How to Structure Your React Projects: A Guide for Beginners
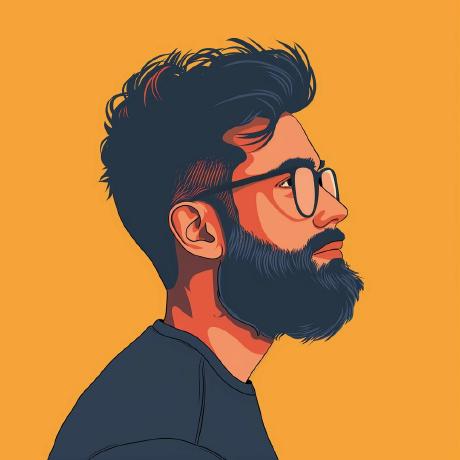
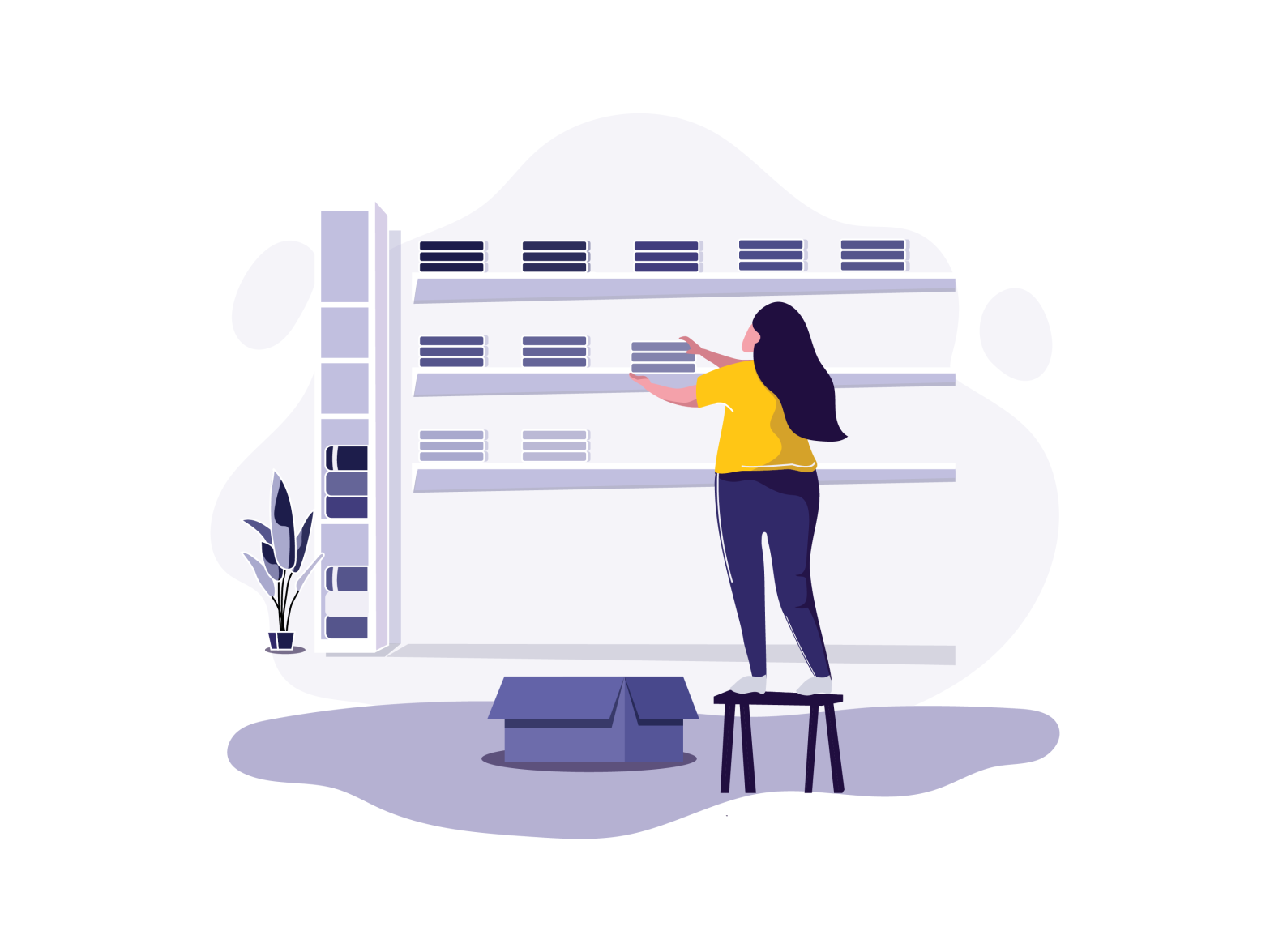
Creating a well-structured React project can be challenging, especially when you're just starting out. In this guide, we'll walk you through three different ways to organize your React project files and folders, suitable for beginner, intermediate, and advanced users. Let's dive in!
Beginner Project Structure
For small projects, keeping things simple is key. Here's a basic structure to get you started:
my-beginner-app/
├── public/
├── src/
│ ├── components/
│ │ ├── Button.js
│ │ ├── FormInput.js
│ │ ├── HomePage.js
│ │ └── NavBar.js
│ ├── hooks/
│ │ └── useTodo.js
│ ├── App.js
│ ├── index.js
│ ├── formatDate.js
│ ├── formatCurrency.js
│ └── App.css
├── package.json
└── .eslintrc
Key Points:
components/: Store all your UI components here.
hooks/: Custom hooks go here.
App.js and index.js: The entry points of your app.
Utility functions: Place them directly in the
src
folder for easy access.
This structure works great for small applications where you don't have too many files to manage.
Intermediate Project Structure
As your project grows, you need a bit more organization. Here’s a structure for medium-sized projects:
my-intermediate-app/
├── public/
├── src/
│ ├── assets/
│ │ ├── images/
│ │ ├── svgs/
│ │ └── global.css
│ ├── components/
│ │ ├── form/
│ │ │ └── FormInput.js
│ │ ├── ui/
│ │ │ ├── Button.js
│ │ │ └── Modal.js
│ │ └── NavBar.js
│ ├── context/
│ │ └── AuthContext.js
│ ├── data/
│ │ └── constants.js
│ ├── hooks/
│ │ └── useTodo.js
│ ├── pages/
│ │ ├── HomePage/
│ │ │ ├── ToDoForm.js
│ │ │ ├── ToDoList.js
│ │ │ ├── ToDoItem.js
│ │ │ ├── NewTodoModal.js
│ │ │ └── index.js
│ │ ├── LoginPage/
│ │ │ └── LoginForm.js
│ │ └── SettingsPage/
│ │ └── SettingsForm.js
│ ├── utils/
│ │ ├── formatDate.js
│ │ └── formatCurrency.js
│ ├── App.js
│ └── index.js
├── package.json
└── .eslintrc
Key Points:
assets/: Store images, SVGs, and global CSS here.
components/: Further organise components into subfolders like
form
andui
.context/: Manage React context files.
data/: Store constants or json data like "list of office locations", "list of courses" etc...
pages/: Each page has its own folder containing all related components.
utils/: For utility functions.
This structure helps keep your files organized as your application grows, making it easier to maintain and scale.
Advanced Project Structure
my-advanced-app/
├── public/
├── src/
│ ├── assets/
│ │ ├── images/
│ │ ├── svgs/
│ │ └── global.css
│ ├── components/
│ │ ├── form/
│ │ │ └── FormInput.js
│ │ ├── ui/
│ │ │ ├── Button.js
│ │ │ └── Modal.js
│ │ └── NavBar.js
│ ├── context/
│ │ └── AuthContext.js
│ ├── data/
│ │ └── constants.js
│ ├── hooks/
│ │ └── useTodo.js
│ ├── layouts/
│ │ ├── NavBarLayout.js
│ │ └── SideBarLayout.js
│ ├── lib/
│ │ └── axios.js
│ ├── services/
│ │ ├── apiService.js
│ │ └── authService.js
│ ├── utils/
│ │ ├── formatDate.js
│ │ └── formatCurrency.js
│ ├── features/
│ │ ├── authentication/
│ │ │ ├── components/
│ │ │ │ └── LoginForm.js
│ │ │ ├── hooks/
│ │ │ │ └── useAuth.js
│ │ │ ├── services/
│ │ │ │ └── authService.js
│ │ │ └── index.js
│ │ ├── todos/
│ │ │ ├── components/
│ │ │ │ ├── ToDoForm.js
│ │ │ │ ├── ToDoItem.js
│ │ │ │ ├── ToDoList.js
│ │ │ │ └── NewTodoModal.js
│ │ │ ├── context/
│ │ │ │ └── ToDoContext.js
│ │ │ ├── hooks/
│ │ │ │ └── useTodo.js
│ │ │ ├── services/
│ │ │ │ └── todoService.js
│ │ │ └── index.js
│ ├── pages/
│ │ ├── HomePage.js
│ │ ├── LoginPage.js
│ │ ├── ProjectsPage.js
│ │ ├── SettingsPage.js
│ │ └── SignUpPage.js
│ ├── App.js
│ └── index.js
├── package.json
└── .eslintrc
Key Points:
layouts/: For layout components like navbars and sidebars.
lib/: Wrap third-party libraries.
services/: API service calls.
features/: Encapsulate all code related to specific features in their own folders.
pages/: Only contain the main components for each page.
This advanced structure ensures your codebase remains maintainable and scalable as your application grows larger and more complex.
Conclusion
Choosing the right project structure depends on the size and complexity of your React application. Start simple, and as your project grows, gradually adopt more sophisticated structures. Happy coding!
Subscribe to my newsletter
Read articles from Rafin Rahman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
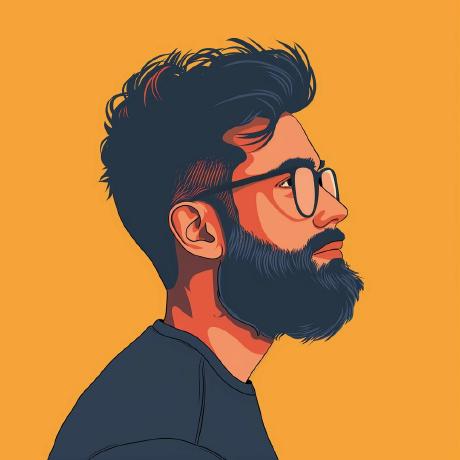
Rafin Rahman
Rafin Rahman
I'm a London-based Software Engineer with over 7 years of experience in the EdTech industry. I love sharing valuable tips, insights, and providing mentorship to aspiring developers. Join me as I explore the world of software engineering, offer career advice, and help new minds thrive in the tech field.