"React Essentials: A Comprehensive Guide to Core Concepts for Interview and Job Readiness"
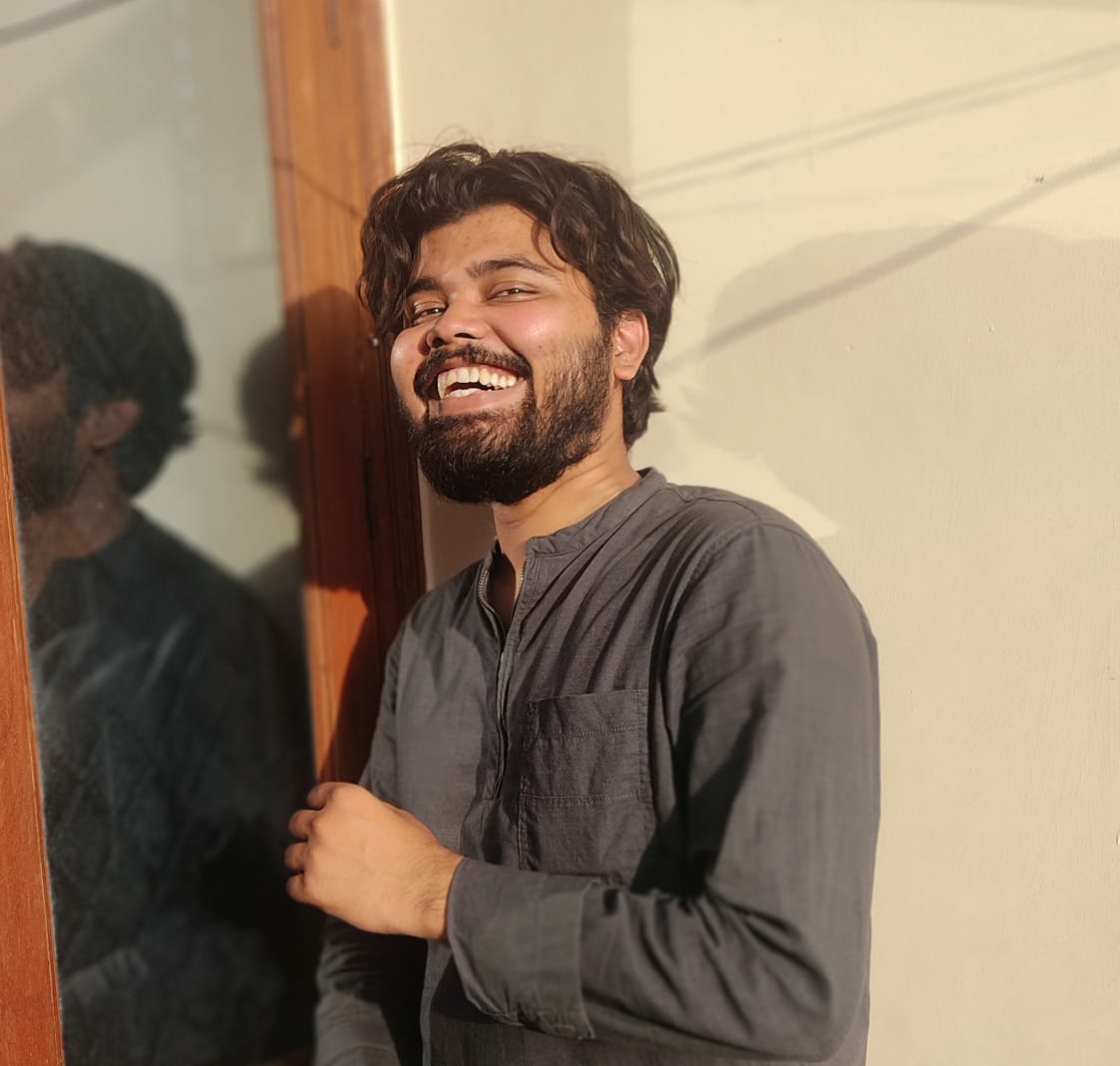
Table of contents
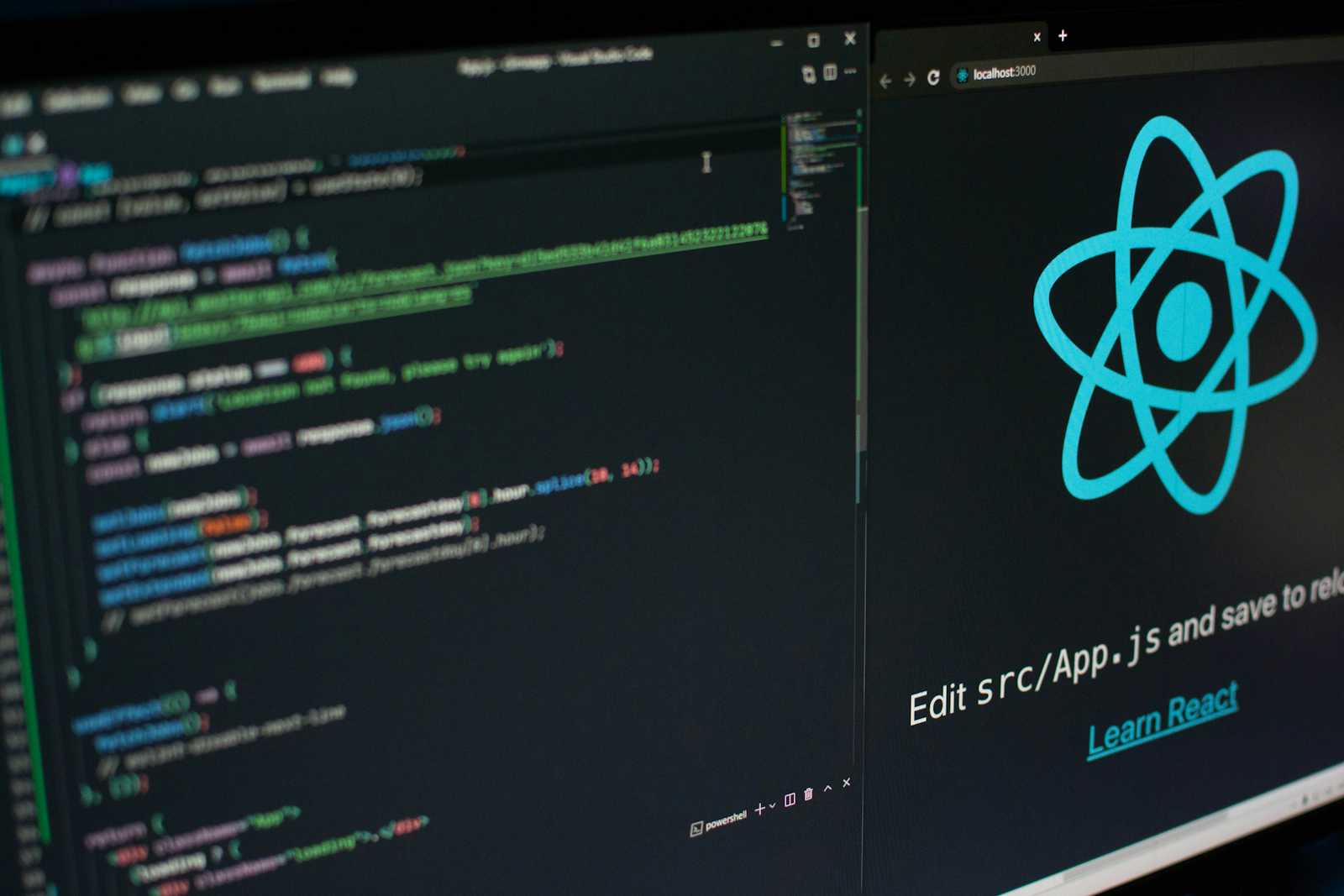
- JSX Syntax and Usage
Theory: JSX is a syntax extension for JavaScript that allows you to write HTML-like code in your JavaScript files. It makes it easier to describe what the UI should look like and is compiled to regular JavaScript at runtime.
const name = 'John Doe';
const element = (
<div>
<h1>Hello, {name}</h1>
<p>Welcome to React!</p>
</div>
);
ReactDOM.render(element, document.getElementById('root'));
- Components: Function Components and Class Components
Theory: React components are the building blocks of React applications. They can be defined as functions or classes. Function components are simpler and are preferred in modern React development, especially with the introduction of Hooks.
// Function Component
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
// Class Component
class Welcome extends React.Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
// Usage
function App() {
return (
<div>
<Welcome name="Alice" />
<Welcome name="Bob" />
</div>
);
}
- Props: Passing and Using Props
Theory: Props (short for properties) are a way of passing data from parent to child components. They are read-only and help make your components reusable.
function User(props) {
return (
<div>
<h1>{props.name}</h1>
<p>Age: {props.age}</p>
</div>
);
}
function App() {
return (
<div>
<User name="Alice" age={30} />
<User name="Bob" age={25} />
</div>
);
}
- State: Managing Component State
Theory: State is a JavaScript object that stores dynamic data in a component. When state changes, React re-renders the component.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
- Lifecycle Methods (for Class Components)
Theory: Lifecycle methods are special methods that automatically get called as your component gets rendered and updated. They give you control over what happens when each tiny section of your UI renders, updates, thinks about re-rendering and then disappears entirely.
class Clock extends React.Component {
constructor(props) {
super(props);
this.state = {date: new Date()};
}
componentDidMount() {
this.timerID = setInterval(
() => this.tick(),
1000
);
}
componentWillUnmount() {
clearInterval(this.timerID);
}
tick() {
this.setState({
date: new Date()
});
}
render() {
return (
<div>
<h2>It is {this.state.date.toLocaleTimeString()}.</h2>
</div>
);
}
}
- Hooks: useState, useEffect, useContext, useRef
Theory: Hooks are functions that let you "hook into" React state and lifecycle features from function components. They don't work inside classes — they let you use React without classes.
import React, { useState, useEffect, useContext, useRef } from 'react';
// useState
function Example() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
// useEffect
function Example() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
});
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
// useContext
const ThemeContext = React.createContext('light');
function ThemedButton() {
const theme = useContext(ThemeContext);
return <button theme={theme}>I am styled by theme context!</button>;
}
// useRef
function TextInputWithFocusButton() {
const inputEl = useRef(null);
const onButtonClick = () => {
inputEl.current.focus();
};
return (
<>
<input ref={inputEl} type="text" />
<button onClick={onButtonClick}>Focus the input</button>
</>
);
PIKLP
- Event Handling in React
Theory: React events are named using camelCase and passed as functions rather than strings. React wraps the browser's native event and provides a consistent interface across different browsers.
function ActionLink() {
function handleClick(e) {
e.preventDefault();
console.log('The link was clicked.');
}
return (
<a href="#" onClick={handleClick}>
Click me
</a>
);
}
class Toggle extends React.Component {
constructor(props) {
super(props);
this.state = {isToggleOn: true};
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
this.setState(prevState => ({
isToggleOn: !prevState.isToggleOn
}));
}
render() {
return (
<button onClick={this.handleClick}>
{this.state.isToggleOn ? 'ON' : 'OFF'}
</button>
);
}
}
- Conditional Rendering
Theory: In React, you can create distinct components that encapsulate behavior you need. Then, you can render only some of them, depending on the state of your application.
function UserGreeting(props) {
return <h1>Welcome back!</h1>;
}
function GuestGreeting(props) {
return <h1>Please sign up.</h1>;
}
function Greeting(props) {
const isLoggedIn = props.isLoggedIn;
if (isLoggedIn) {
return <UserGreeting />;
}
return <GuestGreeting />;
}
// Usage
ReactDOM.render(
<Greeting isLoggedIn={false} />,
document.getElementById('root')
);
- Lists and Keys
Theory: When rendering multiple components from an array, keys help React identify which items have changed, been added, or been removed. Keys should be given to the elements inside the array to give the elements a stable identity.
function ListItem(props) {
return <li>{props.value}</li>;
}
function NumberList(props) {
const numbers = props.numbers;
const listItems = numbers.map((number) =>
<ListItem key={number.toString()} value={number} />
);
return (
<ul>
{listItems}
</ul>
);
}
const numbers = [1, 2, 3, 4, 5];
ReactDOM.render(
<NumberList numbers={numbers} />,
document.getElementById('root')
);
- Forms and Controlled Components
Theory: In HTML, form elements typically maintain their own state. In React, mutable state is typically kept in the state property of components, and only updated with setState(). A form element whose value is controlled by React in this way is called a "controlled component".
class NameForm extends React.Component {
constructor(props) {
super(props);
this.state = {value: ''};
this.handleChange = this.handleChange.bind(this);
this.handleSubmit = this.handleSubmit.bind(this);
}
handleChange(event) {
this.setState({value: event.target.value});
}
handleSubmit(event) {
alert('A name was submitted: ' + this.state.value);
event.preventDefault();
}
render() {
return (
<form onSubmit={this.handleSubmit}>
<label>
Name:
<input type="text" value={this.state.value} onChange={this.handleChange} />
</label>
<input type="submit" value="Submit" />
</form>
);
}
}
- Lifting State Up
Theory: Often, several components need to reflect the same changing data. We recommend lifting the shared state up to their closest common ancestor. This is called "lifting state up".
function BoilingVerdict(props) {
if (props.celsius >= 100) {
return <p>The water would boil.</p>;
}
return <p>The water would not boil.</p>;
}
class Calculator extends React.Component {
constructor(props) {
super(props);
this.handleChange = this.handleChange.bind(this);
this.state = {temperature: ''};
}
handleChange(e) {
this.setState({temperature: e.target.value});
}
render() {
const temperature = this.state.temperature;
return (
<fieldset>
<legend>Enter temperature in Celsius:</legend>
<input
value={temperature}
onChange={this.handleChange} />
<BoilingVerdict
celsius={parseFloat(temperature)} />
</fieldset>
);
}
}
- Composition vs Inheritance
Theory: React has a powerful composition model, and we recommend using composition instead of inheritance to reuse code between components.
function FancyBorder(props) {
return (
<div className={'FancyBorder FancyBorder-' + props.color}>
{props.children}
</div>
);
}
function WelcomeDialog() {
return (
<FancyBorder color="blue">
<h1 className="Dialog-title">
Welcome
</h1>
<p className="Dialog-message">
Thank you for visiting our spacecraft!
</p>
</FancyBorder>
);
}
These examples provide both theoretical explanations and practical code implementations for each topic. This approach gives a comprehensive understanding of these React concepts, suitable for interview preparation and real-world application.
Subscribe to my newsletter
Read articles from Tejas Jaiswal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
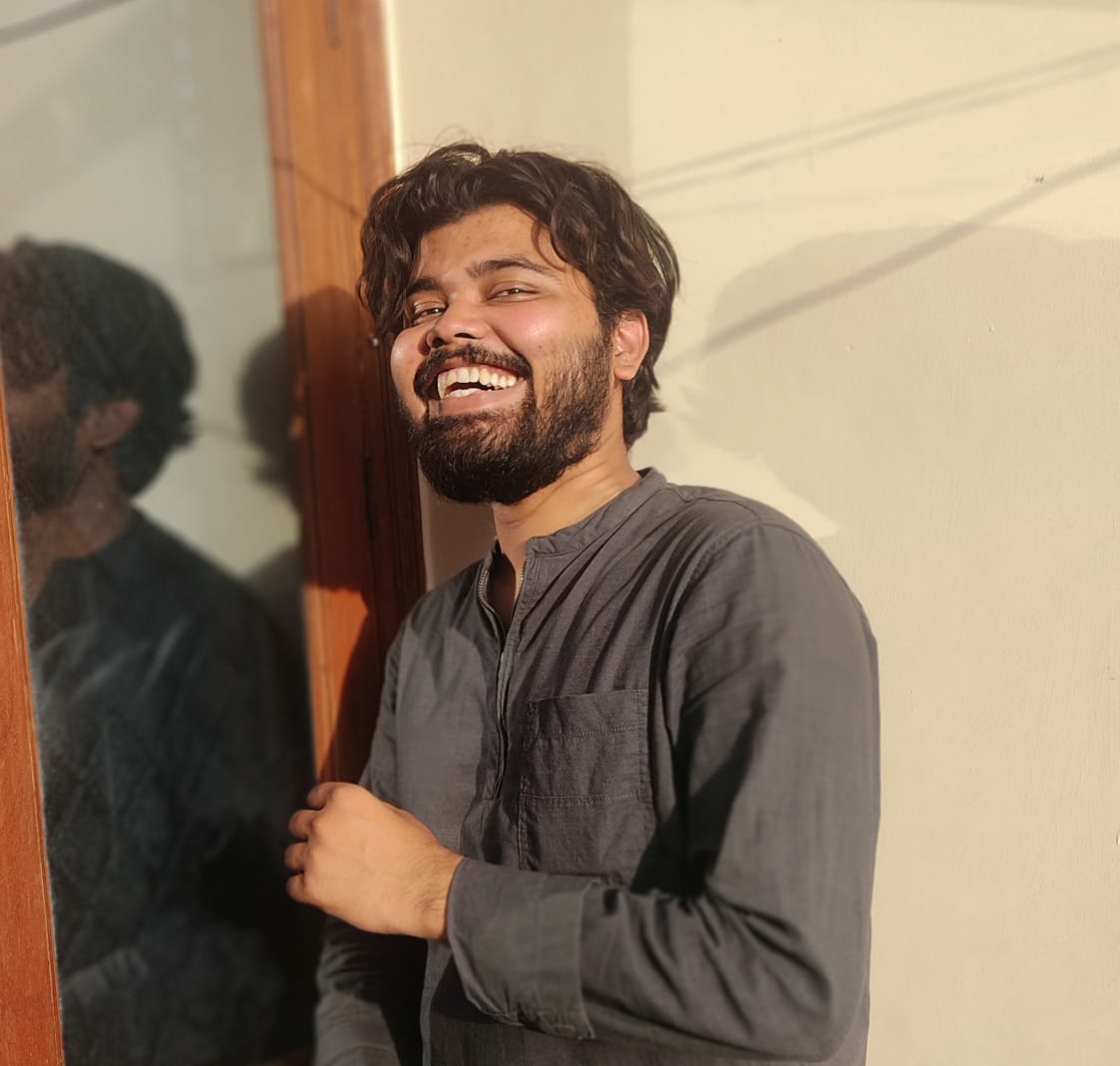
Tejas Jaiswal
Tejas Jaiswal
As a full-stack developer, I enjoy creating visually appealing and responsive websites using the MERN stack, SQL database administration, and HTTP. I have one year of experience, where I worked on various web development projects and learning new technologies and frameworks. I also completed a two-month contract as a full-stack developer at Eviox Technology Pvt Ltd, where I developed and deployed a dynamic e-commerce website for a client. In addition to web development, I am passionate about artificial intelligence and its applications. I am currently pursuing a Bachelor of Technology degree in Electronics and Communications Engineering from JSS Academy of Technical Education, Noida, where I have taken courses on machine learning, computer vision, and natural language processing. I am also a freelance video editor with one year of experience, using software such as Adobe Premiere Pro and DaVinci Resolve to create captivating visual stories. My goal is to combine my web development and AI skills to create innovative and impactful solutions for real-world problems.