Learn the Factory Pattern: Easy Implementation Tips
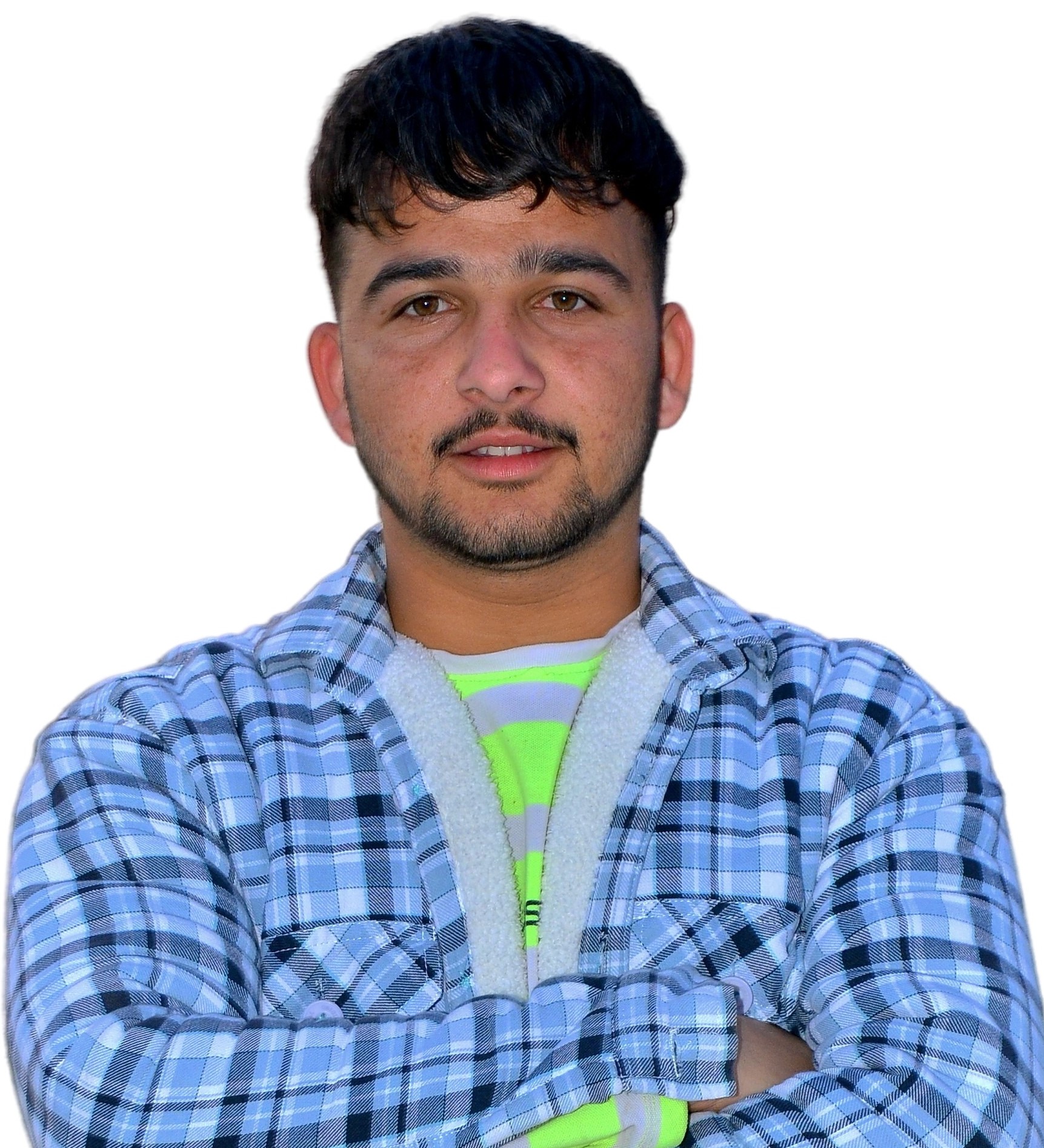
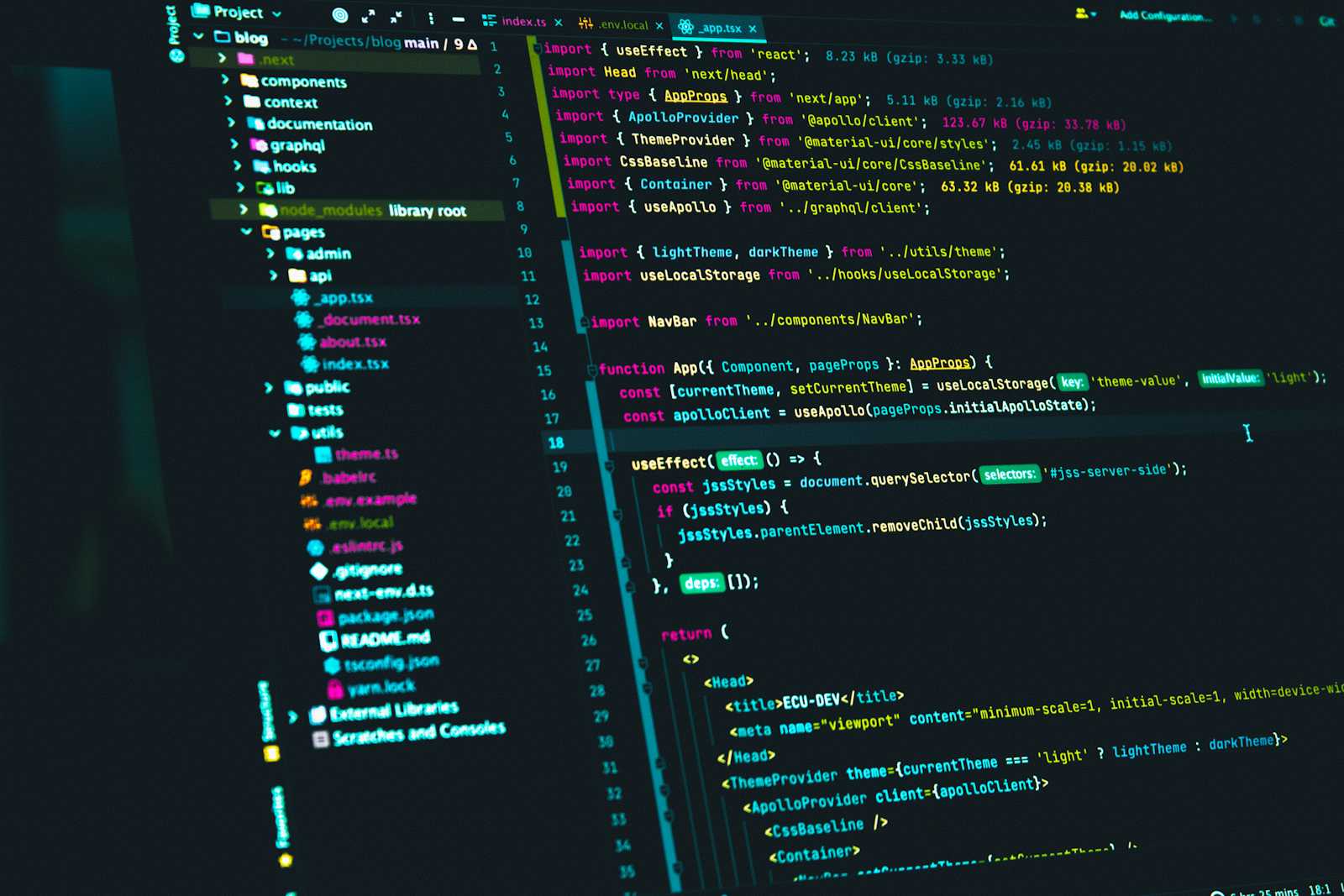
Design patterns are an essential part of software development. They provide a tried-and-tested way of solving common problems, making our code more flexible, reusable, and easier to maintain. One such powerful design pattern is the Factory Pattern. Recently, I had the opportunity to learn and implement this pattern, and I'd love to share my insights with you.
What is a Factory Pattern?
The Factory Pattern is a creational design pattern that provides an interface for creating objects in a superclass but allows subclasses to alter the type of objects that will be made. In simple terms, it delegates the responsibility of object instantiation to subclasses.
Why use the Factory Pattern?
Encapsulation:- The Factory Pattern promotes encapsulation by hiding the object creation logic from the client. The client only interacts with the factory to get the desired object.
Flexibility and Scalability:- This pattern provides flexibility by allowing subclasses to determine which class to instantiate. It is particularly useful when the exact type of object isn't known until runtime.
Code Reusability:- By using the factory pattern, we can reuse code across different parts of the application, reducing redundancy and making the codebase more manageable.
Implementing the Factory Pattern
Let's dive into an example to understand how to implement the Factory Pattern. Suppose we have a simple application that needs to create different types of notifications (e.g., Email, SMS, and Push notifications).
Step1: Define a Notification Interface
First, we create a Notification
interface that defines a common method for all notification types.
// Notification.js
export default class Notification {
send() {
throw new Error("Method 'send()' must be implemented.");
}
}
Step2: Create a Concrete Notification Classes
Next, we create concrete classes that implement the Notification
interface.
// EmailNotification.js
import Notification from './Notification.js';
export default class EmailNotification extends Notification {
send() {
console.log('Sending Email Notification');
}
}
// SMSNotification.js
import Notification from './Notification.js';
export default class SMSNotification extends Notification {
send() {
console.log('Sending SMS Notification');
}
}
// PushNotification.js
import Notification from './Notification.js';
export default class PushNotification extends Notification {
send() {
console.log('Sending Push Notification');
}
}
Step3: Create a Notification Factory
Now, we create a factory class that will return the appropriate notification object based on the input.
// NotificationFactory.js
import EmailNotification from './EmailNotification.js';
import SMSNotification from './SMSNotification.js';
import PushNotification from './PushNotification.js';
export default class NotificationFactory {
static createNotification(type) {
switch (type) {
case 'email':
return new EmailNotification();
case 'sms':
return new SMSNotification();
case 'push':
return new PushNotification();
default:
throw new Error('Invalid notification type');
}
}
}
Step 4: Using the Factory to Create Notifications
Finally, we use the NotificationFactory
to create and send notifications.
// main.js
import NotificationFactory from './NotificationFactory.js';
const emailNotification = NotificationFactory.createNotification('email');
emailNotification.send(); // Output: Sending Email Notification
const smsNotification = NotificationFactory.createNotification('sms');
smsNotification.send(); // Output: Sending SMS Notification
const pushNotification = NotificationFactory.createNotification('push');
pushNotification.send(); // Output: Sending Push Notification
Conclusion
The Factory Pattern is a powerful design pattern that helps in creating objects in a flexible and scalable way. By encapsulating the object creation logic, it makes the code more maintainable and easier to extend.
Learning and implementing the Factory Pattern has been a rewarding experience. It not only enhances the code quality but also prepares us to tackle more complex design scenarios in the future.
Happy coding!
Subscribe to my newsletter
Read articles from Sunil Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
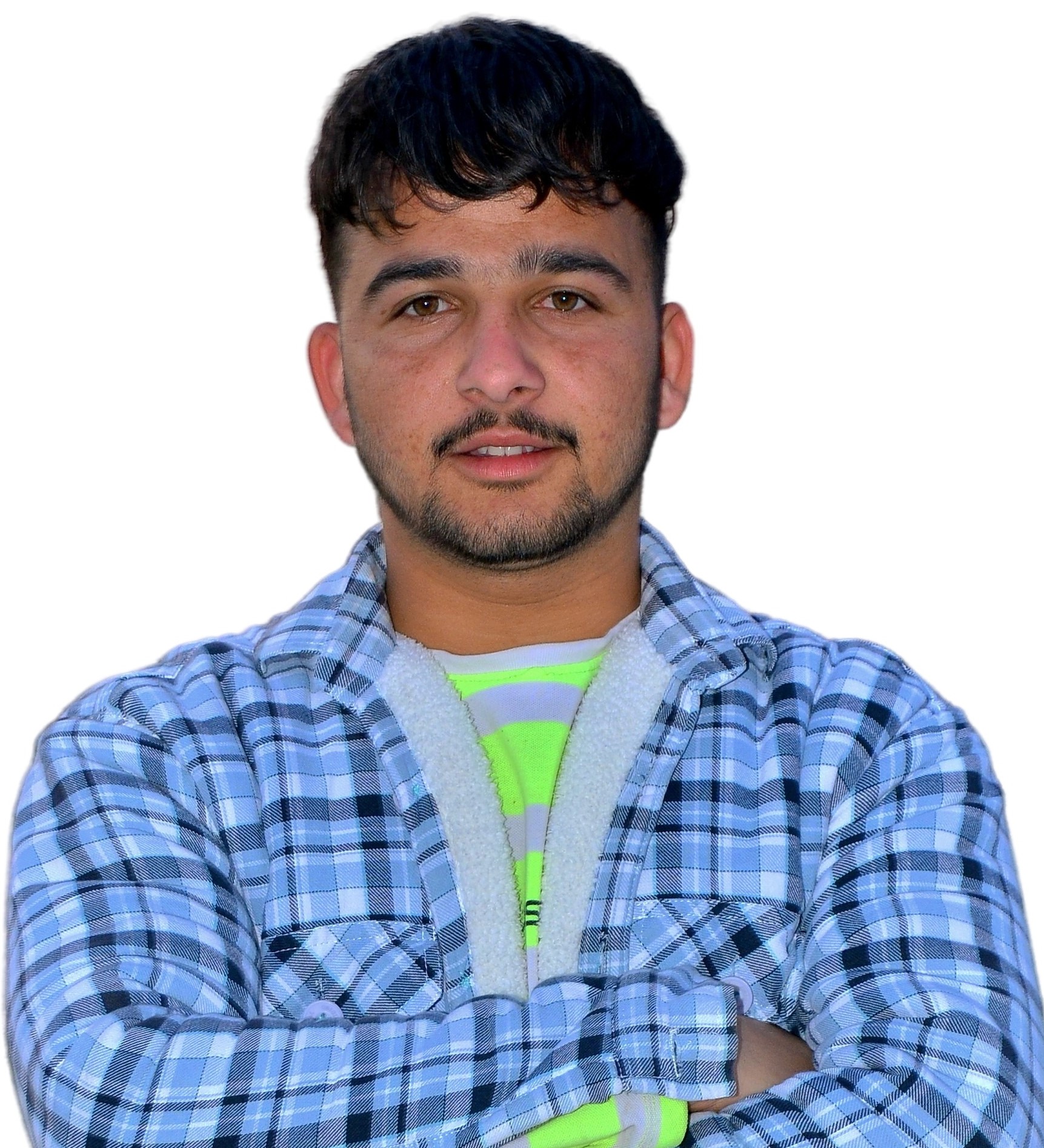
Sunil Kumar
Sunil Kumar
Teaching myself how to code