Building a Simple Machine Learning Model: A Step-by-Step Guide
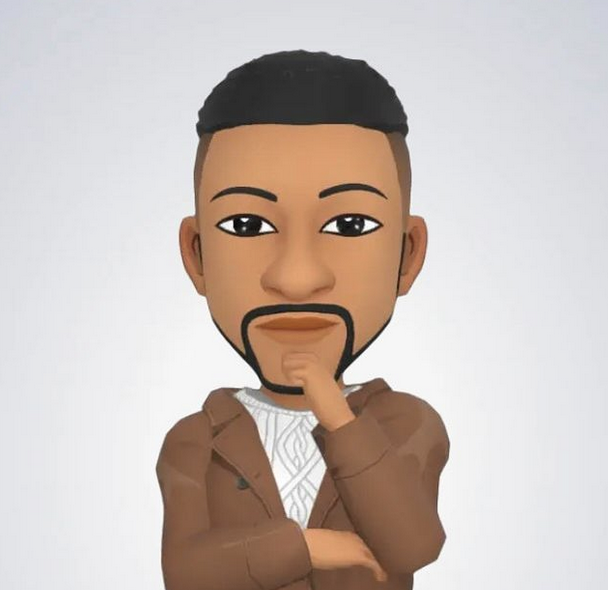
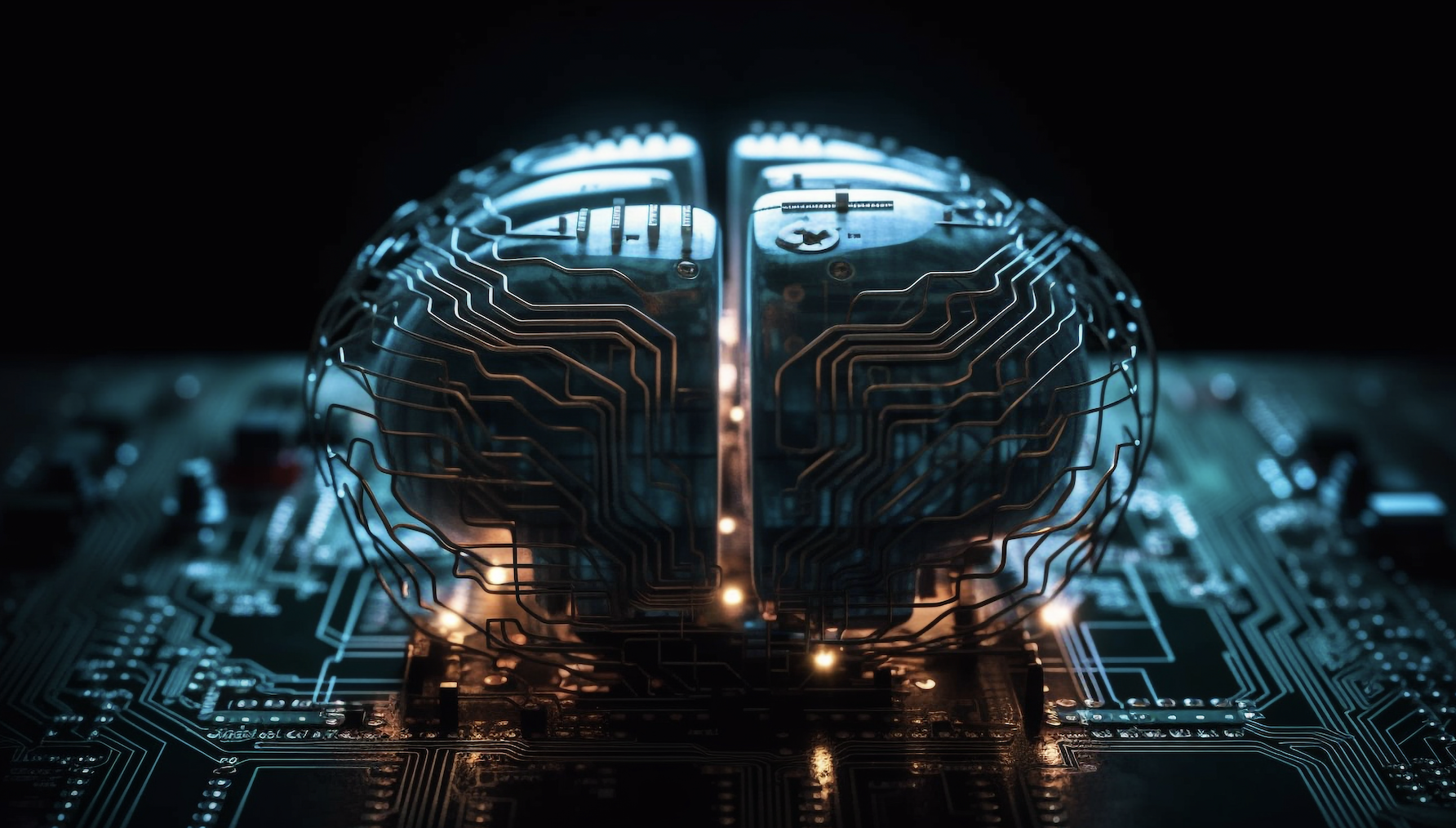
Machine learning, a revolutionary tool in data interpretation, is transforming how we handle and interpret data. It's the driving force behind many modern applications, from predicting stock prices to recommending products. In this guide, we will walk through the process of building a simple machine-learning model using Python, a skill that can be applied to a wide range of practical scenarios. We'll use the popular Scikit-learn library and the Iris dataset to demonstrate the steps in creating, training, and evaluating a machine-learning model.
Step 1: Setting Up the Environment
Before we begin, we must set up our Python environment with the necessary libraries. For this tutorial, we will be using Jupyter Notebook. You can install the required libraries using pip.
pip install numpy pandas scikit-learn matplotlib
Step 2: Understanding the Dataset
The Iris dataset is a well-known dataset used to benchmark machine learning algorithms. It consists of 150 iris flowers, each with four features: sepal length, sepal width, petal length, and petal width. The objective is to classify the flowers into three species: setosa, versicolor, and virginica.
Now, let's load and explore the dataset.
import pandas as pd
from sklearn.datasets import load_iris
# Load the dataset
iris = load_iris()
df = pd.DataFrame(data=iris.data, columns=iris.feature_names)
df['species'] = iris.target
# Display the first few rows
print(df.head())
Step 3: Data Preprocessing
Data preprocessing is a critical step in creating a machine-learning model. It includes cleaning and transforming the data to prepare it for training. This may involve addressing missing values, scaling the features, and dividing the data into training and testing sets.
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# Split the data into features and target
X = df.drop('species', axis=1)
y = df['species']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Scale the features
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
Step 4: Choosing and Training the Model
Let's begin with a straightforward yet effective algorithm: the Decision Tree classifier. Decision Trees are supervised learning algorithms that can handle classification and regression tasks. The model functions by dividing the dataset into subsets based on the input features' values. This process is repeated recursively, forming a tree-like structure of decisions. What is a Decision Tree?
A Decision Tree consists of nodes, branches, and leaves:
Nodes represent a feature (or attribute) in the dataset.
Branches represent a decision rule or condition based on the feature.
Leaves represent the outcome or class label.
The tree begins with a root node and then divides into branches based on feature values. Each inner node corresponds to a decision based on an attribute, and each leaf node represents a class label or a continuous value. The objective is to create a model that predicts the target variable by learning straightforward decision rules derived from the data features.
Real-Life Example
Consider a real-life example of a Decision Tree in action: a healthcare application predicting whether a patient has a particular disease based on symptoms and medical test results. The decision tree might use features like age, blood pressure, cholesterol levels, and family history to make predictions.
Root Node: The root node might ask whether the patient's age is above a certain threshold.
Branching: If yes, the tree branches into another decision node, asking if the patient has high blood pressure.
Leaves: Ultimately, the leaves will predict the likelihood of having the disease based on the answers to these questions.
This hierarchical structure makes Decision Trees easy to understand and interpret, as the decisions at each node are clear and intuitive.
Training a Decision Tree Classifier
Let's see how we can implement a Decision Tree classifier using Python's Scikit-learn library. For this example, we'll use the Iris dataset.
from sklearn.tree import DecisionTreeClassifier
# Initialise the model
model = DecisionTreeClassifier()
# Train the model
model.fit(X_train, y_train)
In this code, we initialise the DecisionTreeClassifier
from Scikit-learn and train it on our preprocessed training data (X_train
and y_train
). The model learns to classify the Iris species based on the features provided.
With the Decision Tree model trained, we can evaluate its performance on the test data.
Step 5: Evaluating the Model
After training the model, we must evaluate its performance on the test data. We'll use metrics such as accuracy, precision, and recall to assess the model's performance.
from sklearn.metrics import accuracy_score, classification_report
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
report = classification_report(y_test, y_pred)
print(f'Accuracy: {accuracy}')
print('Classification Report:')
print(report)
Step 6: Improving the Model
There are various ways to improve a machine learning model. We can tune the hyperparameters, try different algorithms, or even ensemble multiple models. Here, we'll demonstrate hyperparameter tuning using GridSearchCV.
from sklearn.model_selection import GridSearchCV
# Define the parameter grid
param_grid = {
'criterion': ['gini', 'entropy'],
'max_depth': [None, 10, 20, 30],
'min_samples_split': [2, 10, 20],
}
# Initialise the GridSearchCV
grid_search = GridSearchCV(estimator=model, param_grid=param_grid, cv=5)
# Train the model
grid_search.fit(X_train, y_train)
# Best parameters
print(f'Best parameters: {grid_search.best_params_}')
# Evaluate the best model
best_model = grid_search.best_estimator_
y_pred = best_model.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
report = classification_report(y_test, y_pred)
print(f'Accuracy after tuning: {accuracy}')
print('Classification Report after tuning:')
print(report)
Conclusion
In this guide, we have created a simple machine-learning model using Python and Scikit-learn. We have also covered data preprocessing, model training, evaluation, and improvement. This is just the start; there are numerous possibilities and more complex models to explore. Experiment with different datasets and algorithms to deepen your understanding of machine learning. Feel free to share your experiments and insights in the comments below!
Subscribe to my newsletter
Read articles from TemiTope Kayode directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
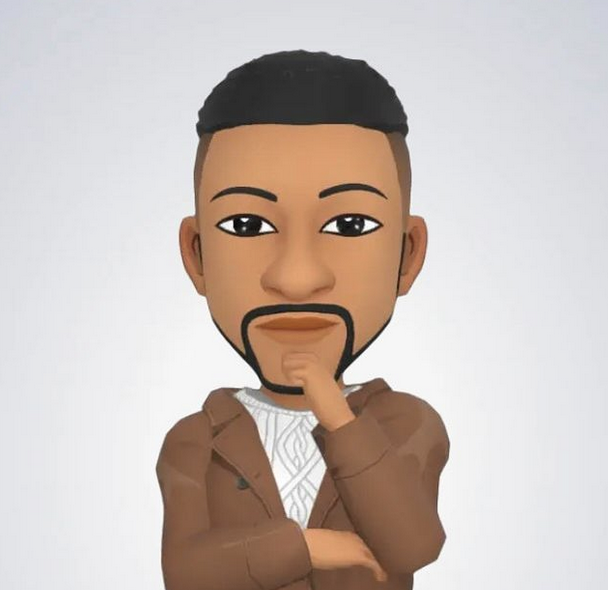
TemiTope Kayode
TemiTope Kayode
Seasoned software engineer specialized in web and mobile applications, cloud computing, and DevOps using tools like Django, React, Flutter, AWS, and DigitalOcean. Currently a Senior Software Developer and an avid tutor, I balance coding with family and leisure. Holds a distinction in a Masters in Computer Science from Coventry University, blending education with practical prowess. Passionate about technology and innovation, eager to connect and explore new possibilities.