Cross-Platform Rest and WebSockets Client Application: Setup
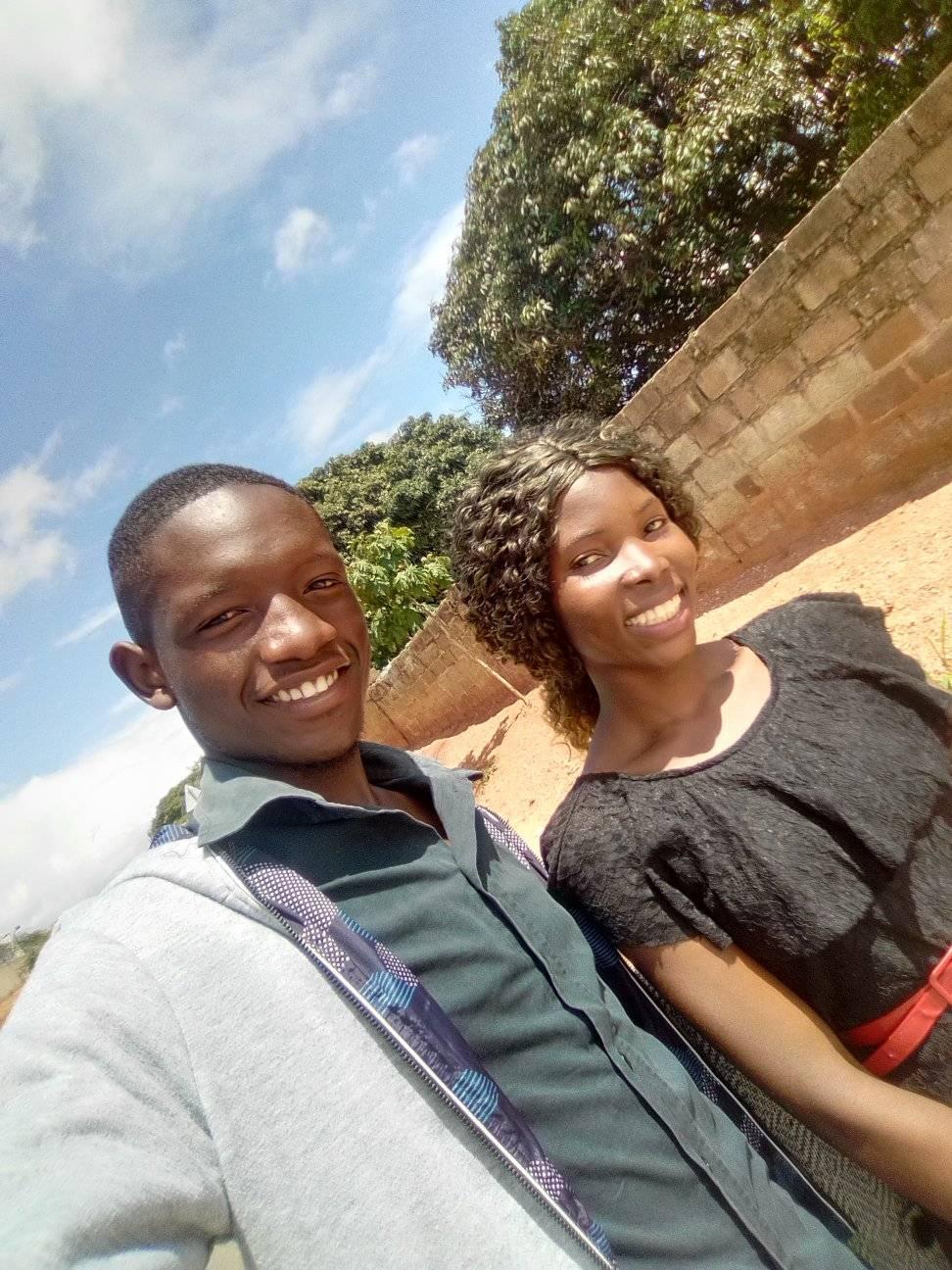
Table of contents
Introduction
There are a lot of frameworks we can use to write this application. The choice of the programming language to use depends on what framework we decide is best suited to build the application.
With flutter we can have the same code-base compiled for all the platforms and it also easily gives us the access to the native code in case there is a native functionality we would like to implement.
Initialization
We create a new flutter application using the command below.
flutter create --org com.fshangala.apps restapi_websockets_client && cd restapi_websockets_client
Our application requires some way of navigating across screens. The default way will work just fine, but it is going to be difficult for us to extract route parameters when we build for the web. The suitable solution to this problem is using go_router.
We install go_router using the command below.
flutter pub add go_router
We create a minimal implementation of the home screen at lib/screens/home_screen.dart
import 'package:flutter/material.dart';
class HomeScreen extends StatefulWidget {
const HomeScreen({super.key});
@override
State<StatefulWidget> createState() {
return HomeScreenState();
}
}
class HomeScreenState extends State<HomeScreen> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text("RestAPI WebSockets Client"),
),
);
}
}
We configure the router and add HomeScreen
as the default route.
import 'package:flutter/material.dart';
import 'package:go_router/go_router.dart';
import 'package:restapi_websockets_client/screens/home_screen.dart';
void main() {
runApp(const MyApp());
}
// GoRouter configuration
final _router = GoRouter(
routes: [
GoRoute(
path: '/',
builder: (context, state) => const HomeScreen(),
),
],
);
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp.router(
routerConfig: _router,
title: "RestAPI WebSockets Client",
debugShowCheckedModeBanner: false,
);
}
}
This is what we have so far.
Subscribe to my newsletter
Read articles from Funduluka Shangala directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
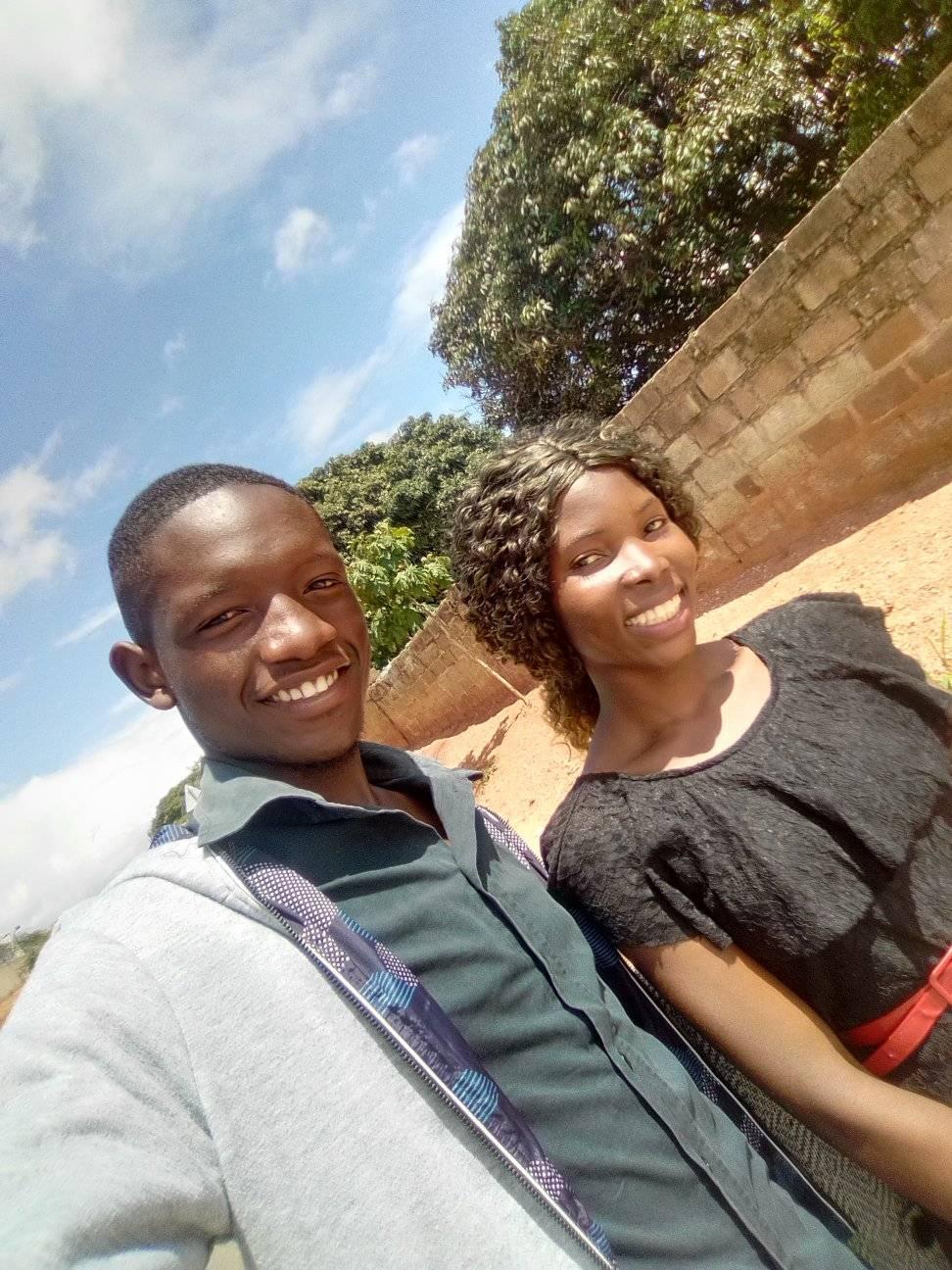
Funduluka Shangala
Funduluka Shangala
I am a full stack software developer.