Advanced Hooks: useMemo and useCallback

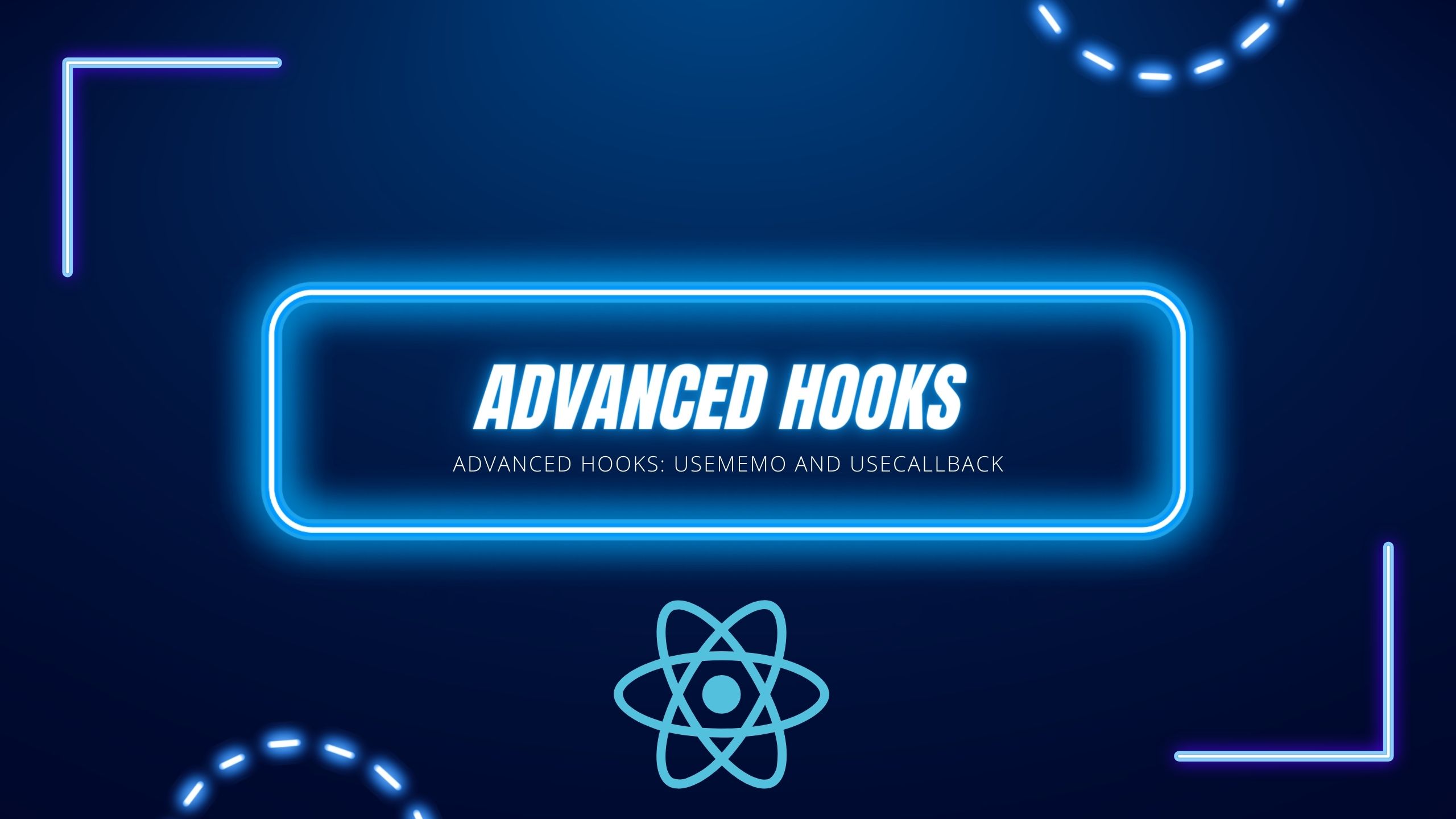
Introduction
Understanding the Importance of Optimization in React
In the rapidly evolving landscape of web development, React has cemented its place as a go-to library for building dynamic and interactive user interfaces. However, as applications grow in complexity, the need for performance optimization becomes paramount. Efficiently managing rendering processes ensures a seamless user experience, minimizes lag, and enhances the overall responsiveness of the application. This is where the strategic use of advanced hooks, such as useMemo
and useCallback
, comes into play. These hooks provide developers with powerful tools to fine-tune their applications, ensuring that unnecessary computations and re-renders are avoided.
Overview of useMemo and useCallback Hooks
React's useMemo
and useCallback
hooks are designed to optimize functional components by controlling how and when computations and callbacks are executed. useMemo
is used to memoize values, effectively caching the results of expensive calculations so that they are only recalculated when necessary. On the other hand, useCallback
is used to memoize functions, ensuring that the same function instance is returned unless its dependencies change. Together, these hooks play a crucial role in enhancing performance and maintaining the efficiency of React applications.
Deep Dive into useMemo
What is useMemo?
useMemo
is a React hook that allows developers to memoize the result of a computation. By storing the computed value and returning it only when its dependencies change, useMemo
helps to avoid unnecessary recalculations. This can be particularly beneficial in scenarios where a function performs intensive operations or when rendering large lists.
How useMemo Works: Under the Hood
Under the hood, useMemo
works by comparing the dependencies array provided by the developer with the previous render's dependencies. If there are no changes, React returns the previously memoized value. If there are changes, the function inside useMemo
is executed again to compute and memoize the new result. This mechanism ensures that expensive calculations are not performed on every render, thereby improving performance.
When to Use useMemo: Practical Scenarios
Using useMemo
is particularly advantageous in scenarios involving computationally heavy operations, such as filtering large datasets or performing complex mathematical calculations. It is also useful when rendering components that involve intricate logic, ensuring that these components only re-render when absolutely necessary.
Benefits of useMemo: Speeding Up Your App
The primary benefit of useMemo
is its ability to speed up React applications by reducing the frequency of expensive calculations. By memoizing the results of these calculations, useMemo
ensures that React components remain performant, even as they grow in complexity. This leads to a smoother user experience and more efficient use of resources.
Common Pitfalls and How to Avoid Them
Despite its benefits, useMemo
can introduce pitfalls if not used correctly. Overusing useMemo
can lead to unnecessary complexity and memory overhead. Developers should carefully consider whether the performance gains justify the added complexity. Additionally, failing to provide accurate dependencies can result in stale or incorrect values being returned. To avoid these issues, it is essential to thoroughly understand the specific needs of your application and to use useMemo
judiciously.
Deep Dive into useCallback
What is useCallback?
useCallback
is a React hook that memoizes functions, ensuring that the same function instance is returned across renders unless its dependencies change. This is particularly useful for preventing unnecessary re-renders of child components that depend on callback functions.
How useCallback Works: An In-Depth Look
Similar to useMemo
, useCallback
works by comparing the dependencies array between renders. If the dependencies remain unchanged, useCallback
returns the previously memoized function. If the dependencies change, a new function instance is created. This mechanism helps to maintain stable references to functions, reducing the likelihood of unwanted re-renders.
When to Use useCallback: Real-World Examples
useCallback
is most effective in scenarios where callback functions are passed down to child components. For example, in a complex form with multiple input fields, useCallback
can be used to ensure that the change handlers for these inputs do not trigger unnecessary re-renders of the entire form. This can lead to significant performance improvements in large applications.
Advantages of useCallback: Enhancing Performance
The primary advantage of useCallback
is its ability to enhance performance by maintaining stable function references. This reduces the overhead associated with creating new functions on every render and helps to prevent excessive rendering of child components. As a result, useCallback
can lead to more responsive and efficient React applications.
Misconceptions and Mistakes to Watch Out For
A common misconception about useCallback
is that it should be used indiscriminately to memoize all functions. However, overusing useCallback
can lead to unnecessary complexity and can sometimes have a negative impact on performance. It is crucial to understand when memoizing functions will provide tangible benefits and to use useCallback
accordingly.
Practical Applications of useMemo
Memoizing Expensive Calculations
One of the most common use cases for useMemo
is memoizing expensive calculations. By caching the results of these calculations, useMemo
ensures that they are only re-executed when their dependencies change. This can significantly improve the performance of components that rely on complex or resource-intensive operations.
Optimizing Component Re-renders
useMemo
can also be used to optimize component re-renders by memoizing derived state or computed values. This ensures that components only re-render when their inputs change, reducing the overall rendering workload and improving performance.
Improving List Rendering Performance
In scenarios where a component renders a large list of items, useMemo
can be used to memoize the list or its transformations. This prevents the list from being re-calculated on every render, resulting in smoother and more efficient rendering.
Case Study: Applying useMemo in a Data-Heavy App
Consider a data-heavy application that displays a large table of information. By using useMemo
to memoize the filtered and sorted versions of the data, the application can avoid recalculating these transformations on every render. This leads to a more responsive user interface and a better user experience.
Practical Applications of useCallback
Preventing Unnecessary Re-renders in Child Components
useCallback
is particularly useful for preventing unnecessary re-renders in child components that depend on callback functions. By memoizing these functions, useCallback
ensures that child components only re-render when necessary, improving overall performance.
Handling Event Listeners Efficiently
In scenarios where event listeners are dynamically created and attached, useCallback
can be used to memoize the event handler functions. This prevents the need to re-attach event listeners on every render, leading to more efficient handling of user interactions.
Optimizing Callback Functions in Large Applications
For large applications with complex state management, useCallback
can be used to optimize the performance of callback functions that are passed down through multiple levels of the component tree. This helps to maintain stable references and prevents excessive re-renders.
Case Study: Using useCallback in a Complex UI
Consider a complex UI with multiple interactive components, such as a dashboard with various widgets. By using useCallback
to memoize the event handlers for these widgets, the application can avoid unnecessary re-renders and ensure that the UI remains responsive and performant.
Combining useMemo and useCallback
How They Work Together: A Synergistic Approach
useMemo
and useCallback
can be used together to create a synergistic approach to performance optimization. By memoizing both values and functions, developers can ensure that their applications run efficiently, even as they grow in complexity.
Strategies for Effective Use
To use useMemo
and useCallback
effectively, developers should identify the parts of their application that would benefit the most from memoization. This involves analyzing the performance characteristics of different components and determining where memoization can provide the greatest impact.
Example: Building a High-Performance Dashboard
Consider a high-performance dashboard that displays real-time data. By using useMemo
to memoize the processed data and useCallback
to memoize the event handlers for interacting with the dashboard, developers can ensure that the application remains responsive and performant, even under heavy load.
Best Practices and Tips
Identifying When to Use useMemo and useCallback
The key to using useMemo
and useCallback
effectively is to identify when their use is warranted. Developers should focus on parts of their application that involve expensive calculations or frequent re-renders and consider whether memoization can provide meaningful performance improvements.
Measuring Performance Improvements
To measure the impact of useMemo
and useCallback
, developers can use tools such as React's profiling API and browser performance tools. These tools provide insights into rendering times and can help to identify bottlenecks and areas for optimization.
Debugging and Profiling Optimizations
Debugging and profiling optimizations involving useMemo
and useCallback
can be challenging. Developers should use tools such as React DevTools to inspect component hierarchies and identify issues related to memoization. Profiling tools can also help to pinpoint performance bottlenecks and guide optimization efforts.
Common Challenges and Solutions
Dealing with Dependency Arrays
One of the most common challenges when using useMemo
and useCallback
is managing dependency arrays. Developers need to ensure that these arrays accurately reflect the dependencies of the memoized values and functions. Failing to do so can result in stale values or unnecessary re-renders.
Managing State and Props with useMemo and useCallback
Managing state and props with useMemo
and useCallback
requires careful consideration. Developers need to ensure that the memoized values and functions are correctly updated in response to changes in state and props, without introducing bugs or performance issues.
Avoiding Over-Optimization
While `use
Memoand
useCallback` can provide significant performance improvements, over-optimizing can lead to unnecessary complexity and maintenance challenges. Developers should use these hooks judiciously and focus on optimizing parts of their application where the performance gains justify the added complexity.
Conclusion
Recap of Key Points
In summary, useMemo
and useCallback
are powerful tools for optimizing React applications. By memoizing values and functions, developers can reduce unnecessary computations and re-renders, leading to more efficient and responsive applications.
Future Trends in React Optimization
Looking ahead, future trends in React optimization are likely to focus on enhancing the performance and scalability of applications. This may involve new hooks and tools for managing state and rendering, as well as improvements to existing optimization techniques.
Further Reading and Resources
For those interested in learning more about useMemo
and useCallback
, there are numerous resources available, including the official React documentation, online tutorials, and community forums. These resources provide in-depth explanations and examples to help developers master the use of these advanced hooks.
Subscribe to my newsletter
Read articles from Manvendra Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Manvendra Singh
Manvendra Singh
R3F | Laravel | Babylon | Three.js | WordPress Performance Enthusiast