Day 5 : Advanced Linux Shell Scripting for DevOps Engineers with User Management

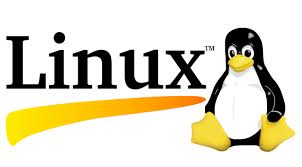
Mastering Shell Scripting for Directory Creation and Automation in DevOps
Shell scripting is a vital skill for DevOps engineers, enabling the automation of repetitive tasks and efficient management of system operations. This blog will guide you through:
Creating directories using a shell script.
Writing a backup script.
Automating the backup script using cron.
Managing users in Linux.
Let’s dive into each topic with examples and explanations.
1. Creating Directories Using Shell Script
Creating multiple directories manually can be tedious. With a shell script, you can automate this task efficiently.
Example: createDirectories.sh
This script takes three arguments (directory name, start number, and end number) and creates a specified number of directories.
#!/bin/bash
# Check if the correct number of arguments is provided
if [ $# -ne 3 ]; then
echo "Usage: $0 directory_name start_number end_number"
exit 1
fi
# Assign arguments to variables
dir_name=$1
start=$2
end=$3
# Loop to create directories
for ((i=start; i<=end; i++)); do
mkdir "${dir_name}${i}"
done
echo "Directories created from ${dir_name}${start} to ${dir_name}${end}"
Example 1: Creating 90 directories named day1 to day90
./createDirectories.sh day 1 90
📸 Screenshot:
Example 2: Creating 31 directories named Movie20 to Movie50
./createDirectories.sh Movie 20 50
📸 Screenshot:
2. Create a Script to Backup All Your Work
Backups are crucial in ensuring data safety. Here’s a script to back up your work to a specified directory.
Example: backupScript.sh
#!/bin/bash
# Define source and backup directories
source_dir="/path/to/your/work"
backup_dir="/path/to/backup/directory"
timestamp=$(date +%Y%m%d%H%M%S)
# Create a backup
tar -czvf "${backup_dir}/backup_${timestamp}.tar.gz" -C "${source_dir}" .
echo "Backup completed successfully at ${backup_dir}/backup_${timestamp}.tar.gz"
📸 Screenshot:
3. Automate the Backup Script Using Cron
Cron is the task scheduler in Unix-like operating systems, and crontab is the file where you define your cron jobs.
Example: Scheduling the Backup Script
- Edit the crontab file:
crontab -e
- Add the following line to run the backup script every day at 2 AM:
0 2 * * * /path/to/backupScript.sh
📸 Screenshot:
4. User Management in Linux
User management is an essential task in Linux systems. Here’s how to create users and display their usernames.
Example: Creating Users
- Create two users:
sudo adduser user1
sudo adduser user2
- Display their usernames:
cut -d: -f1 /etc/passwd | grep 'user1\|user2'
📸 Screenshot:
Conclusion
Shell scripting is a powerful tool for DevOps professionals, enabling automation of tasks, efficient management of directories, data backup, and user management. By mastering these skills, you can streamline your DevOps processes and enhance your productivity. Happy scripting! 🚀
Subscribe to my newsletter
Read articles from Himanshu Palhade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
