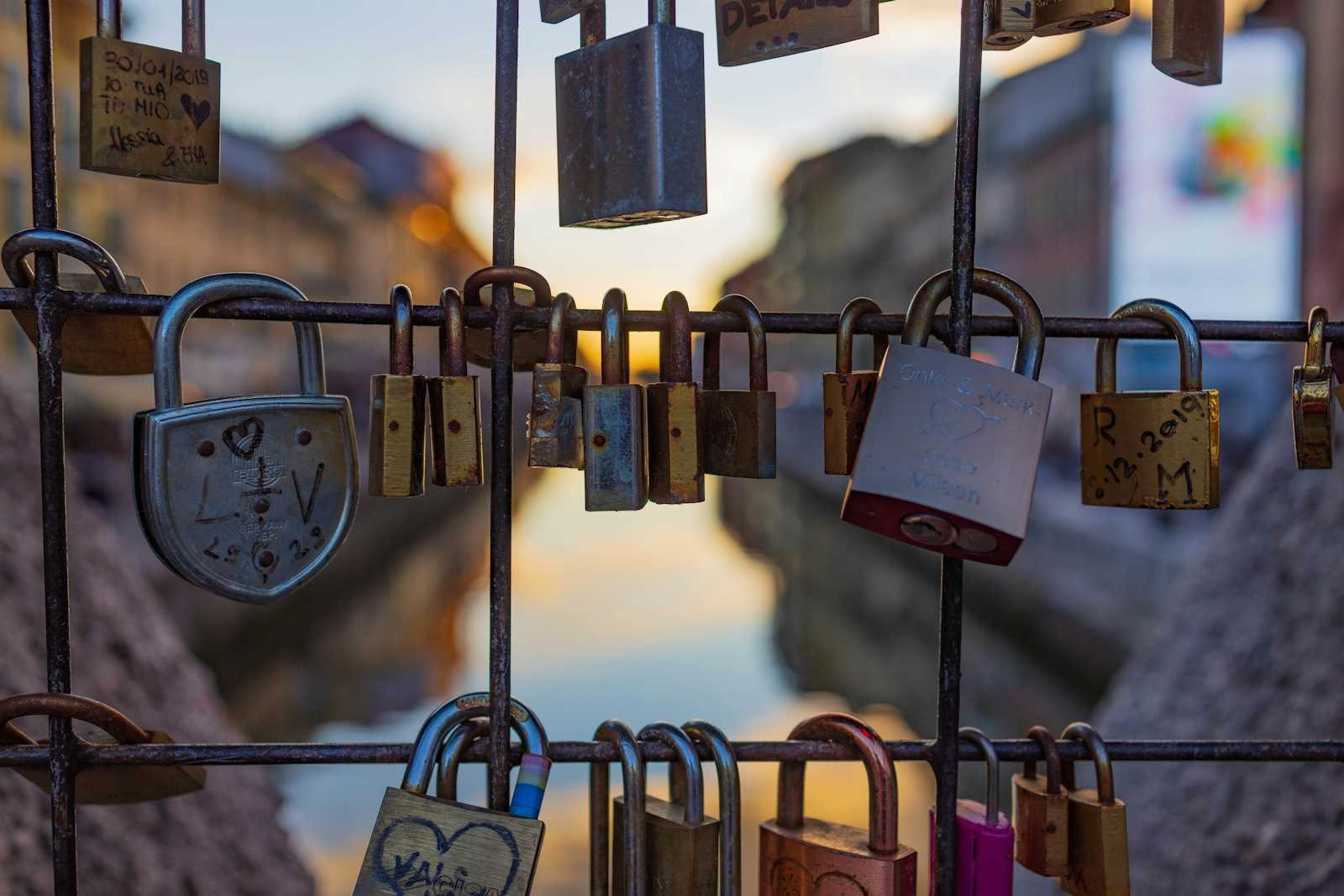
Introduction
This technical article delves into the workings of a Python script designed to generate configuration files with unique UUIDs. The script also allows customization of network and proxy settings, clears the directory of all files, and creates a zip file containing the generated configuration files. Here's a detailed explanation of each part of the code and how it works.
Step-by-Step Code Explanation
Step 1: Uninstall Conflicting Packages
!pip uninstall -y pathlib
The script starts by uninstalling any existing pathlib
package to avoid conflicts. The -y
flag automatically confirms the uninstallation.
Step 2: Install Necessary Libraries
!pip install pyinstaller
It then installs pyinstaller
, a package used to convert Python scripts into standalone executables.
Step 3: Clear the Directory
import os
import shutil
def clear_directory(path='.'):
for root, dirs, files in os.walk(path, topdown=False):
for name in files:
os.remove(os.path.join(root, name))
for name in dirs:
shutil.rmtree(os.path.join(root, name))
clear_directory()
This function, clear_directory
, removes all files and directories in the specified path (current directory by default). It uses os.walk
to traverse the directory tree from the bottom up, deleting files and directories.
Step 4: Generate a New UUID
import uuid
def generate_new_uuid():
new_uuid = str(uuid.uuid4())
print(f"UUID Generator Output: {new_uuid}")
return new_uuid
The generate_new_uuid
function uses the uuid
library to create a new UUID and returns it as a string. It also prints the generated UUID for verification.
Step 5: Replace UUID in Template Content
def replace_uuid_in_file(template_content, new_uuid):
return template_content.replace('aa9c5023-3f0e-4d0f-904b-5fbc5da0c9bd', new_uuid.strip())
This function, replace_uuid_in_file
, takes the template content and a new UUID as inputs. It replaces the placeholder UUID (aa9c5023-3f0e-4d0f-904b-5fbc5da0c9bd
) in the template content with the new UUID.
Step 6: Define the Configuration Template
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<!-- Configuration details here -->
</dict>
</plist>
The template_content
variable holds the XML configuration template. This template includes placeholders for UUIDs that will be replaced by the script.
Step 7: Network Configurations
network_configs = {
"All Network": {},
"Hamrah Aval": {},
"Irancel": {},
"Rightel": {},
}
A dictionary, network_configs
, defines different network configurations. Each key represents a network type, and the values can hold specific settings for that network.
Step 8: User Configuration Selection
print("Please select a configuration:")
for index, config_name in enumerate(network_configs.keys(), start=1):
print(f"{index}. {config_name}")
selected_index = int(input("Enter the number of your desired configuration: "))
selected_config_name = list(network_configs.keys())[selected_index - 1]
selected_config = network_configs[selected_config_name]
The script prompts the user to select a network configuration from the network_configs
dictionary. It then retrieves the chosen configuration based on user input.
Step 9: Proxy Settings
need_proxy = input("Do you need proxy settings? (yes/no): ").strip().lower()
if need_proxy == "yes":
proxy_server = input("Please enter the proxy server address: ")
proxy_port = int(input("Please enter the proxy port: "))
else:
proxy_server = ""
proxy_port = 0
The script asks the user if they need proxy settings. If the user responds with "yes", it prompts for the proxy server address and port. Otherwise, it sets empty values for these settings.
Step 10: Generate Unique IDs
unique_ids = [str(uuid.uuid4()) for _ in range(5)]
It generates a list of five unique UUIDs using a list comprehension and the uuid4
function from the uuid
library.
Step 11: Add Unique IDs to Configuration
selected_config["UniqueIDs"] = unique_ids
The script adds the list of unique IDs to the selected configuration dictionary.
Step 12: Add Proxy Settings to Configuration
if need_proxy == "yes":
selected_config["ProxyServer"] = proxy_server
selected_config["ProxyPort"] = proxy_port
If proxy settings were provided by the user, they are added to the selected configuration dictionary.
Step 13: Display Final Configuration
print("\\nFinal configuration:")
print(selected_config)
The final configuration, including unique IDs and proxy settings, is printed for user verification.
Step 14: Custom Profile Name
custom_name = input("Enter the custom name for the generated profiles: ")
The script prompts the user to enter a custom name for the generated profiles.
Step 15: List of Template Files
templates = [
"Alloprators-template.mobileconfig",
"HamrahAval-template.mobileconfig",
"Irancell-template.mobileconfig",
"irancell+mci.mobileconfig",
"Rightel-template.mobileconfig"
]
A list of template file names is defined, representing different network operator configurations.
Step 16: Process Each Template
output_files = []
for template in templates:
new_uuid = generate_new_uuid()
output_content = replace_uuid_in_file(template_content, new_uuid)
output_path = f"{custom_name}-{template}"
with open(output_path, 'w') as f:
f.write(output_content)
output_files.append(output_path)
The script processes each template file:
Generates a new UUID.
Replaces the placeholder UUID in the template content with the new UUID.
Writes the modified content to a new file with a name based on the custom name and template name.
Adds the new file path to the
output_files
list.
Step 17: Print Generated Files
print("\\nGenerated configuration files:")
for output_file in output_files:
print(output_file)
The script prints the paths of the generated configuration files.
Step 18: Create Zip File
import zipfile
zip_filename = f"{custom_name}_profiles.zip"
with zipfile.ZipFile(zip_filename, 'w') as zipf:
for output_file in output_files:
zipf.write(output_file)
The script creates a zip file containing all the generated configuration files.
Step 19: Trigger File Download
from google.colab import files
files.download(zip_filename)
Finally, the script triggers the download of the zip file in the Google Colab interface.
Conclusion
This script automates the process of generating unique configuration files with customizable settings, making it easier for users to manage network and proxy settings on their devices. Each step of the script is designed to ensure that the generated profiles are unique and properly configured based on user input.
Subscribe to my newsletter
Read articles from TheMehranKhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
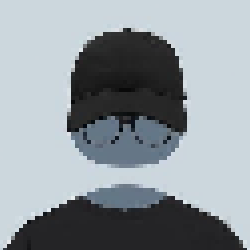
TheMehranKhan
TheMehranKhan
I'm Mehran, a software developer and digital nomad who traded my 9-to-5 job for a life of remote work and global adventures, sharing my experiences and tips on themehrankhan.site