A Nuts and Bolts approach to differentiate between Perl tk and Ruby tk

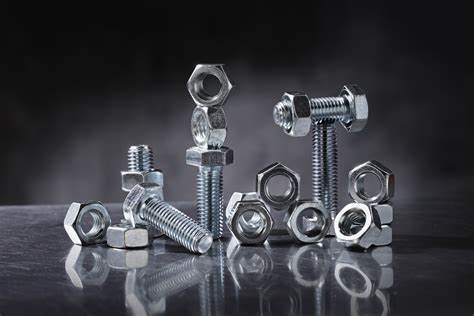
Introduction
When we talk about the "nuts and bolts" of something, we refer to the essential components and mechanisms that make it work. In the context of scripting languages and GUI toolkits, these "nuts and bolts" include the core syntax, paradigms, widget implementation, event handling, extensibility, and performance characteristics. This blog provides a detailed comparative analysis of Perl Tk and Ruby Tk by focusing on these fundamental aspects, helping you understand the differences and make an informed decision for your next project.
What Are the Nuts and Bolts?
Real-Life Example
Imagine building a piece of furniture, like a bookshelf. The "nuts and bolts" in this scenario are the screws, nuts, bolts, and brackets that hold the shelves together. Without these components, the structure would be unstable or fall apart. Similarly, in programming, the "nuts and bolts" are the fundamental elements that ensure the code is functional, efficient, and maintainable.
Importance in Scripting Languages
In scripting languages, the "nuts and bolts" refer to the core components that define how the language and its libraries operate. These include:
Syntax: The set of rules that define the combinations of symbols that are considered valid in the language.
Paradigms: The overall approach or methodology used for writing programs (e.g., procedural, object-oriented).
Widget Implementation: The way graphical components (like buttons, labels, and text fields) are created and managed.
Event Handling: The mechanisms for responding to user inputs and other events.
Extensibility: The ability to extend and customize the toolkit or language.
Performance: How efficiently the language and toolkit execute tasks.
In this comparative analysis, we'll delve into these nuts and bolts to differentiate between Perl Tk and Ruby Tk.
Perl tk and ruby tk installation
First we check the version of perl:
perl -v
If not available, we install perl and tk as well, the dependencies being first:
sudo apt-get update
sudo apt-get install build-essential libx11-dev libxft-dev libxext-dev libxi-dev libxrandr-dev libxinerama-dev libxcursor-dev libxss-dev libxt-dev
Then we install the cpanminus to install tk:
sudo apt-get install cpanminus
Then using it we install the tk:
sudo cpanm Tk
This should run perl and tk programs with ease.
Now we install ruby with tk:
sudo apt update
sudo apt install ruby-full
sudo apt install tk-dev
Then install the tk using:
sudo gem install tk -- --with-tcltkversion=8.6 \
--with-tcl-lib=/usr/lib/x86_64-linux-gnu \
--with-tk-lib=/usr/lib/x86_64-linux-gnu \
--with-tcl-include=/usr/include/tcl8.6 \
--with-tk-include=/usr/include/tcl8.6 \
--enable-pthread
Language Syntax and Paradigms
Perl Tk
Perl is known for its flexibility and "there's more than one way to do it" philosophy. This flexibility extends to Perl Tk, where the syntax can sometimes be verbose and less structured compared to Ruby.
Example of creating a simple button in Perl Tk:
use Tk;
my $mw = MainWindow->new;
my $button = $mw->Button(-text => "Click Me", -command => sub { print "Button Clicked\n" });
$button->pack;
MainLoop;
Syntax: Perl’s syntax can be less intuitive, especially for those not familiar with its conventions.
Paradigm: Perl Tk follows a procedural programming style, which can lead to less organized code structures.
Ruby Tk
Ruby, in contrast, emphasizes simplicity and productivity with an elegant syntax. Ruby Tk reflects this philosophy by offering a more readable and structured approach to GUI programming.
Example of creating a simple button in Ruby Tk:
require 'tk'
root = TkRoot.new { title "Hello, Tk!" }
TkButton.new(root) do
text "Click Me"
command { puts "Button Clicked" }
pack
end
Tk.mainloop
Syntax: Ruby’s syntax is cleaner and more readable, making it easier for developers to understand and maintain their code.
Paradigm: Ruby Tk leverages Ruby’s object-oriented nature, promoting a more organized and modular approach to GUI development.
Widget Implementation
Perl Tk
In Perl Tk, widgets are created and configured using method calls. The toolkit provides a comprehensive set of widgets, but the syntax for creating and configuring them can be cumbersome.
Example of creating a label and entry widget in Perl Tk:
use Tk;
my $mw = MainWindow->new;
my $label = $mw->Label(-text => "Enter your name:")->pack;
my $entry = $mw->Entry()->pack;
MainLoop;
Widgets: Perl Tk has a wide range of widgets, but the configuration options can be verbose.
Customization: Customizing widgets often involves chaining multiple method calls, which can be less intuitive.
Ruby Tk
Ruby Tk provides a more concise and elegant way to create and configure widgets. Widgets are typically instantiated as objects, and their properties are set using block syntax.
Example of creating a label and entry widget in Ruby Tk:
require 'tk'
root = TkRoot.new { title "Hello, Tk!" }
TkLabel.new(root) { text "Enter your name:"; pack }
TkEntry.new(root) { pack }
Tk.mainloop
Widgets: Ruby Tk also offers a rich set of widgets, but with a cleaner and more intuitive syntax for configuration.
Customization: The block syntax allows for easy and readable customization of widgets.
Below is the code for widget in tcl using tk for comparision:
package require Tk
label .l -text "Enter your name:"
entry .e
pack .l .e
Event Handling
Perl Tk
Event handling in Perl Tk is done through binding events to widget methods. The syntax can be less straightforward, requiring explicit binding of events to callback functions.
Example of handling a button click event in Perl Tk:
use Tk;
my $mw = MainWindow->new;
my $button = $mw->Button(-text => "Click Me")->pack;
$button->configure(-command => sub { print "Button Clicked\n" });
MainLoop;
Event Binding: Requires explicit configuration, which can be less intuitive for beginners.
Callbacks: Perl Tk uses anonymous subroutines for callbacks, which can lead to complex and hard-to-read code.
Ruby Tk
Ruby Tk simplifies event handling by using blocks for callback functions. This approach aligns with Ruby’s design philosophy, making event handling more intuitive and readable.
Example of handling a button click event in Ruby Tk:
require 'tk'
root = TkRoot.new { title "Hello, Tk!" }
button = TkButton.new(root) {
text "Click Me"
command { puts "Button Clicked" }
pack
}
Tk.mainloop
Event Binding: More straightforward with block syntax, making it easier to bind events to widgets.
Callbacks: Blocks provide a cleaner and more readable way to define callback functions.
Additionally provided code for tcl tk implementation of events:
package require Tk
button .b -text "Click Me" -command {puts "Button Clicked"}
pack .b
Extensibility and Customization
Perl Tk
Perl Tk allows for extensive customization and extension of widgets, but the process can be complex and requires a deep understanding of the Tk toolkit and Perl’s syntax.
Example of extending a Perl Tk widget:
use Tk;
package MyButton;
use base qw(Tk::Derived Tk::Button);
Construct Tk::Widget 'MyButton';
sub InitObject {
my ($self, $args) = @_;
$self->SUPER::InitObject($args);
$self->configure(-text => "My Custom Button");
}
package main;
my $mw = MainWindow->new;
my $button = $mw->MyButton(-command => sub { print "Button Clicked\n" })->pack;
MainLoop;
Customization: Customizing widgets often involves subclassing and method overriding, which can be verbose.
Extensibility: Extending widgets requires a good grasp of Perl’s object-oriented features and Tk’s architecture.
Ruby Tk
Ruby Tk also supports customization and extension of widgets, but the process is more intuitive and aligns well with Ruby’s object-oriented design.
Example of extending a Ruby Tk widget:
require 'tk'
root = TkRoot.new { title "Hello, Tk!" }
button = TkButton.new(root) {
text "Custom Button"
command { puts "Custom Button Clicked" }
pack
}
Tk.mainloop
Customization: Easier and more intuitive with Ruby’s clean syntax and object-oriented features.
Extensibility: Extending widgets is straightforward, thanks to Ruby’s flexible and expressive object model.
Additionally TCL tk implementation of extendability:
package require Tk
button .b -text "Custom Button" -command {puts "Custom Button Clicked"}
pack .b
Performance Considerations
Perl Tk
Perl Tk is known for its stability and performance, particularly in applications where Perl’s text processing capabilities are advantageous. However, the GUI performance might lag behind more modern toolkits.
Performance: Generally stable but may not be as responsive as newer GUI frameworks.
Resource Management: Requires careful management of resources to ensure smooth performance, especially in complex applications.
Ruby Tk
Ruby Tk offers competitive performance, leveraging Ruby’s efficient memory management and garbage collection. The performance is generally suitable for most desktop applications.
Performance: Good overall performance, with efficient memory usage and garbage collection.
Resource Management: Ruby’s garbage collection helps in managing resources effectively, ensuring better performance in complex applications.
Community and Support
Perl Tk
Perl Tk has been around for a long time and has a mature community. There are many resources available, but the community size has diminished over the years.
Community: Mature but shrinking, with a wealth of legacy resources.
Support: Good documentation and community support, though finding active contributors can be challenging.
Ruby Tk
Ruby Tk benefits from Ruby’s vibrant and active community. The resources and support available are continuously growing, making it easier for new developers to get help.
Community: Vibrant and active, with a growing number of resources and contributors.
Support: Excellent documentation and active community support, making it easier to find help and examples.
Summary
Aspect | Perl Tk | Ruby Tk |
Language Syntax | Verbose, less intuitive, procedural | Cleaner, more readable, object-oriented |
Widget Implementation | Comprehensive but verbose, method chaining | Rich set of widgets, concise and elegant block syntax |
Event Handling | Explicit binding, anonymous subroutines | Straightforward block syntax, cleaner callbacks |
Extensibility | Complex customization, requires deep understanding | Intuitive customization, aligns with object-oriented design |
Performance | Stable but less responsive | Good performance, efficient memory management |
Community and Support | Mature but shrinking, wealth of legacy resources | Vibrant and active, excellent documentation and support |
Conclusion
Choosing between Perl Tk and Ruby Tk depends on several factors, including the developer’s familiarity with the language, the complexity of the application, and the desired development speed.
Perl Tk: Best for developers with experience in Perl who need to leverage its powerful text processing capabilities. The procedural style might appeal to those who prefer Perl’s flexibility.
Ruby Tk: Ideal for developers who appreciate clean syntax and object-oriented programming. Ruby Tk’s intuitive and readable code structure makes it a strong choice for new and experienced developers alike.
Both Perl Tk and Ruby Tk have their strengths and can be powerful tools for creating GUIs. By understanding the nuts and bolts of each toolkit, developers can make an informed decision based on their specific needs and preferences.
Bonus Section: Comparing Perl Tk, Ruby Tk, and Tcl Tk
To provide a broader perspective, here's a comparison table that includes Tcl Tk, another popular Tk toolkit.
Feature | Perl Tk | Ruby Tk | Tcl Tk |
Syntax | Verbose, Procedural | Clean, Object-Oriented | Simple, Procedural |
Widget Implementation | Comprehensive, Verbose | Rich Set, Concise | Comprehensive, Easy to Use |
Event Handling | Explicit Binding, Anonymous Subs | Block Syntax, Clean Callbacks | Direct Binding, Inline Commands |
Extensibility | Complex, Deep Understanding Needed | Intuitive, OOP Aligned | Flexible, Easily Extendable |
Performance | Stable, Less Responsive | Efficient Memory, Good Performance | Highly Efficient, Fast Execution |
Community Support | Mature, Shrinking | Vibrant, Growing | Mature, Stable |
Tcl Tk
Syntax: Tcl Tk uses a simple, procedural syntax that is easy to learn and use.
Widget Implementation: Provides a comprehensive set of widgets that are straightforward to implement and configure.
Event Handling: Uses direct binding of events with inline commands, making it easy to handle user interactions.
Extensibility: Highly flexible and easily extendable, making it a robust choice for a wide range of applications.
Performance: Known for its high efficiency and fast execution, making it suitable for performance-critical applications.
Community Support: Mature and stable community with extensive resources and documentation.
Conclusion for Tcl Tk
Tcl Tk is a robust and efficient toolkit that is easy to learn and use, making it an excellent choice for developers who prefer a simple, procedural approach to GUI development. It is highly flexible and extendable, with a mature community that provides extensive support and resources.
By understanding the unique strengths and characteristics of Perl Tk, Ruby Tk, and Tcl Tk, developers can make more informed decisions based on their specific requirements and preferences. Each toolkit offers distinct advantages, making them suitable for different types of projects and developer expertise.
Subscribe to my newsletter
Read articles from Debanjan Chakraborty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
