Javascript objects

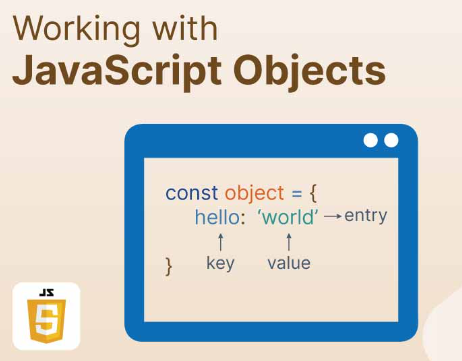
javascript is an object-oriented programming language.
Javascript object is a collection of properties each property is key -> value pair.
ways of creating an object
object literal notation
is a list of name : values inside curly braces
var obj = {
name: "sammy",
age: 14,
isMale: true
};
new Object()
var obj = new Object();
obj.name = "sammy"
obj.age = 15;
obj.isMale = true;
constructor function
function Person(name, age, isStudent) {
this.name = name;
this.age = age;
this.isStudent = isStudent;
};
var student = Person("sammy", 32, true);
object properties
values associated with an object. They can be accessed using the dot notation or bracket notation
console.log(Person.name) // dot notation
console.log(Person["age"]) // bracket notation
object methods
These are functions that are properties of an object. they allow an object to perform actions.
var person = {
name: "sammy"
age: 33
greet: function Developer() {
return "Hello my name is " + this.name;
}};
console.log(person.greet()); // calling an object method
Object Accessors
allows us to define custom behavior of getting and setting values of a property.
let car= {
model: "subaru",
color: "orange",
fuel_type: "diesel",
get fuel() {
return this.fuel_type
}
}
console.log(car.fuel)
setter methods
let car = {
model: "subaru",
color: "orange",
fuel_type: "",
set fuel(fuel) {
this.fuel_type = fuel
}
};
car.fuel = "petrol"
console.log(car.fuel_type)
Subscribe to my newsletter
Read articles from sammy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

sammy
sammy
I am an aspiring software engineer.By writing this article.I am commit myself accountable to provide valuable content to you reader oftenly every weekend about amazing programming concepts and technologies.To help you in your learning journey also.