Migrating a Monolith Python Django Application to a Scalable Microservice Architecture
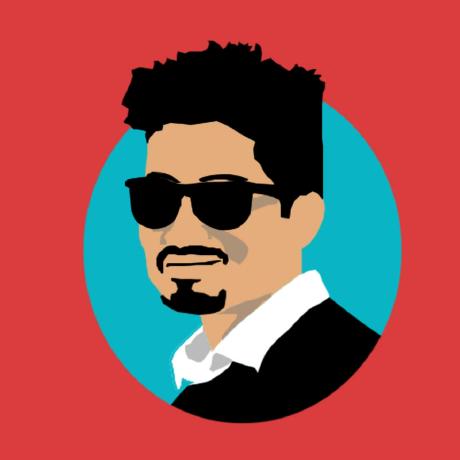
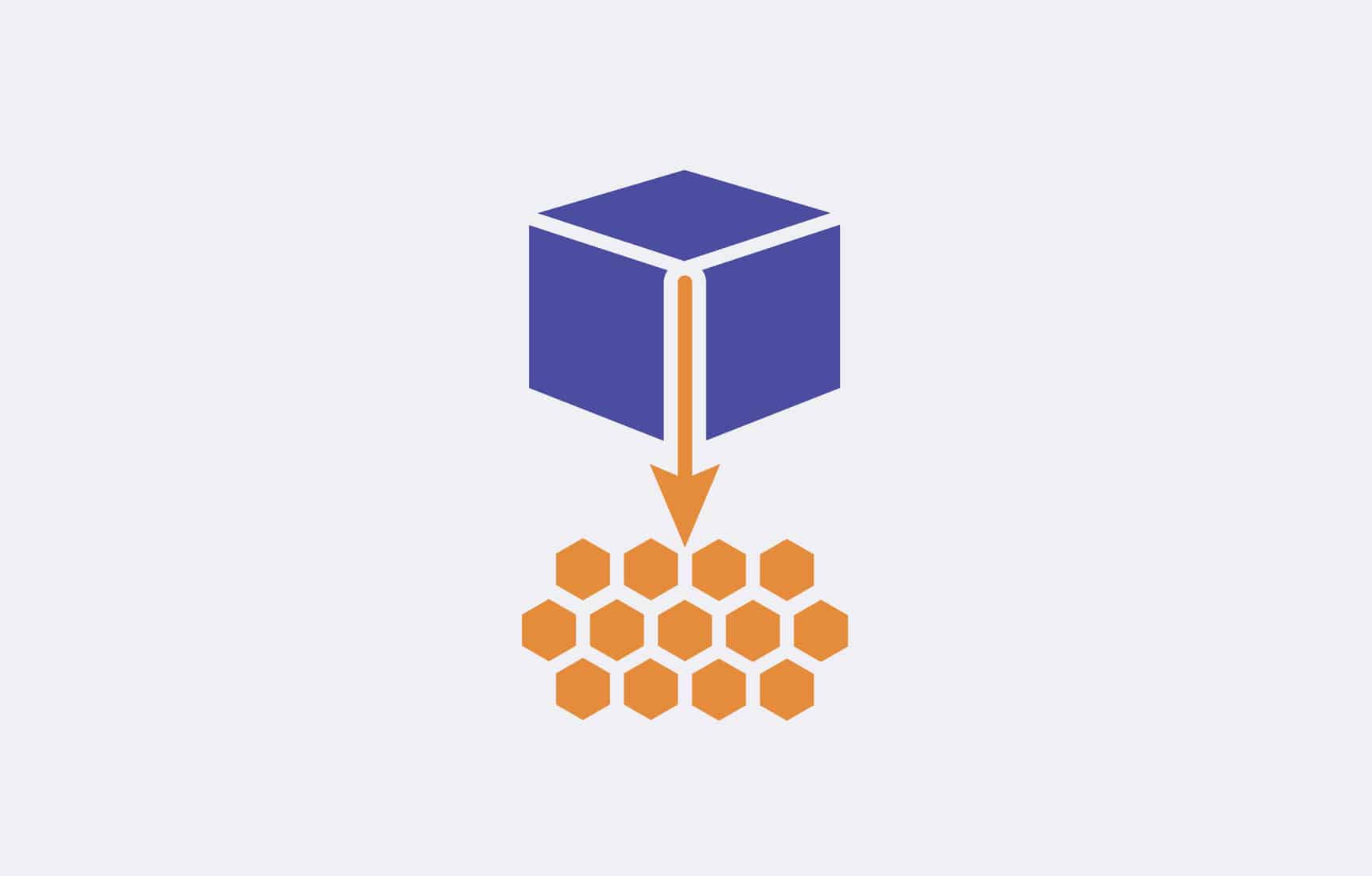
Transitioning a monolithic MVP to a scalable architecture is a critical task that ensures your application can handle increasing loads and complexity. This guide focuses on migrating a monolithic Django application, hosted on EC2 with Nginx as a reverse proxy and GitLab for CI/CD, to a microservices-based architecture on AWS. We'll dive deep into code refactoring, inter-service communication, database restructuring, and server architecture redesign, and step-by-step instructions.
Current Setup Overview
Framework: Python Django
Hosting: AWS EC2
Web Server: Nginx as a reverse proxy
CI/CD: GitLab
Step 1: Assessing the Monolithic Application
1.1 Identify Core Components
Identify the core components of your app portal (For our case - A freelance job portal):
User Management: Registration, authentication, and user profiles.
Job Management: Posting jobs, applying for jobs, job listings.
Client Management: Client profiles, job postings, hiring freelancers.
Freelancer Management: Freelancer profiles, application tracking, job history.
Messaging System: Communication between clients and freelancers.
Payment Processing: Handling payments and invoicing.
1.2 Analyze Dependencies and Boundaries
Analyze the interactions and dependencies between these components to determine how they can be decoupled.
Step 2: Redesigning the Codebase for Scalability
2.1 Microservices Architecture
Pros:
Independent deployment and scaling
Improved fault isolation
Technology agnostic
Cons:
Increased complexity
Network latency and overhead
Preferred Approach: Microservices, due to their scalability and fault isolation benefits.
2.2 Refactoring into Microservices
User Management Service: Create a new Django project for user management. Use Django Rest Framework (DRF) for building REST APIs.
# user_service/urls.py
from django.urls import path
from .views import RegisterView, LoginView, ProfileView
urlpatterns = [
path('register/', RegisterView.as_view(), name='register'),
path('login/', LoginView.as_view(), name='login'),
path('profile/', ProfileView.as_view(), name='profile'),
]
# user_service/views.py
from rest_framework import generics
from .models import User
from .serializers import UserSerializer, RegisterSerializer, LoginSerializer
class RegisterView(generics.CreateAPIView):
queryset = User.objects.all()
serializer_class = RegisterSerializer
class LoginView(generics.GenericAPIView):
serializer_class = LoginSerializer
def post(self, request, *args, **kwargs):
# Handle login logic here
pass
class ProfileView(generics.RetrieveUpdateAPIView):
queryset = User.objects.all()
serializer_class = UserSerializer
Job Management Service: Create a separate Django project for job management.
# job_service/urls.py
from django.urls import path
from .views import JobListView, JobDetailView, JobCreateView
urlpatterns = [
path('jobs/', JobListView.as_view(), name='job-list'),
path('jobs/<int:id>/', JobDetailView.as_view(), name='job-detail'),
path('jobs/create/', JobCreateView.as_view(), name='job-create'),
]
# job_service/views.py
from rest_framework import generics
from .models import Job
from .serializers import JobSerializer
class JobListView(generics.ListAPIView):
queryset = Job.objects.all()
serializer_class = JobSerializer
class JobDetailView(generics.RetrieveAPIView):
queryset = Job.objects.all()
serializer_class = JobSerializer
class JobCreateView(generics.CreateAPIView):
queryset = Job.objects.all()
serializer_class = JobSerializer
Client Management Service: Create another Django project for client management.
# client_service/urls.py
from django.urls import path
from .views import ClientListView, ClientDetailView
urlpatterns = [
path('clients/', ClientListView.as_view(), name='client-list'),
path('clients/<int:id>/', ClientDetailView.as_view(), name='client-detail'),
]
# client_service/views.py
from rest_framework import generics
from .models import Client
from .serializers import ClientSerializer
class ClientListView(generics.ListAPIView):
queryset = Client.objects.all()
serializer_class = ClientSerializer
class ClientDetailView(generics.RetrieveAPIView):
queryset = Client.objects.all()
serializer_class = ClientSerializer
Freelancer Management Service: Separate project for handling freelancer management.
# freelancer_service/urls.py
from django.urls import path
from .views import FreelancerListView, FreelancerDetailView
urlpatterns = [
path('freelancers/', FreelancerListView.as_view(), name='freelancer-list'),
path('freelancers/<int:id>/', FreelancerDetailView.as_view(), name='freelancer-detail'),
]
# freelancer_service/views.py
from rest_framework import generics
from .models import Freelancer
from .serializers import FreelancerSerializer
class FreelancerListView(generics.ListAPIView):
queryset = Freelancer.objects.all()
serializer_class = FreelancerSerializer
class FreelancerDetailView(generics.RetrieveAPIView):
queryset = Freelancer.objects.all()
serializer_class = FreelancerSerializer
Messaging Service: Create a project for messaging between clients and freelancers.
# messaging_service/urls.py
from django.urls import path
from .views import MessageListView, MessageCreateView
urlpatterns = [
path('messages/', MessageListView.as_view(), name='message-list'),
path('messages/create/', MessageCreateView.as_view(), name='message-create'),
]
# messaging_service/views.py
from rest_framework import generics
from .models import Message
from .serializers import MessageSerializer
class MessageListView(generics.ListAPIView):
queryset = Message.objects.all()
serializer_class = MessageSerializer
class MessageCreateView(generics.CreateAPIView):
queryset = Message.objects.all()
serializer_class = MessageSerializer
Payment Processing Service: Separate project for payment processing.
# payment_service/urls.py
from django.urls import path
from .views import PaymentView, InvoiceView
urlpatterns = [
path('payments/', PaymentView.as_view(), name='payments'),
path('invoices/', InvoiceView.as_view(), name='invoices'),
]
# payment_service/views.py
from rest_framework import generics
from .models import Payment, Invoice
from .serializers import PaymentSerializer, InvoiceSerializer
class PaymentView(generics.CreateAPIView):
queryset = Payment.objects.all()
serializer_class = PaymentSerializer
class InvoiceView(generics.ListAPIView):
queryset = Invoice.objects.all()
serializer_class = InvoiceSerializer
2.3 Inter-Service Communication
REST APIs: Use RESTful APIs for synchronous communication between services.
# Example of making a REST API call from the Job Service to the User Service
import requests
def get_user_profile(user_id):
response = requests.get(f'http://user-service/api/profile/{user_id}/')
return response.json()
Message Broker: Implement an event-driven architecture using RabbitMQ or AWS SQS for asynchronous communication.
# Example of publishing an event to RabbitMQ
import pika
import json
def publish_event(event):
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.queue_declare(queue='job_events')
channel.basic_publish(exchange='', routing_key='job_events', body=json.dumps(event))
connection.close()
Step 3: Redesigning the Server Architecture on AWS
3.1 Decoupling the Monolith
ECS (Elastic Container Service): Containerize each microservice using Docker. Use ECS for deploying and managing Docker containers.
# Dockerfile for user_service
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
CMD ["gunicorn", "user_service.wsgi:application", "--bind", "0.0.0.0:8000"]
# Dockerfile for job_service
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
CMD ["gunicorn", "job_service.wsgi:application", "--bind", "0.0.0.0:8000"]
Fargate: Use AWS Fargate to run containers without managing the underlying infrastructure.
3.2 Load Balancing and Auto Scaling
Application Load Balancer (ALB): Set up an ALB to distribute traffic among microservices.
# AWS CloudFormation template for ALB
Resources:
LoadBalancer:
Type: AWS::ElasticLoadBalancingV2::LoadBalancer
Properties:
Name: "MyALB"
Scheme: internet-facing
LoadBalancerAttributes:
- Key: idle_timeout.timeout_seconds
Value: 60
Subnets:
- subnet-12345678
- subnet-23456789
Auto Scaling: Configure auto-scaling policies to handle varying loads.
# AWS CloudFormation template for Auto Scaling
Resources:
AutoScalingGroup:
Type: AWS::AutoScaling::AutoScalingGroup
Properties:
AutoScalingGroupName: "MyAutoScalingGroup"
LaunchConfigurationName: !Ref LaunchConfiguration
MinSize: 1
MaxSize: 10
DesiredCapacity: 2
VPCZoneIdentifier:
- subnet-12345678
- subnet-23456789
TargetGroupARNs:
- !Ref TargetGroup
3.3 Database Architecture
RDS (Relational Database Service): Use Amazon RDS for a managed SQL database. Each microservice that requires relational storage can have its own RDS instance or schema.
# AWS CloudFormation template for RDS
Resources:
MyDB:
Type: AWS::RDS::DBInstance
Properties:
DBInstanceClass: db.t2.micro
Engine: MySQL
MasterUsername: admin
MasterUserPassword: mypassword
AllocatedStorage: 20
DBName: user_service_db
DynamoDB: Use DynamoDB for services requiring NoSQL databases, like the messaging system for fast read/write operations.
3.4 CI/CD Pipeline
Container Registry: Use Amazon ECR (Elastic Container Registry) to store Docker images.
GitLab CI/CD Configuration:
# .gitlab-ci.yml
stages:
- build
- deploy
build:
stage: build
script:
- docker build -t user_service:$CI_COMMIT_SHA .
- docker tag user_service:$CI_COMMIT_SHA $AWS_ACCOUNT_ID.dkr.ecr.$AWS_REGION.amazonaws.com/user_service:$CI_COMMIT_SHA
- aws ecr get-login-password --region $AWS_REGION | docker login --username AWS --password-stdin $AWS_ACCOUNT_ID.dkr.ecr.$AWS_REGION.amazonaws.com
- docker push $AWS_ACCOUNT_ID.dkr.ecr.$AWS_REGION.amazonaws.com/user_service:$CI_COMMIT_SHA
deploy:
stage: deploy
script:
- aws ecs update-service --cluster user-cluster --service user-service --force-new-deployment
only:
- master
Conclusion
Migrating a monolithic application to a scalable architecture is a multi-faceted process that involves careful planning, refactoring, and leveraging modern cloud infrastructure. By breaking down the monolithic Django application into microservices and utilizing AWS services like ECS, Fargate, ALB, and RDS, you can achieve a scalable and maintainable application.
By following these best practices, you'll be well on your way to building a robust and scalable application that can grow and adapt to future demands.
For further help please visit: AhmadWKhan.com
Subscribe to my newsletter
Read articles from Ahmad W Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
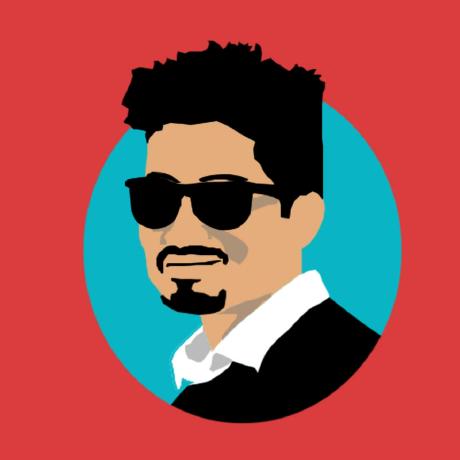