Wrapper classes

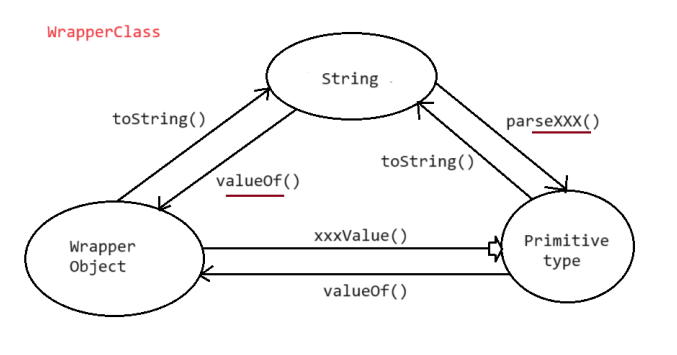
Java has two categories of data types: primitive and reference. Primitive types are the basic types that store values directly in memory, such as int, char, boolean, and so on. Reference types are the types that store references to objects in memory, such as String, Integer, Boolean, and so on. Objects are instances of classes that have attributes and methods. Reference types are also known as wrapper classes, because they wrap primitive values in objects.
Wrapper class and its associated constructor
Byte => byte and String
Short => short and String
Integer => int and String
Long => long and String
Float => float ,String and double
Double => double and String
Character=> character
Boolean => boolean and String
Wrapper class utiltiy methods
1. valueOf() method
To create a wrapper object from primitive type or String we use valueOf(). It is alternative to constructor of Wrapper class, not suggestable to use. Every Wrapper class, except character class contain static valueOf() to create a Wrapper Object. eg#1.
Integer i=Integer.valueOf("10");
Double d=Double.valueOf("10.5");
Boolean b=Boolean.valueOf("anjan");
System.out.println(i);
System.out.println(d);
System.out.println(b);
2. XXXValue() method
We can use xxxValue() to get primitive type for the given Wrapper Object. These methods are a part of every Number type Object. (Byte,Short,Integer,Long,Float,Double) all these classes have these 6 methods which is Written as shown below.
Methods
public byte byteValue();
public short shortValue();
public int intValue();
public long longValue();
public float floatValue();
public double doubleValue();
eg#1.
Integer i=new Integer(130);
System.out.println(i.byteValue());//-126
System.out.println(i.shortValue());//130
System.out.println(i.intValue());//130
System.out.println(i.longValue());//130
System.out.println(i.floatValue());//130.0
System.out.println(i.doubleValue());//130.0
3. parseXxx() method
We use parseXXXX() to convert String object into primitive type.
form-1
public static primitive parseXXX(String s)
Every wrapper class,except Character class has parseXXX() to convert String into primitive type.
eg:
int i=Integer.parseInt("10");
double d =Double.parseInt("10.5");
boolean b=Boolean.parseBoolean("true");
form-2
public static primitive parseXXXX(String s, int radix) ;
|=> range is from 2 to 36
Every Integral type Wrapper class(Byte,Short,Integer,Long) contains the following parseXXXX() to convert Specified radix String to primitive type.
eg:
int i=Integer.parseInt("1111",2);
System.out.println(i);//15
4. toString() method
To convert the Wrapper Object or primitive to String. Every Wrapper class contain toString().
eg:
String s=Integer.toString(10);
String s=Boolean.toString(true);
String s=Character.toString('a');
Subscribe to my newsletter
Read articles from Anjan kumar Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anjan kumar Sharma
Anjan kumar Sharma
I am Java developer. I am seeking microservices in java . Life is always about creating and exploring yourself.