Boost Your App's Performance: How MongoDB Optimizes Data Storage(lt.44)

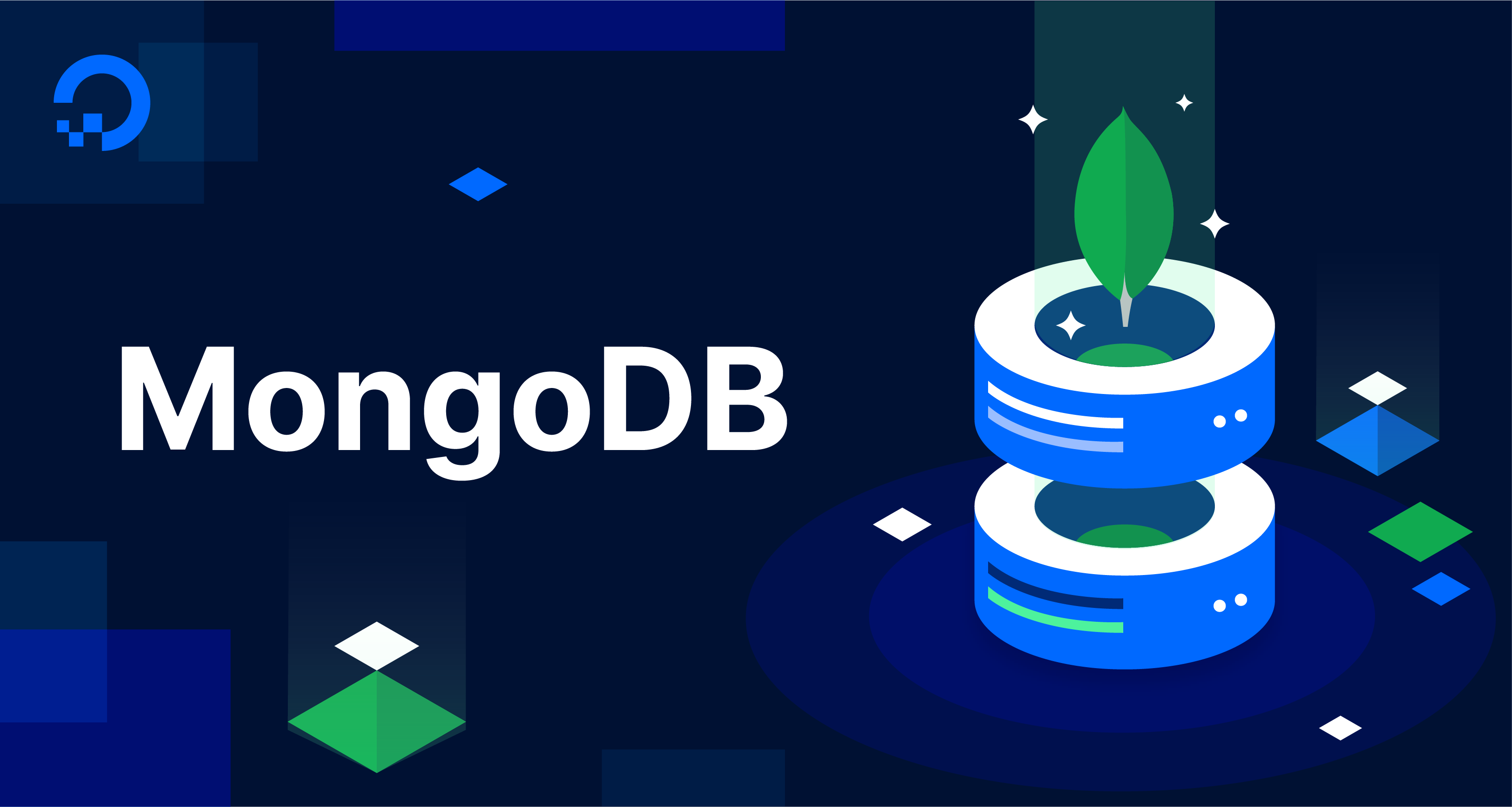
A brief about data base management system.
DBMS : It is software that provides an interface for users and applications to interact with a database. It allows for the storage, modification, and retrieval of data in a structured and efficient manner.
Relational Database : A database where information is stored in the form of tables.
MySQL , MariaDB , PgSQL are some of the examples of rdbms.
NoSQL : NoSQL databases are a type of database designed to handle large amounts of diverse and unstructured data. They generally don't store data in the forms of table. In most of the NoSQL databases we generally don't need SQL commands for any kind of modification in the database.
MongoDB ,Cassandra ,Redis ,Neo4j are some of the examples of NoSQL.
Types of NoSQL Databases
Key-Value Stores
Data Model: Simple key-value pairs.
Use Cases: Caching, session management, and real-time data.
Examples: Redis, Amazon DynamoDB, Riak.
Document Stores
Data Model: Documents (typically JSON or BSON) that can contain nested structures.
Use Cases: Content management, e-commerce, and real-time analytics.
Examples: MongoDB, CouchDB, Amazon DocumentDB.
Column-Family Stores
Data Model: Tables with rows and dynamic columns, grouped into column families.
Use Cases: Large-scale data warehousing, real-time data processing, and time-series data.
Examples: Apache Cassandra, HBase, ScyllaDB.
Graph Databases
Data Model: Graph structures with nodes, edges, and properties.
Use Cases: Social networks, recommendation engines, and network analysis.
Examples: Neo4j, Amazon Neptune, ArangoDB.
Introduction to MongoDB
MongoDB is a popular NoSQL document based database known for its flexibility and scalability. It stores data in a format similar to JSON (JavaScript Object Notation), called BSON (Binary JSON), which allows for nested documents and arrays.
JSON is nothing but a way to send and receive data between a sever and the web application .It is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate.
Uses of JSON is to exchange data , configure files and storing data.
Whereas BSON is advanced form of JSON where the data is stored in the form of binary values which is easy for the computer to read and traverse.
MongoDB stores data in the form of BSON internally, but for sending and retrieving data it is in form of JSON.
LETS start:
MongoDB is a case sensitive database.
Some commands in MongoDB
write mongosh in cmd
for showing current databases write:
show databases / show dbs.For selecting a database
select database_namefor viewing of the data inside a database
show collectionsto print all the data information inside a database
db.collection_name.find()to create a database
use database_name
it creates a new database if database is not present and selects a database if it is already present. Without adding a new collection mongoDB will not show any database when we will run the query.to add a new collection
db.createCollection("collection_name")to insert a record inside a collection
db.collection_name.insertOne({})db.collection_name.insertMany([{ }])
If there is space in collection name don't use space when writing any of the commands it may result to an error.to find number of records
db.collection_name.find().count()to get certain number of data records
db.collection_name.find().limit()filter records based on conditions
db.collection_name.find ({condition here})
projection in MongoDB
db.collection_name.find ({ filter1: value1....} , {property1: true .......})
deleting a document
db.collection_name.deleteOne({ condition to be deleted})
db.collection_name.deleteMany({ condition to be deleted})for updating
db.collection_name.updateOne({ filter1 : value1 } , {$ any_operator : {key : value }})
for distinct
db.collection_name.distinct("property")
we cant compare the float values directly therefore we need operator
In RDBMS data was stored in the form of table i.e. row represent information about a data record whereas column used to represent properties of an entity. But in case of MongoDB for storing data in the form of BSON /JSON and to represent these real life entity we use Collections. Collections are generally group of JSON documents.
MongoDB stores data in the form of BSON i.e. it has multiple key value pairs the key represent the property of an entity.
What row is for RDBMS document is for MongoDB and what column is for RDBMS key is for MongoDB.
Below is the coding demonstration
The below database is taken from GitHub
GitHub link:https://github.com/neelabalan/mongodb-sample-dataset
(c) Microsoft Corporation. All rights reserved.
C:\Users\Lenovo IG>mongosh
test> show dbs
admin 40.00 KiB
airbnb 52.01 MiB
config 108.00 KiB
local 88.00 KiB
movies 80.00 KiB
sales 968.00 KiB
students 8.00 KiB
university 40.00 KiB
weather 7.18 MiB
test>use weather
switched to db weather
weather> show collections
weather_
weather_data
weather>db.weather_.find()
[
{
_id: ObjectId("5553a998e4b02cf7151190b8"),
st: 'x+47600-047900',
ts: ISODate("1984-03-05T13:00:00.000Z"),
position: { type: 'Point', coordinates: [ -47.9, 47.6 ] },
elevation: 10,
callLetters: 'VCSZ',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -3.1, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1015.3, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a998e4b02cf7151190b9"),
st: 'x+45200-066500',
ts: ISODate("1984-03-05T14:00:00.000Z"),
position: { type: 'Point', coordinates: [ -66.5, 45.2 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -4.7, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1025.9, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a998e4b02cf7151190bb"),
st: 'x+60900-005300',
ts: ISODate("1984-03-05T15:00:00.000Z"),
position: { type: 'Point', coordinates: [ -5.3, 60.9 ] },
elevation: 9999,
callLetters: 'TFRB',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 7.5, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1018.5, quality: '1' },
wind: {
direction: { angle: 220, quality: '1' },
type: 'N',
speed: { rate: 12.3, quality: '1' }
},
visibility: {
distance: { value: 4000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 3, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '08', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '99', quality: '9' },
lowCloudGenus: { value: '99', quality: '9' },
lowestCloudBaseHeight: { value: 99999, quality: '9' },
midCloudGenus: { value: '99', quality: '9' },
highCloudGenus: { value: '99', quality: '9' }
},
presentWeatherObservationManual: [ { condition: '02', quality: '1' } ]
},
{
_id: ObjectId("5553a998e4b02cf7151190bc"),
st: 'x+66300-025200',
ts: ISODate("1984-03-05T15:00:00.000Z"),
position: { type: 'Point', coordinates: [ -25.2, 66.3 ] },
elevation: 9999,
callLetters: 'TFBY',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 0.4, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1003.6, quality: '1' },
wind: {
direction: { angle: 40, quality: '1' },
type: 'N',
speed: { rate: 6.7, quality: '1' }
},
visibility: {
distance: { value: 20000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 7500, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 3, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '05', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '02', quality: '1' },
lowCloudGenus: { value: '00', quality: '1' },
lowestCloudBaseHeight: { value: 1750, quality: '1' },
midCloudGenus: { value: '07', quality: '1' },
highCloudGenus: { value: '02', quality: '1' }
},
presentWeatherObservationManual: [ { condition: '02', quality: '1' } ]
},
{
_id: ObjectId("5553a998e4b02cf7151190bd"),
st: 'x+59800-029700',
ts: ISODate("1984-03-05T15:00:00.000Z"),
position: { type: 'Point', coordinates: [ -29.7, 59.8 ] },
elevation: 9999,
callLetters: 'TFWB',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 3.1, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1019, quality: '1' },
wind: {
direction: { angle: 250, quality: '1' },
type: 'N',
speed: { rate: 15.4, quality: '1' }
},
visibility: {
distance: { value: 10000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 750, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '1', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 3, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '07', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '07', quality: '1' },
lowCloudGenus: { value: '05', quality: '1' },
lowestCloudBaseHeight: { value: 800, quality: '1' },
midCloudGenus: { value: '00', quality: '1' },
highCloudGenus: { value: '00', quality: '1' }
},
presentWeatherObservationManual: [ { condition: '02', quality: '1' } ]
},
{
_id: ObjectId("5553a998e4b02cf7151190be"),
st: 'x+79800-172000',
ts: ISODate("1984-03-05T15:00:00.000Z"),
position: { type: 'Point', coordinates: [ -172, 79.8 ] },
elevation: 9999,
callLetters: 'EMIO',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -26.7, quality: '1' },
dewPoint: { value: -29.8, quality: '1' },
pressure: { value: 1016.6, quality: '1' },
wind: {
direction: { angle: 210, quality: '1' },
type: 'N',
speed: { rate: 10, quality: '1' }
},
visibility: {
distance: { value: 2100, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 2100, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MA1', 'MD1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 1 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '7', quality: '1' },
period: { value: 3, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '08', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '08', quality: '1' },
lowCloudGenus: { value: '00', quality: '1' },
lowestCloudBaseHeight: { value: 2250, quality: '1' },
midCloudGenus: { value: '01', quality: '1' },
highCloudGenus: { value: '99', quality: '9' }
},
atmosphericPressureObservation: {
altimeterSetting: { value: 9999.9, quality: '9' },
stationPressure: { value: 1016.6, quality: '1' }
},
atmosphericPressureChange: {
tendency: { code: '7', quality: '1' },
quantity3Hours: { value: 0.7, quality: '1' },
quantity24Hours: { value: 99.9, quality: '9' }
},
presentWeatherObservationManual: [ { condition: '71', quality: '1' } ]
},
{
_id: ObjectId("5553a998e4b02cf7151190bf"),
st: 'x+49700-055900',
ts: ISODate("1984-03-05T15:00:00.000Z"),
position: { type: 'Point', coordinates: [ -55.9, 49.7 ] },
elevation: 9999,
callLetters: 'SCGB',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -5.1, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1020.8, quality: '1' },
wind: {
direction: { angle: 100, quality: '1' },
type: 'N',
speed: { rate: 3.1, quality: '1' }
},
visibility: {
distance: { value: 20000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 22000, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MD1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 3, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '01', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '01', quality: '1' },
lowCloudGenus: { value: '01', quality: '1' },
lowestCloudBaseHeight: { value: 800, quality: '1' },
midCloudGenus: { value: '00', quality: '1' },
highCloudGenus: { value: '00', quality: '1' }
},
atmosphericPressureChange: {
tendency: { code: '8', quality: '1' },
quantity3Hours: { value: 0.5, quality: '1' },
quantity24Hours: { value: 99.9, quality: '9' }
},
presentWeatherObservationManual: [ { condition: '02', quality: '1' } ]
},
{
_id: ObjectId("5553a998e4b02cf7151190c1"),
st: 'x+55600+004800',
ts: ISODate("1984-03-05T15:00:00.000Z"),
position: { type: 'Point', coordinates: [ 4.8, 55.6 ] },
elevation: 9999,
callLetters: 'OWXP',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 6.6, quality: '1' },
dewPoint: { value: 4.4, quality: '1' },
pressure: { value: 1033.4, quality: '1' },
wind: {
direction: { angle: 320, quality: '1' },
type: 'N',
speed: { rate: 6.2, quality: '1' }
},
visibility: {
distance: { value: 20000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 22000, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [
'AG1', 'AY1',
'GF1', 'MD1',
'MW1', 'SA1',
'UA1'
],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '1', quality: '1' },
period: { value: 3, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '04', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '00', quality: '1' },
lowCloudGenus: { value: '00', quality: '1' },
lowestCloudBaseHeight: { value: 99999, quality: '9' },
midCloudGenus: { value: '00', quality: '1' },
highCloudGenus: { value: '08', quality: '1' }
},
atmosphericPressureChange: {
tendency: { code: '2', quality: '1' },
quantity3Hours: { value: 1.3, quality: '1' },
quantity24Hours: { value: 99.9, quality: '9' }
},
presentWeatherObservationManual: [ { condition: '01', quality: '1' } ],
seaSurfaceTemperature: { value: 3.5, quality: '9' },
waveMeasurement: {
method: 'M',
waves: { period: 2, height: 0.5, quality: '9' },
seaState: { code: '99', quality: '9' }
}
},
{
_id: ObjectId("5553a998e4b02cf7151190c2"),
st: 'x+68400+011800',
ts: ISODate("1984-03-05T15:00:00.000Z"),
position: { type: 'Point', coordinates: [ 11.8, 68.4 ] },
elevation: 9999,
callLetters: 'LAQU',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 2, quality: '1' },
dewPoint: { value: -2, quality: '1' },
pressure: { value: 999.3, quality: '1' },
wind: {
direction: { angle: 280, quality: '1' },
type: 'N',
speed: { rate: 12.3, quality: '1' }
},
visibility: {
distance: { value: 4000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 420, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [
'AG1', 'AY1',
'GF1', 'MD1',
'MW1', 'SA1',
'UA1'
],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 2 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '8', quality: '1' },
period: { value: 3, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '08', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '08', quality: '1' },
lowCloudGenus: { value: '09', quality: '1' },
lowestCloudBaseHeight: { value: 450, quality: '1' },
midCloudGenus: { value: '99', quality: '9' },
highCloudGenus: { value: '99', quality: '9' }
},
atmosphericPressureChange: {
tendency: { code: '2', quality: '1' },
quantity3Hours: { value: 1.3, quality: '1' },
quantity24Hours: { value: 99.9, quality: '9' }
},
presentWeatherObservationManual: [ { condition: '26', quality: '1' } ],
seaSurfaceTemperature: { value: 6, quality: '9' },
waveMeasurement: {
method: 'M',
waves: { period: 6, height: 3.5, quality: '9' },
seaState: { code: '99', quality: '9' }
}
},
{
_id: ObjectId("5553a998e4b02cf7151190c4"),
st: 'x+47600-000700',
ts: ISODate("1984-03-05T15:00:00.000Z"),
position: { type: 'Point', coordinates: [ -0.7, 47.6 ] },
elevation: 9999,
callLetters: 'FNUI',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 999.9, quality: '9' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1032.6, quality: '1' },
wind: {
direction: { angle: 60, quality: '1' },
type: 'N',
speed: { rate: 7.2, quality: '1' }
},
visibility: {
distance: { value: 50000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 22000, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [
'AG1', 'AY1',
'GF1', 'MD1',
'MW1', 'SA1',
'UA1'
],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 3, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '01', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '99', quality: '9' },
lowCloudGenus: { value: '00', quality: '1' },
lowestCloudBaseHeight: { value: 450, quality: '1' },
midCloudGenus: { value: '04', quality: '1' },
highCloudGenus: { value: '00', quality: '1' }
},
atmosphericPressureChange: {
tendency: { code: '4', quality: '1' },
quantity3Hours: { value: 0, quality: '1' },
quantity24Hours: { value: 99.9, quality: '9' }
},
presentWeatherObservationManual: [ { condition: '01', quality: '1' } ],
seaSurfaceTemperature: { value: 12.7, quality: '9' },
waveMeasurement: {
method: 'M',
waves: { period: 2, height: 1.5, quality: '9' },
seaState: { code: '99', quality: '9' }
}
},
{
_id: ObjectId("5553a998e4b02cf7151190c5"),
st: 'x+43700-059700',
ts: ISODate("1984-03-05T15:00:00.000Z"),
position: { type: 'Point', coordinates: [ -59.7, 43.7 ] },
elevation: 9999,
callLetters: 'VCNP',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -4.5, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1024.6, quality: '1' },
wind: {
direction: { angle: 340, quality: '1' },
type: 'N',
speed: { rate: 11.3, quality: '1' }
},
visibility: {
distance: { value: 2000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 900, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [
'AG1', 'AY1',
'GF1', 'MD1',
'MW1', 'SA1',
'UA1', 'UG1'
],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 1 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '8', quality: '1' },
period: { value: 3, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '08', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '08', quality: '1' },
lowCloudGenus: { value: '08', quality: '1' },
lowestCloudBaseHeight: { value: 450, quality: '1' },
midCloudGenus: { value: '99', quality: '9' },
highCloudGenus: { value: '99', quality: '9' }
},
atmosphericPressureChange: {
tendency: { code: '1', quality: '1' },
quantity3Hours: { value: 0.8, quality: '1' },
quantity24Hours: { value: 99.9, quality: '9' }
},
presentWeatherObservationManual: [ { condition: '85', quality: '1' } ],
seaSurfaceTemperature: { value: 1, quality: '9' },
waveMeasurement: {
method: 'M',
waves: { period: 5, height: 1, quality: '9' },
seaState: { code: '99', quality: '9' }
}
},
{
_id: ObjectId("5553a998e4b02cf7151190c6"),
st: 'x-39600+173400',
ts: ISODate("1984-03-05T15:00:00.000Z"),
position: { type: 'Point', coordinates: [ 173.4, -39.6 ] },
elevation: 9999,
callLetters: 'SINL',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 17.2, quality: '1' },
dewPoint: { value: 16.5, quality: '1' },
pressure: { value: 1009.1, quality: '1' },
wind: {
direction: { angle: 110, quality: '1' },
type: 'N',
speed: { rate: 2.1, quality: '1' }
},
visibility: {
distance: { value: 20000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [
'AG1', 'AY1',
'GF1', 'MD1',
'MW1', 'SA1',
'UA1', 'UG1'
],
precipitationEstimatedObservation: { discrepancy: '0', estimatedWaterDepth: 999 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '6', quality: '1' },
period: { value: 3, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '08', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '99', quality: '9' },
lowCloudGenus: { value: '99', quality: '9' },
lowestCloudBaseHeight: { value: 99999, quality: '9' },
midCloudGenus: { value: '99', quality: '9' },
highCloudGenus: { value: '99', quality: '9' }
},
atmosphericPressureChange: {
tendency: { code: '8', quality: '1' },
quantity3Hours: { value: 0.5, quality: '1' },
quantity24Hours: { value: 99.9, quality: '9' }
},
presentWeatherObservationManual: [ { condition: '15', quality: '1' } ],
seaSurfaceTemperature: { value: 19, quality: '9' },
waveMeasurement: {
method: 'M',
waves: { period: 0, height: 0, quality: '9' },
seaState: { code: '99', quality: '9' }
}
},
{
_id: ObjectId("5553a998e4b02cf7151190c7"),
st: 'x+67400+010500',
ts: ISODate("1984-03-05T15:00:00.000Z"),
position: { type: 'Point', coordinates: [ 10.5, 67.4 ] },
elevation: 9999,
callLetters: 'UUQR',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 0.9, quality: '1' },
dewPoint: { value: -4.3, quality: '1' },
pressure: { value: 1002.8, quality: '1' },
wind: {
direction: { angle: 260, quality: '1' },
type: 'N',
speed: { rate: 11, quality: '1' }
},
visibility: {
distance: { value: 200, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 30, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [
'AG1', 'AY1',
'GA1', 'GF1',
'MD1', 'MW1',
'SA1', 'UA1',
'UG1'
],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 2 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '8', quality: '1' },
period: { value: 3, quality: '1' }
}
],
skyCoverLayer: [
{
coverage: { value: '09', quality: '1' },
baseHeight: { value: 99999, quality: '9' },
cloudType: { value: '99', quality: '9' }
}
],
skyConditionObservation: {
totalCoverage: { value: '09', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '09', quality: '1' },
lowCloudGenus: { value: '09', quality: '1' },
lowestCloudBaseHeight: { value: 250, quality: '1' },
midCloudGenus: { value: '99', quality: '9' },
highCloudGenus: { value: '99', quality: '9' }
},
atmosphericPressureChange: {
tendency: { code: '2', quality: '1' },
quantity3Hours: { value: 2.5, quality: '1' },
quantity24Hours: { value: 99.9, quality: '9' }
},
presentWeatherObservationManual: [ { condition: '26', quality: '1' } ],
seaSurfaceTemperature: { value: 8.9, quality: '9' },
waveMeasurement: {
method: 'M',
waves: { period: 5, height: 2, quality: '9' },
seaState: { code: '99', quality: '9' }
}
},
{
_id: ObjectId("5553a998e4b02cf7151190ca"),
st: 'x+21100+116200',
ts: ISODate("1984-03-05T18:00:00.000Z"),
position: { type: 'Point', coordinates: [ 116.2, 21.1 ] },
elevation: 9999,
callLetters: 'UYOK',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 17, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1017.2, quality: '1' },
wind: {
direction: { angle: 40, quality: '1' },
type: 'N',
speed: { rate: 10, quality: '1' }
},
visibility: {
distance: { value: 20000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 22000, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 6, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '00', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '00', quality: '1' },
lowCloudGenus: { value: '00', quality: '1' },
lowestCloudBaseHeight: { value: 99999, quality: '9' },
midCloudGenus: { value: '00', quality: '1' },
highCloudGenus: { value: '00', quality: '1' }
},
presentWeatherObservationManual: [ { condition: '02', quality: '1' } ]
},
{
_id: ObjectId("5553a998e4b02cf7151190cb"),
st: 'x+43400-065600',
ts: ISODate("1984-03-05T18:00:00.000Z"),
position: { type: 'Point', coordinates: [ -65.6, 43.4 ] },
elevation: 9999,
callLetters: 'CG26',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 999.9, quality: '9' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 9999.9, quality: '9' },
wind: {
direction: { angle: 270, quality: '1' },
type: 'N',
speed: { rate: 7.7, quality: '1' }
},
visibility: {
distance: { value: 10000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 22000, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 6, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '04', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '99', quality: '9' },
lowCloudGenus: { value: '99', quality: '9' },
lowestCloudBaseHeight: { value: 99999, quality: '9' },
midCloudGenus: { value: '99', quality: '9' },
highCloudGenus: { value: '99', quality: '9' }
},
presentWeatherObservationManual: [ { condition: '02', quality: '1' } ]
},
{
_id: ObjectId("5553a998e4b02cf7151190cc"),
st: 'x+47400-070400',
ts: ISODate("1984-03-05T18:00:00.000Z"),
position: { type: 'Point', coordinates: [ -70.4, 47.4 ] },
elevation: 9999,
callLetters: 'VA71',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 999.9, quality: '9' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 9999.9, quality: '9' },
wind: {
direction: { angle: 180, quality: '1' },
type: 'N',
speed: { rate: 3.6, quality: '1' }
},
visibility: {
distance: { value: 10000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 6, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '07', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '99', quality: '9' },
lowCloudGenus: { value: '99', quality: '9' },
lowestCloudBaseHeight: { value: 99999, quality: '9' },
midCloudGenus: { value: '99', quality: '9' },
highCloudGenus: { value: '99', quality: '9' }
},
presentWeatherObservationManual: [ { condition: '02', quality: '1' } ]
},
{
_id: ObjectId("5553a998e4b02cf7151190cd"),
st: 'x+55300+005000',
ts: ISODate("1984-03-05T18:00:00.000Z"),
position: { type: 'Point', coordinates: [ 5, 55.3 ] },
elevation: 9999,
callLetters: 'SHIP',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 6.7, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1032, quality: '1' },
wind: {
direction: { angle: 290, quality: '1' },
type: 'N',
speed: { rate: 7.2, quality: '1' }
},
visibility: {
distance: { value: 10000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 22000, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 6, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '03', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '00', quality: '1' },
lowCloudGenus: { value: '00', quality: '1' },
lowestCloudBaseHeight: { value: 99999, quality: '9' },
midCloudGenus: { value: '00', quality: '1' },
highCloudGenus: { value: '05', quality: '1' }
},
presentWeatherObservationManual: [ { condition: '02', quality: '1' } ]
},
{
_id: ObjectId("5553a998e4b02cf7151190ce"),
st: 'x+63200-016100',
ts: ISODate("1984-03-05T18:00:00.000Z"),
position: { type: 'Point', coordinates: [ -16.1, 63.2 ] },
elevation: 9999,
callLetters: 'TFWI',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 4.8, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1007.2, quality: '1' },
wind: {
direction: { angle: 270, quality: '1' },
type: 'N',
speed: { rate: 19, quality: '1' }
},
visibility: {
distance: { value: 10000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 2 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '8', quality: '1' },
period: { value: 6, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '06', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '99', quality: '9' },
lowCloudGenus: { value: '99', quality: '9' },
lowestCloudBaseHeight: { value: 1750, quality: '1' },
midCloudGenus: { value: '99', quality: '9' },
highCloudGenus: { value: '99', quality: '9' }
},
presentWeatherObservationManual: [ { condition: '26', quality: '1' } ]
},
{
_id: ObjectId("5553a998e4b02cf7151190cf"),
st: 'x-19300+060300',
ts: ISODate("1984-03-05T18:00:00.000Z"),
position: { type: 'Point', coordinates: [ 60.3, -19.3 ] },
elevation: 9999,
callLetters: 'FNPG',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 26.2, quality: '1' },
dewPoint: { value: 21.1, quality: '1' },
pressure: { value: 1016.2, quality: '1' },
wind: {
direction: { angle: 90, quality: '1' },
type: 'N',
speed: { rate: 9.8, quality: '1' }
},
visibility: {
distance: { value: 50000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 22000, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '0', estimatedWaterDepth: 999 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 6, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '00', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '00', quality: '1' },
lowCloudGenus: { value: '00', quality: '1' },
lowestCloudBaseHeight: { value: 99999, quality: '9' },
midCloudGenus: { value: '00', quality: '1' },
highCloudGenus: { value: '00', quality: '1' }
},
presentWeatherObservationManual: [ { condition: '02', quality: '1' } ]
},
{
_id: ObjectId("5553a998e4b02cf7151190d0"),
st: 'x+24700-061200',
ts: ISODate("1984-03-05T18:00:00.000Z"),
position: { type: 'Point', coordinates: [ -61.2, 24.7 ] },
elevation: 9999,
callLetters: 'URUX',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 20.7, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1020.4, quality: '1' },
wind: {
direction: { angle: 50, quality: '1' },
type: 'N',
speed: { rate: 9, quality: '1' }
},
visibility: {
distance: { value: 50000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 22000, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MD1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 6, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '06', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '02', quality: '1' },
lowCloudGenus: { value: '05', quality: '1' },
lowestCloudBaseHeight: { value: 800, quality: '1' },
midCloudGenus: { value: '00', quality: '1' },
highCloudGenus: { value: '04', quality: '1' }
},
atmosphericPressureChange: {
tendency: { code: '2', quality: '1' },
quantity3Hours: { value: 6, quality: '1' },
quantity24Hours: { value: 99.9, quality: '9' }
},
presentWeatherObservationManual: [ { condition: '02', quality: '1' } ]
}
]
Type "it" for more
weather> db.weather_.find().count()
9472
weather> db.weather_.find().limit(2)
[
{
_id: ObjectId("5553a998e4b02cf7151190b8"),
st: 'x+47600-047900',
ts: ISODate("1984-03-05T13:00:00.000Z"),
position: { type: 'Point', coordinates: [ -47.9, 47.6 ] },
elevation: 10,
callLetters: 'VCSZ',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -3.1, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1015.3, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a998e4b02cf7151190b9"),
st: 'x+45200-066500',
ts: ISODate("1984-03-05T14:00:00.000Z"),
position: { type: 'Point', coordinates: [ -66.5, 45.2 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -4.7, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1025.9, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
}
]
weather> db.weather_.find({callLetters :'VC81'})
[
{
_id: ObjectId("5553a998e4b02cf7151190b9"),
st: 'x+45200-066500',
ts: ISODate("1984-03-05T14:00:00.000Z"),
position: { type: 'Point', coordinates: [ -66.5, 45.2 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -4.7, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1025.9, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a998e4b02cf7151196d5"),
st: 'x+45100-066300',
ts: ISODate("1984-03-06T02:00:00.000Z"),
position: { type: 'Point', coordinates: [ -66.3, 45.1 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -3.2, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1019.3, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a998e4b02cf7151198a0"),
st: 'x+45200-066400',
ts: ISODate("1984-03-05T13:00:00.000Z"),
position: { type: 'Point', coordinates: [ -66.4, 45.2 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -7.8, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1026.4, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a998e4b02cf715119a94"),
st: 'x+45100-066500',
ts: ISODate("1984-03-05T19:00:00.000Z"),
position: { type: 'Point', coordinates: [ -66.5, 45.1 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -3.7, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1023.2, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a998e4b02cf715119b43"),
st: 'x+45100-066300',
ts: ISODate("1984-03-06T00:00:00.000Z"),
position: { type: 'Point', coordinates: [ -66.3, 45.1 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -2.7, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1020.4, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 }
},
{
_id: ObjectId("5553a998e4b02cf715119d07"),
st: 'x+45100-066400',
ts: ISODate("1984-03-05T21:00:00.000Z"),
position: { type: 'Point', coordinates: [ -66.4, 45.1 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -3.2, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1022.3, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 }
},
{
_id: ObjectId("5553a999e4b02cf71511b86e"),
st: 'x+42200-068400',
ts: ISODate("1984-03-07T23:00:00.000Z"),
position: { type: 'Point', coordinates: [ -68.4, 42.2 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 2.8, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1012.7, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a999e4b02cf71511b8e2"),
st: 'x+41200-069100',
ts: ISODate("1984-03-08T01:00:00.000Z"),
position: { type: 'Point', coordinates: [ -69.1, 41.2 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 2.5, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1014.9, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a999e4b02cf71511bc99"),
st: 'x+42900-068000',
ts: ISODate("1984-03-07T14:00:00.000Z"),
position: { type: 'Point', coordinates: [ -68, 42.9 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 0.5, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1010.5, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a999e4b02cf71511beac"),
st: 'x+39800-070000',
ts: ISODate("1984-03-08T10:00:00.000Z"),
position: { type: 'Point', coordinates: [ -70, 39.8 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -1.2, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1020.4, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a999e4b02cf71511c0dd"),
st: 'x+39600-070200',
ts: ISODate("1984-03-08T15:00:00.000Z"),
position: { type: 'Point', coordinates: [ -70.2, 39.6 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: -0.2, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1022.6, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 }
},
{
_id: ObjectId("5553a999e4b02cf71511c14d"),
st: 'x+42100-068400',
ts: ISODate("1984-03-07T18:00:00.000Z"),
position: { type: 'Point', coordinates: [ -68.4, 42.1 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 999.9, quality: '9' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 9999.9, quality: '9' },
wind: {
direction: { angle: 350, quality: '1' },
type: 'N',
speed: { rate: 2.6, quality: '1' }
},
visibility: {
distance: { value: 20000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 22000, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 6, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '02', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '99', quality: '9' },
lowCloudGenus: { value: '99', quality: '9' },
lowestCloudBaseHeight: { value: 99999, quality: '9' },
midCloudGenus: { value: '99', quality: '9' },
highCloudGenus: { value: '99', quality: '9' }
},
presentWeatherObservationManual: [ { condition: '01', quality: '1' } ]
},
{
_id: ObjectId("5553a999e4b02cf71511c265"),
st: 'x+39700-070200',
ts: ISODate("1984-03-08T12:00:00.000Z"),
position: { type: 'Point', coordinates: [ -70.2, 39.7 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 999.9, quality: '9' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 9999.9, quality: '9' },
wind: {
direction: { angle: 300, quality: '1' },
type: 'N',
speed: { rate: 5.7, quality: '1' }
},
visibility: {
distance: { value: 20000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 22000, quality: '1', determination: 'C' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 6, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '01', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '99', quality: '9' },
lowCloudGenus: { value: '99', quality: '9' },
lowestCloudBaseHeight: { value: 99999, quality: '9' },
midCloudGenus: { value: '99', quality: '9' },
highCloudGenus: { value: '99', quality: '9' }
},
presentWeatherObservationManual: [ { condition: '02', quality: '1' } ]
},
{
_id: ObjectId("5553a999e4b02cf71511e08f"),
st: 'x+36700-072700',
ts: ISODate("1984-03-10T06:00:00.000Z"),
position: { type: 'Point', coordinates: [ -72.7, 36.7 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 999.9, quality: '9' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 9999.9, quality: '9' },
wind: {
direction: { angle: 320, quality: '1' },
type: 'N',
speed: { rate: 7.7, quality: '1' }
},
visibility: {
distance: { value: 20000, quality: '1' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1', 'AY1', 'GF1', 'MW1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 },
pastWeatherObservationManual: [
{
atmosphericCondition: { value: '0', quality: '1' },
period: { value: 6, quality: '1' }
}
],
skyConditionObservation: {
totalCoverage: { value: '06', opaque: '99', quality: '1' },
lowestCloudCoverage: { value: '99', quality: '9' },
lowCloudGenus: { value: '99', quality: '9' },
lowestCloudBaseHeight: { value: 99999, quality: '9' },
midCloudGenus: { value: '99', quality: '9' },
highCloudGenus: { value: '99', quality: '9' }
},
presentWeatherObservationManual: [ { condition: '01', quality: '1' } ]
},
{
_id: ObjectId("5553a999e4b02cf71511e221"),
st: 'x+36100-073800',
ts: ISODate("1984-03-10T20:00:00.000Z"),
position: { type: 'Point', coordinates: [ -73.8, 36.1 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 3.1, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1026, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a999e4b02cf71511e3ef"),
st: 'x+36400-073200',
ts: ISODate("1984-03-10T14:00:00.000Z"),
position: { type: 'Point', coordinates: [ -73.2, 36.4 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 2.7, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1026.7, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a99ae4b02cf71512068b"),
st: 'x+32800-077500',
ts: ISODate("1984-03-12T09:00:00.000Z"),
position: { type: 'Point', coordinates: [ -77.5, 32.8 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 15, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1024.4, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 0 }
},
{
_id: ObjectId("5553a99ae4b02cf71512097b"),
st: 'x+33400-077000',
ts: ISODate("1984-03-12T01:00:00.000Z"),
position: { type: 'Point', coordinates: [ -77, 33.4 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 14.2, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1021.2, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a99ae4b02cf715120aeb"),
st: 'x+32800-077500',
ts: ISODate("1984-03-12T11:00:00.000Z"),
position: { type: 'Point', coordinates: [ -77.5, 32.8 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 15.3, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1025.5, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
},
{
_id: ObjectId("5553a99ae4b02cf715120cc2"),
st: 'x+30600-078400',
ts: ISODate("1984-03-13T01:00:00.000Z"),
position: { type: 'Point', coordinates: [ -78.4, 30.6 ] },
elevation: 9999,
callLetters: 'VC81',
qualityControlProcess: 'V020',
dataSource: '4',
type: 'FM-13',
airTemperature: { value: 21, quality: '1' },
dewPoint: { value: 999.9, quality: '9' },
pressure: { value: 1023.5, quality: '1' },
wind: {
direction: { angle: 999, quality: '9' },
type: '9',
speed: { rate: 999.9, quality: '9' }
},
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
},
skyCondition: {
ceilingHeight: { value: 99999, quality: '9', determination: '9' },
cavok: 'N'
},
sections: [ 'AG1' ],
precipitationEstimatedObservation: { discrepancy: '2', estimatedWaterDepth: 999 }
}
]
Type "it" for more
// find count
weather> db.weather_.find({callLetters :'VC81'}).count()
22
// projections
weather> db.weather_.find({callLetters :'VC81'},{elevation : true , position: true , visibility: true})
[
{
_id: ObjectId("5553a998e4b02cf7151190b9"),
position: { type: 'Point', coordinates: [ -66.5, 45.2 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a998e4b02cf7151196d5"),
position: { type: 'Point', coordinates: [ -66.3, 45.1 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a998e4b02cf7151198a0"),
position: { type: 'Point', coordinates: [ -66.4, 45.2 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a998e4b02cf715119a94"),
position: { type: 'Point', coordinates: [ -66.5, 45.1 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a998e4b02cf715119b43"),
position: { type: 'Point', coordinates: [ -66.3, 45.1 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a998e4b02cf715119d07"),
position: { type: 'Point', coordinates: [ -66.4, 45.1 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a999e4b02cf71511b86e"),
position: { type: 'Point', coordinates: [ -68.4, 42.2 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a999e4b02cf71511b8e2"),
position: { type: 'Point', coordinates: [ -69.1, 41.2 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a999e4b02cf71511bc99"),
position: { type: 'Point', coordinates: [ -68, 42.9 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a999e4b02cf71511beac"),
position: { type: 'Point', coordinates: [ -70, 39.8 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a999e4b02cf71511c0dd"),
position: { type: 'Point', coordinates: [ -70.2, 39.6 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a999e4b02cf71511c14d"),
position: { type: 'Point', coordinates: [ -68.4, 42.1 ] },
elevation: 9999,
visibility: {
distance: { value: 20000, quality: '1' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a999e4b02cf71511c265"),
position: { type: 'Point', coordinates: [ -70.2, 39.7 ] },
elevation: 9999,
visibility: {
distance: { value: 20000, quality: '1' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a999e4b02cf71511e08f"),
position: { type: 'Point', coordinates: [ -72.7, 36.7 ] },
elevation: 9999,
visibility: {
distance: { value: 20000, quality: '1' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a999e4b02cf71511e221"),
position: { type: 'Point', coordinates: [ -73.8, 36.1 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a999e4b02cf71511e3ef"),
position: { type: 'Point', coordinates: [ -73.2, 36.4 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a99ae4b02cf71512068b"),
position: { type: 'Point', coordinates: [ -77.5, 32.8 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a99ae4b02cf71512097b"),
position: { type: 'Point', coordinates: [ -77, 33.4 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a99ae4b02cf715120aeb"),
position: { type: 'Point', coordinates: [ -77.5, 32.8 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
},
{
_id: ObjectId("5553a99ae4b02cf715120cc2"),
position: { type: 'Point', coordinates: [ -78.4, 30.6 ] },
elevation: 9999,
visibility: {
distance: { value: 999999, quality: '9' },
variability: { value: 'N', quality: '9' }
}
}
]
Type "it" for more
// deleting
weather> db.weather_.deleteOne({callLetters :'VC81'})
{ acknowledged: true, deletedCount: 1 }
weather> db.weather_.find({callLetters :'VC81'}).count()
20
weather> db.weather_.deleteMany({callLetters :'VC81'})
{ acknowledged: true, deletedCount: 20 }
weather> db.weather_.find({callLetters :'VC81'}).count()
0
// update
weather> db.weather_.updateOne({callLetters :'VC81'} ,{$set:{elevation:10}})
{
acknowledged: true,
insertedId: null,
matchedCount: 0,
modifiedCount: 0,
upsertedCount: 0
}
//for distinct
weather> db.weather_.distinct("type")
[ 'FM-13', 'SAO' ]
weather> db.weather_.distinct('type')
[ 'FM-13', 'SAO' ]
weather> db.weather_.find({type :'SAO'}).count()
6
weather> db.weather_.find({type :'FM-13'}).count()
9444
weather> db.weather_.find().count()
9450
Operators in MongoDB
Comparison Operators
$eq
: Matches values that are equal to a specified value.$ne
: Matches values that are not equal to a specified value.$gt
: Matches values that are greater than a specified value.$gte
: Matches values that are greater than or equal to a specified value.$lt
: Matches values that are less than a specified value.$lte
: Matches values that are less than or equal to a specified value.$in
: Matches any of the values specified in an array.$nin
: Matches none of the values specified in an array.
Logical Operators
$and
: Joins query clauses with a logical AND.$or
: Joins query clauses with a logical OR.$not
: Inverts the effect of a query expression.$nor
: Joins query clauses with a logical NOR.
Element Operators
$exists
: Matches documents that have the specified field.$type
: Matches documents with the specified field type.
Field Update Operators
$set
: Sets the value of a field in a document.$unset
: Removes the specified field from a document.$inc
: Increments the value of a field by a specified amount.$mul
: Multiplies the value of a field by a specified amount.$rename
: Renames a field.$min
: Updates the field to a specified value if the specified value is less than the current value of the field.$max
: Updates the field to a specified value if the specified value is greater than the current value of the field.
Array Update Operators
$push
: Adds an element to an array.$pop
: Removes the first or last element of an array.$pull
: Removes all array elements that match a specified query.$addToSet
: Adds elements to an array only if they do not already exist in the array.$each
: Modifies the$push
and$addToSet
operators to add multiple items to an array.
Arithmetic Operators
$add
: Adds numbers together or adds numbers and dates.$subtract
: Subtracts two numbers or two dates.$multiply
: Multiplies numbers.$divide
: Divides one number by another.$mod
: Takes the remainder of the division of two numbers.
Array Operators
$arrayElemAt
: Returns the element at the specified array index.$concatArrays
: Concatenates arrays to return the result.$filter
: Selects a subset of an array to return based on the specified condition.$size
: Returns the number of elements in an array.
String Operators
$concat
: Concatenates strings.$substr
: Returns a substring of a string.$toLower
: Converts a string to lowercase.$toUpper
: Converts a string to uppercase.$trim
: Removes whitespace or other specified characters from the beginning and end of a string.
Conditional Operators
$cond
: A ternary operator that evaluates a condition and returns one of two expressions.$ifNull
: Evaluates an expression and returns a specified value if the expression is null.
Whenever we have to access the nested key value pairs then we have to provide the key in form of a string.
Coding demonstration of operators
weather> db.weather_.find({type: {$ne :'FM-13'}}).count()
6
weather> db.weather_.find({type: {$eq :'FM-13'}}).count()
9444
weather> db.weather_.find({"visibility.distance.value": {$eq :0}}).count()
31
weather> db.weather_.find({"visibility.distance.value": {$eq :0}},
{wind : true,callLetters : true})
weather> db.weather_.find({$and:[{"wind.direction.angle": {$gt :22}},
{"wind.direction.angle":{$lt : 32}}]}).count()
255
// explain execution stats
weather> db.weather_.find({type :'SAO'}).explain("executionStats")
//get index
weather> db.weather_.getIndexes()
[ { v: 2, key: { _id: 1 }, name: '_id_' } ]
//create Index
weather> db.weather_data.createIndex({elevation : 1}) //1 for ascending order
elevation_1
weather> db.weather_.getIndexes()
[
{ v: 2, key: { _id: 1 }, name: '_id_' },
{ v: 2, key: { elevation: 1 }, name: 'elevation_1' }
]
//running execution stats
weather> db.weather_.find({elevation: {$lt :10000}}).explain("executionStats")
{
explainVersion: '2',
queryPlanner: {
namespace: 'weather.weather_',
indexFilterSet: false,
parsedQuery: { elevation: { '$lt': 10000 } },
queryHash: '48238B16',
planCacheKey: '799F462C',
maxIndexedOrSolutionsReached: false,
maxIndexedAndSolutionsReached: false,
maxScansToExplodeReached: false,
winningPlan: {
queryPlan: {
stage: 'FETCH',
planNodeId: 2,
inputStage: {
stage: 'IXSCAN',
planNodeId: 1,
keyPattern: { elevation: 1 },
indexName: 'elevation_1',
isMultiKey: false,
multiKeyPaths: { elevation: [] },
isUnique: false,
isSparse: false,
isPartial: false,
indexVersion: 2,
direction: 'forward',
indexBounds: { elevation: [ '[-inf.0, 10000)' ] }
}
},
slotBasedPlan: {
slots: '$$RESULT=s11 env: { s5 = KS(1F00000000000000000104), s2 = Nothing (SEARCH_META), s1 = TimeZoneDatabase(America/Argentina/Tucuman...Asia/Dubai) (timeZoneDB), s6 = KS(2C4E200104), s10 = {"elevation" : 1}, s3 = 1720332139755 (NOW) }',
stages: '[2] nlj inner [] [s4, s7, s8, s9, s10] \n' +
' left \n' +
' [1] cfilter {(exists(s5) && exists(s6))} \n' +
' [1] ixseek s5 s6 s9 s4 s7 s8 [] @"9093bb45-8f87-4c90-8ef0-1644fcc57e2f" @"elevation_1" true \n' +
' right \n' +
' [2] limit 1 \n' +
' [2] seek s4 s11 s12 s7 s8 s9 s10 [] @"9093bb45-8f87-4c90-8ef0-1644fcc57e2f" true false \n'
}
},
rejectedPlans: []
},
executionStats: {
executionSuccess: true,
nReturned: 9450,
executionTimeMillis: 14,
totalKeysExamined: 9450,
totalDocsExamined: 9450,
executionStages: {
stage: 'nlj',
planNodeId: 2,
nReturned: 9450,
executionTimeMillisEstimate: 14,
opens: 1,
closes: 1,
saveState: 9,
restoreState: 9,
isEOF: 1,
totalDocsExamined: 9450,
totalKeysExamined: 9450,
collectionScans: 0,
collectionSeeks: 9450,
indexScans: 0,
indexSeeks: 1,
indexesUsed: [ 'elevation_1' ],
innerOpens: 9450,
innerCloses: 1,
outerProjects: [],
outerCorrelated: [ Long("4"), Long("7"), Long("8"), Long("9"), Long("10") ],
outerStage: {
stage: 'cfilter',
planNodeId: 1,
nReturned: 9450,
executionTimeMillisEstimate: 14,
opens: 1,
closes: 1,
saveState: 9,
restoreState: 9,
isEOF: 1,
numTested: 1,
filter: '(exists(s5) && exists(s6)) ',
inputStage: {
stage: 'ixseek',
planNodeId: 1,
nReturned: 9450,
executionTimeMillisEstimate: 14,
opens: 1,
closes: 1,
saveState: 9,
restoreState: 9,
isEOF: 1,
indexName: 'elevation_1',
keysExamined: 9450,
seeks: 1,
numReads: 9451,
indexKeySlot: 9,
recordIdSlot: 4,
snapshotIdSlot: 7,
indexIdentSlot: 8,
outputSlots: [],
indexKeysToInclude: '00000000000000000000000000000000',
seekKeyLow: 's5 ',
seekKeyHigh: 's6 '
}
},
innerStage: {
stage: 'limit',
planNodeId: 2,
nReturned: 9450,
executionTimeMillisEstimate: 0,
opens: 9450,
closes: 1,
saveState: 9,
restoreState: 9,
isEOF: 1,
limit: 1,
inputStage: {
stage: 'seek',
planNodeId: 2,
nReturned: 9450,
executionTimeMillisEstimate: 0,
opens: 9450,
closes: 1,
saveState: 9,
restoreState: 9,
isEOF: 0,
numReads: 9450,
recordSlot: 11,
recordIdSlot: 12,
seekKeySlot: 4,
snapshotIdSlot: 7,
indexIdentSlot: 8,
indexKeySlot: 9,
indexKeyPatternSlot: 10,
fields: [],
outputSlots: []
}
}
}
},
command: {
find: 'weather_',
filter: { elevation: { '$lt': 10000 } },
'$db': 'weather'
},
serverInfo: {
host: 'Anonymous',
port: 27017,
version: '7.0.0',
gitVersion: '37d84072b5c5b9fd723db5fa133fb202ad2317f1'
},
serverParameters: {
internalQueryFacetBufferSizeBytes: 104857600,
internalQueryFacetMaxOutputDocSizeBytes: 104857600,
internalLookupStageIntermediateDocumentMaxSizeBytes: 104857600,
internalDocumentSourceGroupMaxMemoryBytes: 104857600,
internalQueryMaxBlockingSortMemoryUsageBytes: 104857600,
internalQueryProhibitBlockingMergeOnMongoS: 0,
internalQueryMaxAddToSetBytes: 104857600,
internalDocumentSourceSetWindowFieldsMaxMemoryBytes: 104857600,
internalQueryFrameworkControl: 'trySbeEngine'
},
ok: 1
}
weather>
//creating airbnb index
airbnb> db.names.createIndex({ number_of_reviews : 1})
number_of_reviews_1
airbnb> db.names.getIndexes()
[
{ v: 2, key: { _id: 1 }, name: '_id_' },
{ v: 2, key: { number_of_reviews: 1 }, name: 'number_of_reviews_1' }
]
//searching using different techniques
airbnb> db.names.find({number_of_reviews : {$gt : 5}}).explain("executionStats")
{
explainVersion: '2',
queryPlanner: {
namespace: 'airbnb.names',
indexFilterSet: false,
parsedQuery: { number_of_reviews: { '$gt': 5 } },
queryHash: 'A09CB50A',
planCacheKey: '890B366F',
maxIndexedOrSolutionsReached: false,
maxIndexedAndSolutionsReached: false,
maxScansToExplodeReached: false,
winningPlan: {
queryPlan: {
stage: 'FETCH',
planNodeId: 2,
inputStage: {
stage: 'IXSCAN',
planNodeId: 1,
keyPattern: { number_of_reviews: 1 },
indexName: 'number_of_reviews_1',
isMultiKey: false,
multiKeyPaths: { number_of_reviews: [] },
isUnique: false,
isSparse: false,
isPartial: false,
indexVersion: 2,
direction: 'forward',
indexBounds: { number_of_reviews: [ '(5, inf.0]' ] }
}
},
slotBasedPlan: {
slots: '$$RESULT=s11 env: { s1 = TimeZoneDatabase(America/Argentina/Tucuman...Asia/Dubai) (timeZoneDB), s6 = KS(33FFFFFFFFFFFFFFFFFE04), s3 = 1720334526009 (NOW), s10 = {"number_of_reviews" : 1}, s5 = KS(2B0AFE04), s2 = Nothing (SEARCH_META) }',
stages: '[2] nlj inner [] [s4, s7, s8, s9, s10] \n' +
' left \n' +
' [1] cfilter {(exists(s5) && exists(s6))} \n' +
' [1] ixseek s5 s6 s9 s4 s7 s8 [] @"fa48c668-df99-4211-b9a8-f33781955f06" @"number_of_reviews_1" true \n' +
' right \n' +
' [2] limit 1 \n' +
' [2] seek s4 s11 s12 s7 s8 s9 s10 [] @"fa48c668-df99-4211-b9a8-f33781955f06" true false \n'
}
},
rejectedPlans: []
},
executionStats: {
executionSuccess: true,
nReturned: 2765,
executionTimeMillis: 10,
totalKeysExamined: 2765,
totalDocsExamined: 2765,
executionStages: {
stage: 'nlj',
planNodeId: 2,
nReturned: 2765,
executionTimeMillisEstimate: 10,
opens: 1,
closes: 1,
saveState: 2,
restoreState: 2,
isEOF: 1,
totalDocsExamined: 2765,
totalKeysExamined: 2765,
collectionScans: 0,
collectionSeeks: 2765,
indexScans: 0,
indexSeeks: 1,
indexesUsed: [ 'number_of_reviews_1' ],
innerOpens: 2765,
innerCloses: 1,
outerProjects: [],
outerCorrelated: [ Long("4"), Long("7"), Long("8"), Long("9"), Long("10") ],
outerStage: {
stage: 'cfilter',
planNodeId: 1,
nReturned: 2765,
executionTimeMillisEstimate: 10,
opens: 1,
closes: 1,
saveState: 2,
restoreState: 2,
isEOF: 1,
numTested: 1,
filter: '(exists(s5) && exists(s6)) ',
inputStage: {
stage: 'ixseek',
planNodeId: 1,
nReturned: 2765,
executionTimeMillisEstimate: 10,
opens: 1,
closes: 1,
saveState: 2,
restoreState: 2,
isEOF: 1,
indexName: 'number_of_reviews_1',
keysExamined: 2765,
seeks: 1,
numReads: 2766,
indexKeySlot: 9,
recordIdSlot: 4,
snapshotIdSlot: 7,
indexIdentSlot: 8,
outputSlots: [],
indexKeysToInclude: '00000000000000000000000000000000',
seekKeyLow: 's5 ',
seekKeyHigh: 's6 '
}
},
innerStage: {
stage: 'limit',
planNodeId: 2,
nReturned: 2765,
executionTimeMillisEstimate: 0,
opens: 2765,
closes: 1,
saveState: 2,
restoreState: 2,
isEOF: 1,
limit: 1,
inputStage: {
stage: 'seek',
planNodeId: 2,
nReturned: 2765,
executionTimeMillisEstimate: 0,
opens: 2765,
closes: 1,
saveState: 2,
restoreState: 2,
isEOF: 0,
numReads: 2765,
recordSlot: 11,
recordIdSlot: 12,
seekKeySlot: 4,
snapshotIdSlot: 7,
indexIdentSlot: 8,
indexKeySlot: 9,
indexKeyPatternSlot: 10,
fields: [],
outputSlots: []
}
}
}
},
command: {
find: 'names',
filter: { number_of_reviews: { '$gt': 5 } },
'$db': 'airbnb'
},
serverInfo: {
host: 'Anonymous',
port: 27017,
version: '7.0.0',
gitVersion: '37d84072b5c5b9fd723db5fa133fb202ad2317f1'
},
serverParameters: {
internalQueryFacetBufferSizeBytes: 104857600,
internalQueryFacetMaxOutputDocSizeBytes: 104857600,
internalLookupStageIntermediateDocumentMaxSizeBytes: 104857600,
internalDocumentSourceGroupMaxMemoryBytes: 104857600,
internalQueryMaxBlockingSortMemoryUsageBytes: 104857600,
internalQueryProhibitBlockingMergeOnMongoS: 0,
internalQueryMaxAddToSetBytes: 104857600,
internalDocumentSourceSetWindowFieldsMaxMemoryBytes: 104857600,
internalQueryFrameworkControl: 'trySbeEngine'
},
ok: 1
}
// //searching with and without the use of index
airbnb> db.names.getIndexes()
[
{ v: 2, key: { _id: 1 }, name: '_id_' },
{ v: 2, key: { number_of_reviews: 1 }, name: 'number_of_reviews_1' }
]
airbnb> db.names.distinct("country_name")
[]
airbnb> db.names.distinct("address.country")
[
'Australia',
'Brazil',
'Canada',
'China',
'Hong Kong',
'Portugal',
'Spain',
'Turkey',
'United States'
]
airbnb> db.names.find({address.country :"Spain"}).count()
Uncaught:
SyntaxError: Unexpected token, expected "," (1:22)
> 1 | db.names.find({address.country :"Spain"}).count()
| ^
2 |
airbnb> db.names.find({"address.country" :"Spain"}).count()
633
airbnb> db.names.find({"address.country" :"Spain"}).limit(1)
[
{
_id: '10082422',
listing_url: 'https://www.airbnb.com/rooms/10082422',
name: 'Nice room in Barcelona Center',
summary: 'Hi! Cozy double bed room in amazing flat next to Passeig de Sant Joan and to metro stop Verdaguer. 3 streets to Sagrada Familia and 4 streets to Passeig de Gracia. Flat located in the center of the city. View to Sagrada Familia and Torre Agbar.',
space: 'Nice flat in the central neighboorhood of Eixample.',
description: "Hi! Cozy double bed room in amazing flat next to Passeig de Sant Joan and to metro stop Verdaguer. 3 streets to Sagrada Familia and 4 streets to Passeig de Gracia. Flat located in the center of the city. View to Sagrada Familia and Torre Agbar. Nice flat in the central neighboorhood of Eixample. Ideal couple or 2 friends. Dreta de l'Eixample",
neighborhood_overview: "Dreta de l'Eixample",
notes: '',
transit: '',
access: 'Ideal couple or 2 friends.',
interaction: '',
house_rules: '',
property_type: 'Apartment',
room_type: 'Private room',
bed_type: 'Real Bed',
minimum_nights: '1',
maximum_nights: '9',
cancellation_policy: 'flexible',
last_scraped: ISODate("2019-03-08T05:00:00.000Z"),
calendar_last_scraped: ISODate("2019-03-08T05:00:00.000Z"),
accommodates: 2,
bedrooms: 1,
beds: 2,
number_of_reviews: 0,
bathrooms: Decimal128("1.0"),
amenities: [
'Internet',
'Wifi',
'Kitchen',
'Elevator',
'Heating',
'Washer',
'Shampoo',
'Hair dryer',
'Iron',
'Laptop friendly workspace'
],
price: Decimal128("50.00"),
security_deposit: Decimal128("100.00"),
cleaning_fee: Decimal128("10.00"),
extra_people: Decimal128("0.00"),
guests_included: Decimal128("1"),
images: {
thumbnail_url: '',
medium_url: '',
picture_url: 'https://a0.muscache.com/im/pictures/aed1923a-69a6-4614-99d0-fd5c8f41ebda.jpg?aki_policy=large',
xl_picture_url: ''
},
host: {
host_id: '30393403',
host_url: 'https://www.airbnb.com/users/show/30393403',
host_name: 'Anna',
host_location: 'Barcelona, Catalonia, Spain',
host_about: "I'm Anna, italian. I'm 28, friendly and easygoing. I love travelling, reading, dancing tango. Can wait to meet new people! :)",
host_thumbnail_url: 'https://a0.muscache.com/im/users/30393403/profile_pic/1427876639/original.jpg?aki_policy=profile_small',
host_picture_url: 'https://a0.muscache.com/im/users/30393403/profile_pic/1427876639/original.jpg?aki_policy=profile_x_medium',
host_neighbourhood: "Dreta de l'Eixample",
host_is_superhost: false,
host_has_profile_pic: true,
host_identity_verified: false,
host_listings_count: 1,
host_total_listings_count: 1,
host_verifications: [ 'phone', 'facebook' ]
},
address: {
street: 'Barcelona, Catalunya, Spain',
suburb: 'Eixample',
government_area: "la Dreta de l'Eixample",
market: 'Barcelona',
country: 'Spain',
country_code: 'ES',
location: {
type: 'Point',
coordinates: [ 2.16942, 41.40082 ],
is_location_exact: true
}
},
availability: {
availability_30: 0,
availability_60: 0,
availability_90: 0,
availability_365: 0
},
review_scores: {},
reviews: []
}
]
airbnb> db.names.find({"address.country" :"Spain"}).explain("executionStats")
{
explainVersion: '2',
queryPlanner: {
namespace: 'airbnb.names',
indexFilterSet: false,
parsedQuery: { 'address.country': { '$eq': 'Spain' } },
queryHash: '7F4E5F2D',
planCacheKey: 'A47DAE5A',
maxIndexedOrSolutionsReached: false,
maxIndexedAndSolutionsReached: false,
maxScansToExplodeReached: false,
winningPlan: {
queryPlan: {
stage: 'COLLSCAN',
planNodeId: 1,
filter: { 'address.country': { '$eq': 'Spain' } },
direction: 'forward'
},
slotBasedPlan: {
slots: '$$RESULT=s5 env: { s1 = TimeZoneDatabase(America/Argentina/Tucuman...Asia/Dubai) (timeZoneDB), s7 = "Spain", s3 = 1720335368663 (NOW), s2 = Nothing (SEARCH_META) }',
stages: '[1] filter {traverseF(s4, lambda(l1.0) { traverseF(getField(l1.0, "country"), lambda(l2.0) { ((l2.0 == s7) ?: false) }, false) }, false)} \n' +
'[1] scan s5 s6 none none none none lowPriority [s4 = address] @"fa48c668-df99-4211-b9a8-f33781955f06" true false '
}
},
rejectedPlans: []
},
executionStats: {
executionSuccess: true,
nReturned: 633,
executionTimeMillis: 7,
totalKeysExamined: 0,
totalDocsExamined: 5555,
executionStages: {
stage: 'filter',
planNodeId: 1,
nReturned: 633,
executionTimeMillisEstimate: 7,
opens: 1,
closes: 1,
saveState: 5,
restoreState: 5,
isEOF: 1,
numTested: 5555,
filter: 'traverseF(s4, lambda(l1.0) { traverseF(getField(l1.0, "country"), lambda(l2.0) { ((l2.0 == s7) ?: false) }, false) }, false) ',
inputStage: {
stage: 'scan',
planNodeId: 1,
nReturned: 5555,
executionTimeMillisEstimate: 7,
opens: 1,
closes: 1,
saveState: 5,
restoreState: 5,
isEOF: 1,
numReads: 5555,
recordSlot: 5,
recordIdSlot: 6,
fields: [ 'address' ],
outputSlots: [ Long("4") ]
}
}
},
command: {
find: 'names',
filter: { 'address.country': 'Spain' },
'$db': 'airbnb'
},
serverInfo: {
host: 'Anonymous',
port: 27017,
version: '7.0.0',
gitVersion: '37d84072b5c5b9fd723db5fa133fb202ad2317'
},
serverParameters: {
internalQueryFacetBufferSizeBytes: 104857600,
internalQueryFacetMaxOutputDocSizeBytes: 104857600,
internalLookupStageIntermediateDocumentMaxSizeBytes: 104857600,
internalDocumentSourceGroupMaxMemoryBytes: 104857600,
internalQueryMaxBlockingSortMemoryUsageBytes: 104857600,
internalQueryProhibitBlockingMergeOnMongoS: 0,
internalQueryMaxAddToSetBytes: 104857600,
internalDocumentSourceSetWindowFieldsMaxMemoryBytes: 104857600,
internalQueryFrameworkControl: 'trySbeEngine'
},
ok: 1
}
/* +++++++++++++++++++++++ index creation ++++++++++++++++++*/
airbnb> db.names.createIndex({"address.country" :'text'})
address.country_text
airbnb> db.names.getIndexes()
[
{ v: 2, key: { _id: 1 }, name: '_id_' },
{ v: 2, key: { number_of_reviews: 1 }, name: 'number_of_reviews_1' },
{
v: 2,
key: { _fts: 'text', _ftsx: 1 },
name: 'address.country_text',
weights: { 'address.country': 1 },
default_language: 'english',
language_override: 'language',
textIndexVersion: 3
}
]
// // ++++++ +++++ searching now
airbnb> db.names.find({$text :{$search:'Spain'}}).explain("executionStats")
{
explainVersion: '2',
queryPlanner: {
namespace: 'airbnb.names',
indexFilterSet: false,
parsedQuery: {
'$text': {
'$search': 'Spain',
'$language': 'english',
'$caseSensitive': false,
'$diacriticSensitive': false
}
},
queryHash: '77237B6B',
planCacheKey: '65F93C53',
maxIndexedOrSolutionsReached: false,
maxIndexedAndSolutionsReached: false,
maxScansToExplodeReached: false,
winningPlan: {
queryPlan: {
stage: 'TEXT_MATCH',
planNodeId: 3,
indexPrefix: {},
indexName: 'address.country_text',
parsedTextQuery: {
terms: [ 'spain' ],
negatedTerms: [],
phrases: [],
negatedPhrases: []
},
textIndexVersion: 2,
inputStage: {
stage: 'FETCH',
planNodeId: 2,
inputStage: {
stage: 'IXSCAN',
planNodeId: 1,
keyPattern: { _fts: 'text', _ftsx: 1 },
indexName: 'address.country_text',
isMultiKey: true,
isUnique: false,
isSparse: false,
isPartial: false,
indexVersion: 2,
direction: 'backward',
indexBounds: {}
}
}
},
slotBasedPlan: {
slots: '$$RESULT=s9 env: { s2 = Nothing (SEARCH_META), s3 = 1720336435988 (NOW), s1 = TimeZoneDatabase(America/Argentina/Tucuman...Asia/Dubai) (timeZoneDB), s8 = {"_fts" : "text", "_ftsx" : 1} }',
stages: '[3] filter {\n' +
' if isObject(s9) \n' +
' then ftsMatch(FtsMatcher({"terms" : ["spain"], "negatedTerms" : [], "phrases" : [], "negatedPhrases" : []}), s9) \n' +
' else fail(4623400, "textmatch requires input to be an object") \n' +
'} \n' +
'[2] nlj inner [] [s4, s5, s6, s7, s8] \n' +
' left \n' +
' [1] unique [s4] \n' +
' [1] ixseek KS(3C737061696E002E77359400FE04) KS(3C737061696E00290104) s7 s4 s5 s6 [] @"fa48c668-df99-4211-b9a8-f33781955f06" @"address.country_text" false \n' +
' right \n' +
' [2] limit 1 \n' +
' [2] seek s4 s9 s10 s5 s6 s7 s8 [] @"fa48c668-df99-4211-b9a8-f33781955f06" true false \n'
}
},
rejectedPlans: []
},
executionStats: {
executionSuccess: true,
nReturned: 633,
executionTimeMillis: 10,
totalKeysExamined: 633,
totalDocsExamined: 633,
executionStages: {
stage: 'filter',
planNodeId: 3,
nReturned: 633,
executionTimeMillisEstimate: 8,
opens: 1,
closes: 1,
saveState: 0,
restoreState: 0,
isEOF: 1,
numTested: 633,
filter: '\n' +
' if isObject(s9) \n' +
' then ftsMatch(FtsMatcher({"terms" : ["spain"], "negatedTerms" : [], "phrases" : [], "negatedPhrases" : []}), s9) \n' +
' else fail(4623400, "textmatch requires input to be an object") \n',
inputStage: {
stage: 'nlj',
planNodeId: 2,
nReturned: 633,
executionTimeMillisEstimate: 8,
opens: 1,
closes: 1,
saveState: 0,
restoreState: 0,
isEOF: 1,
totalDocsExamined: 633,
totalKeysExamined: 633,
collectionScans: 0,
collectionSeeks: 633,
indexScans: 0,
indexSeeks: 1,
indexesUsed: [ 'address.country_text' ],
innerOpens: 633,
innerCloses: 1,
outerProjects: [],
outerCorrelated: [ Long("4"), Long("5"), Long("6"), Long("7"), Long("8") ],
outerStage: {
stage: 'unique',
planNodeId: 1,
nReturned: 633,
executionTimeMillisEstimate: 8,
opens: 1,
closes: 1,
saveState: 0,
restoreState: 0,
isEOF: 1,
dupsTested: 633,
dupsDropped: 0,
keySlots: [ Long("4") ],
inputStage: {
stage: 'ixseek',
planNodeId: 1,
nReturned: 633,
executionTimeMillisEstimate: 8,
opens: 1,
closes: 1,
saveState: 0,
restoreState: 0,
isEOF: 1,
indexName: 'address.country_text',
keysExamined: 633,
seeks: 1,
numReads: 634,
indexKeySlot: 7,
recordIdSlot: 4,
snapshotIdSlot: 5,
indexIdentSlot: 6,
outputSlots: [],
indexKeysToInclude: '00000000000000000000000000000000',
seekKeyLow: 'KS(3C737061696E002E77359400FE04) ',
seekKeyHigh: 'KS(3C737061696E00290104) '
}
},
innerStage: {
stage: 'limit',
planNodeId: 2,
nReturned: 633,
executionTimeMillisEstimate: 0,
opens: 633,
closes: 1,
saveState: 0,
restoreState: 0,
isEOF: 1,
limit: 1,
inputStage: {
stage: 'seek',
planNodeId: 2,
nReturned: 633,
executionTimeMillisEstimate: 0,
opens: 633,
closes: 1,
saveState: 0,
restoreState: 0,
isEOF: 0,
numReads: 633,
recordSlot: 9,
recordIdSlot: 10,
seekKeySlot: 4,
snapshotIdSlot: 5,
indexIdentSlot: 6,
indexKeySlot: 7,
indexKeyPatternSlot: 8,
fields: [],
outputSlots: []
}
}
}
}
},
command: {
find: 'names',
filter: { '$text': { '$search': 'Spain' } },
'$db': 'airbnb'
},
serverInfo: {
host: 'Anonymous',
port: 27017,
version: '7.0.0',
gitVersion: '37d84072b5c5b9fd723db5fa133fb202ad2317f1'
},
serverParameters: {
internalQueryFacetBufferSizeBytes: 104857600,
internalQueryFacetMaxOutputDocSizeBytes: 104857600,
internalLookupStageIntermediateDocumentMaxSizeBytes: 104857600,
internalDocumentSourceGroupMaxMemoryBytes: 104857600,
internalQueryMaxBlockingSortMemoryUsageBytes: 104857600,
internalQueryProhibitBlockingMergeOnMongoS: 0,
internalQueryMaxAddToSetBytes: 104857600,
internalDocumentSourceSetWindowFieldsMaxMemoryBytes: 104857600,
internalQueryFrameworkControl: 'trySbeEngine'
},
ok: 1
}
// +++++++++++++++++++++ // for updating a value to an array
airbnb> db.names.updateOne({_id: '10009999'},{$set :{bedrooms: [1,5,6,7,8]}})
{
acknowledged: true,
insertedId: null,
matchedCount: 1,
modifiedCount: 1,
upsertedCount: 0
}
//--------------------------checking the update
airbnb> db.names.find({_id: '10009999'})
[
{
_id: '10009999',
listing_url: 'https://www.airbnb.com/rooms/10009999',
name: 'Horto flat with small garden',
summary: 'One bedroom + sofa-bed in quiet and bucolic neighbourhood right next to the Botanical Garden. Small garden, outside shower, well equipped kitchen and bathroom with shower and tub. Easy for transport with many restaurants and basic facilities in the area.',
space: 'Lovely one bedroom + sofa-bed in the living room, perfect for two but fits up to four comfortably. There´s a small outside garden with a shower There´s a well equipped open kitchen with both 110V / 220V wall plugs and one bathroom with shower, tub and even a sauna machine! All newly refurbished!',
description: 'One bedroom + sofa-bed in quiet and bucolic neighbourhood right next to the Botanical Garden. Small garden, outside shower, well equipped kitchen and bathroom with shower and tub. Easy for transport with many restaurants and basic facilities in the area. Lovely one bedroom + sofa-bed in the living room, perfect for two but fits up to four comfortably. There´s a small outside garden with a shower There´s a well equipped open kitchen with both 110V / 220V wall plugs and one bathroom with shower, tub and even a sauna machine! All newly refurbished! I´ll be happy to help you with any doubts, tips or any other information needed during your stay. This charming ground floor flat is located in Horto, a quiet and bucolic neighborhood just next to the Botanical Garden, where most of the descendants of it´s first gardeners still live. You´ll be 30 minutes walk from waterfalls in the rainforest with easy hiking trails! There are nice bars and restaurants as well as basic facilities - pharmacy, b',
neighborhood_overview: 'This charming ground floor flat is located in Horto, a quiet and bucolic neighborhood just next to the Botanical Garden, where most of the descendants of it´s first gardeners still live. You´ll be 30 minutes walk from waterfalls in the rainforest with easy hiking trails! There are nice bars and restaurants as well as basic facilities - pharmacy, bakery, small market - in the area.',
notes: 'There´s a table in the living room now, that does not show in the photos.',
transit: 'Easy access to transport (bus, taxi, car) and easy free parking around. Very close to Gávea, Leblon, Ipanema, Copacabana and Botafogo.',
access: '',
interaction: 'I´ll be happy to help you with any doubts, tips or any other information needed during your stay.',
house_rules: 'I just hope the guests treat the space as they´re own, with respect to it as well as to my neighbours! Espero apenas que os hóspedes tratem o lugar com carinho e respeito aos vizinhos!',
property_type: 'Apartment',
room_type: 'Entire home/apt',
bed_type: 'Real Bed',
minimum_nights: '2',
maximum_nights: '1125',
cancellation_policy: 'flexible',
last_scraped: ISODate("2019-02-11T05:00:00.000Z"),
calendar_last_scraped: ISODate("2019-02-11T05:00:00.000Z"),
accommodates: 4,
bedrooms: [ 1, 5, 6, 7, 8 ],
beds: 2,
number_of_reviews: 0,
bathrooms: Decimal128("1.0"),
amenities: [
'Wifi',
'Wheelchair accessible',
'Kitchen',
'Free parking on premises',
'Smoking allowed',
'Hot tub',
'Buzzer/wireless intercom',
'Family/kid friendly',
'Washer',
'First aid kit',
'Essentials',
'Hangers',
'Hair dryer',
'Iron',
'Laptop friendly workspace'
],
price: Decimal128("317.00"),
weekly_price: Decimal128("1492.00"),
monthly_price: Decimal128("4849.00"),
cleaning_fee: Decimal128("187.00"),
extra_people: Decimal128("0.00"),
guests_included: Decimal128("1"),
images: {
thumbnail_url: '',
medium_url: '',
picture_url: 'https://a0.muscache.com/im/pictures/5b408b9e-45da-4808-be65-4edc1f29c453.jpg?aki_policy=large',
xl_picture_url: ''
},
host: {
host_id: '1282196',
host_url: 'https://www.airbnb.com/users/show/1282196',
host_name: 'Ynaie',
host_location: 'Rio de Janeiro, State of Rio de Janeiro, Brazil',
host_about: "I am an artist and traveling is a major part of my life. I love treating visitors the way I like to be treated when I´m abroad and I'm usually renting my flat while I'm away. I can recommend some cool parties and nights out as well as advise on some hidden secrets of Rio’s nature!",
host_thumbnail_url: 'https://a0.muscache.com/im/pictures/9681e3cc-4af1-4046-b294-2881dffb4ff8.jpg?aki_policy=profile_small',
host_picture_url: 'https://a0.muscache.com/im/pictures/9681e3cc-4af1-4046-b294-2881dffb4ff8.jpg?aki_policy=profile_x_medium',
host_neighbourhood: 'Jardim Botânico',
host_is_superhost: false,
host_has_profile_pic: true,
host_identity_verified: false,
host_listings_count: 1,
host_total_listings_count: 1,
host_verifications: [ 'email', 'phone', 'facebook' ]
},
address: {
street: 'Rio de Janeiro, Rio de Janeiro, Brazil',
suburb: 'Jardim Botânico',
government_area: 'Jardim Botânico',
market: 'Rio De Janeiro',
country: 'Brazil',
country_code: 'BR',
location: {
type: 'Point',
coordinates: [ -43.23074991429229, -22.966253551739655 ],
is_location_exact: true
}
},
availability: {
availability_30: 0,
availability_60: 0,
availability_90: 0,
availability_365: 0
},
review_scores: {},
reviews: []
}
]
Subscribe to my newsletter
Read articles from himanshu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
