Comprehensive Guide to Docker Concepts: A Step-by-Step Guide for Beginners to Intermediate Users
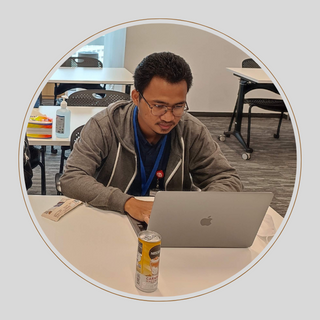
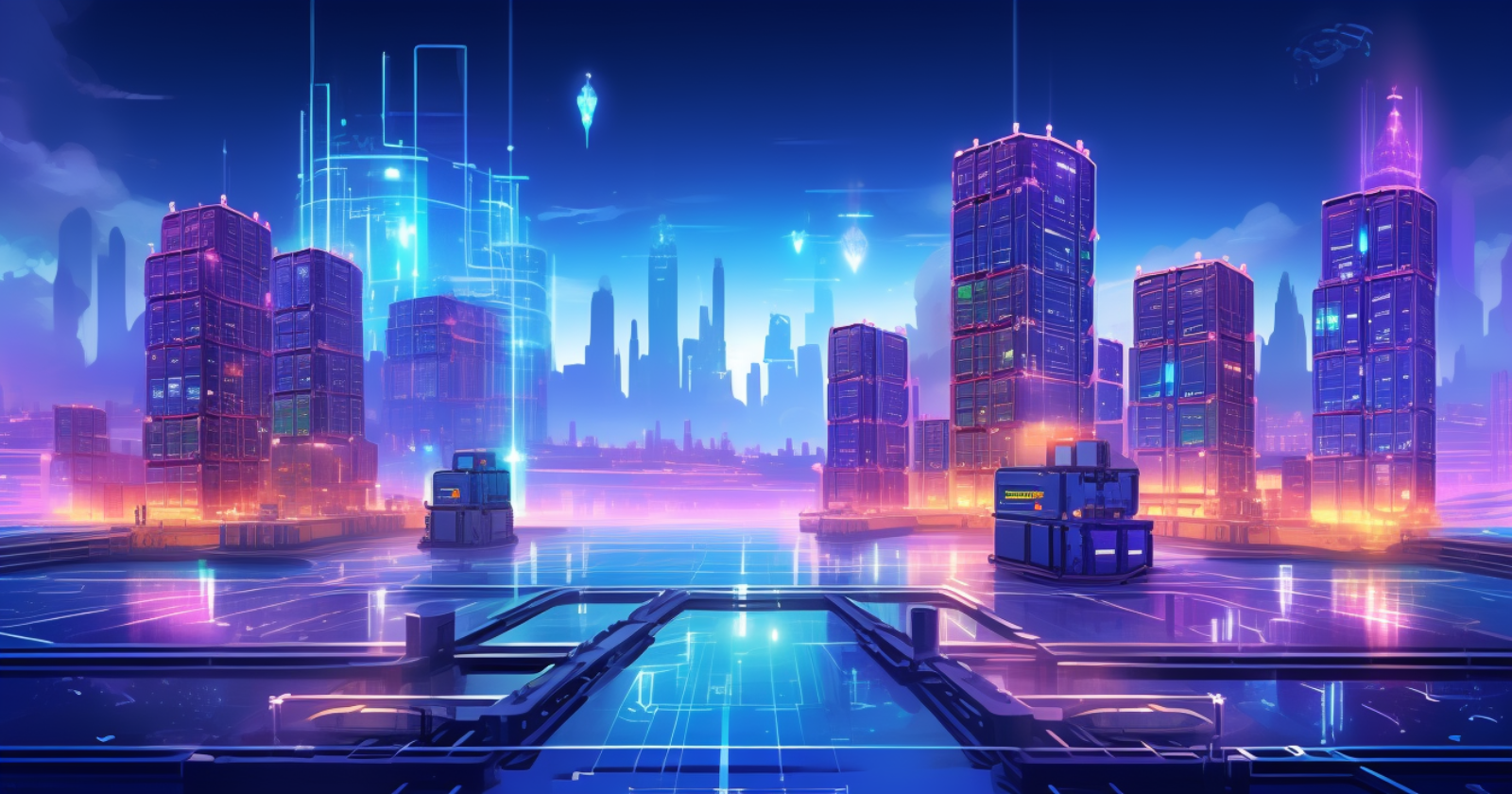
Docker has revolutionized the way we develop, ship, and run applications. It allows developers to package applications into containers—standardized executable components that combine application source code with the operating system libraries and dependencies required to run that code in any environment. This guide will walk you through Docker concepts and provide step-by-step instructions to get you started.
Introduction to Docker
Docker is an open-source platform designed to automate the deployment, scaling, and management of applications within lightweight containers. Containers provide a standardized unit of software, ensuring that an application runs consistently across different computing environments.
Key Docker Concepts
Docker Engine
The Docker Engine is the core component of Docker. It creates and manages Docker containers on the host machine. It consists of three parts:
Server: A long-running daemon process (dockerd).
REST API: To interact with the Docker daemon and instruct it what to do.
CLI: Command-line interface client.
Docker Images
Docker images are read-only templates used to create containers. Images can be built from scratch or derived from existing images.
Docker Containers
Containers are runnable instances of images. They are isolated from each other and the host system, providing a secure and consistent runtime environment.
Docker Registry
A Docker registry is a repository for Docker images. Docker Hub is a public registry that anyone can use, while private registries can be set up for internal use.
Installing Docker
To start using Docker, you need to install Docker Engine. Below are the steps for installing Docker on different operating systems.
Installing Docker on Windows
Download Docker Desktop from the Docker website.
Run the installer and follow the on-screen instructions.
Start Docker Desktop from the Start menu.
Verify installation by opening a command prompt and running:
docker --version
Installing Docker on macOS
Download Docker Desktop from the Docker website.
Open the downloaded .dmg file and drag Docker to Applications.
Run Docker Desktop from the Applications folder.
Verify installation by opening a terminal and running:
docker --version
Installing Docker on Linux (Ubuntu)
Update the apt package index and install packages to allow apt to use a repository over HTTPS:
sudo apt-get update sudo apt-get install apt-transport-https ca-certificates curl gnupg lsb-release
Add Docker’s official GPG key:
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo gpg --dearmor -o /usr/share/keyrings/docker-archive-keyring.gpg
Set up the stable repository:
echo "deb [arch=$(dpkg --print-architecture) signed-by=/usr/share/keyrings/docker-archive-keyring.gpg] https://download.docker.com/linux/ubuntu $(lsb_release -cs) stable" | sudo tee /etc/apt/sources.list.d/docker.list > /dev/null
Install Docker Engine:
sudo apt-get update sudo apt-get install docker-ce docker-ce-cli containerd.io
Verify installation by running:
docker --version
Docker Basics
Starting Docker
Ensure Docker is running on your system. For Docker Desktop on Windows and macOS, it starts automatically when you log in. On Linux, you can start Docker using:
sudo systemctl start docker
Hello World Container
Run your first Docker container using the Hello World image:
docker run hello-world
This command downloads the Hello World image, creates a container, and runs it.
Working with Docker Images
Pulling Images
To download images from Docker Hub:
docker pull <image_name>
Example:
docker pull nginx
Listing Images
To list all downloaded images:
docker images
Building Images
Create a Dockerfile
with the following content:
# Use an official Python runtime as a parent image
FROM python:3.8-slim-buster
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
Build the Docker image:
docker build -t my-python-app .
Managing Docker Containers
Running Containers
To run a container from an image:
docker run -d -p 8080:80 --name mynginx nginx
-d
: Run container in detached mode.-p
: Map host port 8080 to container port 80.--name
: Assign a name to the container.
Listing Containers
To list all running containers:
docker ps
To list all containers (running and stopped):
docker ps -a
Stopping and Removing Containers
To stop a running container:
docker stop <container_id>
To remove a container:
docker rm <container_id>
Docker Networking
Default Network
Docker provides a default bridge network. Containers on the same bridge network can communicate using their container names.
Creating a Custom Network
Create a custom bridge network:
docker network create mynetwork
Run containers on the custom network:
docker run -d --name mynginx --network mynetwork nginx
Docker Volumes
Creating Volumes
Create a volume to persist data:
docker volume create myvolume
Using Volumes
Mount the volume in a container:
docker run -d -p 8080:80 --name mynginx -v myvolume:/usr/share/nginx/html nginx
Docker Compose
Docker Compose allows you to define and manage multi-container Docker applications.
Creating a docker-compose.yml
Create a file named docker-compose.yml
:
version: '3.8'
services:
web:
image: nginx
ports:
- "8080:80"
volumes:
- ./html:/usr/share/nginx/html
app:
image: my-python-app
build:
context: .
ports:
- "5000:5000"
volumes:
- .:/app
Running Docker Compose
Start the application:
docker-compose up -d
Stop the application:
docker-compose down
Best Practices
Keep Images Small
Use minimal base images and clean up unnecessary files to keep images small.
Use Multi-Stage Builds
Multi-stage builds reduce the size of the final image by using multiple FROM
statements in a single Dockerfile.
Secure Your Containers
Run containers with the least privileges required and keep your Docker daemon secure.
Monitor and Log
Use monitoring tools like Prometheus and logging tools like ELK stack to monitor and log container activities.
Conclusion
Docker simplifies application deployment and scaling by containerizing applications. By following the best practices and understanding key Docker concepts, you can efficiently manage your applications and ensure smooth operations. Happy containerizing!
Subscribe to my newsletter
Read articles from Anaz S. Aji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
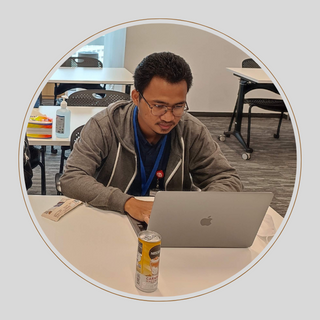
Anaz S. Aji
Anaz S. Aji
I am a dedicated Mobile Developer with a strong passion for exploring the latest advancements in technology and the dynamic world of cryptocurrency. My curiosity and enthusiasm drive me to continuously seek out and experiment with innovative solutions, ensuring that I stay at the forefront of industry trends and developments.