Let's Create a Basic HTTP Server in Go (Golang)
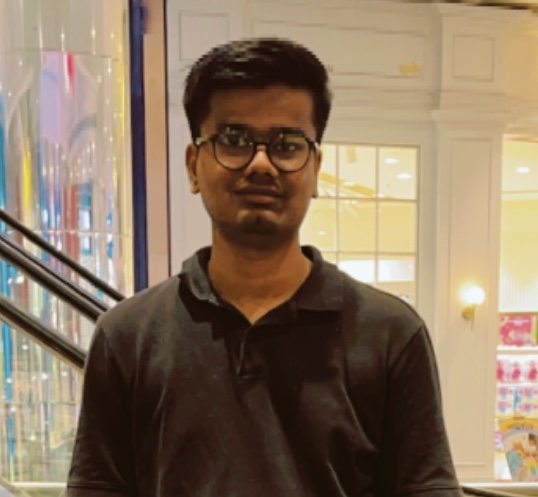
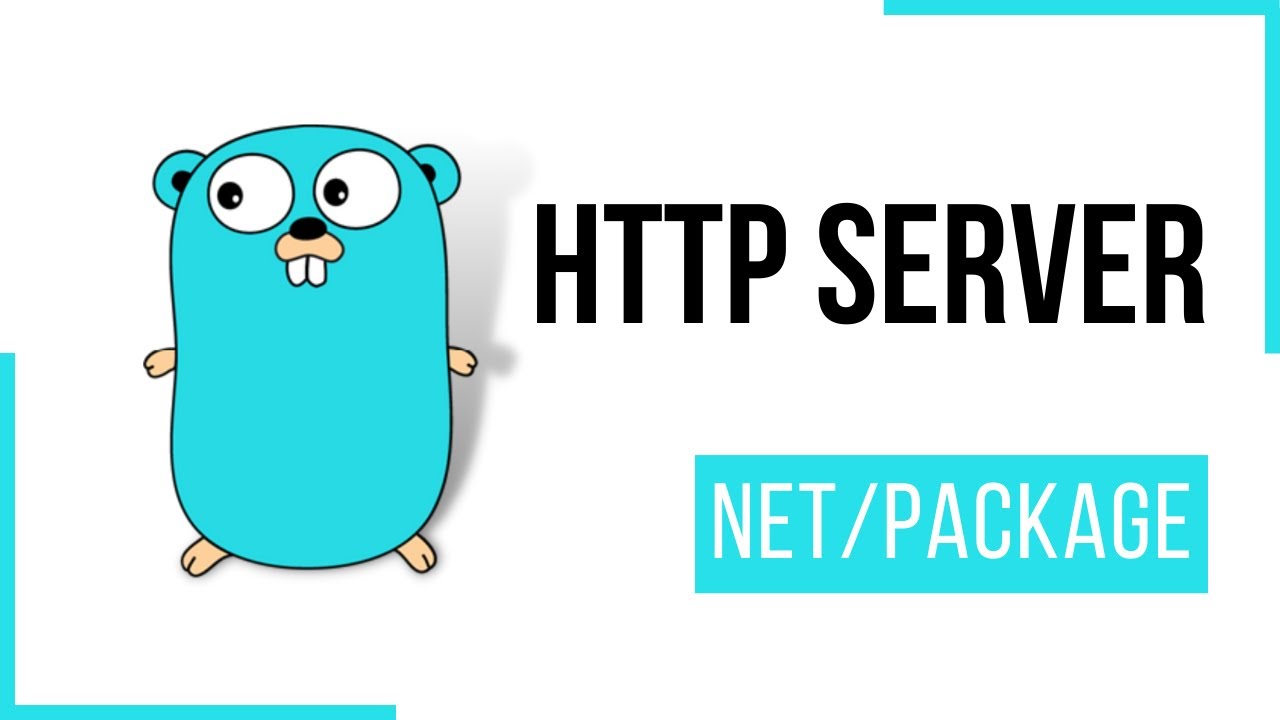
As a JavaScript developer diving into Go (Golang), understanding how to build an HTTP server is crucial for efficiently handling web requests and responses. In this tutorial, we'll guide you through the process of setting up a simple HTTP server using Go, exploring the net/http
package and its core components.
Prerequisites
Before we begin, ensure you have Go installed on your system. If not, download it from golang.org and follow the installation instructions for your operating system.
Step-by-Step Guide
Step 1: Setting Up Your Project
- Create a new directory for your Go project. Inside this directory, create a file named
main.go
.
Step 2: Writing the Go Code
- Open
main.go
in your preferred text editor and add the following code:
Understanding the Code
Package Declaration (package main
)
Every Go file starts with a package declaration. main
indicates that this file will compile into an executable program.
Imports (import "fmt"
and import "net/http"
)
Imports necessary packages:
fmt
: Provides formatted I/O operations.net/http
: Provides HTTP client and server implementations.
Handler Function (func handler(w http.ResponseWriter, r *http.Request)
)
Defines a function handler
that takes two parameters:
w http.ResponseWriter
: Allows constructing and sending an HTTP response back to the client.r *http.Request
: Represents the HTTP request received from the client, containing request details like method, headers, and URL parameters.
Inside handler
:
- Uses
fmt.Fprintf(w, "Hello from GO server!")
to write "Hello from GO server!" as the response body tow
, which sends it back to the client.
Main Function (func main()
)
The entry point of the program:
http.HandleFunc("/", handler)
: Registers thehandler
function to respond to requests to the root URL ("/"
).http.ListenAndServe(":8080", nil)
: Starts the HTTP server on port8080
. If an error occurs during startup, it's printed to the console.
Running Your Go Server
To run the server:
Save
main.go
.Open a terminal or command prompt.
Navigate to the directory where
main.go
is saved.Run
go run main.go
.
You should see 'Server listening on port 8080' in the console, indicating your server is running. And boom! You can access your server now on port 8080.
Conclusion
Congratulations! You've successfully created a basic HTTP server in Go. This example demonstrates the foundational aspects of handling HTTP requests and responses using Go's standard library. Next steps involve exploring middleware, handling different HTTP methods, and integrating with databases or external APIs to build robust web applications.
Learn More
For a deeper dive into Go's HTTP server capabilities, including advanced routing, middleware implementation, and scaling for production environments, explore additional resources and tutorials. Happy coding with Go!
Subscribe to my newsletter
Read articles from Yash Thakur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
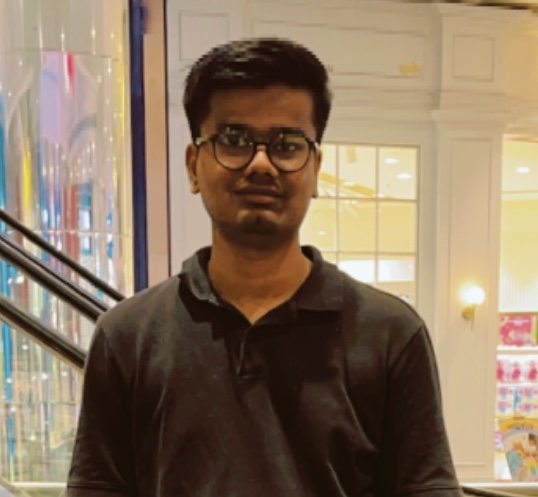