Printing a Square shape in COBOL

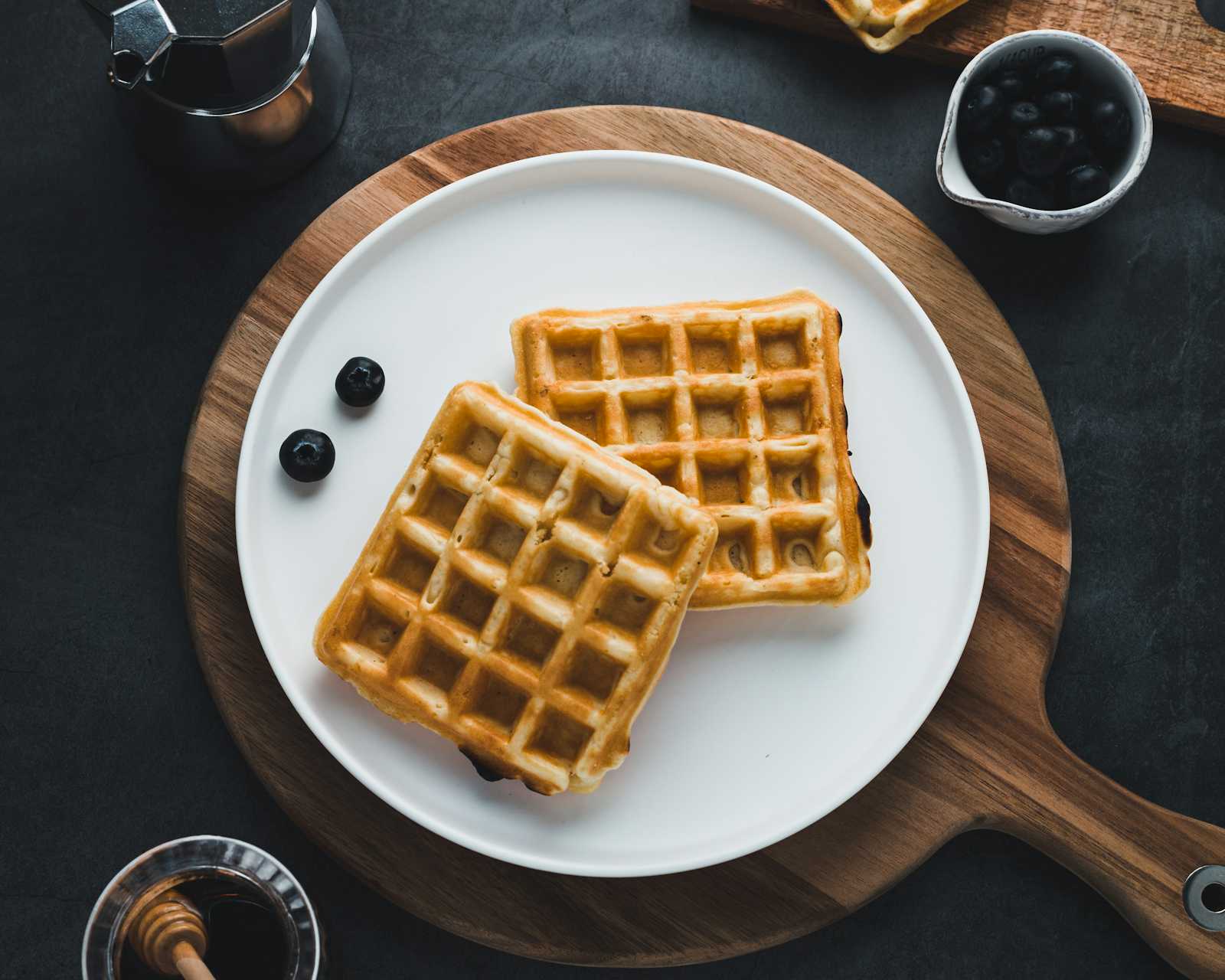
Introduction
In this post, I'll show you how to generate a square shape ๐ฅ using COBOL.
We'll use asterisk (*) symbol to form the shape. Not just the border, but we'll also be using the asterisks to fill up the square shape.
Approach
All four sides of the square are equal, so we will be declaring a data item, WS-N which will represent both the number of lines in which the shape will be spread out as well as the number of asterisks in each given line.
PERFORM loop and DISPLAY statements in COBOL will be used to generate the shape.
Square shape in COBOL
Here ๐ is the COBOL program to print the square shape.
IDENTIFICATION DIVISION.
PROGRAM-ID. SQUARE-PATTERN.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-OUT PIC X(80) VALUE ALL '*'.
01 WS-I PIC 9(2) VALUE 0.
01 WS-N PIC 9(2) VALUE 10.
01 WS-CENTER PIC 9(2) VALUE 1.
PROCEDURE DIVISION.
PERFORM VARYING WS-I FROM 1 BY 1 UNTIL WS-I > WS-N
DISPLAY WS-OUT(WS-CENTER:WS-N)
END-PERFORM.
STOP RUN.
What the code does?
In the WORKING-STORAGE SECTION, we have the following data-items defined:
WS-OUT is an 80-character string initialized with asterisks (*). I've programmatically limited the maximum size of the square to 80.
WS-I is the loop counter.
WS-N defines the size of the sides of the square and is initialized with a value of 10. The entire square shape will be spread out in 10 lines, with 10 characters in each line.
WS-CENTER specifies the starting position for the shape.
In the PROCEDURE DIVISION, we have the instructions to be executed.
The PERFORM loop starts from 1 and goes up till WS-N (i.e., 10)
Inside the PERFORM loop, we simply DISPLAY the sub-string of WS-OUT in each iteration of the loop, using reference modification. This way, we can print each line with increasing number of stars.
In the first iteration, we display WS-OUT(1:10) i.e., 10 characters starting from the first position. Since WS-OUT is already initialized with asterisks, this will DISPLAY '**********' on the SYSOUT.
We repeat the same DISPLAY statement until WS-I is greater than WS-N (i.e., 10 times).
Output
**********
**********
**********
**********
**********
**********
**********
**********
**********
**********
Hmm, this may look more like a rectangle than a square because the code editor has put a good number of spaces between each line, and they are not compactly packed.
Nevertheless, you've got the shape. If you count the total number of asterisks on each side of the shape, they will match.
You can run the program on JDoodle by clicking here.
Conclusion
That's it, folks. This post contributes to a series titled 'Printing Shapes Using COBOL' and there are more to come.
Should you have any questions/feedback, please keep them coming in the comments section below.
Thanks for reading!
๐
Subscribe to my newsletter
Read articles from Srinivasan JV directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Srinivasan JV
Srinivasan JV
I have been a mainframe developer for the past 10 years. As part of work obligations, I am currently in pursuit of learning Java. You'll find me writing more about the mainframe.