[TWIL] Week of June 16, 2024
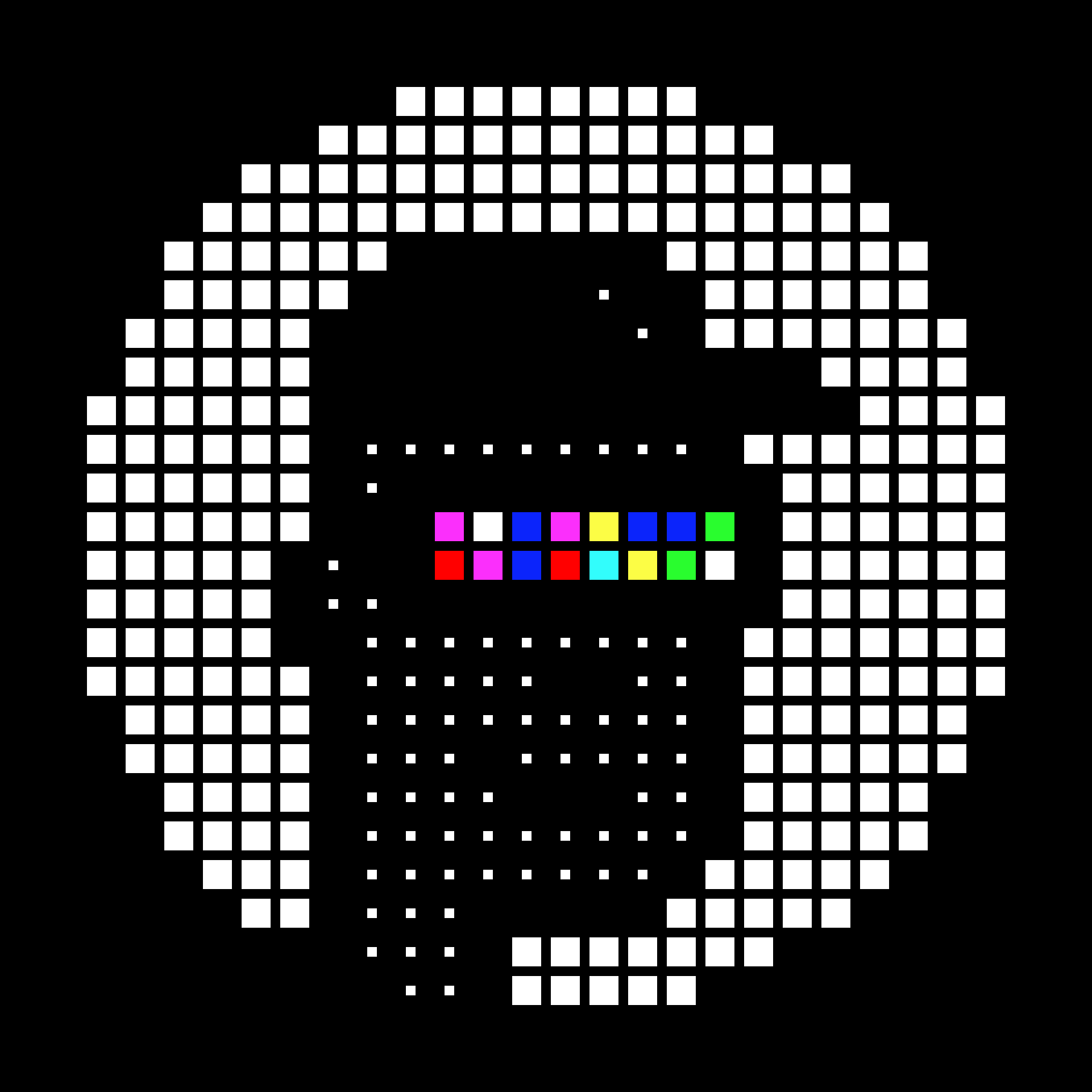
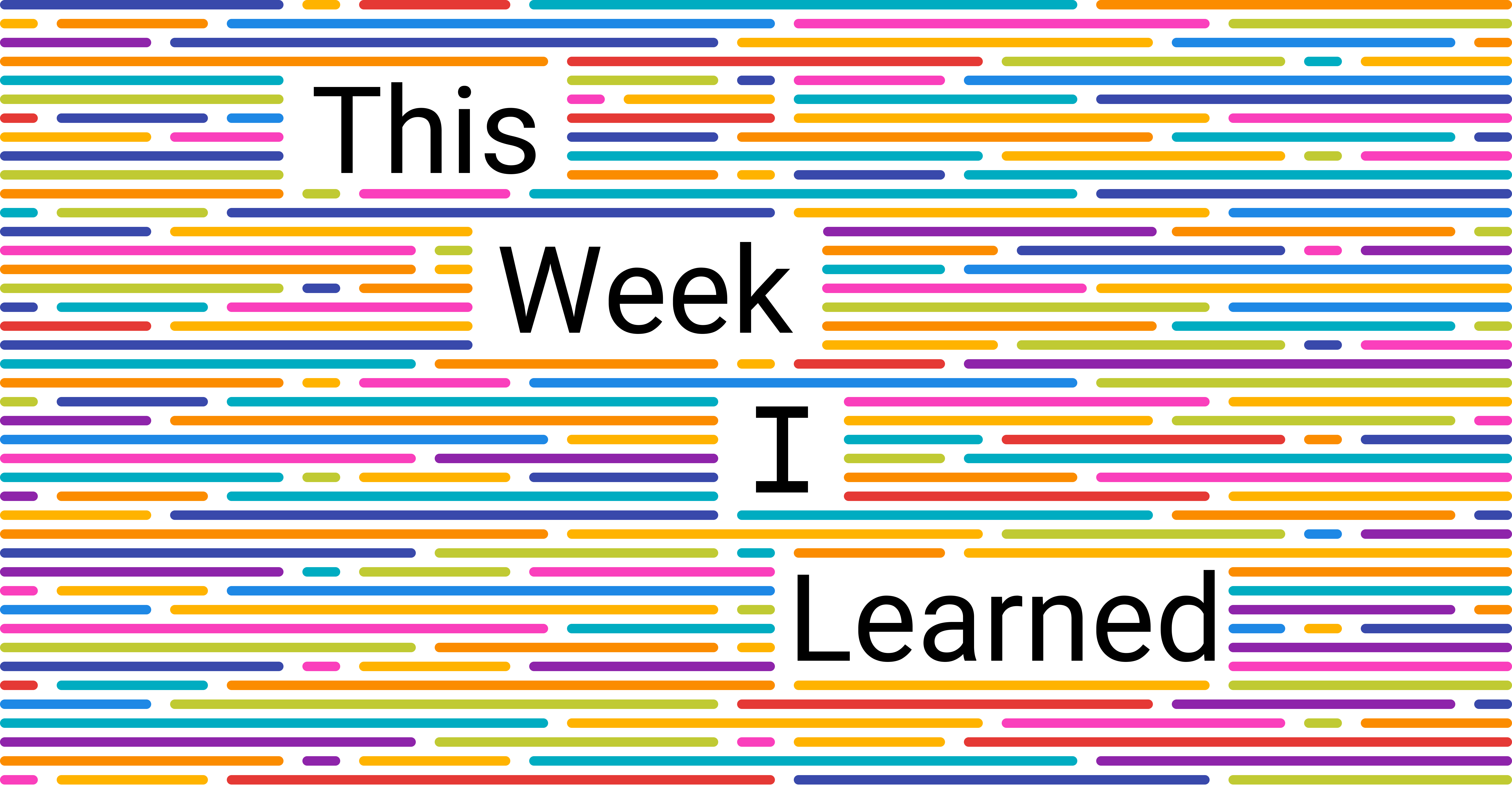
This week marked the third week of the Onchain Summer Buildathon, where our focus was on refining our code to support interactions with Externally Owned Accounts (EOAs). Here’s a deep dive into what I learned and achieved during this week of building.
EOA with Smart Wallet Integration
Our platform already had artists using EOAs to mint music NFTs, so migrating them entirely to Smart Wallets wasn't feasible. Instead, we had to adapt our client-side code to support both Smart Wallet accounts and EOAs. This involved implementing conditional logic to handle each type of account using the useCapabilities
hook.
Here's an example of how we managed batch transactions for both account types:
const Component = () => {
const { data: capabilities } = useCapabilities({
account,
});
const isSmartWallet = Object.keys(capabilities).length > 0;
const methodOne = {
// args...
}
const methodTwo = {
// args...
}
const { writeContractsAsync } = useWriteContracts();
const { writeContractAsync } = useWriteContract();
const { data: methodOneData } = useSimulateContract(methodOne);
const { data: methodTwoData } = useSimulateContract({
...methodTwo,
skipSimulation: true
});
const handler = async () => {
if (isSmartWallet) {
await writeContractAsync({
contracts: [methodOne, methodTwo]
});
return;
}
const hash = await writeContractAsync(methodOneData);
await waitForTransactionReceipt(config, { hash });
await writeContractAsync(methodTwoData);
}
}
Key Learnings and Highlights
Detecting Smart Wallet vs. EOA: We used the
useCapabilities
hook to check if the user has capabilities associated with a Smart Wallet. Ifcapabilities
has at least one key-value pair, it indicates a Smart Wallet; otherwise, it’s an EOA. TheisSmartWallet
variable serves as this indicator.Handling Simulation and Execution: Include
skipSimulation: true
on the seconduseSimulateContract
argument if themethodTwo
transaction depends onmethodOne
being executed and confirmed. This avoids errors during simulation. Additionally, invokewaitForTransactionReceipt
to ensure the first transaction is confirmed before executing the second one.
Hardhat Network Variable
The Hardhat npm package provides many useful variables, including network
, which tells you which chain you're executing your script on at runtime. This is particularly useful if you have identical smart contracts deployed on multiple blockchains. Here’s a handy way to get smart contract addresses across supported blockchains:
// main.ts
import { network } from "hardhat";
enum EthereumSCAddresses {
NFT = "<smart-contract-address>"
}
enum BaseSCAddresses {
NFT = "<smart-contract-address>"
}
function getContractAddress() {
switch (network.name) {
case "ethereum":
return EthereumSCAddresses
case "base":
return BaseSCAddresses;
default:
throw new Error("Invalid network name");
}
}
function main() {
const nftAddress = getContractAddress().NFT;
}
When you run npx hardhat run main.ts --network ethereum
, getContractAddress
will return EthereumSCAddresses
, and .NFT
will return the corresponding smart contract address.
Wrapping Up
That’s it for this week! As we head into the final week of the Onchain Summer Buildathon, we'll continue to fix issues and build new features. Happy hacking everyone ☕️!
Subscribe to my newsletter
Read articles from Sean Kim directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
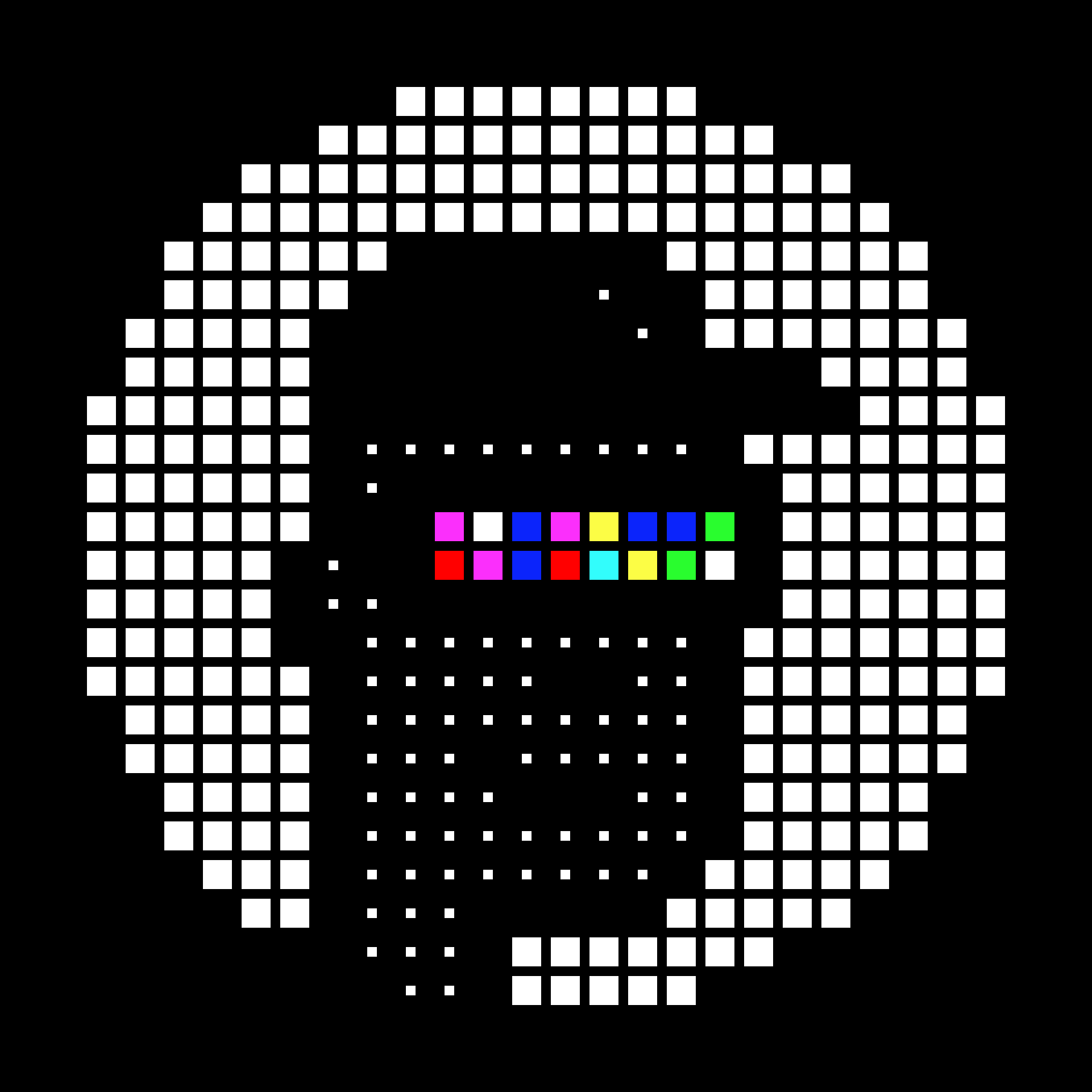
Sean Kim
Sean Kim
Engineer @ ___ | Ex-Ourkive | Ex-Meta