Hoisting In Javascript
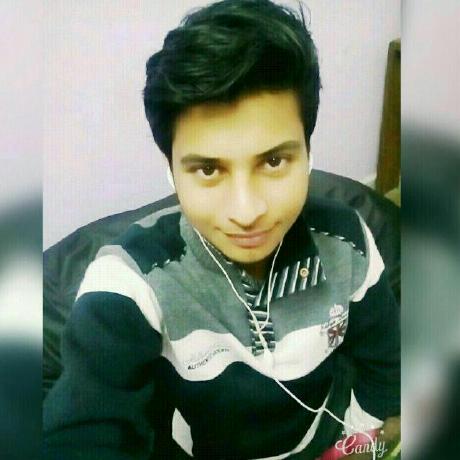
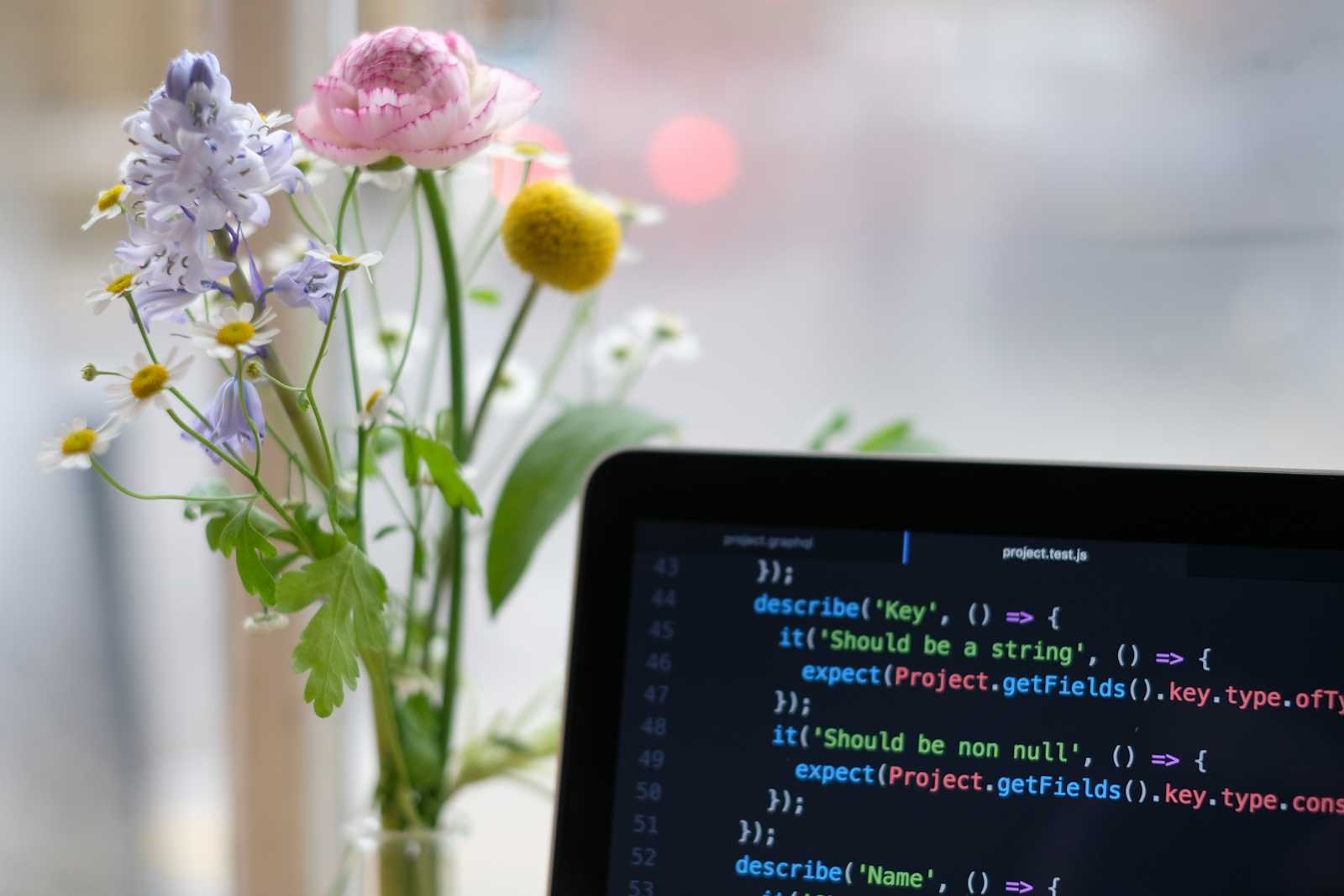
In Javascript "Hoisting" is a behavior where the declaration of variables, functions, and classes move up to their scope where they are defined.
Let's see an example
console.log(x); //It will log undefined, and will not throw any error
var x = 10;
In the example, we try to access the variable x
before defining it. Instead of throwing an error, JavaScript logs undefined
. This is due to hoisting. When executing a program, JavaScript performs two phases:
Memory Allocation Phase: The code is scanned, and memory is allocated for variables and functions. Variables declared with
var
are initialized toundefined
.Execution Phase: The code is executed line by line. Since
x
is already in memory withundefined
,console.log(x)
logsundefined
without error.
When we examine our code example, the JavaScript engine first scans the code, creating a memory location for the variable x
and assigning it an undefined
value. During the execution phase, when JavaScript reaches console.log(x)
, it doesn't throw an error because x
is already present in memory with an undefined
value, which is then logged.
- For variables defined with the var keyword, we can use the reference of the variable but the value will be undefined
console.log(x); //Logs undefined because x is hoisted and initialized as undefined
var x = 10;
- For functions and imports, we can use their value in their scope before the declaration
printHello(); // logs Hello
function printHello() {
console.log("Hello")
}
- Let, const, and class are also hoisted but because of the temporal dead zone they will throw a reference error if we try to access them before the declaration
const a = 10;
{
console.log(a); // logs 10
}
const b = 10;
{
console.log(b); // Throws Reference Error
const b = 5;
}
Subscribe to my newsletter
Read articles from Kaushal Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
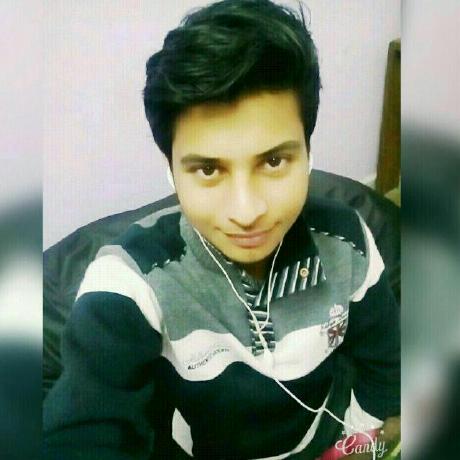
Kaushal Pandey
Kaushal Pandey
Software Engineer | Full Stack Dev