Understanding React Hooks
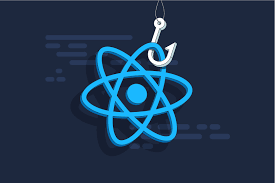
React Hooks are a powerful feature that allows you to use state and other React features without writing a class. Introduced in React 16.8, hooks have changed how we write React applications by making code simpler and more readable. In this blog, we will dive into the most commonly used hooks, how they work, and provide examples to help you understand them better.
What Are React Hooks?
Hooks are special functions that let you “hook into” React features. Before hooks, you had to use classes to manage state and lifecycle methods. Now, with hooks, you can use these features in functional components.
Why Use Hooks?
Simpler Code: Hooks make your code easier to read and maintain.
Reuse Logic: You can extract and reuse stateful logic between components.
No More Classes: You can write components without needing to understand class components.
Basic Hooks
Let's explore the most commonly used hooks: useState
, useEffect
, and useContext
.
useState
The useState
hook allows you to add state to your functional components. It returns an array with two elements: the current state and a function to update it.
Example:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
In this example, count
is the state variable, and setCount
is the function to update it. Initially, count
is set to 0.
useEffect
The useEffect
hook lets you perform side effects in your components, such as fetching data, directly updating the DOM, and more. It runs after every render by default.
Example:
import React, { useState, useEffect } from 'react';
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() => {
setSeconds(prevSeconds => prevSeconds + 1);
}, 1000);
return () => clearInterval(interval);
}, []);
return (
<div>
<p>Seconds: {seconds}</p>
</div>
);
}
Here, useEffect
sets up an interval that updates the seconds
state every second. The cleanup function clears the interval when the component unmounts.
useContext
The useContext
hook allows you to use context, making it easier to share data between components without passing props down manually through every level.
Example:
import React, { useContext } from 'react';
const ThemeContext = React.createContext('light');
function DisplayTheme() {
const theme = useContext(ThemeContext);
return <p>Current theme: {theme}</p>;
}
function App() {
return (
<ThemeContext.Provider value="dark">
<DisplayTheme />
</ThemeContext.Provider>
);
}
In this example, useContext
retrieves the current value of ThemeContext
, which is "dark" in the App
component.
Advanced Hooks
Besides the basic hooks, there are advanced hooks like useReducer
, useMemo
, and useCallback
that help manage more complex state logic and performance optimizations.
useReducer
The useReducer
hook is a good alternative to useState
for managing complex state logic. It is similar to Redux but built into React.
Example:
import React, { useReducer } from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</div>
);
}
Here, useReducer
takes a reducer function and an initial state. It returns the current state and a dispatch function to trigger state changes.
useMemo
The useMemo
hook memoizes expensive calculations, recomputing them only when dependencies change.
Example:
import React, { useState, useMemo } from 'react';
function ExpensiveCalculationComponent({ num }) {
const expensiveCalculation = useMemo(() => {
let result = 0;
for (let i = 0; i < num * 1000; i++) {
result += i;
}
return result;
}, [num]);
return <div>Result: {expensiveCalculation}</div>;
}
Here, useMemo
memoizes the result of the expensive calculation, preventing unnecessary recalculations unless num
changes.
useCallback
The useCallback
hook returns a memoized version of a callback function, which is useful for passing callbacks to optimized child components.
Example:
import React, { useState, useCallback } from 'react';
function Button({ onClick }) {
return <button onClick={onClick}>Click me</button>;
}
function App() {
const [count, setCount] = useState(0);
const increment = useCallback(() => {
setCount(count + 1);
}, [count]);
return (
<div>
<p>Count: {count}</p>
<Button onClick={increment} />
</div>
);
}
Here, useCallback
memoizes the increment
function, preventing unnecessary re-renders of the Button
component.
Conclusion
React Hooks have revolutionized how we write React applications, making our code more concise and easier to understand. By using hooks like useState
, useEffect
, and useContext
, we can manage state and side effects more efficiently. Advanced hooks like useReducer
, useMemo
, and useCallback
provide additional tools for handling complex state logic and performance optimizations.
Always remember to experiment with these hooks and see how they can simplify your React code.
Liked reading?
Let me know in the comments section, and do like my blog & subscribe to my newsletter.
Subscribe to my newsletter
Read articles from Animesh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Animesh Kumar
Animesh Kumar
I’m a software developer who loves problem-solving, data structures, algorithms, and competitive coding. I’ve a keen interest in product development. I’m passionate about AI, ML, and Python. I love exploring new ideas and enjoy innovating with advanced tech. I am eager to learn and contribute effectively to teams.