Gradients: The Building Blocks of Backpropagation in TensorFlow

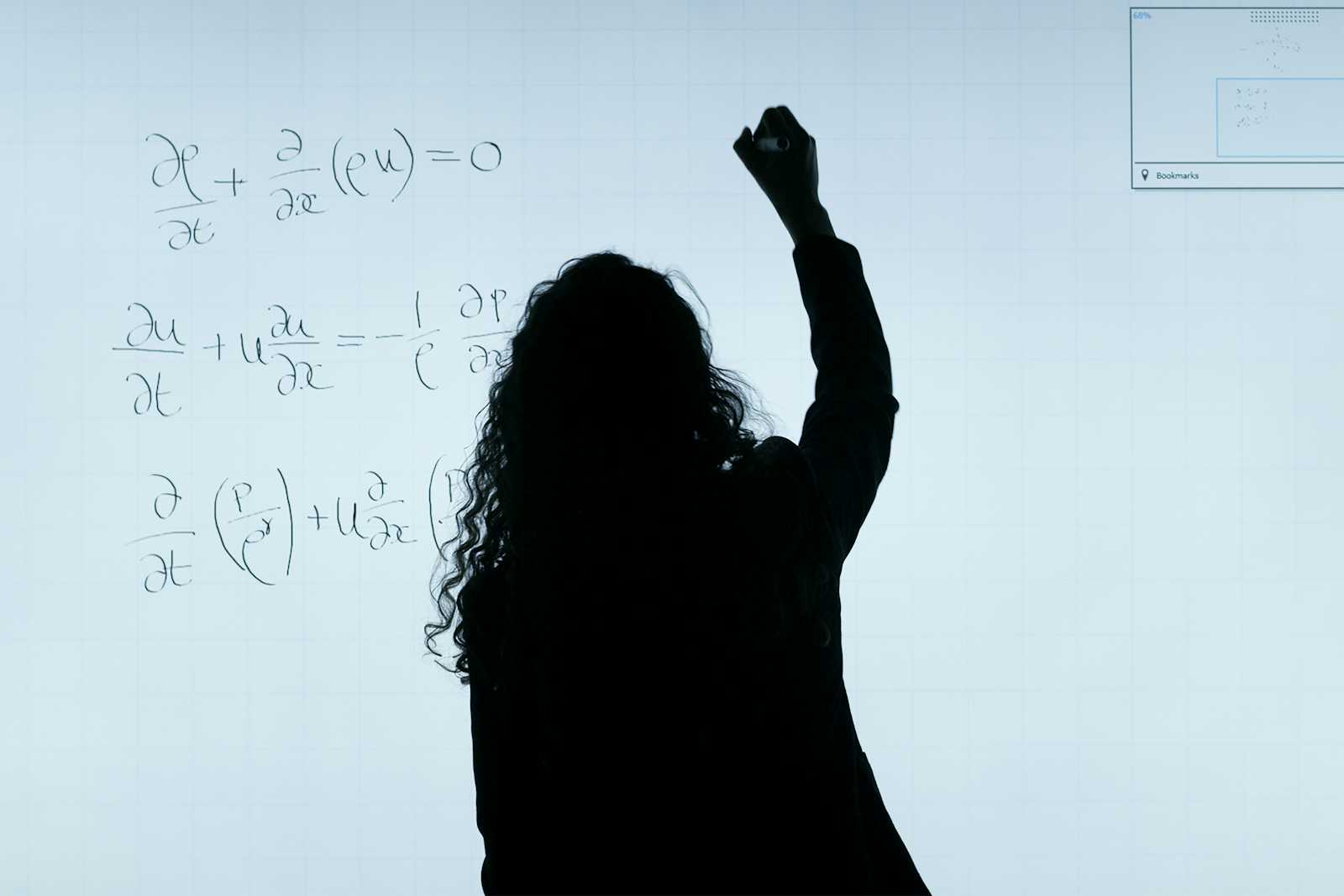
In a neural network, backpropagation is essential for error minimization. It involves calculating the partial derivatives, or gradients, of the loss function with respect to trainable parameters. Manually computing and implementing these derivatives in Python can be complex and require mathematical knowledge. Fortunately, TensorFlow simplifies this with tf.GradientTape
.
What is tf.GradientTape
?
tf.GradientTape
in TensorFlow is used to calculate the gradients of computations involving tensors.
How Does It Calculate Gradients?
TensorFlow records operations applied to tensors, especially tf. Variable
objects, within the scope of tf.GradientTape
. When the gradient
method is called on the tape, it calculates the gradient with respect to the specified inputs.
Example Code:
import tensorflow as tf
w = tf.Variable(initial_value=5.)
with tf.GradientTape() as tape:
loss = tf.square(w)
gradient = tape.gradient(loss, w)
print(gradient.numpy()) # Output: 10.0
Explanation:
w
is initialized as a TensorFlow variable with a value of 5.tf.GradientTape
records operations to compute gradients.loss
is defined as the square ofw
.The gradient computes the derivative of
loss
with respect tow
.The gradient of w^2 is 2w. So for w = 5, the gradient will be 2×5 = 10.
Note: tf.GradientTape
records operations on tf.Variable
. To compute gradients for constants, explicitly tell the tape to watch them using tape.watch(const_var)
.
Subscribe to my newsletter
Read articles from Rashid Ul Haq directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rashid Ul Haq
Rashid Ul Haq
I am a passionate AI and machine learning expert with extensive experience in deep learning, TensorFlow, and advanced data analytics. Having completed numerous specializations and projects, I have a wealth of knowledge and practical insights into the field. I am sharing my journey and expertise through detailed articles on neural networks, deep learning frameworks, and the latest advancements in AI technology.