Python's Built In Modules: The OS Module

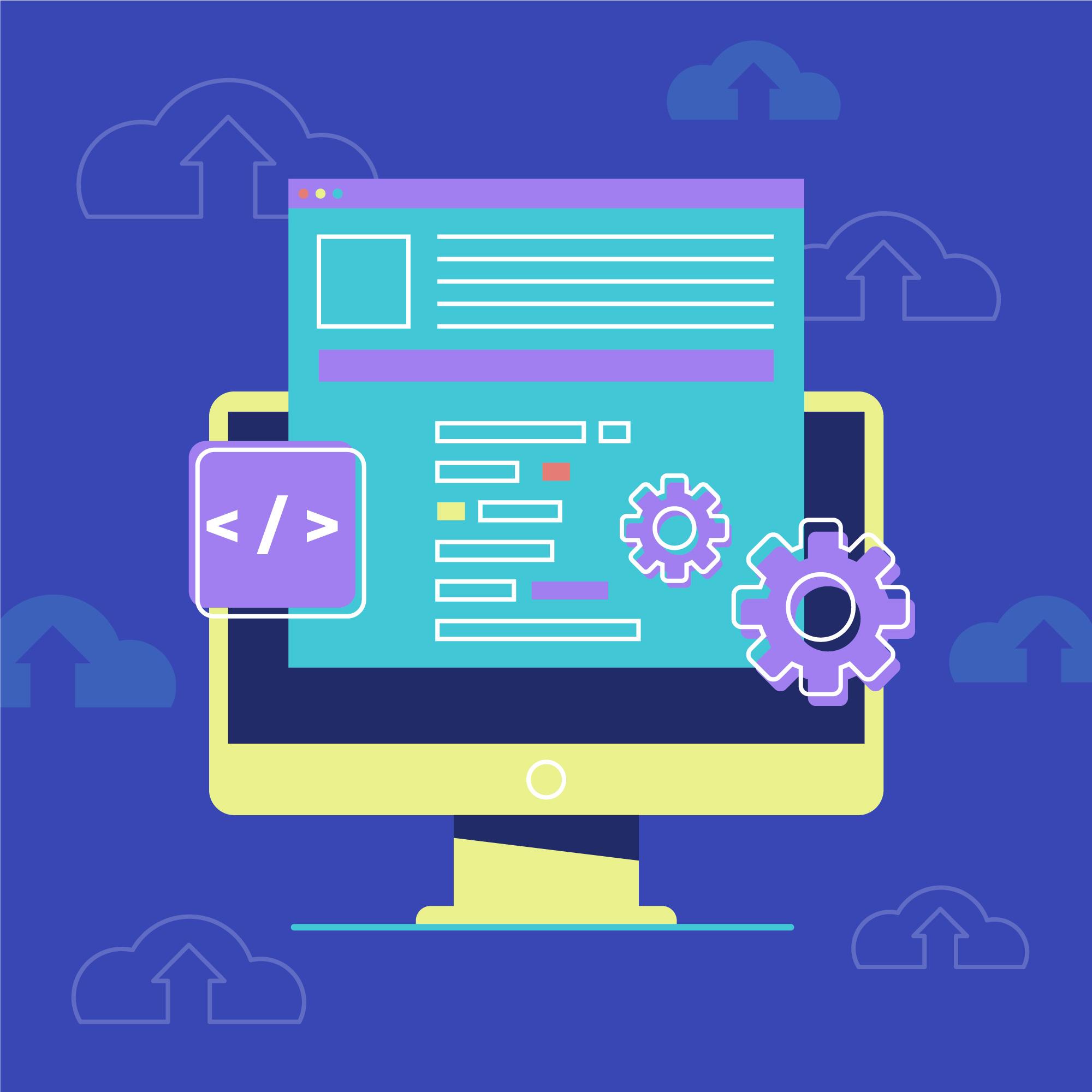
Welcome to another bitesize episode of the built-in Python module series. The last time we had a look at the sys module and now we'd be looking at something somewhat similar known as the operating system (os) module. A major difference between the two is that while the os module is primarily concerned with file paths and directories as well as their operations, the sys module is more about runtime operations, such as working with the command line like we saw in my previous post. If you’d like to know some way to interact with the file system of your computer you may want to try the os module. With this module you can perform several operating system tasks such as opening files and folders and creating new directories as well as hacking systems…well…not exactly that last one. You can use this module by simply importing it:
import os
Let’s have a look at some of the methods used in this module .
getcwd()
You use this when you want to know what directory you are currently working on. Getcwd stands for ‘get current working directory’.
import os
print(os.getcwd())
mkdir()
This stands for ‘make directory’ and simply means creating a new directory.
import os
os.mkdir('hackNasaSecrets')
Unless otherwise specified, you’d normally have the directory created in your current working directory. If I were to run that code again, I'd get my terminal shouting at me with errors because the directory would already exist.
listdir()
Use this if you’d like to list all files and directories in the current working directory. That is however, the default and you could list files and directory in a different directory if specified.
import os
print(os.listdir('./otherSecrets')
In this directory, I found these files:
['codeThatCouldSaveTheWorld.txt', 'coolDataStuff.txt']
open(), read() and close()
If you want to open a file with python programmatically, you use this method. Let's try opening the codeThatCouldSaveTheWorld.txt and see what we find using the code below;
import os
myFile = os.open('./otherSecrets/codeThatCouldSaveTheWorld.txt', os.O_RDONLY)
data = os.read(myFile,1000)
print(data.decode('utf-8'))
os.close(myFile)
This prints out the following:
43494de455ef73d34aeebd043ea47e4aa5b916f6caba899f142b8021eb2270c255100
a55883649365e412e2fd382edc40c427ad22571410eb2ea77c1b17cb64f285170ae76
39d75d869a450eb18eb0d50d42a87db7623b55aa322ca2d9c41c8930e6665243cbebd
cb1201e5049482b0578a743edcff5eae553214ec1059b762d5595c993b1e3b9759a76
4a2d195d623b255ed2d49d71306baa808c7298dccb824c34c0d446c73dbd39c3532e5
5b4f5a54a429225725438f2b7f0725db19be0fbb957ec6a632ba400c960ae4d6444c9
4cccc43a4569840d6c405280a61e5a9ca05b20cb36fb497bda9514f647e4bda98aa92
595b85f75df226520d4818d81681c02cbe9099d44d80a8ac550b5cca4c71933ef7ed8
3ee34521917da8c703135666c95b174abe2daf88b6555ea6ef7c0d892ef2889368262
08cbce98e0d9c59493551601b6db66c4c27bed65865c4586d39b38e8b6db5915286dd
91564e0656a762e08add5ad5a4750502dee6c7948f98f4e93833c0e68532a950f5fc7
96f201cad89e0bad902a4f036546df74b5edb56eaf29b074d56
Well, I guess hex data was the answer for saving the world all along.
Let's work through the first code snippet before our hacked data above.
The open method is used to open a file. os.O_RDONLY is one of the many modes of file handing using this method. After our file is opened, we use os.read() to read data in bytes in the file. Here we read 1000 bytes of data. We then decode the data into utf-8 format using decode(), print it and finally we close the file.
In reality, the full form of the os.open() method with all its parameters is:
os.open(file, flags, mode, *, dir_fd)
The first is the file path, second is the flag. We have looked at those. The last two are optional, so we can get away with not using them. mode is for specifying what permissions are allowed if a new file is created. This might be strange to see if you haven't come across it before but you can check out unix file permissions here. It's default is octal 777. This means it can be read, written and executed by everyone. dir_fd refers to a directory, that the path of your file is relative to. Below is a list of flags used.
Flag Parameter | Use |
os.O_RDONLY | To open a file for reading only |
os.O_WRONLY | To open a file for writing only |
os.O_RDWR | To open a file for both reading and writing |
os.O_APPEND | To append data to a file |
os.O_CREAT | For creating a file if it doesn't exist |
os.O_DIRECT | reduce or get rid of caching effects |
Use | to combine two of these such as in;
myFile = os.open('kaliStuff.txt', os.O_RDWR | os.O_CREAT)
chdir()
Use this method if you’d like to change your current directory to another.
import os
print(f"Old directory: {os.getcwd()}") #prints your current directory
os.chdir('./hackNasaSecrets')
print(f"New directory: {os.getcwd()}") #prints the new directory
rmdir()
This is handy for when you’d like to get rid of a directory. Do note however that the directory must be empty before you are able to remove it.
import os
os.rmdir('./someEmptyDirectory')
os.chdir('./someEmptyDirectory')
You'd get a wonderful write up errors as someEmptyDirectory has been removed.
remove()
You use this if your aim is to get rid of a single file.
import os
os.remove('./hackNasaSecrets/endanger.txt')
rename()
This is pretty self explanatory; it simply means you’d like to rename a file.
import os
os.rename('codeThatCouldSaveTheWorld.txt', 'unsuspectingFile.txt')
os.path()
This is a submodule that helps you with a host of methods for file path handling. Some of these methods are shown in the snippet below:
import os
# to get the directory name of the path supplied
os.path.dirname('./otherSecrets/codeThatCouldSaveTheWorld')
# to get the basename of the path supplied
os.path.basename('./otherSecrets/codeThatCouldSaveTheWorld')
# to check if a file exists
os.path.exists('./otherSecrets/codeThatCouldSaveTheWorld')
# to get the last time the file was accessed (in seconds since the epoch)
os.path.getatime('./otherSecrets/codeThatCouldSaveTheWorld')
# to get the time the file was created (in seconds since the epoch)
os.path.getctime('./otherSecrets/codeThatCouldSaveTheWorld')
# to return the size (in bytes) of the file
os.path.getsize('./otherSecrets/codeThatCouldSaveTheWorld')
# to find out if a path is a directory
os.path.isdir('./otherSecrets/codeThatCouldSaveTheWorld')
# to find out if a path is a file
os.path.isfile('./otherSecrets/codeThatCouldSaveTheWorld')
# join one or more paths
os.path.join('instructions', './otherSecrets/codeThatCouldSaveTheWorld')
# returns the absolute path of a file
os.path.abspath('./otherSecrets/codeThatCouldSaveTheWorld')
# Please note that the epoch is just January 1, 1970, 00:00:00 (UTC), so
# os.path.getatime() and os.path.getctime() are measuring the number of seconds
# since that date and time.
There are other methods that you could look up.
The os module is extensive and used for a variety of operations. You could search for files, check file systems, find out if particular documents exist and more. You can use these operations as building blocks for more complicated tasks and projects. Let your imagination run as you start building with the os module. You've gained access into new knowledge :)
Subscribe to my newsletter
Read articles from Amanda Ene Adoyi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amanda Ene Adoyi
Amanda Ene Adoyi
I am a Software Developer from Nigeria. I love learning about new technologies and talking about coding. I talk about coding so much that I genuinely think I could explode if I don't . I have been freelance writing for years and now I do the two things I love the most: writing and talking about tech.