The Future of Web Development: Embracing JavaScript Web Components π
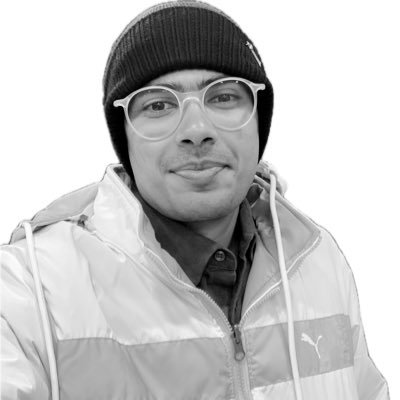
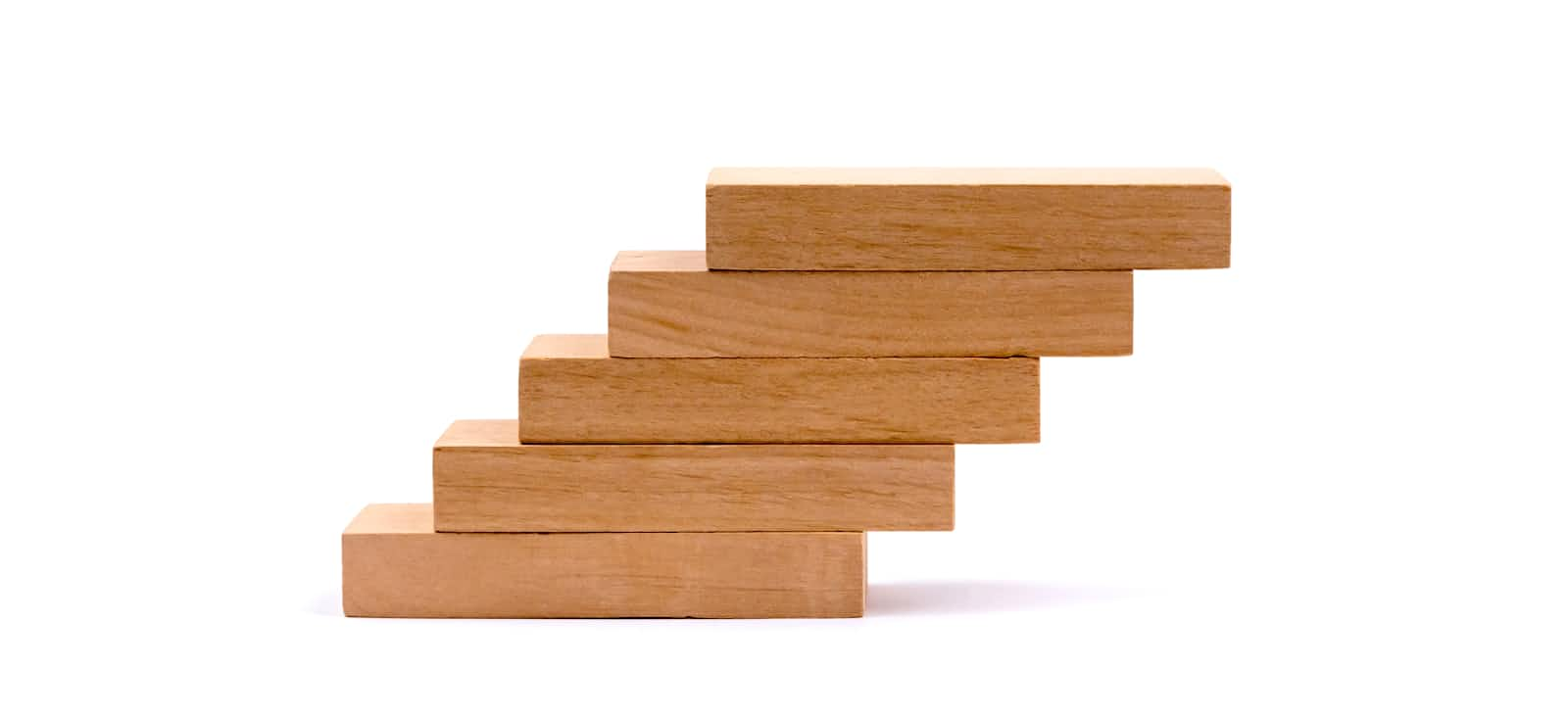
The world of development is forever changing, and this has placed JavaScript Web Components at the threshold of becoming an enormous potential instrument set that might change how we build and deploy applications. They are potential replacements for very popular component-based libraries and frameworks, like React and Angular, with the promise of a no-build development using ES Modules. Web Components are thus staging a scenario of easiness, fluency, and efficiency in the development of applications for the future. We will explain in detail exactly what Web Components are, their benefits, and how they are going to take over the future of web development in this blog.
What are JavaScript Web Components? π€
Web Components is a suite of standardized technologies that allow the development of reusable and encapsulated HTML elements. It is comprised of three main specifications:
Custom Elements ποΈ
They define means of defining custom HTML elements, which imbue custom behavior.
class MyCustomElement extends HTMLElement {
constructor() {
super();
this.attachShadow({ mode: 'open' });
this.shadowRoot.innerHTML = `
<style>
p {
color: blue;
}
</style>
<p>Hello, I am a custom element!</p>
`;
}
}
customElements.define('my-custom-element', MyCustomElement);
Shadow DOM π
They provide a way to encapsulate the internal structure and style of a Web component.
class ShadowElement extends HTMLElement {
constructor() {
super();
const shadow = this.attachShadow({ mode: 'open' });
shadow.innerHTML = `
<style>
span {
color: red;
}
</style>
<span>Shadow DOM example!</span>
`;
}
}
customElements.define('shadow-element', ShadowElement);
HTML Templates π
They define fragments of HTML that are not displayed until they are instantiated.
<template id="my-template">
<style>
div {
color: green;
}
</style>
<div>Template content</div>
</template>
<script>
class TemplateElement extends HTMLElement {
constructor() {
super();
const template = document.getElementById('my-template').content;
const shadow = this.attachShadow({ mode: 'open' });
shadow.appendChild(template.cloneNode(true));
}
}
customElements.define('template-element', TemplateElement);
</script>
These technologies bring together features that can help in the construction of self-contained, reusable components that come in handy across a number of different projects and frameworks.
Advantages of Web Components π
1. Reusability β»οΈ
Any web applicationβirrespective of the framework or library in useβcan use Web Components. This makes them pretty reusable, in that developers can easily share components across projects and teams without fearing compatibility issues.
2. Encapsulation π‘οΈ
Shadow DOM is when Web Components internalize their structure and styles, shielding them from external styles and scripts. As a result of this encapsulation, the code is more predictable and easier to maintain.
3. Interoperability π
Web Components are based on open web platform standards; this means they will work in any modern browser, with no extra libraries or frameworks required. It is also one of the big advantages of Web Components over framework-specific components.
4. Performance β‘
Web Components are based on the native elements of the browser; hence, it gives them an upper hand on performance compared to any other component model, which essentially is based upon the JavaScript libraries that imply overhead processing.
Web Components vs. React and Angular βοΈ
While React and Angular dominated the web development space, Web Components offered many compelling advantages, such as:
Framework Agnosticism π
React and Angular are coupled to their respective ecosystems; this means that components developed using either cannot be reused outside of them. Web Components, on their part, remain decoupled from any particular framework. This provides better flexibility and reusability.
Easy Development π οΈ
Web Components can be developed without any complex build processes. Conversely, ES Modules can be used to create and import Web Components directly in a browser, eliminating the use of bundlers and transpilers. No-build approaches make development easy and reduce the impedance of setting up and maintaining build configurations.
Longevity β³
Web standards evolve more slowly than frameworks, providing a stable foundation for Web Components. This longevity assures that components written today will work tomorrow and way beyond at the top speed of the ever-changing landscape of web development.
Example Comparison
Web Components
class MyComponent extends HTMLElement {
constructor() {
super();
const shadow = this.attachShadow({ mode: 'open' });
shadow.innerHTML = `
<style>
h1 {
color: purple;
}
</style>
<h1>My Web Component</h1>
`;
}
}
customElements.define('my-component', MyComponent);
React
import React from 'react';
const MyComponent = () => {
return (
<h1 style={{ color: 'purple' }}>My React Component</h1>
);
};
export default MyComponent;
Vue
<template>
<h1 :style="{ color: 'purple' }">My Vue Component</h1>
</template>
<script>
export default {
name: 'MyComponent'
};
</script>
The No-Build Future with ES Modules π
ES Modules imply one of the top-level goals of organizing JavaScript code into modules for reuse. They are natively supported in modern browsers and mean the ability for a developer to import and export functionality without a build step.
Advantages of No-Build Development π οΈ
Simplified Workflow: Developers can write code and run it in a browser directly, eliminating development complexity.
Faster Iteration: Changes can be applied and tested on the fly; no build process is necessary.
Reduced Dependencies: By developing against a native browser capability, it significantly reduces the need for additional tooling or dependencies.
Putting ES Modules and Web Components Together π‘
Together with ES Modules, Web Components are a very powerful paradigm of development on the web. Modular, reusable component development means they are developed as modules and then can be imported for direct use within the browser, so development becomes much easier and less frustrating.
// myComponent.js
class MyComponent extends HTMLElement {
constructor() {
super();
const shadow = this.attachShadow({ mode: 'open' });
shadow.innerHTML = `
<style>
h1 {
color: purple;
}
</style>
<h1>My Web Component with ES Modules</h1>
`;
}
}
customElements.define('my-component', MyComponent);
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Web Components with ES Modules</title>
</head>
<body>
<my-component></my-component>
<script type="module" src="./myComponent.js"></script>
</body>
</html>
JavaScript Web Components are simply huge in potential and will revolutionize the future of web development. Added to their long list of advantages are reusability, encapsulation, and interoperability; with performance benefits that make them a very strong candidate to replace framework-specific components in React and Angular. Paired with ES Modules and the no-build approach, Web Components can be used to communicate a much easier, more efficient development workflow that adheres to the changing requirements of today's web applications.
Happy Coding π
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
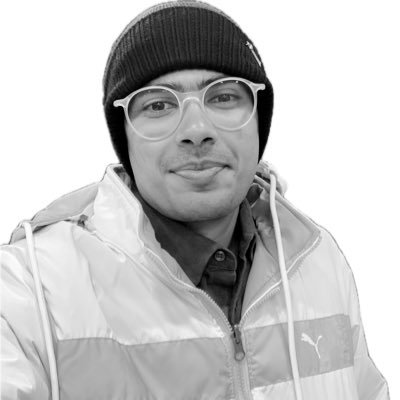
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on β¨οΈ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix π¨π»βπ»