Printing a Pyramid shape

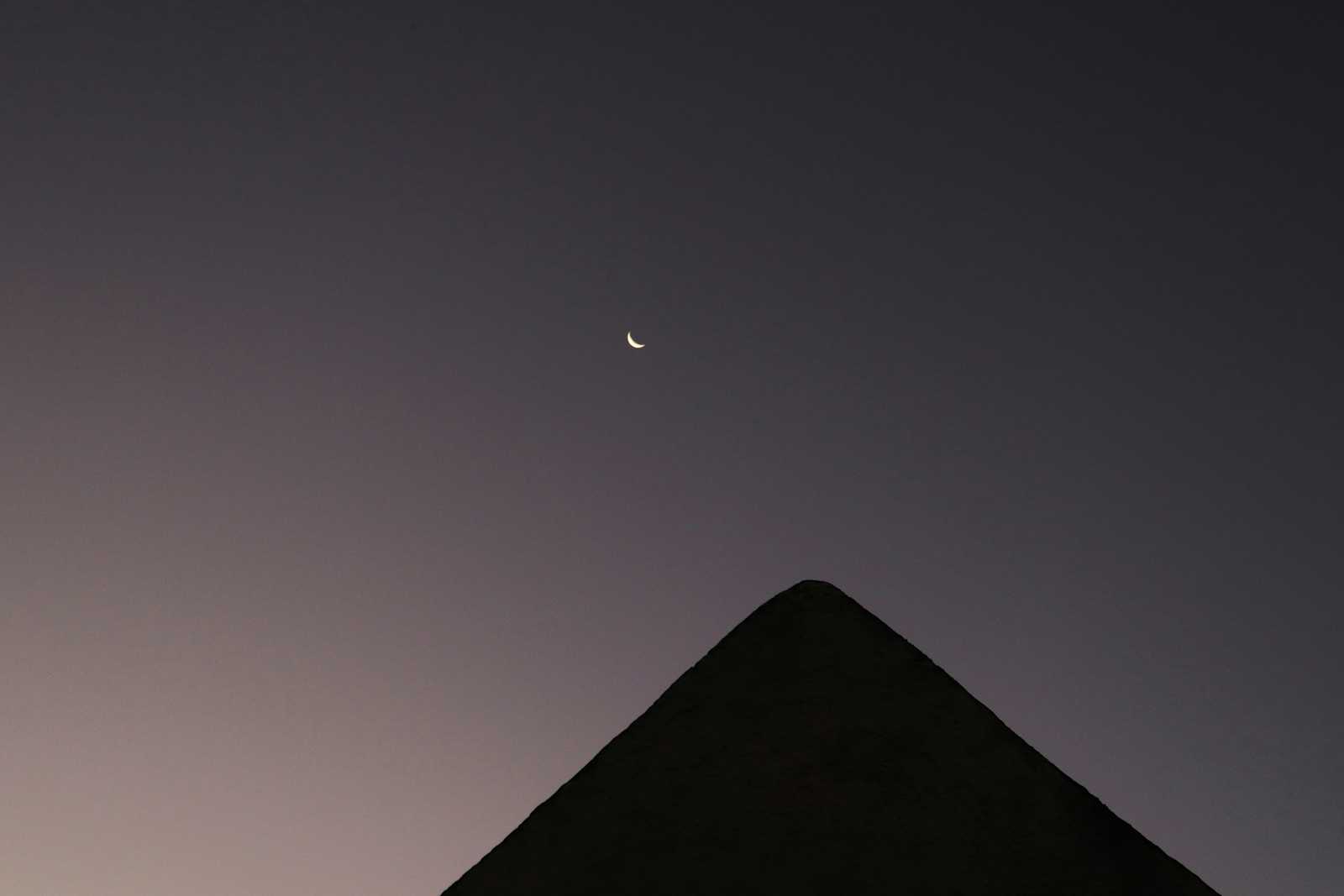
Introduction
This is the third post in the series 'Printing Shapes Using COBOL', and in this post, we will generate a pyramid (or triangle) shape ๐บ.
As always, we'll use the asterisk (*) symbol to form the shape.
Approach
We'll start printing the triangle from the top and go all the way down. We'll print the triangle in the middle of the 80-character line.
PERFORM loop and DISPLAY statements in COBOL will be used to generate the shape.
Triangle shape in COBOL
Here is the COBOL program to print the triangle shape.
IDENTIFICATION DIVISION.
PROGRAM-ID. PYRAMID.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-OUT PIC X(80) VALUE SPACES.
01 WS-N PIC 9(2) VALUE 0.
01 WS-CENTER PIC 9(2) VALUE 40.
PROCEDURE DIVISION.
PERFORM VARYING WS-N FROM 1 BY 2 UNTIL WS-N > 20
MOVE ALL '*' TO WS-OUT(WS-CENTER:WS-N)
COMPUTE WS-CENTER = WS-CENTER - 1
DISPLAY WS-OUT
END-PERFORM.
STOP RUN.
Explanation
In the WORKING-STORAGE SECTION,
WS-OUT is an 80-character Alphanumeric data-item initialized with spaces to ensure each line starts as blank.
WS-N is an Integer data-item to control the number of asterisks to print in each line, starting at 1 and increasing by 2 in each iteration.
WS-CENTER is a data-item to determine the starting position of the asterisks in the line, initialized to 40 to start printing in the middle of the 80-character line.
In the PROCEDURE DIVISION, we have the following instructions to be executed:
The PERFORM loop is set to start WS-N from 1 and increment it by 2 in each iteration until WS-N exceeds 20. This way, the triangle shape will be spread out in 10 lines.
For each value of WS-N, move the appropriate number of asterisks to the variable WS-OUT starting at the position defined by WS-CENTER.
After placing the asterisks, adjust the center position for next iteration by decrementing WS-CENTER by 1 to shift the starting position left for the next line.
Print the current line of WS-OUT.
Output
*
***
*****
*******
*********
***********
*************
***************
*****************
*******************
You can run the program on JDoodle's Online COBOL compiler by clicking ๐ here.
Conclusion
There are multiple ways to create these shapes, and whatever you've read in these posts is just my two cents.
Share your approach to generating these shapes in the comments section below.
Thx!
๐
Subscribe to my newsletter
Read articles from Srinivasan JV directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Srinivasan JV
Srinivasan JV
I have been a mainframe developer for the past 10 years. As part of work obligations, I am currently in pursuit of learning Java. You'll find me writing more about the mainframe.