Understanding Entity Relationships in C#: One-to-One, One-to-Many, and Many-to-Many Explained
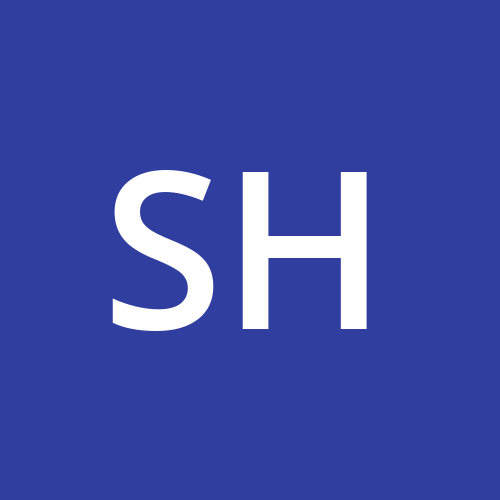
2 min read
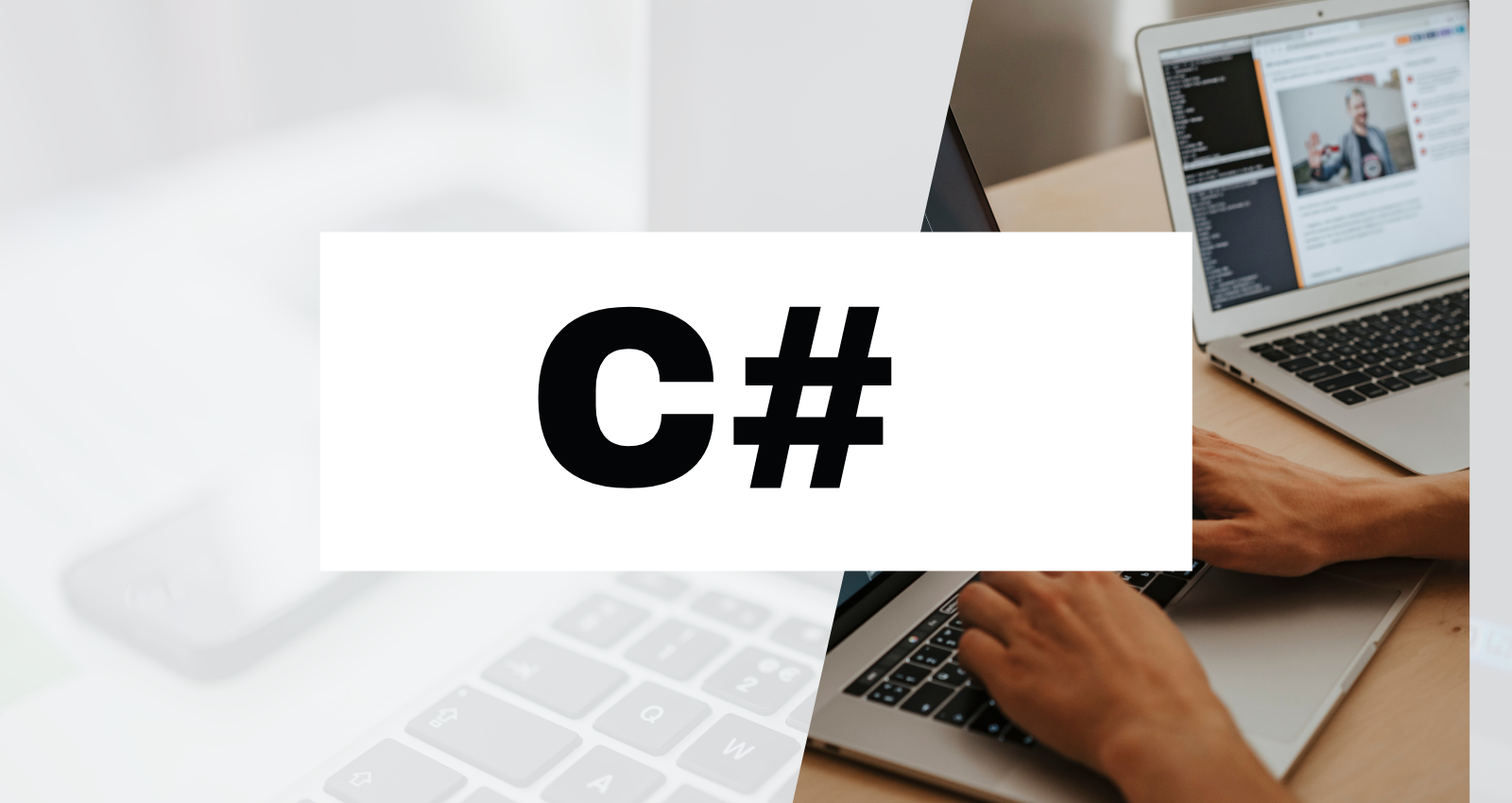
We have different types of Entity Relationships:
One to One
One to Many
Many to Many
One-to-One Relationship
Example: A person has one passport, and a passport is assigned to one person.
Entities:
Person
Passport
Schema:
public class Person
{
public int PersonId { get; set; }
public string Name { get; set; } = string.Empty;
// Navigation property
public Passport Passport { get; set; }
}
public class Passport
{
public int PassportId { get; set; }
public string Number { get; set; } = string.Empty;
public int PersonId { get; set; }
// Navigation property
public Person Person { get; set; }
}
Fluent API Configuration:
modelBuilder.Entity<Person>()
.HasOne(p => p.Passport)
.WithOne(p => p.Person)
.HasForeignKey<Passport>(p => p.PersonId);
One-to-Many Relationship
Example: A teacher can have many students, but a student can only have one teacher.
Entities:
Teacher
Student
Schema:
public class Teacher
{
public int TeacherId { get; set; }
public string Name { get; set; } = string.Empty;
// Navigation property
public ICollection<Student> Students { get; set; } = new List<Student>();
}
public class Student
{
public int StudentId { get; set; }
public string Name { get; set; } = string.Empty;
public int TeacherId { get; set; }
// Navigation property
public Teacher Teacher { get; set; }
}
Fluent API Configuration:
modelBuilder.Entity<Teacher>()
.HasMany(t => t.Students)
.WithOne(s => s.Teacher)
.HasForeignKey(s => s.TeacherId);
Many-to-Many Relationship
Example: A student can enroll in many courses, and a course can have many students.
Entities:
Student
Course
Enrollment
(joining table)
Schema:
public class Student
{
public int StudentId { get; set; }
public string Name { get; set; } = string.Empty;
// Navigation property
public ICollection<Enrollment> Enrollments { get; set; } = new List<Enrollment>();
}
public class Course
{
public int CourseId { get; set; }
public string Title { get; set; } = string.Empty;
// Navigation property
public ICollection<Enrollment> Enrollments { get; set; } = new List<Enrollment>();
}
public class Enrollment
{
public int StudentId { get; set; }
public Student Student { get; set; }
public int CourseId { get; set; }
public Course Course { get; set; }
}
Fluent API Configuration:
modelBuilder.Entity<Enrollment>()
.HasKey(e => new { e.StudentId, e.CourseId });
modelBuilder.Entity<Enrollment>()
.HasOne(e => e.Student)
.WithMany(s => s.Enrollments)
.HasForeignKey(e => e.StudentId);
modelBuilder.Entity<Enrollment>()
.HasOne(e => e.Course)
.WithMany(c => c.Enrollments)
.HasForeignKey(e => e.CourseId);
0
Subscribe to my newsletter
Read articles from Shoyeab directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
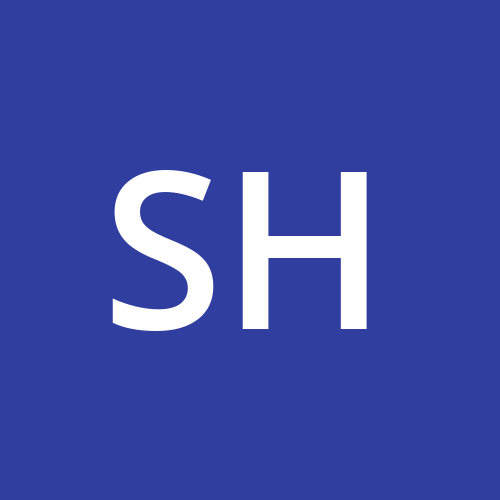
Shoyeab
Shoyeab
Passionate about building Web and mobile applications. Join me as we dive into the world of Tech!