Design Pattern - 3
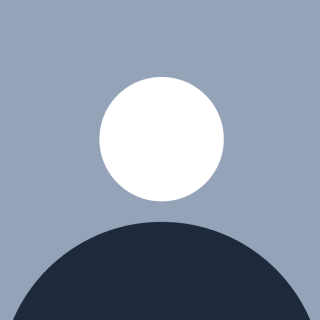
Decorator design pattern saves us from object explosion.
Composition is a design technique in OOP which defines has-a relationship between objects.
public class Job{
// methods and properties...
}
Person HAS-A Job
public class Person{
private Job job;
// other properties and methods ...
}
Composition is acheived by referencing an object into another as instance variable.
With composition we can add new functionality by writing new code rather than altering existing code.
The decorator pattern attaches new functionality to an object dynamically.
Create an abstract class Beverage. Since coffee is a beverage starting with Beverage would be most appropriate.
public abstract class Beverage {
public String description = "Unknown Beverage";
public String getDescription(){
return this.description;
}
public abstract double cost();
}
Next create a decorator class for condiments. This is an abtract class.
public abstract class CondimentsDecorator extends Beverage {
Beverage beverage;
public abstract String getDescription();
}
Since we need to be interchangeable with Beverage class we are extending it.
We are going to require to reimplement getDescription method.
Now , base classes are out if the way lets implement Beverages.
public class HouseBlend extends Beverage{
public HouseBlend(){
description = "House Blend Coffee";
}
@Override
public double cost() {
return 0.89;
}
}
public class Espresso extends Beverage {
public Espresso() {
description = "Espresso";
}
public double cost() {
return 1.99;
}
}
Let's implement concrete decorators.
public class ChocolateChips extends CondimentsDecorator{
private final Beverage beverage;
public ChocolateChipsDecorator(Beverage beverage){
this.beverage = beverage;
}
@Override
public String getDescription() {
return beverage.getDescription() + ", Chocolate Chips";
}
@Override
public double cost() {
return beverage.cost() + 0.20;
}
}
ChocolateChips extends CondimentsDecorator which extends Beverage.
Let's Serve the coffee.
public class Main {
public static void main(String[] args) {
Beverage beverage = new Espresso();
System.out.println("The cost of Espresso is: $" + beverage.cost());
// The cost of Espresso is: $1.99
// let's add some extra things
Beverage chocolateChipsBeverage = new ChocolateChips(beverage);
System.out.println("COst of espresso with Chocolate chips is $ "+ chocolateChipsBeverage.cost());
// COst of espresso with Chocolate chips is $ 2.19
}
}
Credits - Head First Design Patterns
Subscribe to my newsletter
Read articles from Neel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by