Latest Releases of JavaScript ES2023
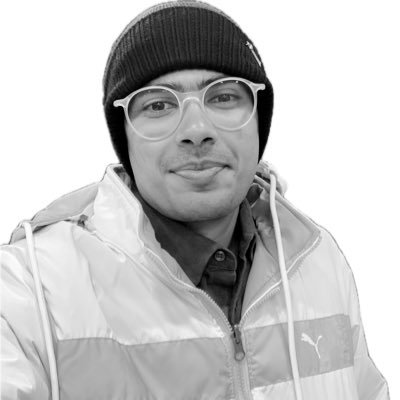
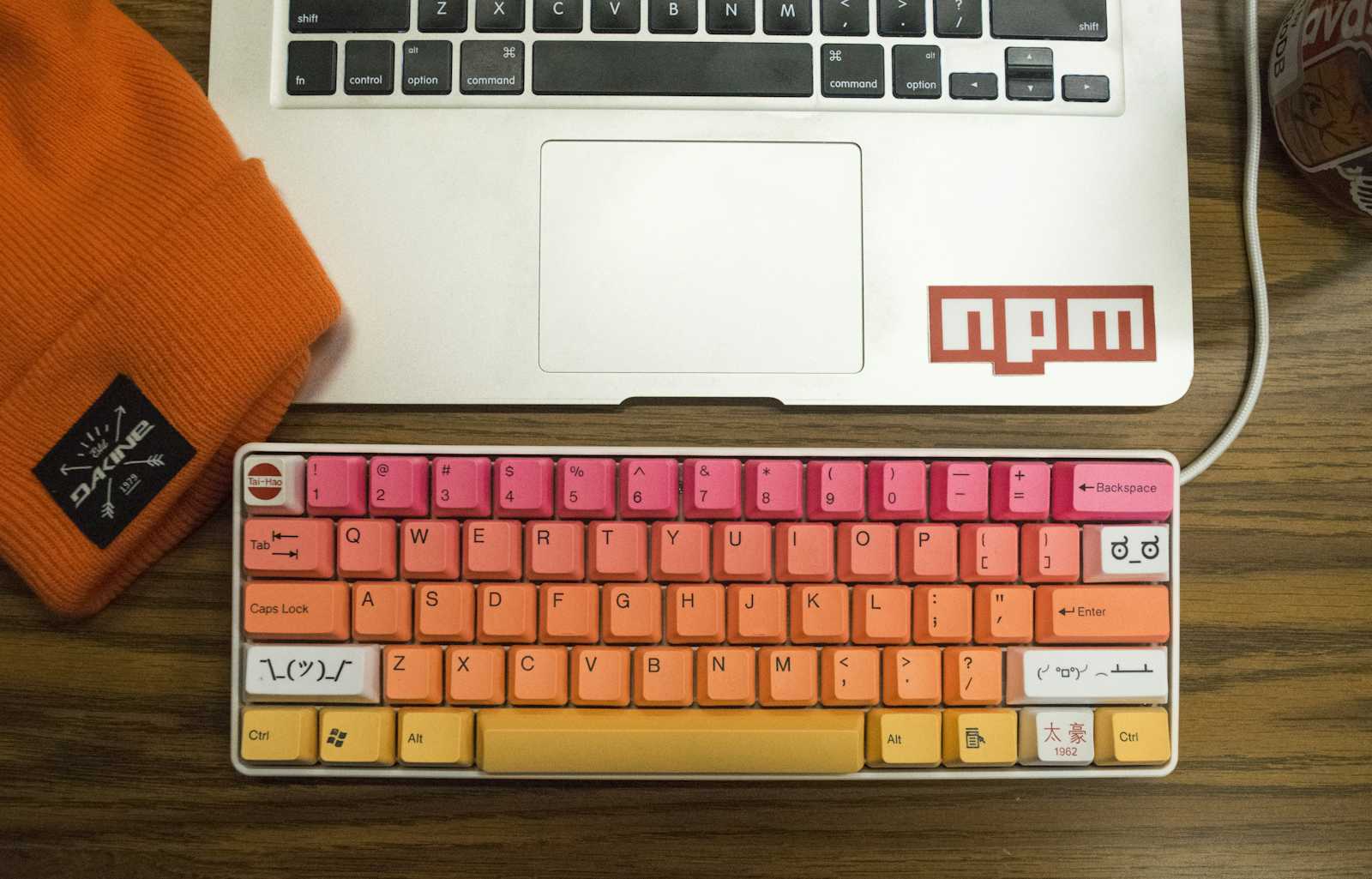
JavaScript has been—the unanimously adopted language of the web—and it's only getting better with time. Plenty of new features and improvements come to the table with each and every edition. Therefore, the ECMAScript specification for 2023 comes loaded with a lot of exciting features and improvements that will certainly make coding in JavaScript more efficient and pleasurable. Let's dive into the latest releases of JavaScript ES2023 and how they can benefit developers.
1. Array Grouping
One of the most awaited features in ES2023 is probably array grouping. It is useful in grouping elements under some category in an array. This specifically comes very much in handy while manipulating and organizing data.
groupBy
The Array.prototype.groupBy
method groups the elements of an array into an object based on the key function.
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const groupedByEvenOdd = numbers.groupBy(num => (num % 2 === 0 ? 'even' : 'odd'));
console.log(groupedByEvenOdd);
// Output: { odd: [1, 3, 5, 7, 9], even: [2, 4, 6, 8, 10] }
groupByToMap
The Array.prototype.groupByToMap
method acts just like groupBy
, but it returns a Map
instead of a plain object. This can be useful when the keys are not strings.
const groupedByValue = numbers.groupByToMap(num => num > 5);
console.log(groupedByValue);
// Output: Map { false => [1, 2, 3, 4, 5], true => [6, 7, 8, 9, 10] }
2. New Hashing Functions
ES2023 comes with two kinds of hashing functions: HashbangGrammar
and StableSort
.
HashbangGrammar
With this grammar, HashbangGrammar
, it enables JavaScript to have hashbang (#!
) syntax in scripts so that one can easily make an executable script runnable in Node.js.
#!/usr/bin/env node
console.log("Hello, world!");
This line is called a shebang and allows a script to be run directly in a Unix-like environment without explicitly invoking Node.js.
StableSort
The Array.prototype.sort
method is now guaranteed to be stable. That means elements which are considered equal by the comparison function retain their relative order in the sorted array.
const items = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 25 },
{ name: 'Eve', age: 30 }
];
items.sort((a, b) => a.age - b.age);
console.log(items);
// Output: [{ name: 'Alice', age: 25 }, { name: 'Bob', age: 25 }, { name: 'Eve', age: 30 }]
3. Decorators
One of the long-awaited features was for a way declaratively to add metadata or change how classes and methods work. This has already existed in TypeScript, but ES2023 finally brings it to standard JavaScript.
function readonly(target, key, descriptor) {
descriptor.writable = false;
return descriptor;
}
class Person {
@readonly
get name() {
return `${this.firstName} ${this.lastName}`;
}
}
const person = new Person();
person.name = function () {
return "Modified Name";
}; // This will not work due to the readonly decorator
4. Top-Level Await
Top-level await permits the use of the await
keyword outside an async function at the top level of modules. It changes how asynchronous code is handled across modules.
// module.mjs
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
Now, top-level await allows one to directly wait on promises at the module level. This cleans up a lot and simplifies the code involved in asynchronous operations.
5. RegExp Match Indices
It adds a 'd' flag to regular expressions that allows for match indices, including start and end indices of the matches.
const regex = /a(b)/d;
const match = regex.exec('abc');
console.log(match.indices);
// [[0, 2], [1, 2]]
This feature is very useful in complex string processes and parsing tasks.
Happy coding!
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
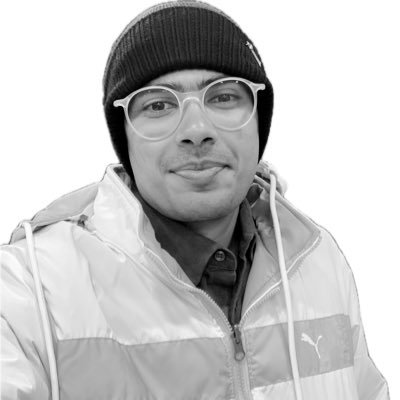
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on ⌨️ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix 👨🏻💻