Handling Sessions & Cookies in PHP - Authentication
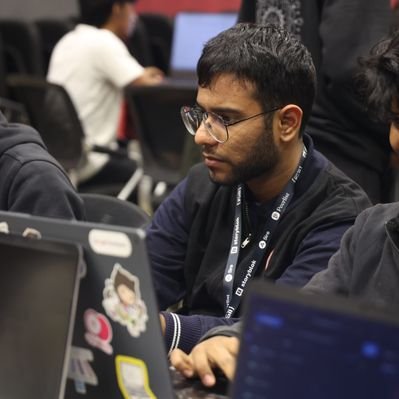
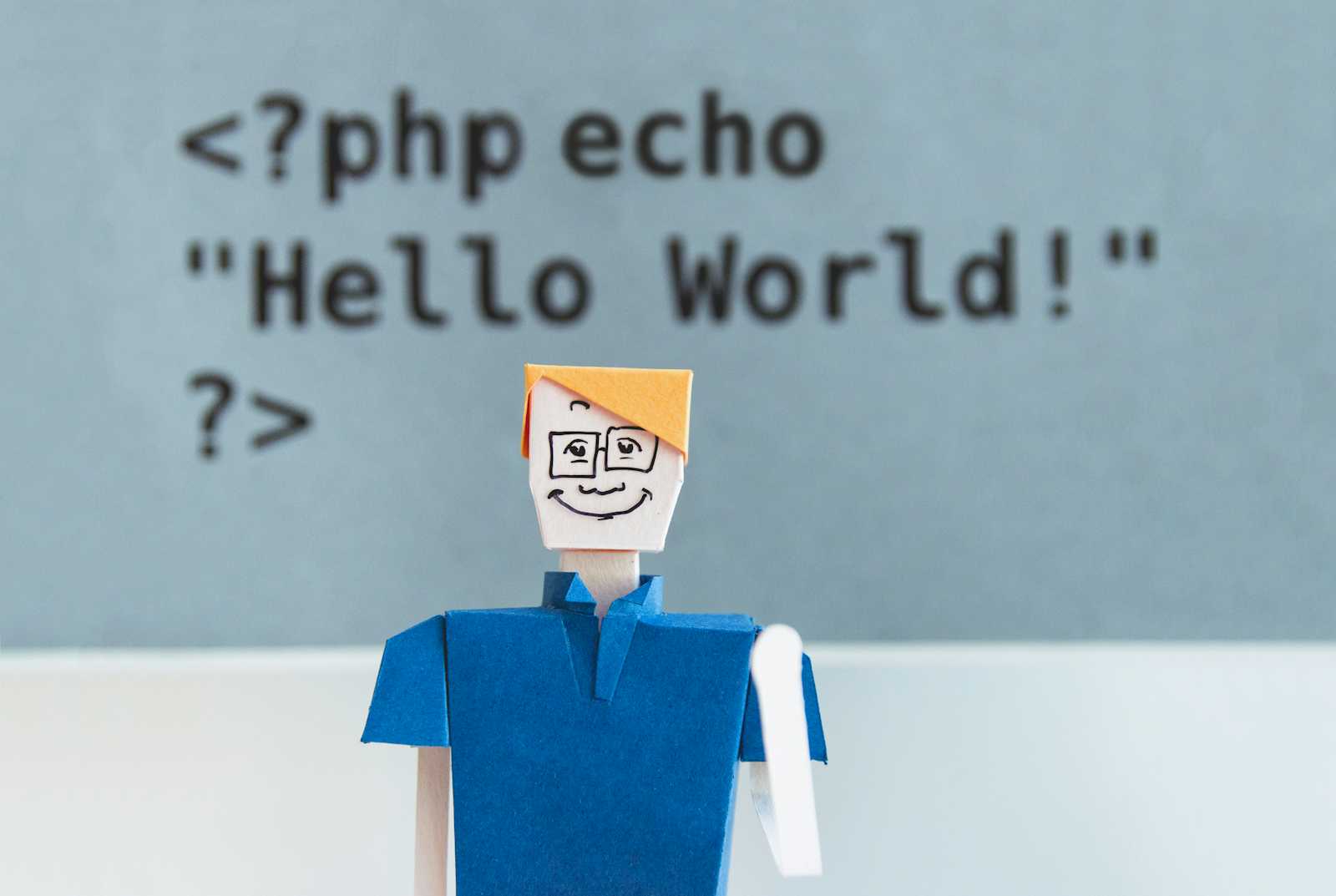
Introduction
Handling sessions and cookies is a fundamental aspect of web development, especially when it comes to user authentication. PHP provides robust mechanisms for managing sessions and cookies, making it possible to maintain user state and secure authentication processes. In this blog, we will explore the concepts of sessions and cookies, how to handle them in PHP, and implement a complete user authentication system.
Understanding Sessions and Cookies
What are Sessions?
Sessions are server-side storage mechanisms that allow you to store user information across multiple pages. A session is created for each user who visits your site, and a unique session ID is assigned to each session. This session ID is used to retrieve stored data for that particular user.
What are Cookies?
Cookies are small pieces of data stored on the client-side (user's browser) that can be used to store information about the user's activity. Cookies are often used to remember user preferences, login information, and other settings.
Differences between Sessions and Cookies
Scope: Sessions are stored on the server, while cookies are stored on the client-side.
Security: Sessions are generally more secure as they are stored on the server. Cookies can be easily manipulated by users.
Lifespan: Sessions last until the browser is closed or the session is destroyed, whereas cookies can have a set expiration time.
Setting Up the Environment
Installing PHP and Setting Up a Server
To start working with PHP, you need a server environment. You can use XAMPP, WAMP, or MAMP to set up a local server. These packages include Apache (a web server), MySQL (a database server), and PHP.
Download XAMPP: Go to the XAMPP website and download the appropriate version for your operating system.
Install XAMPP: Follow the installation instructions and start the Apache and MySQL servers.
Creating a Database
To manage user authentication, we need a database to store user information. We will use MySQL for this purpose.
Access phpMyAdmin: Open your browser and go to
http://localhost/phpmyadmin
.Create a Database: Click on "New" and create a database named
auth_db
.Create a Users Table: Run the following SQL query to create a
users
table:
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(50) NOT NULL UNIQUE,
password VARCHAR(255) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
Working with Sessions in PHP
Starting a Session
To start a session in PHP, you use the session_start()
function. This function must be called at the beginning of your script, before any output is sent to the browser.
<?php
session_start();
?>
Storing Data in Sessions
You can store data in the $_SESSION
superglobal array. For example, to store a user's username, you can do the following:
<?php
session_start();
$_SESSION['username'] = 'john_doe';
?>
Retrieving Data from Sessions
To retrieve data from a session, simply access the $_SESSION
array:
<?php
session_start();
if (isset($_SESSION['username'])) {
echo 'Username: ' . $_SESSION['username'];
} else {
echo 'No username set.';
}
?>
Destroying a Session
To destroy a session, use the session_destroy()
function. This will remove all session data:
<?php
session_start();
session_unset(); // Unset all session variables
session_destroy(); // Destroy the session
?>
Working with Cookies in PHP
Setting Cookies
To set a cookie, use the setcookie()
function. This function must be called before any output is sent to the browser:
<?php
setcookie('username', 'john_doe', time() + (86400 * 30), "/"); // 86400 = 1 day
?>
Retrieving Cookies
To retrieve a cookie, access the $_COOKIE
superglobal array:
<?php
if (isset($_COOKIE['username'])) {
echo 'Username: ' . $_COOKIE['username'];
} else {
echo 'No cookie set.';
}
?>
Deleting Cookies
To delete a cookie, set its expiration time to a past time:
<?php
setcookie('username', '', time() - 3600, "/");
?>
Implementing User Authentication
Creating the User Registration Form
Create a file named register.html
with the following content:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Register</title>
</head>
<body>
<h2>Register</h2>
<form action="register.php" method="post">
<label for="username">Username:</label>
<input type="text" name="username" required><br><br>
<label for="password">Password:</label>
<input type="password" name="password" required><br><br>
<input type="submit" name="register" value="Register">
</form>
</body>
</html>
Storing User Data Securely
In the register.php
file, add the following PHP code to handle the registration process:
<?php
if (isset($_POST['register'])) {
$username = $_POST['username'];
$password = password_hash($_POST['password'], PASSWORD_BCRYPT);
$conn = new mysqli('localhost', 'root', '', 'auth_db');
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$stmt = $conn->prepare("INSERT INTO users (username, password) VALUES (?, ?)");
$stmt->bind_param("ss", $username, $password);
if ($stmt->execute()) {
echo "Registration successful!";
} else {
echo "Error: " . $stmt->error;
}
$stmt->close();
$conn->close();
}
?>
Creating the Login Form
Create a file named login.html
with the following content:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Login</title>
</head>
<body>
<h2>Login</h2>
<form action="login.php" method="post">
<label for="username">Username:</label>
<input type="text" name="username" required><br><br>
<label for="password">Password:</label>
<input type="password" name="password" required><br><br>
<input type="submit" name="login" value="Login">
</form>
</body>
</html>
Verifying User Credentials
In the login.php
file, add the following PHP code to handle the login process:
<?php
session_start();
if (isset($_POST['login'])) {
$username = $_POST['username'];
$password = $_POST['password'];
$conn = new mysqli('localhost', 'root', '', 'auth_db');
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$stmt = $conn->prepare("SELECT password FROM users WHERE username = ?");
$stmt->bind_param("s", $username);
$stmt->execute();
$stmt->bind_result($hashed_password);
$stmt->fetch();
if (password_verify($password, $hashed_password)) {
$_SESSION['username'] = $username;
echo "Login successful!";
header("Location: dashboard.php");
} else {
echo "Invalid username or password.";
}
$stmt->close();
$conn->close();
}
?>
Managing User Sessions
Create a file named dashboard.php
to serve as the user's dashboard:
<?php
session_start();
if (!isset($_SESSION['username'])) {
header("Location: login.php");
exit();
}
echo "Welcome, " . $_SESSION['username'];
?>
<a href="logout.php">Logout</a>
Logging Out Users
Create a file named logout.php
to handle the logout process:
<?php
session_start();
session_unset();
session_destroy();
header("Location: login.php");
exit();
?>
Enhancing Security
Using HTTPS
Always use HTTPS to encrypt the data transmitted between the client and the server. This prevents man-in-the-middle attacks and ensures that sensitive information, such as passwords, is transmitted securely.
Implementing Secure Cookie Handling
When setting cookies, use the httponly
and secure
flags to enhance security:
<?php
setcookie('username', 'john_doe', time() + (86400 * 30), "/", "", true, true);
?>
Preventing Session Hijacking
Regenerate session IDs periodically to prevent session hijacking:
<?php
session_start();
session_regenerate_id(true);
?>
Conclusion
In this comprehensive guide, we have covered the essential aspects of handling sessions and cookies in PHP, particularly focusing on user authentication. We discussed the differences between sessions and cookies, how to manage them in PHP, and implemented a complete user authentication system with secure handling of user credentials. Additionally, we created a mini-project that demonstrates these concepts in action. By following this guide, you should have a solid understanding of how to implement and manage user authentication in your PHP applications.
Subscribe to my newsletter
Read articles from Kartik Mehta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
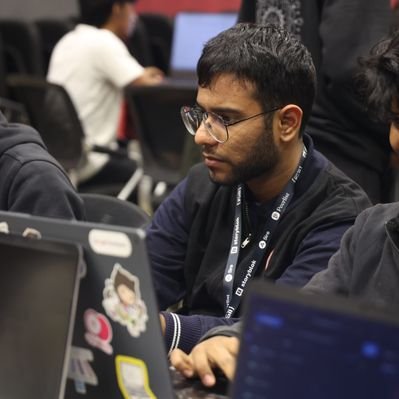
Kartik Mehta
Kartik Mehta
A code dependent life form.