Store the connection string in an environment variable

1 min read
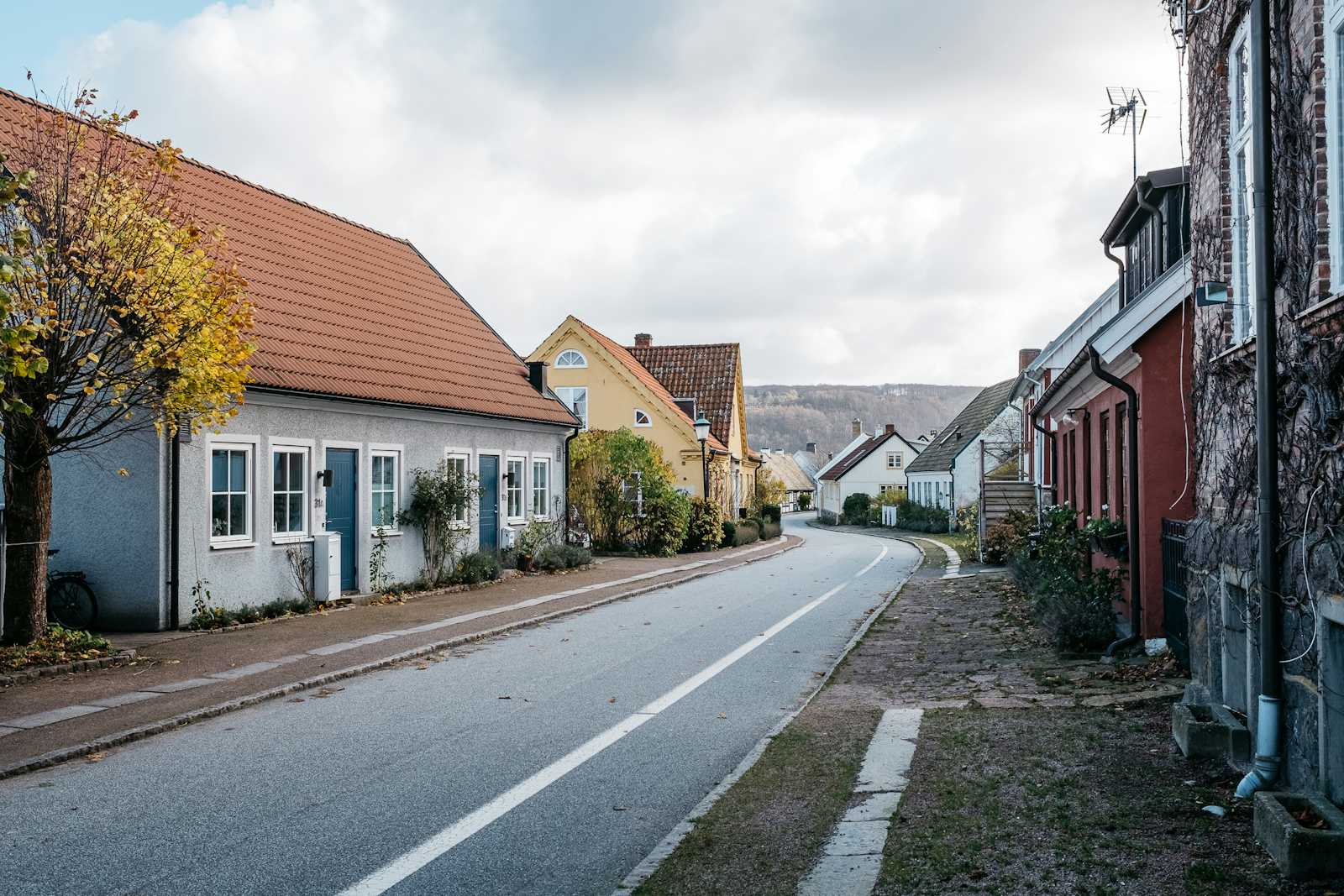
Store the connection string in an environment variable:
Set an environment variable for your connection string. For example, you can add it to your environment variables in the operating system or in the launchSettings.json
file if you are using Visual Studio.
// launchSettings.json
{
"profiles": {
"IIS Express": {
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development",
"DB_CONNECTION_STRING": "Host=192.168.0.118;Database=TESTDB;Username=dbuser;Password=123456;"
}
},
"test1mvc": {
"commandName": "Project",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development",
"DB_CONNECTION_STRING": "Host=192.168.0.118;Database=TESTDB;Username=dbuser;Password=123456;"
}
}
}
}
Modify your Program.cs
to read the connection string from the environment variable:
using Microsoft.AspNetCore.Identity;
using Microsoft.EntityFrameworkCore;
using test1mvc.Data;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
// Use environment variable for connection string
var connectionString = Environment.GetEnvironmentVariable("DB_CONNECTION_STRING")
?? throw new InvalidOperationException("Connection string 'DefaultConnection' not found in environment variables.");
builder.Services.AddDbContext<ApplicationDbContext>(options =>
options.UseNpgsql(connectionString));
builder.Services.AddDatabaseDeveloperPageExceptionFilter();
builder.Services.AddDefaultIdentity<IdentityUser>(options => options.SignIn.RequireConfirmedAccount = true)
.AddEntityFrameworkStores<ApplicationDbContext>();
builder.Services.AddControllersWithViews();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseMigrationsEndPoint();
}
else
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.MapRazorPages();
app.Run();
0
Subscribe to my newsletter
Read articles from GuaSerius24jam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

GuaSerius24jam
GuaSerius24jam
gua memang serious cakap lu, gua serious 24 jam. baik di jamban ,di meja makan atau bersenggama . serius...dohhh