How does React compile code?
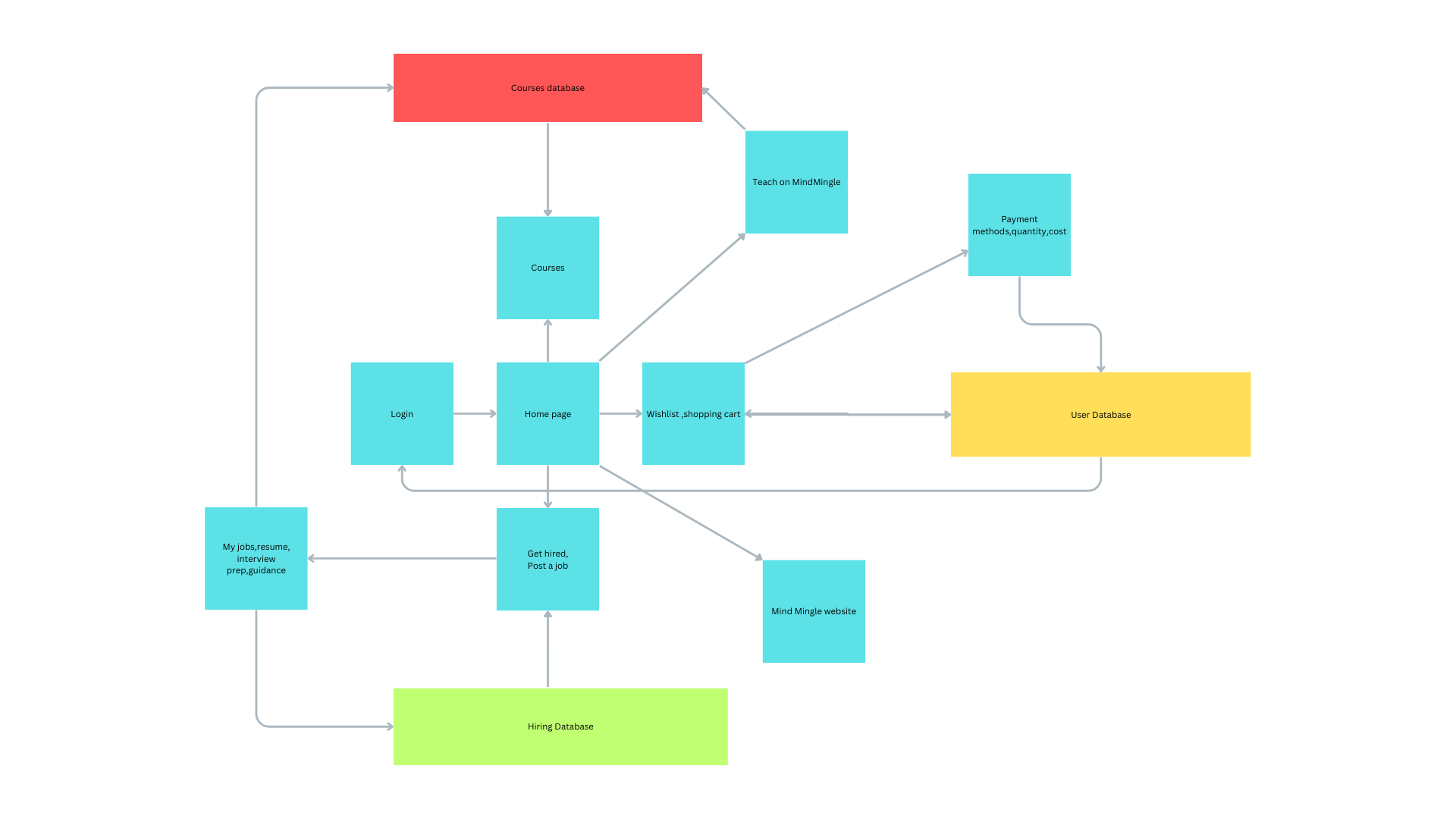
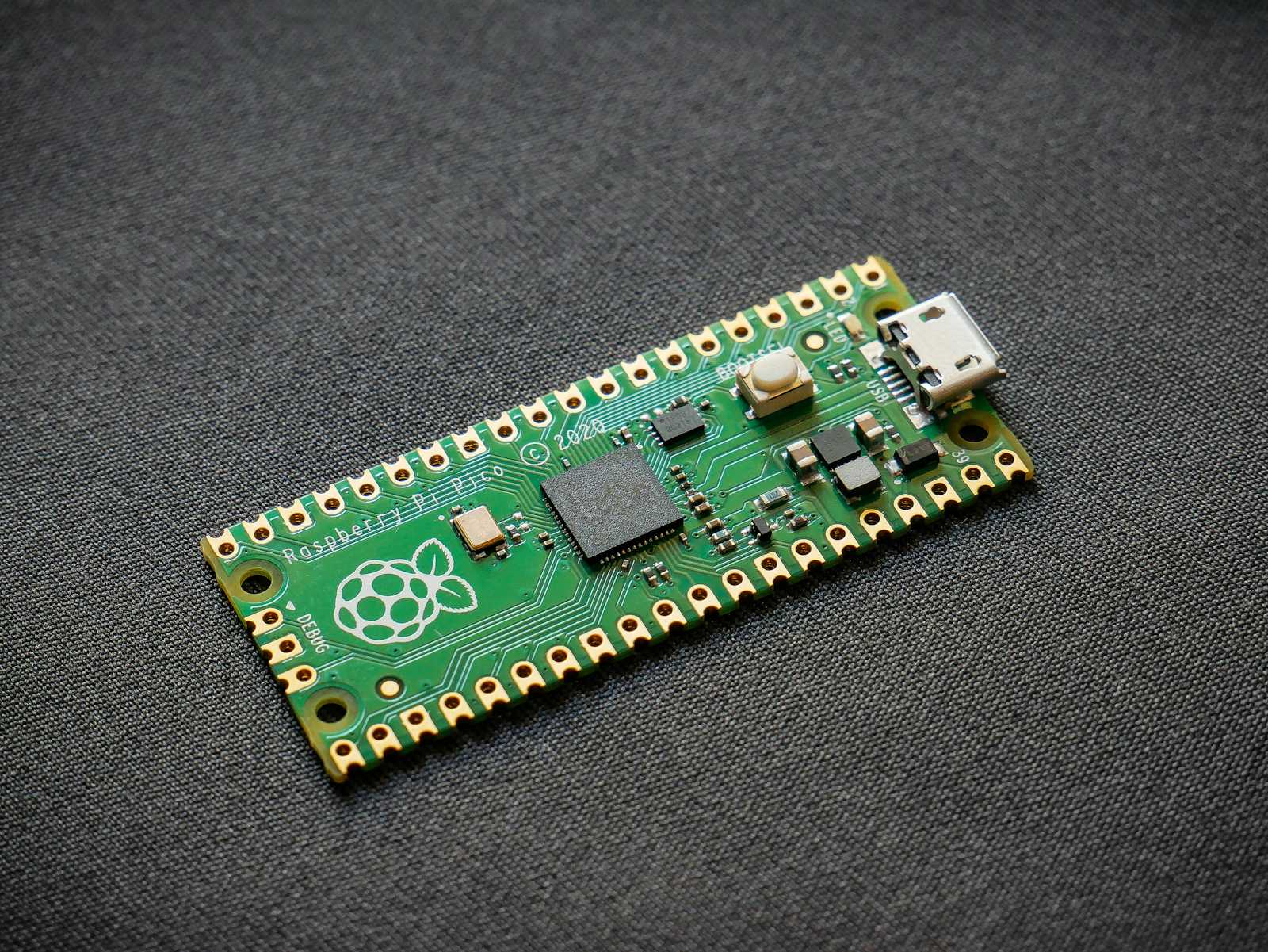
How Does React Compile Its Code?
Introduction
React is a popular JavaScript library for building user interfaces, especially single-page applications. One of the key reasons for its popularity is its simplicity and performance. But have you ever wondered how React code gets transformed from the code you write into something that browsers can understand? In this post, we'll explore how React compiles its code.
The Basics of React Compilation
When we talk about compiling in the context of React, we're referring to the process of transforming JSX (JavaScript XML) and modern JavaScript syntax into code that browsers can execute. This transformation is done by tools like Babel and Webpack.
JSX: The Syntax Extension
JSX is a syntax extension for JavaScript that looks similar to XML or HTML. It allows you to write elements in a way that is intuitive and readable. For example:
const element = <h1>Hello, world!</h1>;
However, browsers don't understand JSX out of the box. This is where the compilation process begins.
Babel: The JavaScript Compiler
Babel is a popular JavaScript compiler that converts JSX and ES6+ (modern JavaScript) code into ES5 (older JavaScript) code that browsers can understand. Here's a step-by-step breakdown of how Babel works:
Parsing: Babel parses the code into an Abstract Syntax Tree (AST). An AST is a tree representation of the code structure.
Transforming: Babel traverses the AST and transforms it according to specified plugins. For example, the JSX plugin transforms JSX syntax into
React.createElement
calls.Generating: Babel generates the transformed code from the modified AST.
For example, Babel transforms this JSX code:
const element = <h1>Hello, world!</h1>;
Into this JavaScript code:
const element = React.createElement('h1', null, 'Hello, world!');
Webpack: The Module Bundler
While Babel handles the syntax transformation, Webpack is responsible for bundling all your modules into a single file (or a few files) that can be served to the browser. Webpack processes your code through a series of loaders and plugins.
Webpack Loaders
Loaders in Webpack are transformations applied to the source code of your modules. For example, the babel-loader
integrates Babel with Webpack, allowing it to process your JavaScript files:
module.exports = {
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: 'babel-loader',
},
],
},
};
Webpack Plugins
Plugins in Webpack extend its capabilities. For example, the HtmlWebpackPlugin
generates an HTML file that includes all your bundled assets:
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
plugins: [
new HtmlWebpackPlugin({
template: './src/index.html',
}),
],
};
The Compilation Process
Here's a high-level overview of the entire compilation process:
Code Writing: You write your React code using JSX and modern JavaScript syntax.
Babel Transformation: Babel transforms your JSX and modern JavaScript code into ES5 syntax.
Webpack Bundling: Webpack bundles all your transformed modules into a single file (or multiple files).
Serving to Browser: The bundled file(s) are served to the browser, which executes the code to render your application.
An Example: Compiling a React App
Let's go through a simple example to see this process in action.
Step 1: Setting Up the Project
First, create a new React project using Create React App (CRA):
npx create-react-app my-app
cd my-app
Step 2: Understanding the Project Structure
CRA sets up a React project with Babel and Webpack pre-configured. The key files include:
src/index.js
: The entry point of your application.src/App.js
: A sample React component.public/index.html
: The HTML template.
Step 3: Writing JSX Code
Open src/App.js
and modify it to include some JSX:
import React from 'react';
function App() {
return (
<div className="App">
<h1>Hello, React!</h1>
</div>
);
}
export default App;
Step 4: Compiling the Code
Run the development server:
npm start
This command starts Webpack's development server, which compiles your code and serves it to http://localhost:3000
. Webpack uses babel-loader
to process your JSX and modern JavaScript syntax.
Step 5: Inspecting the Output
Open the browser's developer tools and inspect the bundled JavaScript. You'll see that the JSX has been transformed into React.createElement
calls, and the code has been bundled into a single file.
Advanced Topics
Code Splitting
Webpack can split your code into multiple bundles, which can be loaded on demand. This improves the performance of your application by reducing the initial load time. React supports code splitting via dynamic import()
and React's React.lazy()
.
Hot Module Replacement
Webpack supports Hot Module Replacement (HMR), which allows you to replace modules in a running application without a full reload. This feature is extremely useful for development, as it enables you to see changes instantly.
Conclusion
React's compilation process, powered by Babel and Webpack, transforms your modern JavaScript and JSX into code that browsers can understand. Understanding this process helps you appreciate the tools that make React development so powerful and efficient. Whether you're a beginner or an experienced developer, knowing how React compiles its code is a valuable piece of knowledge.
Subscribe to my newsletter
Read articles from Rahul Boney directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
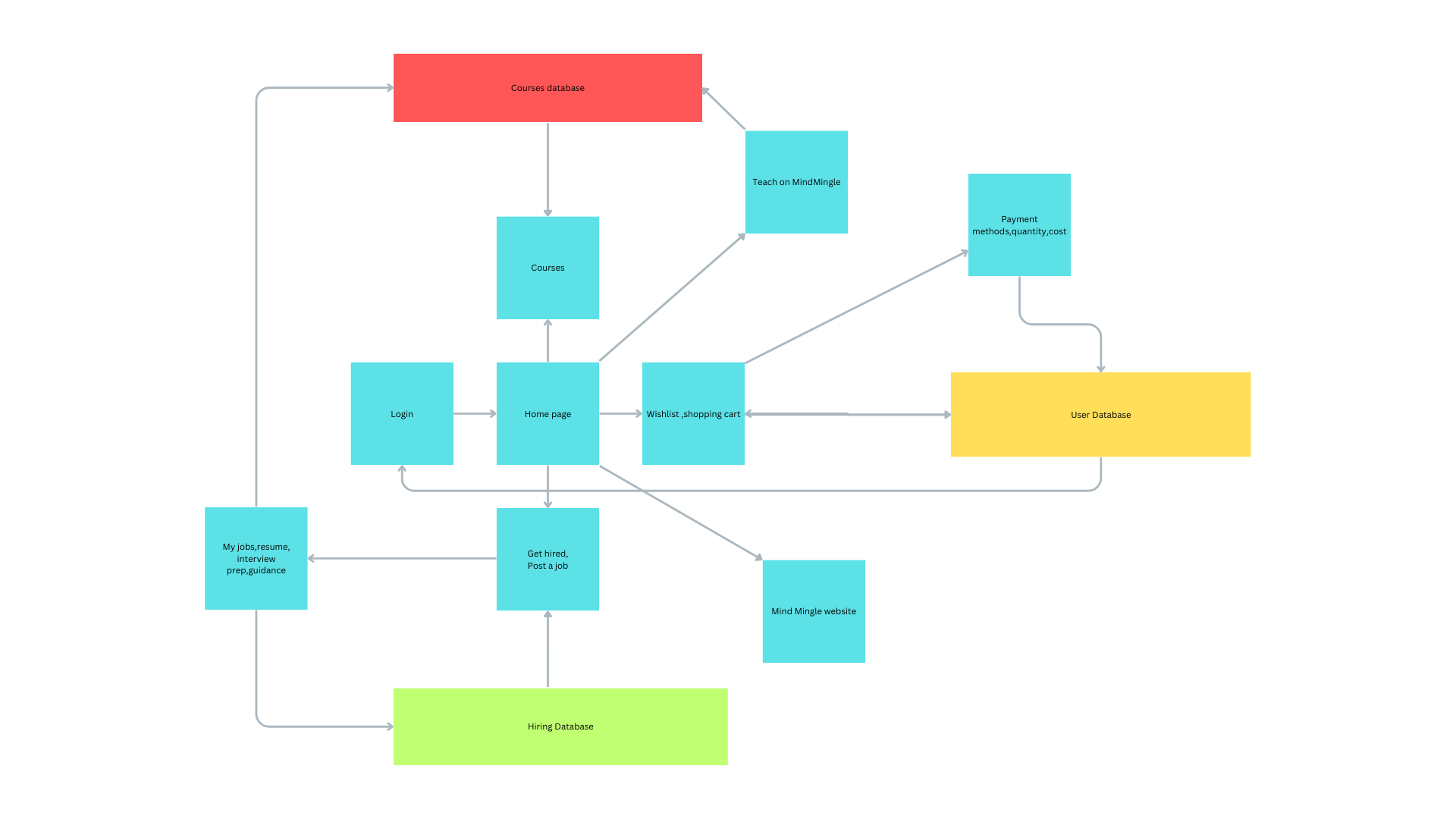
Rahul Boney
Rahul Boney
Hey, I'm Rahul Boney, really into Computer Science and Engineering. I love working on backend development, exploring machine learning, and diving into AI. I am always excited about learning and building new things.