AutoBoxing and Unboxing In Java

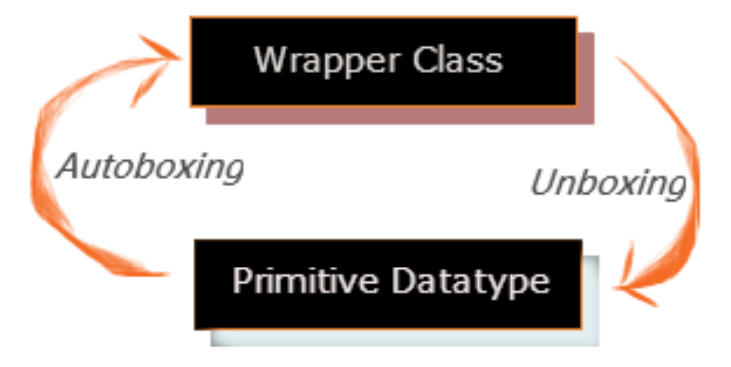
Data types in java
Java has two categories of data types: primitive and reference. Primitive types are the basic types that store values directly in memory, such as int, char, boolean, and so on. Reference types are the types that store references to objects in memory, such as String, Integer, Boolean, and so on. Objects are instances of classes that have attributes and methods.
Autoboxing in java
The automatic conversion of primitive data types into its equivalent Wrapper type is known as autoboxing.Autoboxing is the process of converting a primitive value to a corresponding reference object, such as int to Integer.For example, if you have a list of integers, you can use autoboxing to add primitive values to the list.
Unboxing in java
The automatic conversion of wrapper class type into corresponding primitive type, is known as Unboxing. Unboxing is the process of converting a reference object to a corresponding primitive value, such as Integer to int. For example, if you have a list of integers, you can use unboxing to get primitive values from the list. Autoboxing and unboxing are features that allow the compiler to automatically convert between primitive and reference types, without using explicit cast operators.
Advantages of autoboxing and unboxing
1.More concise and more readable code
2.No Need to perform any typecasting explicitly
3.Make your code more flexible and interoperable
4.Collections Framework Integration like map,list.
5.We can assign “null” to an object of a wrapper class
Example of AutoBoxing
public class Autoboxing{
public static void main(String args[]){
int a=5;
Integer i=new Integer(a);//autoxing
Integer i1=Integer.valueOf(52);//autoboxing
}
}
Example of UnBoxing
public class Unboxing{
public static void main(String args[]){
Integer i=new Integer(5);
int a=i;//boxing
Integer i1=Integer.valueOf(52);
int a=i1.intValue();//boxing
}
}
Subscribe to my newsletter
Read articles from Anjan kumar Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anjan kumar Sharma
Anjan kumar Sharma
I am Java developer. I am seeking microservices in java . Life is always about creating and exploring yourself.