Printing a Right-Angled Triangle shape

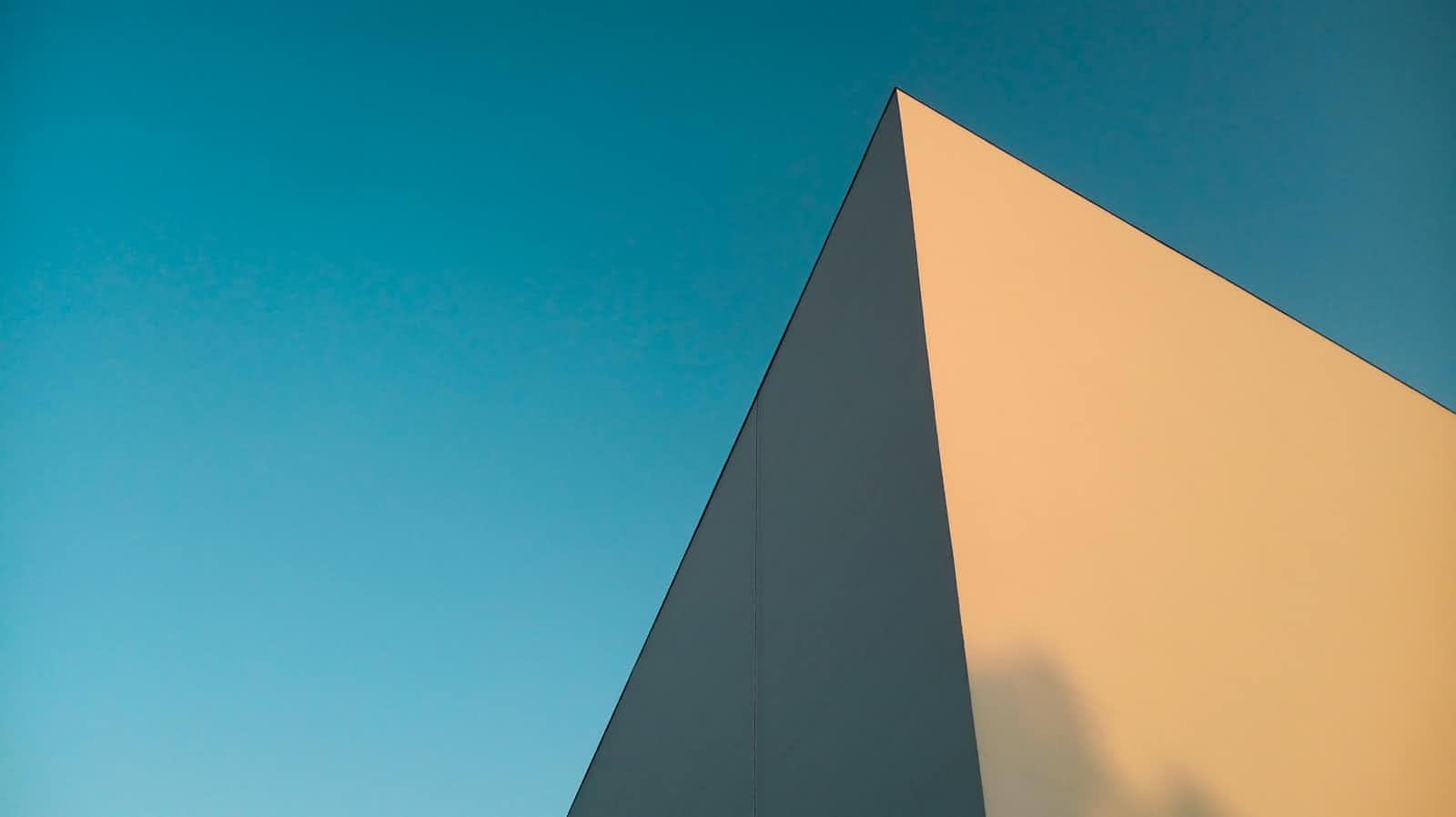
Hello! Welcome to my blog. So far, I've been writing blogs about Mainframe on https://iamamainframer.blogspot.com. A good friend of mine suggested I check out Hashnode, as it offers super cool and awesome content writing tools for developers. That's how I got up here, and this is my first blog post and first series on Hashnode. I hope you enjoy reading it.
Introduction
In the first post of the series 'Printing shapes using COBOL', we'll check out how to print a right-angled triangle.
Unlike shown in the picture above, we'll not leave the triangle hollow. Rather, we'll be filling it up with asterisks.
Approach
The approach is plain. We will be using PERFORM loops and DISPLAY statement(s) in COBOL to generate the shape.
Right-Angled Triangle shape in COBOL
Here is the COBOL program to print the Right-Angled Triangle shape (Unfortunately, Hashnode don't have a COBOL Code editor ๐)
IDENTIFICATION DIVISION.
PROGRAM-ID. RIGHT-ANGLED-TRIANGLE.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-OUT PIC X(10) VALUE ALL '*'.
01 WS-I PIC 9(2) VALUE 0.
01 WS-N PIC 9(2) VALUE 10.
01 WS-CENTER PIC 9(2) VALUE 1.
PROCEDURE DIVISION.
PERFORM VARYING WS-I FROM 1 BY 1 UNTIL WS-I > WS-N
DISPLAY WS-OUT(WS-CENTER:WS-I)
END-PERFORM.
STOP RUN.
Explanation
In the WORKING-STORAGE SECTION, we have the following data-items defined:
WS-OUT is a 10-character string initialized with asterisks (*).
WS-I is the loop counter.
WS-N defines the height of the triangle and is initialized with a value of 10. The entire right-angled triangle will be spread out in 10 lines.
WS-CENTER specifies the starting position for the asterisk.
In the PROCEDURE DIVISION, we have the instructions to be executed.
The PERFORM loop starts from 1 and goes up till WS-N (i.e., 10)
Inside the PERFORM loop, we simply DISPLAY the sub-string of WS-OUT, in each iteration of the loop, using Reference Modification. This way, we can print each line with increasing number of stars.
In the first iteration, we display WS-OUT(1:1) i.e., just one character starting from the first position. Since WS-OUT is already initialized with asterisks, this will DISPLAY a '*' on the SYSOUT.
In the second iteration, we display WS-OUT(1:2) i.e., two characters of WS-OUT starting from the first position. Since WS-OUT is already initialized with asterisks, this will DISPLAY '**' on the SYSOUT.
In the fifth iteration of the loop, 5 characters of WS-OUT starting from the first position will be displayed.
Output
*
**
***
****
*****
******
*******
********
*********
**********
You can execute this code on JDoodle by clicking ๐ here.
Conclusion
To allow the user to select how many lines the triangle should be spread out on, you can make a minor change to the code by accepting the user's value for WS-N.
I hope this helps!
Should you have any questions/suggestions, please post them in the comments section below.
๐
Subscribe to my newsletter
Read articles from Srinivasan JV directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Srinivasan JV
Srinivasan JV
I have been a mainframe developer for the past 10 years. As part of work obligations, I am currently in pursuit of learning Java. You'll find me writing more about the mainframe.