Hoisting & Temporal Dead Zone in JavaScript
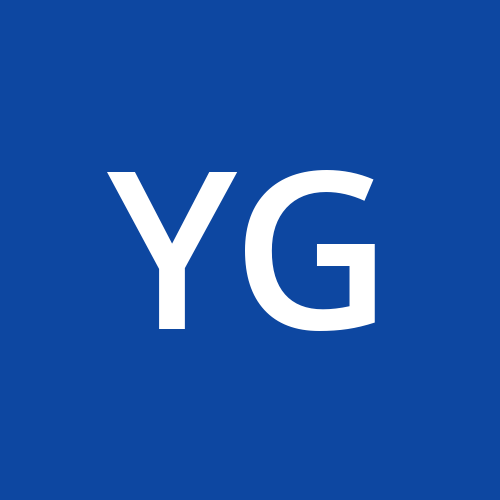
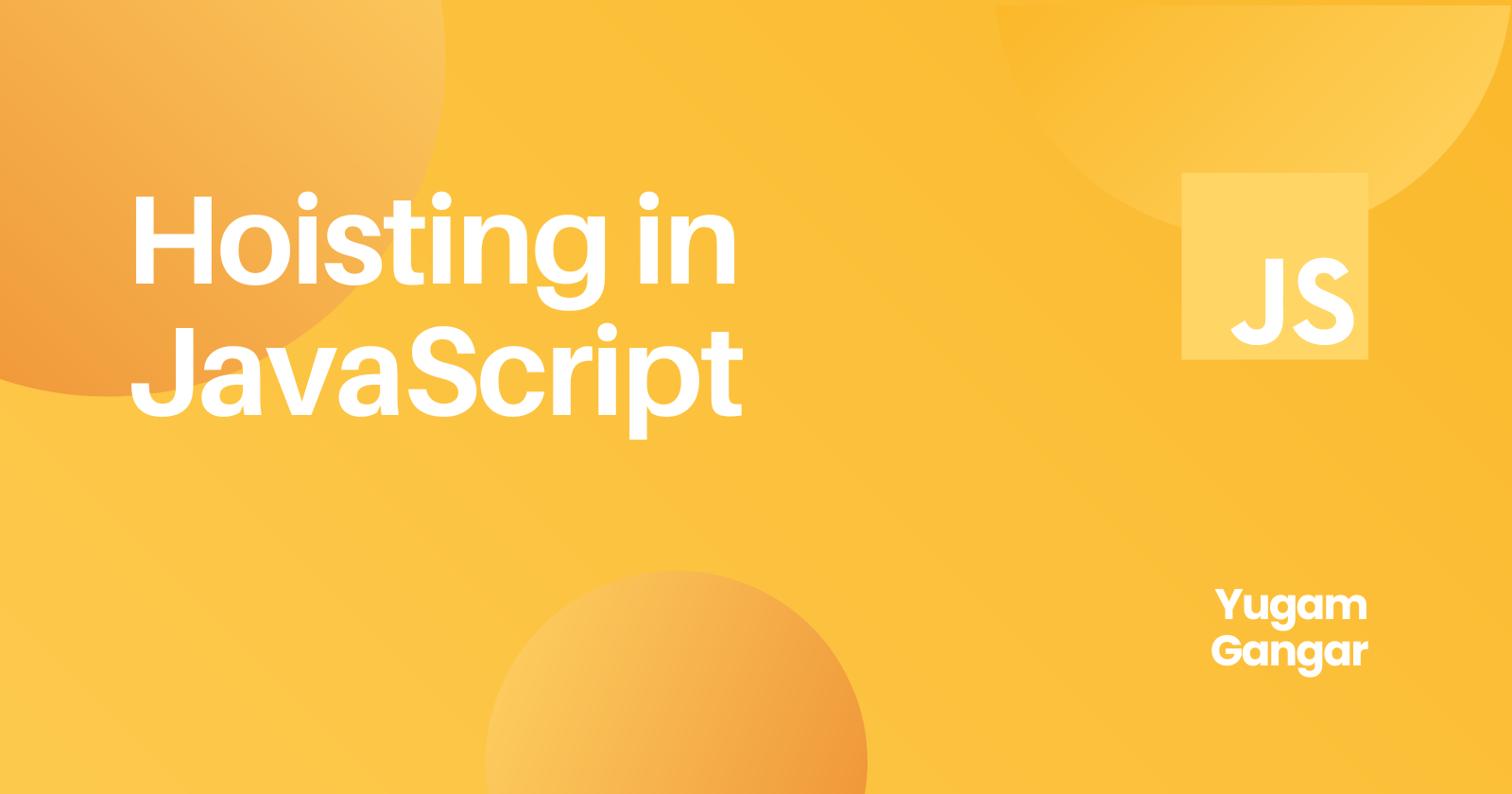
We all might have heard of "hoisting" while working in JavaScript. But not everyone knows in depth about it. In this post let's dive deep into it.
var colour = "white";
console.log(colour); // white
Here it's a simple block of code where the variable is defined and consoled in the next line. But even if the variable is defined in one line the JavaScript interpreter splits the declaration and initializations of variables.
// Variable Lifecycle
var colour; // declaration
colour = "white"; // initialization
console.log(colour); // usage
This means the JavaScript interpreter moves the declaration of variables, functions and classes at the top of its contained scope before its initialization or execution. This process is called hoisting.
Hoisting is the process in JavaScript execution where the interpreter moves declarations of functions, variables & classes to the top of their containing scope before the code execution.
However, hoisting is limited to the declaration and not initialization/assignment. Hoisting plays an important role in how our code is executed. Based on the variable or function declaration it allows us to access a variable or call a function before it is declared in your code, but be aware that it might also result in errors. Let's understand in depth about this with examples.
Variable Hoisting
Variable hoisting in JavaScript works differently based on how the variable is declared. This means hoisting works differently for variables declared with var
& variables declared with let
and const
.
Hoisting of variables declared usingvar
keyword:
console.log(colour); // undefined
var colour = "white";
console.log(colour); // white
// another example
console.log(number); // undefined
number = 001; // This is same as declaring variable using "var"
Do you wonder why this block of code does not throw an error even though we are using colour
variable before its declaration? It's because of hoisting.
Right before the code execution, the JavaScript language interpreter creates the variables declared using var
in the memory heap and assigns them as undefined
.
ReferenceError: variable not defined
Post this the value is assigned to the variable when that line of code is executed which is why we get the string value "white" for the second console after its declaration.
Hoisting of variables declared usinglet
andconst
keyword:
Since using a variable before its declaration is cautious and may lead to confusion or errors in the real world, the let
& const
keywords for variable declaration with the concept of Temporal Dead Zone were introduced in ECMAScript 2015.
console.log(colour); // ReferenceError: Cannot access 'colour' before initialization
let colour = "white"
console.log(colour);
console.log(pi); // ReferenceError: Cannot access 'pi' before initialization
const pi = 3.14;
console.log(pi);
In the example above, an error occurs when attempting to use the variables before they are declared. This is because, during compilation, the JavaScript interpreter declares these variables in a special memory location known as the Temporal Dead Zone.
let
and const
.Inside the TDZ, variables are just declared and not initialized unlike the regular variable declaration using var
keyword. The TDZ starts at the beginning of the contained scope of the variable and ends when it is declared.
let
or const
for variable declaration, as the JavaScript engine prevents them from using before declaration.Function Hoisting
Similar to variables functions in JavaScript are hoisted at the top of their enclosing scope. Function hoisting is also limited to its declaration. It does not execute until it has been called.
console.log(car); // function car () {...}
car(); // Console: Honda Accord
function car(){
var name = "Honda Accord";
console.log(name);
}
The car
function in the above example is hoisted at the top of the code block, so the whole function body is consoled on the first line. In the case of function declarations, the entire function is hoisted, including its body. This means that a function can be called before it is declared.
var
,let
or const
keyword behaves similarly to the variable hoisting.console.log(expressionFunc1); // undefined
expressionFunc1(); // TypeError: expressionFunc1 is not a function
var expressionFunc1 = () => {
console.log("this is an expression function defined using `var`");
}
In this example, due to hoisting, we get undefined
for the console log of expressionFunc1
. When we call the expressionFunc1
function on the next line, it throws an TypeError
because it is not initialized at that time, similar to a variable.
console.log(expressionFunc2); // ReferenceError: Cannot access 'expressionFunc2' before initialization
expressionFunc2(); // execution won't reach here
const expressionFunc2 = () => {
console.log("this is an expression function defined using `const`");
}
In the example above, the first line of the console throws an error due to hoisting, as the variable expressionFunc2
is declared in the Temporal Dead Zone at that point of execution.
Key Takeaways
Hoisting is the declaration of functions, variables & classes at the top of their scope.
Variables declared using
var
keyword are hoisted at the top of their scope and assignedundefined
as the default value.Variables declared using
let
orconst
keyword are declared in the Temporal Dead Zone while hoisting and no value is assigned till their initialization.Regular functions can be used before they are defined due to hoisting.
Function expressions declared using
var
keyword is assignedundefined
while hoisting but can't be called before its definition.Function expressions declared using
let
orconst
keyword is not initialized while hoisting, resulting in the error if used before its declaration.
Subscribe to my newsletter
Read articles from Yugam Gangar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
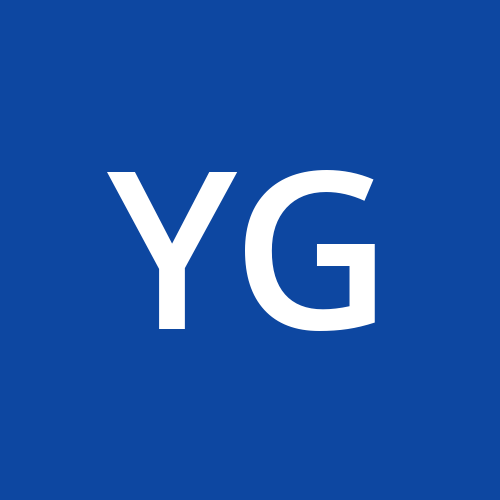
Yugam Gangar
Yugam Gangar
I am another engineer sharpening my technical writing skills and exploring my knowledge through this platform.