Getting Started with C Programming: A Begineer's Guide.

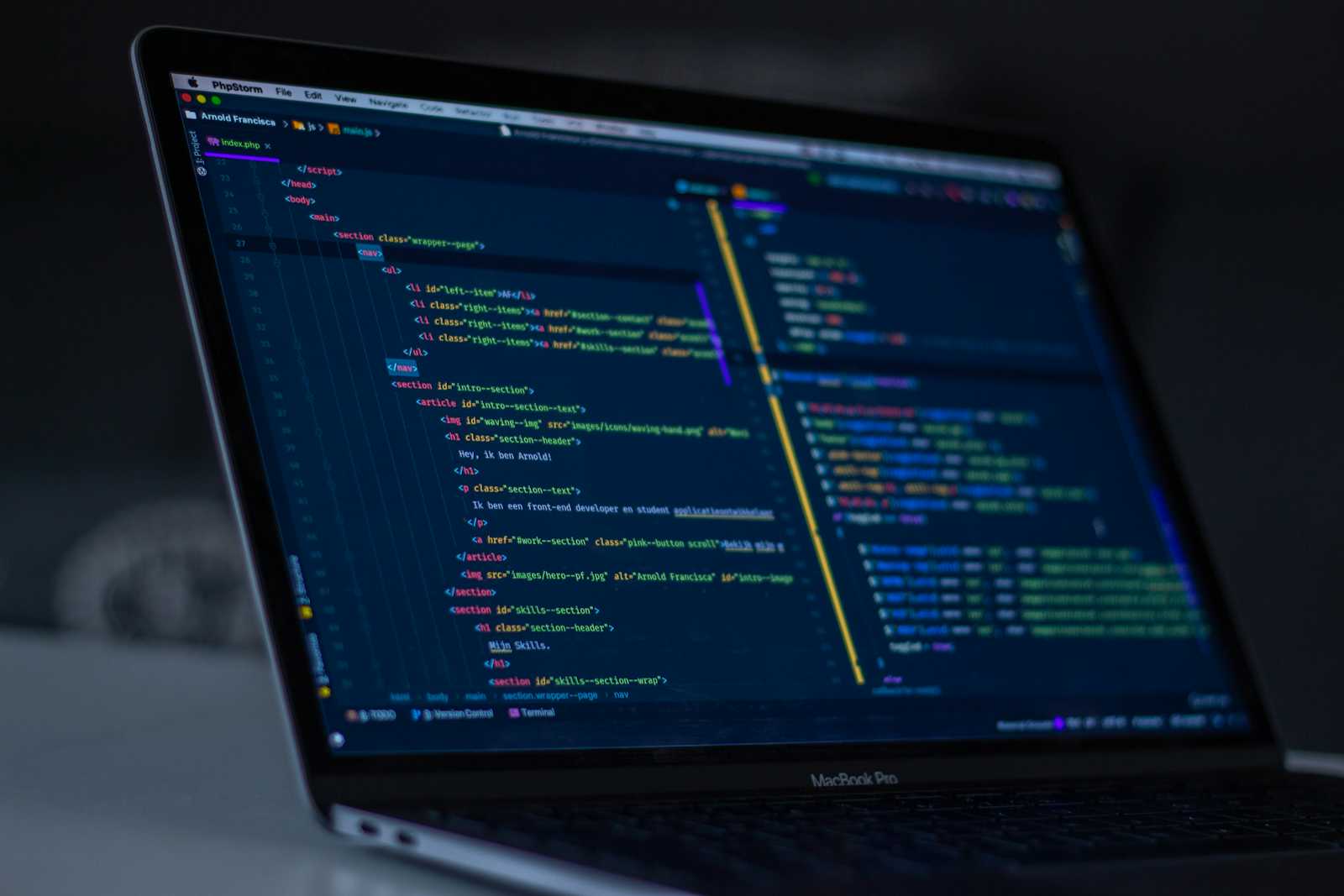
Table of Contents:
1. Introduction to C Programming
- Why Learn C?
2. Setting Up the Environment
Setting up an IDE (VS Code)
Writing and Running Your First C Program
3. Basic Syntax and Structure
Structure of a C Program
Variables and Data Types
4. Operators and Expressions
Arithmetic Operators
Relational Operators
Logical Operators
Assignment Operators
Increment and Decrement Operators
5. Control Flow Statements
6. Functions
Calling Functions
Passing Arguments to Functions
7. Conclusion
In this guide, we will cover the basics of C programming, from setting up your development environment to writing and running your first program. We'll explore fundamental concepts such as variables, data types, operators, control flow statements, functions. By the end of this guide, you will have a solid understanding of C programming and be well-prepared to tackle more advanced topics and real-world coding challenges.
1.Introduction to C Programming
Why Learn C:
Learning C is crucial for anyone serious about programming because it provides a strong foundation in computer science principles. As a low-level language, C offers direct control over memory and hardware, making it essential for developing operating systems, embedded systems, and performance-critical applications. C’s simplicity and efficiency make it a great language for beginners, while its widespread influence on other languages ensures that the skills you gain are transferable
2.Setting Up the Environment:
Before diving into coding, it's essential to set up a conducive environment. Choose a reliable Integrated Development Environment (IDE) like Code::Blocks or Visual Studio Code. These tools provide a user-friendly interface, code highlighting, and debugging capabilities, making the learning process smoother.
First Program:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
3.Basic Syntax and Structure:
In this example(structure and program):
#include <stdio.h>
is a preprocessor directive that tells the compiler to include the standard input/output library, which provides functions like printf
for output.
int main()
declares the main
function with a return type of int
.
{
and }
enclose the body of the main
function. All the executable code of the program goes inside these curly braces.
printf("Hello, World!\n");
is a simple C statement that prints "Hello, World!" to the console.
return 0;
signals to the operating system that the program has executed successfully. The value 0 is returned to the operating system.
Variables and Data Types:
In C, variables are like containers that store data. Before using a variable, you must declare its type, such as int (integer), float (floating-point number), or char (character). Understanding data types is crucial for efficient memory usage and accurate representation of values in your programs.
#include <stdio.h>
int main() {
int number = 42;
float pi = 3.14159;
char grade = 'A';
printf("Number: %d\n", number);
printf("Pi: %f\n", pi);
printf("Grade: %c\n", grade);
return 0;
}
4.Operators and Expressions:
Operators in C programming are symbols used to perform operations on variables and values. They include arithmetic operators (+
, -
, *
, /
, %
), comparison operators (==
, !=
, <
, >
, <=
, >=
), logical operators (&&
, ||
, !
), assignment operators (=
), and increment/decrement operators (++
, --
). Additionally, C supports bitwise operators (&
, |
, ^
, ~
, <<
, >>
) for manipulating bits within variables. Mastery of these operators enables developers to write concise and efficient code for a wide range of computational tasks in C programming.
5.Control Flow Statements:
Conditional Statements:
if
Statement-:
The if
statement allows you to execute a block of code only if a specified condition is true.
if (condition) {
// Code block to be executed if condition is true
}
if-else
Statement-:
The if-else
statement allows you to execute one block of code if the condition is true and another block of code if the condition is false.
if (condition) {
// Code block to be executed if condition is true
} else {
// Code block to be executed if condition is false
}
else if
Statement-:
The else if
statement allows you to check multiple conditions sequentially.
if (condition1) {
// Code block to be executed if condition1 is true
} else if (condition2) {
// Code block to be executed if condition2 is true
} else {
// Code block to be executed if all conditions are false
}
Looping Statement-:
while
Loop:
The while
loop executes a block of code as long as a specified condition is true.
while (condition) {
// Code block to be executed repeatedly as long as condition is true
}
for
Loop:
The for
loop provides a more compact way to iterate over a range of values.
for (initialization; condition; increment/decrement) {
// Code block to be executed repeatedly until condition is false
}
do-while
Loop
The do-while
loop is similar to while
loop, but it guarantees that the code block is executed at least once before checking the condition.
do {
// Code block to be executed at least once
} while (condition);
Jump Statements:
break Statement**:** Terminates the nearest enclosing loop or switch
statement.
continue Statement**:** Skips the rest of the loop's code for the current iteration and moves to the next iteration.
goto Statement**:** Allows jumping to a labeled statement in the program. However, the use of goto
is generally discouraged due to its potential for creating unreadable and hard-to-maintain code.
6.Functions:
Functions are blocks of reusable code that perform specific tasks. They enhance the modularity of your programs and make them easier to understand. In C, a function is declared with a return type, a name, and parameters.
#include <stdio.h>
// Function declaration
int add(int a, int b) {
return a + b;
}
int main() {
int result = add(3, 4);
printf("Sum: %d\n", result);
return 0;
}
7.Conclusion:
Congratulations! You've just scratched the surface of the C programming language. As you embark on your coding journey, continue exploring and experimenting with these foundational concepts. Mastering C will not only provide a solid programming foundation but also the way for delving into more advanced languages and projects. Happy coding.
Subscribe to my newsletter
Read articles from Lopamudra Rout directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
