Understanding Composition API vs Options API in Vue.js: Which One to Choose?
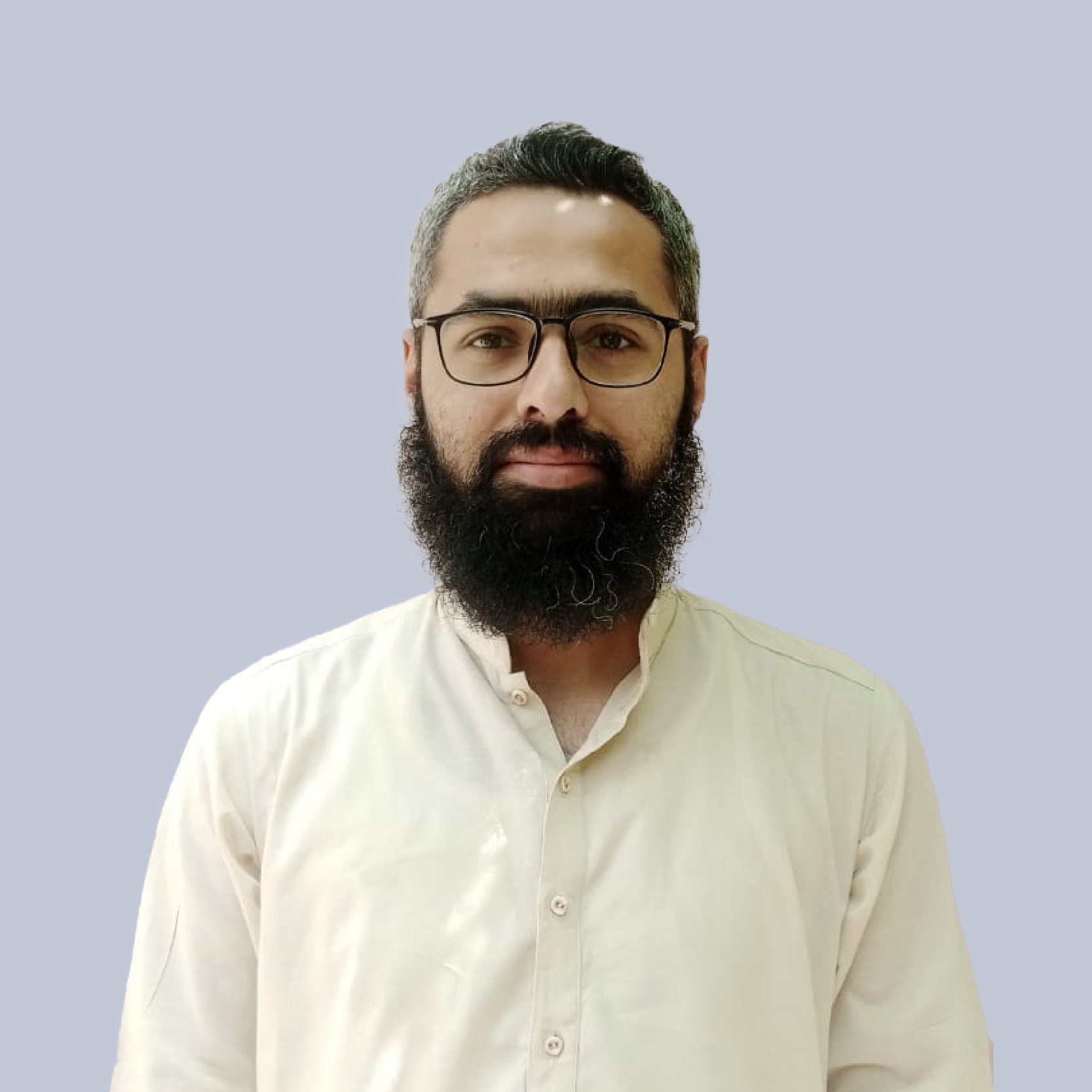
Table of contents
- 1. Introduction to Vue.js APIs
- 2. What is the Options API?
- 3. What is the Composition API?
- 4. Key Differences Between Composition API and Options API
- 5. Pros and Cons of Each API
- 6. When to Use Composition API vs Options API
- 7. Real-World Examples and Use Cases
- 8. Conclusion
- 9. Follow Us on GitHub and LinkedIn
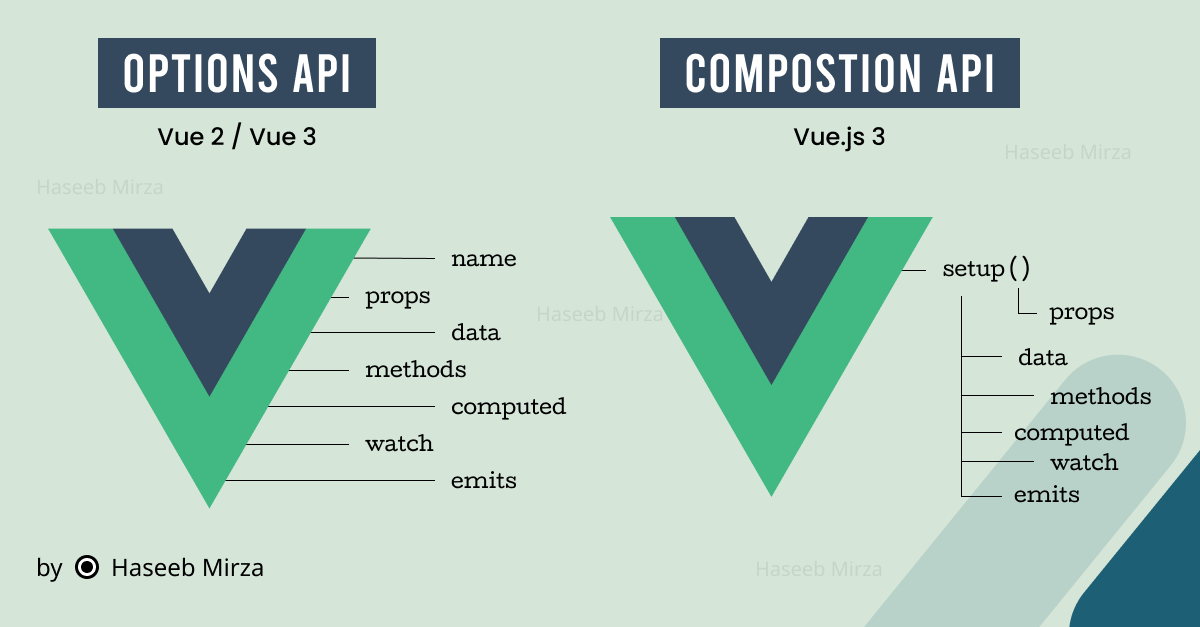
1. Introduction to Vue.js APIs
Vue.js offers two powerful APIs for building components: the Options API and the Composition API. While both serve the same purpose, they offer different approaches to managing your component's logic and state.
2. What is the Options API?
The Options API is the traditional way of defining component logic in Vue.js. It organizes code into different options such as data
, methods
, computed
, and watch
.
3. What is the Composition API?
The Composition API, introduced in Vue 3, provides a more flexible and powerful way to write components by using functions to organize and reuse logic.
4. Key Differences Between Composition API and Options API
Structure and Syntax
Reusability and Composition
TypeScript Support
Learning Curve
5. Pros and Cons of Each API
Options API:
Pros: Simple, intuitive, easy to learn.
Cons: Can become unwieldy in larger components.
Composition API:
Pros: Better logic reusability, improved TypeScript support.
Cons: Steeper learning curve, less intuitive for beginners.
6. When to Use Composition API vs Options API
Options API: Ideal for smaller projects and beginners.
Composition API: Best for larger, more complex applications where logic reuse and better TypeScript support are necessary.
7. Real-World Examples and Use Cases
Example Scenario: A Counter Component
Using Options API:
<template>
<div>
<p>{{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script>
export default {
data() {
return {
count: 0
};
},
methods: {
increment() {
this.count++;
}
}
};
</script>
<style scoped>
button {
padding: 10px;
font-size: 16px;
}
</style>
Using Composition API:
<template>
<div>
<p>{{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const count = ref(0);
const increment = () => {
count.value++;
};
return {
count,
increment
};
}
};
</script>
<style scoped>
button {
padding: 10px;
font-size: 16px;
}
</style>
Explanation
Options API:
The state is defined in the
data
option.Methods are defined in the
methods
option.Template uses
v-bind
andv-on
directives to bind data and events.
Composition API:
State is managed using the
ref
function.Methods are defined within the
setup
function.The
setup
function returns the state and methods, making them available in the template.
Comparison:
The Options API is simpler and more intuitive for beginners.
The Composition API provides better reusability and flexibility, especially in larger and more complex applications.
8. Conclusion
Choosing between the Composition API and Options API depends on your project requirements and familiarity with Vue.js. Both have their own strengths and can be chosen based on the specific needs of your application.
9. Follow Us on GitHub and LinkedIn
If you found this article helpful, follow us on GitHub and LinkedIn for more tips and tutorials!
By understanding the differences and use cases of Composition API and Options API, you can make an informed decision on which to use in your Vue.js projects. Stay tuned for more insights and tutorials, and don’t forget to follow us on GitHub and LinkedIn!
Subscribe to my newsletter
Read articles from Haseeb Mirza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
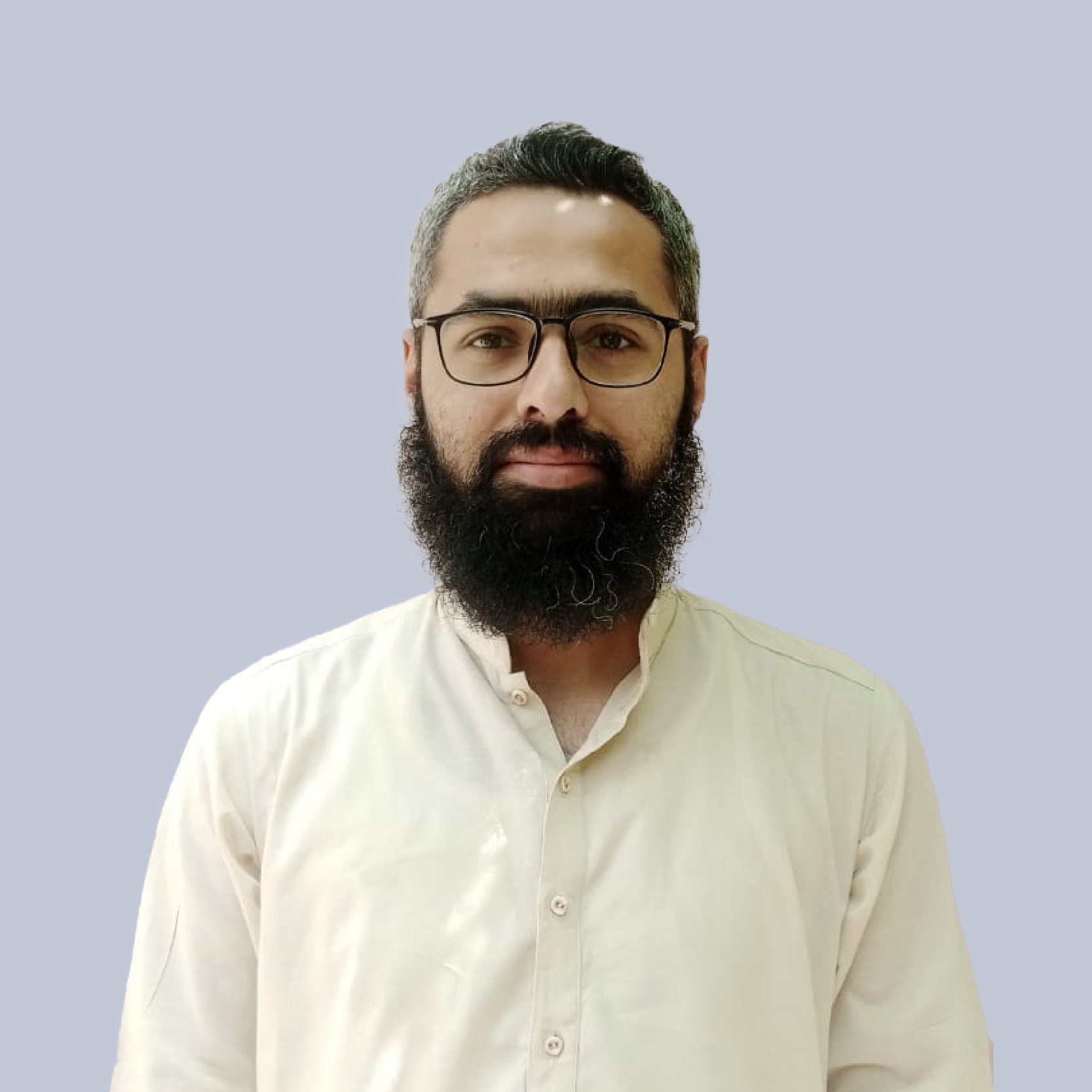
Haseeb Mirza
Haseeb Mirza
Web Dev | Passionate about driving small business success through digital innovation. Let's build, learn, and thrive in the world of bits and bytes! 🚀🖥️