Building a Full-Stack Application with React and Node.js

Table of contents
- Introduction
- Setting Up the Development Environment
- Understanding the Basics
- Project Planning and Structure
- Building the Backend with Node.js and Express
- Database Integration
- User Authentication and Authorization
- Building the Frontend with React
- Integrating Frontend and Backend
- Advanced React Techniques
- Real-Time Features with WebSockets
- Error Handling and Logging
- Testing and Debugging
- Deployment and Scaling
- Security Best Practices
- Performance Optimization
- Monitoring and Maintenance
- Case Study: Building a Real-World Application
- Conclusion
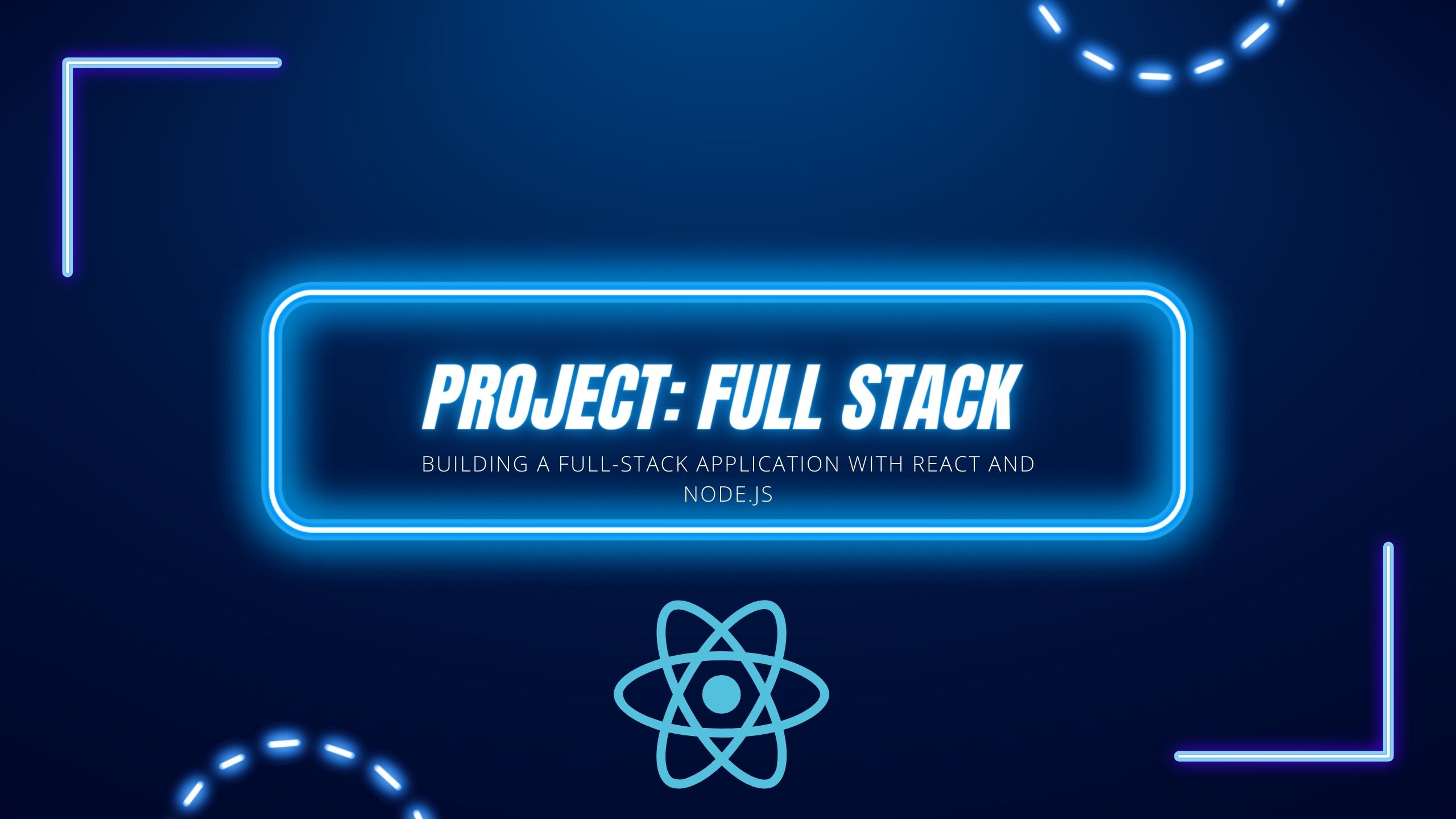
Introduction
Why Choose React and Node.js for Full-Stack Development
In the evolving landscape of web development, selecting the right technology stack is pivotal. React and Node.js have emerged as a formidable duo for building full-stack applications. React, a JavaScript library developed by Facebook, is celebrated for its component-based architecture, enabling developers to create interactive and dynamic user interfaces. Node.js, a server-side platform built on Chrome's V8 JavaScript engine, allows for the development of scalable and high-performance backend services. The synergy between React and Node.js facilitates a seamless development experience, leveraging JavaScript for both client-side and server-side programming, which streamlines the development process and enhances productivity.
Overview of a Full-Stack Application Architecture
A full-stack application encompasses both frontend and backend components, forming a cohesive and functional system. The frontend, powered by React, handles the user interface and client-side logic. It interacts with the backend, built with Node.js and Express, which manages server-side logic, databases, and API endpoints. This architecture ensures a clear separation of concerns, promoting modularity and maintainability. The frontend communicates with the backend through HTTP requests, enabling dynamic data fetching and real-time updates. This harmonious interaction between the client and server forms the backbone of a robust and scalable full-stack application.
Setting Up the Development Environment
Installing Node.js and npm
The first step in building a full-stack application is setting up the development environment. Begin by installing Node.js, which comes with npm, the Node package manager. npm facilitates the installation and management of dependencies required for your project. Visit the official Node.js website, download the installer for your operating system, and follow the instructions to complete the installation.
Setting Up a React Development Environment with Create React App
Create React App is a convenient tool for setting up a React development environment. It provides a boilerplate configuration, eliminating the need for complex setup processes. To get started, open your terminal and run the command npx create-react-app my-app
, replacing my-app
with your desired project name. This command scaffolds a new React project with a predefined structure, enabling you to focus on development rather than configuration.
Installing Essential Tools and Extensions
To enhance your development workflow, install essential tools and extensions. Visual Studio Code is a popular code editor with a rich ecosystem of extensions. Install extensions like ESLint for code linting, Prettier for code formatting, and the React Developer Tools for debugging React applications. These tools streamline development, ensuring code quality and consistency.
Understanding the Basics
Introduction to React: Components, State, and Props
React's architecture revolves around components, which are reusable building blocks that encapsulate UI elements and logic. Components can be functional or class-based, with functional components being more prevalent due to the advent of hooks. State and props are integral to managing component data. State represents mutable data within a component, while props allow for the passing of data between components. This unidirectional data flow promotes predictable and maintainable code.
Introduction to Node.js: Server-Side JavaScript
Node.js revolutionizes server-side programming by enabling JavaScript execution outside the browser. Its non-blocking, event-driven architecture makes it ideal for building scalable and high-performance applications. Node.js applications are built using modules, with the core modules providing essential functionalities like file system access, networking, and HTTP handling. npm further extends Node.js capabilities by offering a vast repository of third-party packages.
Overview of Express.js for Building Node.js Servers
Express.js is a minimalist web framework for Node.js, simplifying the development of server-side applications. It provides a robust set of features for building web and mobile applications, including routing, middleware support, and HTTP utility methods. Express.js abstracts the complexities of low-level HTTP handling, enabling developers to build scalable and maintainable APIs with ease.
Project Planning and Structure
Defining Project Requirements and Scope
Before diving into development, it's crucial to define the project requirements and scope. Identify the core features, target audience, and use cases of your application. This planning phase ensures that development efforts are aligned with the project's goals, preventing scope creep and ensuring a focused development process.
Designing the Application Architecture
Designing a scalable and maintainable application architecture is fundamental to the success of a full-stack project. Break down the application into modular components and services, defining the interactions between the frontend and backend. Consider aspects like data flow, state management, and API design. A well-architected application promotes code reusability, scalability, and ease of maintenance.
Setting Up the Project Directory Structure
A well-organized directory structure enhances the maintainability of your project. Separate frontend and backend code into distinct directories. Within the frontend directory, organize components, hooks, and styles into subdirectories. For the backend, separate routes, controllers, models, and middleware. This modular structure ensures a clear separation of concerns, simplifying development and collaboration.
Building the Backend with Node.js and Express
Setting Up a Basic Express Server
Start by setting up a basic Express server. Create a new directory for your backend and initialize a Node.js project with npm init
. Install Express with npm install express
. Create an index.js
file and set up a basic server:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
Creating and Configuring Routes
Routes define the endpoints of your API. Organize routes based on functionality. Create a routes
directory and define routes for different resources. Use Express's router to modularize route definitions, promoting code organization and maintainability.
Connecting to a Database with Mongoose (MongoDB)
MongoDB, a NoSQL database, pairs seamlessly with Node.js. Use Mongoose, an ODM (Object Data Modeling) library, to interact with MongoDB. Install Mongoose with npm install mongoose
and establish a connection in your server setup. Define schemas and models to structure your data.
Building RESTful API Endpoints
RESTful APIs follow a stateless, client-server architecture. Define endpoints for CRUD operations, adhering to REST principles. Use Express to handle HTTP methods and routes. Implement controllers to encapsulate business logic, ensuring a clean separation of concerns.
Database Integration
Choosing the Right Database: SQL vs. NoSQL
Selecting the appropriate database depends on your application's requirements. SQL databases like MySQL and PostgreSQL offer structured data storage with ACID compliance. NoSQL databases like MongoDB provide flexible schema design, horizontal scalability, and ease of integration with JavaScript-based applications. Assess your data model and scalability needs to make an informed decision.
Setting Up MongoDB with Mongoose
MongoDB's document-oriented storage model pairs well with JavaScript applications. Set up a MongoDB instance locally or use a cloud provider like MongoDB Atlas. Use Mongoose to define schemas, enforce data validation, and interact with the database. Establish a connection in your Express server and create models to represent collections.
Designing Database Schemas
Database schemas define the structure of your data. In Mongoose, schemas allow you to define the shape of documents within a collection. Design schemas that reflect your application's data model, incorporating validation rules and default values. Use schema methods and virtuals to add custom logic and computed properties.
CRUD Operations with Mongoose
Implement CRUD operations using Mongoose models. Define methods for creating, reading, updating, and deleting documents. Use Mongoose's query builders to construct complex queries. Handle errors gracefully and provide meaningful responses to clients.
User Authentication and Authorization
Implementing JWT Authentication
JWT (JSON Web Token) is a popular method for implementing authentication. Generate tokens on successful login and use them to authenticate subsequent requests. Use libraries like jsonwebtoken
to create and verify tokens. Store tokens securely on the client and validate them on the server.
Protecting Routes with Middleware
Middleware functions in Express allow for pre-processing of requests. Use middleware to protect routes, ensuring that only authenticated users can access certain endpoints. Implement role-based access control by checking user roles within middleware.
Managing User Roles and Permissions
Define user roles and permissions to control access to different parts of your application. Store role information in the user model and check permissions in route handlers. This granular control enhances security and allows for differentiated access based on user roles.
Building the Frontend with React
Setting Up a Basic React Application
Use Create React App to scaffold a new React project. This tool provides a pre-configured setup, including Webpack, Babel, and a development server. Run npx create-react-app my-frontend
to create a new React application. Familiarize yourself with the project structure and available scripts.
Creating and Managing React Components
React's component-based architecture promotes code reuse and modularity. Create functional components for different parts of your UI. Use props to pass data between components and state to manage component-specific data. Organize components into directories based on functionality.
State Management with React Hooks
React hooks provide a modern way to manage state and side effects in functional components. Use the useState
hook to manage local state and the useEffect
hook to handle side effects like data fetching and subscriptions. Leverage custom hooks to encapsulate reusable logic.
Using Context API for Global State Management
The Context API allows for global state management without the need for prop drilling. Create a context and a provider component to wrap your application. Use the useContext
hook to access global state within components. This approach simplifies state management for larger applications.
Integrating Frontend and Backend
Making API Calls with Axios
Axios is a popular library for making HTTP requests from the frontend. Install Axios with npm install axios
and use it to fetch data from your backend API. Create a
service layer to encapsulate API calls, promoting code organization and reusability.
Handling Asynchronous Data in React
Handling asynchronous data in React involves managing loading states and errors. Use state variables to track the loading status and error messages. Display loading indicators and error messages to provide feedback to users. Handle API responses and update the component state accordingly.
Displaying Data from the Backend in the React Frontend
Fetch data from your backend API and display it in your React components. Use the useEffect
hook to make API calls when components mount. Map over fetched data to render dynamic lists and tables. Ensure that your UI updates reactively to changes in the data.
Advanced React Techniques
Using React Router for Navigation
React Router enables client-side routing, allowing for single-page application navigation. Install React Router with npm install react-router-dom
and configure routes using the BrowserRouter
, Route
, and Switch
components. Create navigational links and use route parameters to build dynamic routes.
Optimizing Performance with React Memo and Lazy Loading
Optimize React performance by using React.memo
to memoize functional components, preventing unnecessary re-renders. Implement lazy loading with React.lazy
and Suspense
to defer the loading of non-critical components. These techniques enhance the responsiveness of your application.
Implementing Form Validation with Formik and Yup
Formik and Yup simplify form handling and validation in React. Install Formik with npm install formik
and Yup with npm install yup
. Use Formik to manage form state and handle submissions. Define validation schemas with Yup to enforce input validation rules.
Real-Time Features with WebSockets
Introduction to WebSockets
WebSockets enable real-time, bidirectional communication between the client and server. Unlike traditional HTTP requests, WebSockets maintain an open connection, allowing for instant data exchange. This makes WebSockets ideal for applications requiring real-time updates, such as chat applications and live data feeds.
Setting Up Socket.io on the Server
Socket.io is a library that simplifies WebSocket implementation. Install Socket.io on your Node.js server with npm install
socket.io
. Integrate it with your Express server to handle WebSocket connections. Define event handlers to manage real-time communication.
Integrating Socket.io with React for Real-Time Updates
Integrate Socket.io with your React frontend to enable real-time updates. Install the client library with npm install
socket.io
-client
. Establish a connection to the server and listen for events. Use state to update the UI dynamically based on real-time data.
Error Handling and Logging
Implementing Error Handling in Express
Robust error handling enhances the reliability of your application. Use middleware to catch and handle errors in Express. Define custom error classes for different types of errors. Send meaningful error responses to the client and log errors for debugging.
Managing Errors in React
Handle errors gracefully in React components. Use error boundaries to catch rendering errors and display fallback UI. Manage asynchronous errors in API calls and display error messages to users. Ensure that your application recovers gracefully from errors.
Setting Up Logging with Winston
Logging is essential for monitoring and debugging applications. Install Winston, a versatile logging library, with npm install winston
. Configure Winston to log messages to different transports, such as files and consoles. Implement logging in your Express server to track application events and errors.
Testing and Debugging
Writing Unit Tests for Node.js with Jest
Unit testing ensures the reliability of your code. Jest is a popular testing framework for JavaScript. Install Jest with npm install jest
and write unit tests for your Node.js code. Test individual functions and modules to ensure they work as expected.
Testing React Components with React Testing Library
React Testing Library promotes testing React components in a user-centric manner. Install it with npm install @testing-library/react
. Write tests to verify component behavior and interactions. Use assertions to check the rendered output and simulate user events.
Debugging Techniques for Node.js and React
Effective debugging saves time and improves code quality. Use built-in debugging tools in Node.js, such as the debug
module and the Node.js inspector. For React, use browser developer tools and the React Developer Tools extension. Set breakpoints, inspect variables, and step through code to identify and fix issues.
Deployment and Scaling
Preparing the Application for Production
Preparing your application for production involves optimizing performance and ensuring security. Minify and bundle your frontend code. Configure environment variables for different environments. Implement caching and compression to improve load times.
Deploying the Backend with Heroku
Heroku is a cloud platform that simplifies backend deployment. Create a Heroku account and install the Heroku CLI. Initialize a Git repository and deploy your Node.js server to Heroku. Configure environment variables and add necessary buildpacks.
Deploying the Frontend with Vercel
Vercel is a popular platform for deploying frontend applications. Create a Vercel account and install the Vercel CLI. Connect your React project to Vercel and deploy it with a single command. Vercel handles build and deployment, providing a seamless experience.
Scaling the Application for High Traffic
Scaling your application ensures it can handle increased traffic and load. Use load balancers to distribute traffic across multiple servers. Implement horizontal scaling for your backend by deploying multiple instances. Optimize database performance and use caching to reduce load on the server.
Security Best Practices
Protecting Against Common Security Threats
Security is paramount in web development. Protect your application against common threats like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Validate and sanitize user inputs, use secure headers, and implement proper authentication and authorization mechanisms.
Implementing HTTPS and Secure Headers
HTTPS encrypts data transmitted between the client and server, ensuring data integrity and confidentiality. Obtain an SSL certificate and configure your server to use HTTPS. Use secure headers like Content Security Policy (CSP) and Strict-Transport-Security (HSTS) to enhance security.
Regular Security Audits and Updates
Regular security audits identify vulnerabilities in your application. Use tools like npm audit to scan for known security issues in dependencies. Keep your packages and libraries up to date. Conduct periodic security reviews and penetration testing to ensure robust security.
Performance Optimization
Optimizing Database Queries
Efficient database queries reduce load times and improve performance. Use indexes to speed up query execution. Optimize query logic and avoid unnecessary data retrieval. Monitor database performance and identify slow queries using profiling tools.
Improving Server Response Times
Fast server response times enhance user experience. Use caching to store frequently accessed data. Optimize middleware and route handling to reduce processing time. Implement asynchronous processing for long-running tasks.
Enhancing React Performance with Code Splitting and Memoization
Optimize React performance by splitting your code into smaller bundles. Use dynamic imports and React.lazy
to load components on demand. Implement memoization with React.memo
and useMemo
to prevent unnecessary re-renders. These techniques improve load times and responsiveness.
Monitoring and Maintenance
Setting Up Application Monitoring with New Relic
Application monitoring provides insights into performance and errors. New Relic is a comprehensive monitoring tool. Install the New Relic agent in your Node.js server and configure it to collect performance data. Use New Relic's dashboard to monitor application metrics and identify issues.
Managing Application Logs
Logs provide valuable information for debugging and monitoring. Use Winston to manage logs in your application. Configure log levels and transports to capture relevant information. Regularly review logs to identify and resolve issues.
Planning for Regular Maintenance and Updates
Regular maintenance ensures your application remains secure and performant. Schedule periodic updates to dependencies and libraries. Conduct code reviews and refactoring to improve code quality. Plan for feature enhancements and bug fixes to keep your application up to date.
Case Study: Building a Real-World Application
Project Overview and Requirements
To illustrate the concepts covered, let's build a real-world application. Assume we are creating a task management tool. The application will have features like user authentication, task creation, and real-time updates.
Step-by-Step Implementation
Start by setting up the development environment and initializing the project. Build the backend with Node.js and Express, creating API endpoints for user authentication and task management. Set up MongoDB for data storage and implement JWT authentication. Build the frontend with React, creating components for the task list and user interface. Integrate the frontend and backend, making API calls and displaying data.
Challenges and Solutions
Throughout the project, you may encounter challenges like handling real-time updates and optimizing performance. Use WebSockets to implement real-time features and ensure a responsive user experience. Optimize database queries and React performance to handle high traffic. Regularly test and debug the application to identify and fix issues.
Conclusion
Recap of Key Concepts
In this comprehensive guide, we've explored building a full-stack application with React and Node.js. We've covered setting up the development environment, understanding the basics, project planning, building the backend and frontend, integrating the two, and advanced techniques like real-time features and performance optimization.
Future Trends in Full-Stack Development
The future of full-stack development looks promising, with advancements in serverless architecture, GraphQL, and microservices. These trends aim to improve scalability, performance, and developer experience. Staying updated with these trends ensures you remain competitive in the ever-evolving tech landscape.
Additional Resources for Learning React and Node.js
To deepen your knowledge, explore resources like the official React and Node.js documentation, online tutorials, and community forums. Books like "Learning React" by Kirupa Chinnathambi and "Node.js Design Patterns" by Mario Casciaro offer in-depth insights. Engaging with the developer community on platforms like GitHub and Stack Overflow can also provide valuable learning opportunities.
Subscribe to my newsletter
Read articles from Manvendra Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Manvendra Singh
Manvendra Singh
R3F | Laravel | Babylon | Three.js | WordPress Performance Enthusiast