Step-by-Step Guide to Implementing Rainbow Kit and Wagmi in Next.js
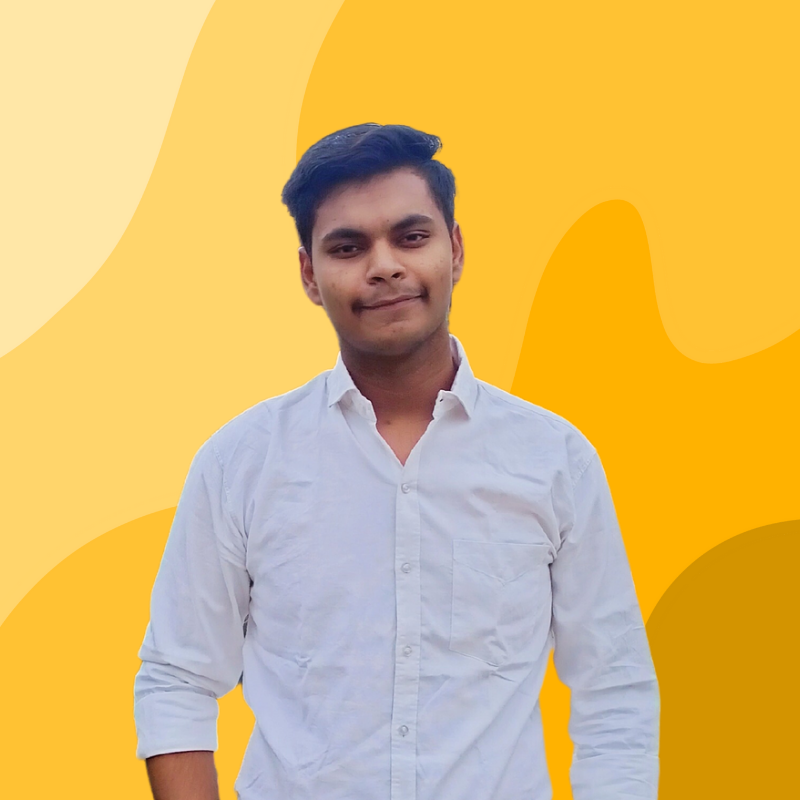
Introduction
This guide will walk you through the process of implementing Rainbow Kit with Wagmi in a Next.js application. Rainbow Kit is a React library that makes it easy to add wallet connection to your dApp, while Wagmi is a collection of React Hooks for Ethereum that makes it easy to work with wallets, ENS, contracts, transactions, and more.
Prerequisites
Node.js (v14 or later)
npm or yarn
A Next.js project
Step 1: Installation
First, install the necessary dependencies:
npm install @rainbow-me/rainbowkit wagmi viem @tanstack/react-query
Step 2: Configuration
Create a wagmi.ts
file in your project root to configure Wagmi and Rainbow Kit:
import { getDefaultConfig } from '@rainbow-me/rainbowkit';
import {
arbitrum,
base,
mainnet,
optimism,
polygon,
sepolia,
} from 'wagmi/chains';
export const config = getDefaultConfig({
appName: 'Your App Name',
projectId: process.env.NEXT_PUBLIC_WALLET_CONNECT_PROJECT_ID as string,
chains: [
mainnet,
polygon,
optimism,
arbitrum,
base,
...(process.env.NEXT_PUBLIC_ENABLE_TESTNETS === 'true' ? [sepolia] : []),
],
ssr: true,
});
Make sure to set the NEXT_PUBLIC_WALLET_CONNECT_PROJECT_ID
in your .env.local
file.
Step 3: Create a Providers Component
Create a providers.tsx
file to wrap your application with the necessary providers:
'use client';
import * as React from 'react';
import { QueryClient, QueryClientProvider } from '@tanstack/react-query';
import { WagmiProvider } from 'wagmi';
import { RainbowKitProvider } from '@rainbow-me/rainbowkit';
import { config } from './wagmi';
const queryClient = new QueryClient();
export function Providers({ children }: { children: React.ReactNode }) {
return (
<WagmiProvider config={config}>
<QueryClientProvider client={queryClient}>
<RainbowKitProvider>{children}</RainbowKitProvider>
</QueryClientProvider>
</WagmiProvider>
);
}
Step 4: Update Your Root Layout
Modify your app/layout.tsx
file to include the Providers and Rainbow Kit styles:
import type { Metadata } from "next";
import { Providers } from './providers';
import '@rainbow-me/rainbowkit/styles.css';
// ... other imports
export default function RootLayout({
children,
}: {
children: React.ReactNode;
}) {
return (
<html lang="en">
<body>
<Providers>
{/* Your other providers and components */}
{children}
</Providers>
</body>
</html>
);
}
Step 5: Using the ConnectButton
Now you can use the ConnectButton
component from Rainbow Kit in any of your components. For example, in a Navbar component:
import { ConnectButton } from '@rainbow-me/rainbowkit';
const Navbar: React.FC = () => {
return (
<nav>
{/* Other navbar items */}
<ConnectButton />
</nav>
);
};
export default Navbar;
Best Practices
Environment Variables: Always use environment variables for sensitive information like project IDs.
Server-Side Rendering (SSR): The
ssr: true
option in the Wagmi configuration ensures compatibility with Next.js SSR.Type Safety: Utilize TypeScript for better type-checking and developer experience.
Error Handling: Implement proper error handling for wallet connection failures and network issues.
Responsive Design: Ensure your wallet connection UI is responsive and works well on both desktop and mobile devices.
Troubleshooting Common Errors
During the implementation of Rainbow Kit with Wagmi in your Next.js application, you may encounter various errors. Here's a list of common issues and their solutions:
1. WagmiProviderNotFoundError
Error Message:
WagmiProviderNotFoundError: `useConfig` must be used within `WagmiProvider`.
Solution:
Ensure that all components using Wagmi hooks or Rainbow Kit components are wrapped within the
Providers
component.Check that your
Providers
component is correctly placed in your component tree, typically in the root layout.
2. RainbowKitProvider Error
Error Message:
Error: RainbowKitProvider must be inside WagmiProvider
Solution:
Verify that your
Providers
component is set up correctly, withRainbowKitProvider
nested insideWagmiProvider
.Double-check the order of providers in your
Providers
component.
3. Invalid Hook Call
Error Message:
Invalid hook call. Hooks can only be called inside of the body of a function component.
Solution:
Ensure you're not using Rainbow Kit or Wagmi hooks outside of React functional components.
If using Next.js 13+ with the App Router, add
'use client'
at the top of files containing client-side only code.
4. Hydration Failed
Error Message:
Hydration failed because the initial UI does not match what was rendered on the server.
Solution:
This often occurs with SSR. Ensure you're using
dynamic
imports with{ ssr: false }
for components that should only render on the client side.Alternatively, wrap the problematic component with a client-side only wrapper.
5. ProjectId Not Found
Error Message:
ProjectId not found. Please provide a project id.
Solution:
Verify that you've set the
NEXT_PUBLIC_WALLET_CONNECT_PROJECT_ID
in your.env.local
file.Ensure that you're correctly accessing the environment variable in your Wagmi configuration.
6. Chain Not Configured
Error Message:
Chain "X" not configured
Solution:
Check that you've properly configured all the chains you intend to use in your
wagmi.ts
file.Ensure you're not trying to connect to a chain that's not included in your configuration.
7. Module Not Found
Error Message:
Module not found: Can't resolve '@rainbow-me/rainbowkit'
Solution:
Run
npm install @rainbow-me/rainbowkit
to ensure the package is installed.Check your
package.json
to verify the package is listed in the dependencies.
General Troubleshooting Tips
Clear Cache and Reinstall Dependencies: Sometimes, clearing your Next.js cache and reinstalling dependencies can resolve mysterious issues:
rm -rf .next rm -rf node_modules npm install
Check Version Compatibility: Ensure that your versions of Next.js, Rainbow Kit, and Wagmi are compatible with each other.
Console Logging: Use console.log statements to debug and track the flow of your application.
React DevTools: Use React DevTools to inspect your component tree and ensure providers are wrapping components correctly.
TypeScript: If you're using TypeScript, make sure your types are up to date and correctly imported.
By addressing these common issues and following the troubleshooting tips, you should be able to resolve most problems encountered while implementing Rainbow Kit with Wagmi in your Next.js application.
Conclusion
By following this guide, you've successfully implemented Rainbow Kit with Wagmi in your Next.js application. This setup provides a robust foundation for building decentralized applications with easy wallet connection functionality.
Remember to keep your dependencies updated and refer to the official documentation of Rainbow Kit and Wagmi for advanced features and updates.
Resources
Subscribe to my newsletter
Read articles from Yash Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
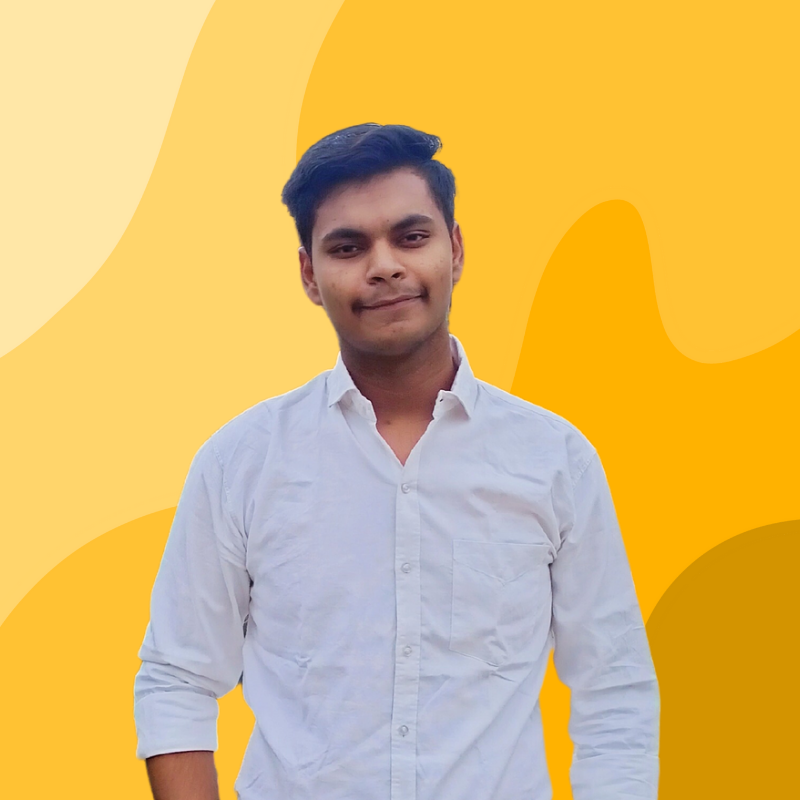
Yash Jain
Yash Jain
Blockchain Developer