Exploring Essential Python Functions

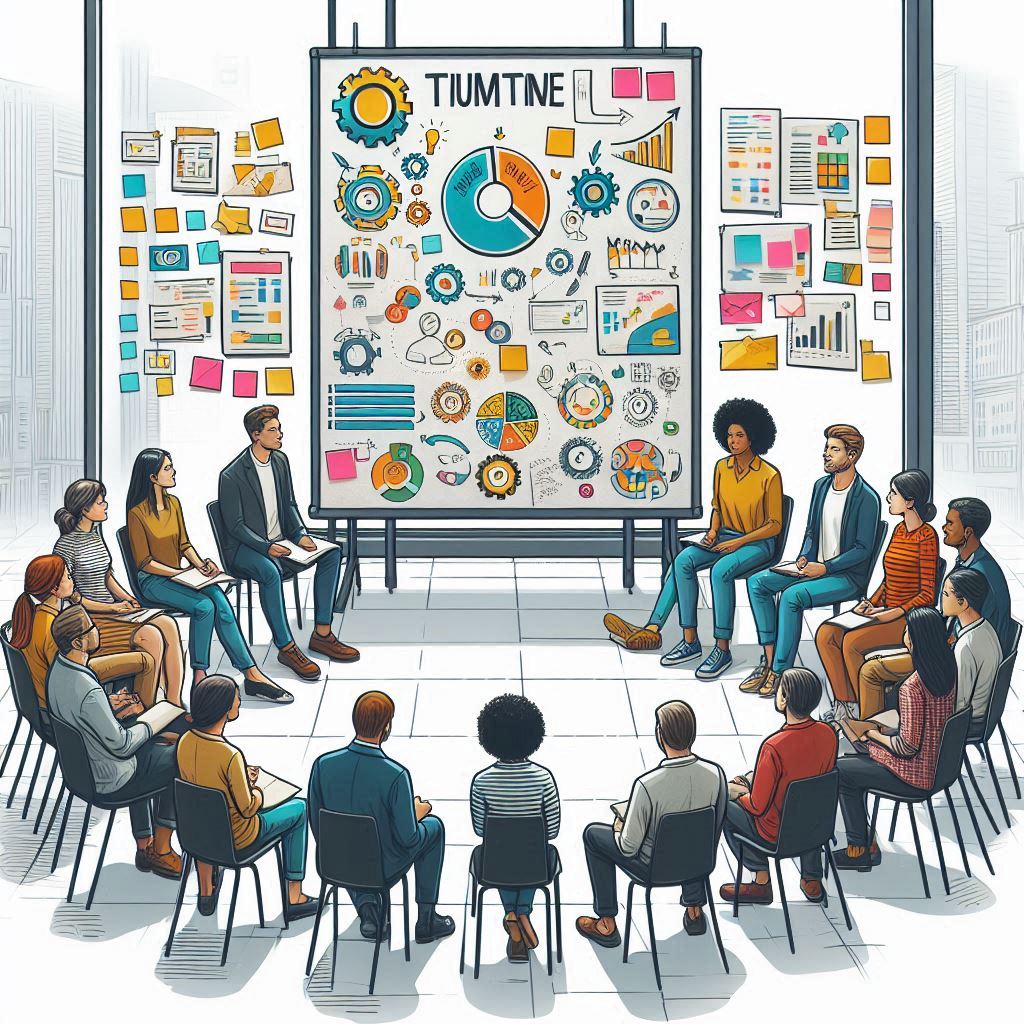
Welcome to the exciting world of Python! Whether you're a seasoned coder or just starting out, Python's built-in functions are here to make your life easier and your code more powerful. These versatile tools can help you perform a myriad of tasks—from manipulating data and handling user input to performing complex calculations and managing collections. Let's dive into the heart of Python and explore these essential functions that will elevate your coding game to the next level!
1. help()
The help()
function is used to display documentation for a module, function, class, method, or keyword.
Example:
help(dir)
This will show the documentation for the dir
function. You can also use help()
with a specific method:
help(str.find)
2. dir()
The dir()
function returns a list of the attributes and methods of any object (functions, modules, strings, lists, dictionaries, etc.).
Example:
print(dir(str))
This will list all the methods available for strings.
3. input()
The input()
function allows user input.
Example:
user_input = input("Enter something: ")
print("You entered:", user_input)
4. max()
The max()
function returns the largest of the input values.
Example:
print(max(10, 20)) # Output: 20
numbers = [1, 2, 3, 4, 5]
print(max(numbers)) # Output: 5
5. min()
The min()
function returns the smallest value.
Example:
numbers = [1, 2, 3, 4, 5]
print(min(numbers)) # Output: 1
6. round()
The round()
function rounds a floating-point number to a specified number of decimal places.
Example:
print(round(3.14159, 2)) # Output: 3.14
7. sorted()
The sorted()
function returns a sorted list of the specified iterable's elements.
Example:
numbers = [5, 2, 3, 1, 4]
print(sorted(numbers)) # Output: [1, 2, 3, 4, 5]
You can specify an index to sort by using a lambda
function:
arr = [(1, 2), (3, 1), (5, 0)]
print(sorted(arr, key=lambda x: x[1])) # Output: [(5, 0), (3, 1), (1, 2)]
8. del
The del
statement removes an element from a list by index.
Example:
numbers = [1, 2, 3, 4, 5]
del numbers[2]
print(numbers) # Output: [1, 2, 4, 5]
9. sum()
The sum()
function adds all the elements in an iterable.
Example:
numbers = [1, 2, 3, 4, 5]
print(sum(numbers)) # Output: 15
10. eval()
The eval()
function parses and evaluates a string expression.
Example:
result = eval("2 + 3 * 5")
print(result) # Output: 17
11. any()
The any()
function returns True
if any element of the iterable is true.
Example:
print(any([False, True, False])) # Output: True
12. all()
The all()
function returns True
if all elements of the iterable are true.
Example:
print(all([True, True, True])) # Output: True
13. map()
The map()
function applies a function to all the items in an input list.
Example:
def square(x):
return x * x
numbers = [1, 2, 3, 4, 5]
squared = map(square, numbers)
print(list(squared)) # Output: [1, 4, 9, 16, 25]
14. set()
The set()
function creates a set, which is an unordered collection with no duplicate elements.
Example:
numbers = [1, 2, 3, 1, 2, 3]
unique_numbers = set(numbers)
print(unique_numbers) # Output: {1, 2, 3}
15. zip()
The zip()
function combines two or more iterables (lists, tuples, etc.) element-wise.
Example:
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
zipped = zip(list1, list2)
print(list(zipped)) # Output: [(1, 'a'), (2, 'b'), (3, 'c')]
16. itertools.zip
_longest()
The itertools.zip
_longest()
function combines iterables, filling in None
for missing values until the longest iterable is exhausted.
Example:
from itertools import zip_longest
list1 = [1, 2, 3]
list2 = ['a', 'b']
zipped = zip_longest(list1, list2)
print(list(zipped)) # Output: [(1, 'a'), (2, 'b'), (3, None)]
17. filter()
The filter()
function constructs an iterator from elements of an iterable for which a function returns true.
Example:
def is_even(n):
return n % 2 == 0
numbers = [1, 2, 3, 4, 5, 6]
evens = filter(is_even, numbers)
print(list(evens)) # Output: [2, 4, 6]
18. iter()
The iter()
function returns an iterator object. The next()
function retrieves the next item from the iterator.
Example:
numbers = [1, 2, 3, 4, 5]
iterator = iter(numbers)
print(next(iterator)) # Output: 1
print(next(iterator)) # Output: 2
print(next(iterator)) # Output: 3
By understanding and utilizing these built-in functions, you can write more efficient and cleaner Python code. Whether you're sorting data, performing mathematical operations, or manipulating lists, these functions provide powerful tools to streamline your programming tasks.
Subscribe to my newsletter
Read articles from Emeron Marcelle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emeron Marcelle
Emeron Marcelle
As a doctoral scholar in Information Technology, I am deeply immersed in the world of artificial intelligence, with a specific focus on advancing the field. Fueled by a strong passion for Machine Learning and Artificial Intelligence, I am dedicated to acquiring the skills necessary to drive growth and innovation in this dynamic field. With a commitment to continuous learning and a desire to contribute innovative ideas, I am on a path to make meaningful contributions to the ever-evolving landscape of Machine Learning.