How to Center an Element Horizontally and Vertically with CSS Transform: A Step-by-Step Guide
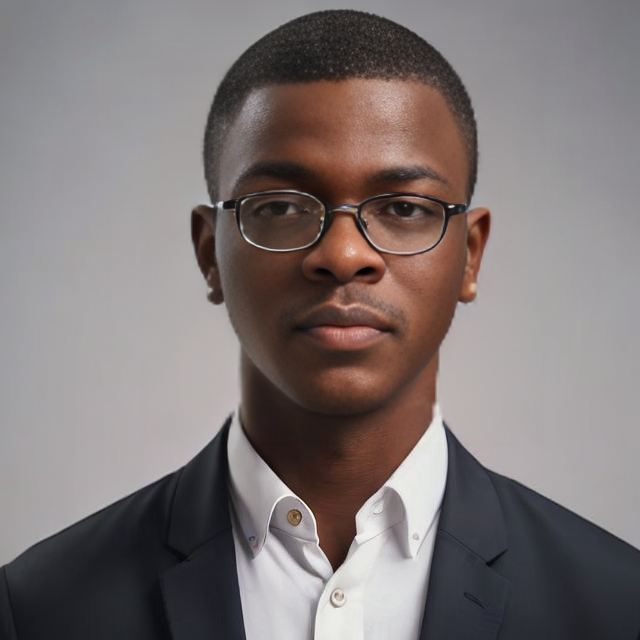
Table of contents
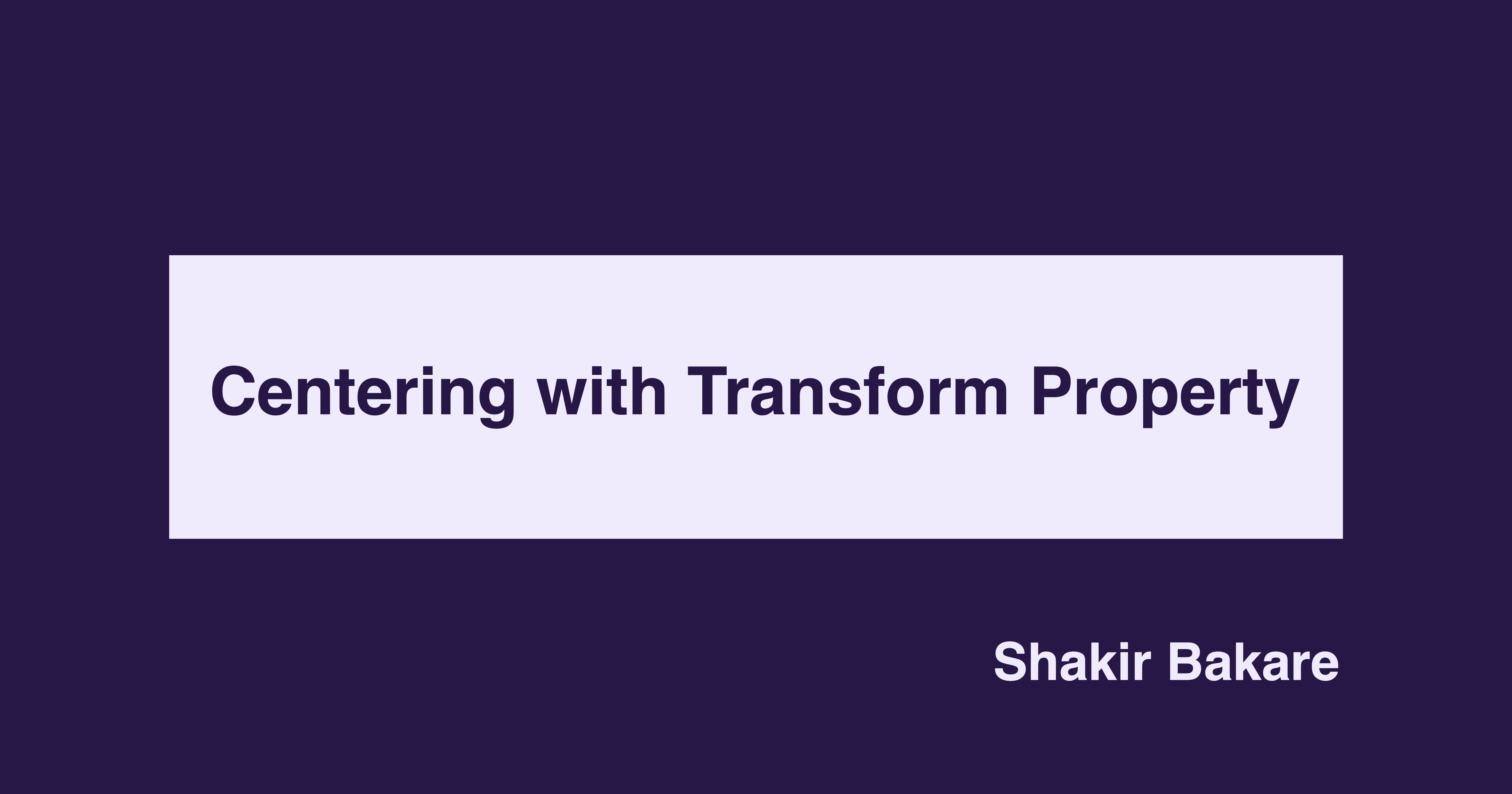
Centering elements in web development is a common task that can sometimes be tricky to achieve. One powerful and versatile method to accomplish this is by using the CSS transform
property. In this article, through straightforward steps and explanations, you will learn how to use the transform
property to center an element inside its parent element horizontally, vertically, and both combined.
Let's take it that we have created and linked an HTML file and a CSS file.
Inside the HTML file, we have:
<div class="parent">
<div class="child"></div>
</div>
Inside the CSS file, we have:
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
}
.child {
background-color: #271147;
height: 90px;
width: 90px;
}
Now, if we run the HTML file in a web browser, we will see this:
These are the child element and parent elements.
Now, let's center the child element inside the parent element horizontally.
How to center the child element horizontally
Step 1
We will add the position: absolute;
declaration to the .child
selector block.
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
}
.child {
background-color: #271147;
height: 90px;
width: 90px;
position: absolute; // here
}
This allows us to position the child element inside a parent element that has the position: relative;
declaration.
Step 2
We will add the position: relative;
declaration to the .parent
selector block.
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
position: relative; // here
}
.child {
background-color: #271147;
height: 90px;
width: 90px;
position: absolute;
}
This allows us to position the child element that has the position: absolute;
declaration inside the parent element.
Step 1 and 2 are necessary because, to position a child element inside a parent element, we must give the child element the position: absolute;
declaration and the parent element the position: relative;
declaration.
Step 3
We will add the left: 50%;
declaration to the .child
selector block.
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
position: relative;
.child {
background-color: #271147;
height: 90px;
width: 90px;
position: absolute;
left: 50%; // here
}
This positions the child element 50% from the left edge of the parent element.
Step 4
We will add the transform: translateX(-50%);
declaration to the .child
selector block.
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
position: relative;
.child {
background-color: #271147;
height: 90px;
width: 90px;
position: absolute;
left: 50%;
transform: translateX(-50%); // here
}
What does this do? We will understand what it does when we understand what the transform
property and the translateX()
function do in the declaration.
What does the transform property do?
It rotates, scales, skews, and moves an element. We are concerned about move, so let's focus on move. The transform property moves an element using the translate()
function.
That's what it does in the declaration.
What does the translate()
function do?
The translate function moves an element. It looks like this: translate(x, y);
x
specifies the distances to move the element horizontallyy
specifies the distances to move the element vertically
There is a syntax to only move the child element horizontally or vertically.
Horizontally, we will use:
transform: translateX(value);
Vertically, we will use:
transform: translateY(value);
The x
and y
value can be any length units like pixels, percentage, em, etc.
These units affect how the translate()
function moves an element.
percentages allows the function to move an element by its size (width for translateX, height for translateY).
pixels allows the function to move an element by pixels.
em allows the function to move an element by its font size.
Also, x
and y
can have negative values.
A negative value for
x
moves an element to the left.A negative value for
y
moves an element to the top.
That's about understanding what the transform
property and the translate()
function do.
Now, let's use this understanding to understand what the transform: translateX(-50%);
declaration does.
The transform property and the
translateX()
function in the declaration means we are moving the child element.translateX()
means we are moving the child element horizontally.-50%
is a negative value, meaning we are moving the child element to the left.The value is in percentages, meaning we are moving the child element by its width.
50% means half.
In one sentence, all these mean that the transform: translateX(-50%);
declaration means we are moving the child element to the left by half of its width.
With that, we have centered the child element horizontally inside the parent element.
But as you can see, the child element is centered horizontally at the top. If we want it centered at the bottom, we will add the bottom: 0;
declaration to the .child
selector block.
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
position: relative;
.child {
background-color: #271147;
height: 90px;
width: 90px;
position: absolute;
left: 50%;
bottom: 0; // here
transform: translateX(-50%);
}
This makes the child element horizontally centered at the bottom edge of the parent element.
Next, let's center the child element inside the parent element vertically.
But before we do this, I want us to remove the declarations we used for centering horizontally.
left: 50%;
transform: translateX(-50%);
bottom: 0;
To make the CSS code look like this:
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
position: relative;
.child {
background-color: #271147;
height: 90px;
width: 90px;
position: absolute;
}
And outputs this:
Why do I want this? I want this so that each centering process can be a standalone and easy to follow. Also to separate the code for centering horizontally from the one for centering vertically.
Now, let's center the child element vertically.
How to center the child element vertically
Step 1
We will add the top: 50%;
declaration to the .child
selector block.
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
position: relative;
.child {
background-color: #271147;
height: 90px;
width: 90px;
position: absolute;
top: 50%; // here
}
This positions the child element 50% from the top edge of the parent element.
Step 2
We will add the transform: translateY(-50%);
declaration to the .child
selector block.
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
position: relative;
.child {
background-color: #271147;
height: 90px;
width: 90px;
position: absolute;
top: 50%;
transform: translateY(-50%); // here
}
This moves the child element to the top by half of its height, and centers the child element vertically.
As you have learned in Step 4 of how to center the child element horizontally,
translateY()
function in the declaration means we are moving the child element vertically.-50%
means half of the child element height.-50%
is a negative value, meaning we are moving the child element to the top.
That is how we center the child element vertically.
Again, as you can see, the child element is centered vertically at the left. If we want it centered at the right, we will add the right: 0;
declaration to the .child
selector block.
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
position: relative;
.child {
background-color: #271147;
height: 90px;
width: 90px;
position: absolute;
top: 50%;
right: 0; // here
transform: translateY(-50%);
}
This makes the child element vertically centered at the right edge of the parent element.
Next, let's center the child element inside the parent element horizontally and vertically.
Again, I want us to remove the declarations we use for centering vertically.
top: 50%;
right: 0;
transform: translateY(-50%);
To make the CSS code look like this:
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
position: relative;
.child {
background-color: #271147;
height: 90px;
width: 90px;
position: absolute;
}
And outputs this:
You already know why I want us to do this.
Now, let's center the child element horizontally and vertically.
How to center the child element horizontally and vertically
Step 1
We will add the left: 50%;
declaration to the .child
selector block.
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
position: relative;
.child {
background-color: #271147;
height: 90px;
width: 90px;
position: absolute;
left: 50%; // here
}
You already know what this does. It positions the child element 50% from the left edge of the parent element.
Step 2
We will add the top: 50%;
declaration to the .child
selector block.
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
position: relative;
.child {
background-color: #271147;
height: 90px;
width: 90px;
position: absolute;
left: 50%;
top: 50%; // here
}
Again, you already know what it does. It positions the child 50% from the top edge of the parent element.
Step 3
We will add the transform: translate(-50%, -50%);
declaration to the .child
selector block.
.parent {
background-color: #f3ebfd;
height: 350px;
width: 350px;
position: relative;
.child {
background-color: #271147;
height: 90px;
width: 90px;
position: absolute;
left: 50%;
top: 50%;
transform: translate(-50%, -50%); // here
}
This moves the child element to the left by half of its width, and to the top by half of its height, centering the child element horizontally and vertically inside the parent element.
How? Here is how using what we have learned about the translate()
function:
In the transform: translate(-50%, -50%);
declaration,
-50%
as a negative value ofx
means we are moving the child element to the left by half of its width-50%
as a negative value ofy
means we are moving the child element to the top by half of its height
That is how we center the child element horizontally and vertically inside the parent element.
Conclusion
In this article, through straightforward steps and explanations, you have learned how to use the CSS transform property to center an element inside its parent element horizontally, vertically, and both combined. By following the steps and understanding the explanations, you can center any element inside its parent element in your web development projects.
Thanks for reading. If you found this article helpful, leave a feedback, like, and share it around for others who might need it.
Let's connect
Subscribe to my newsletter
Read articles from Shakir Bakare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
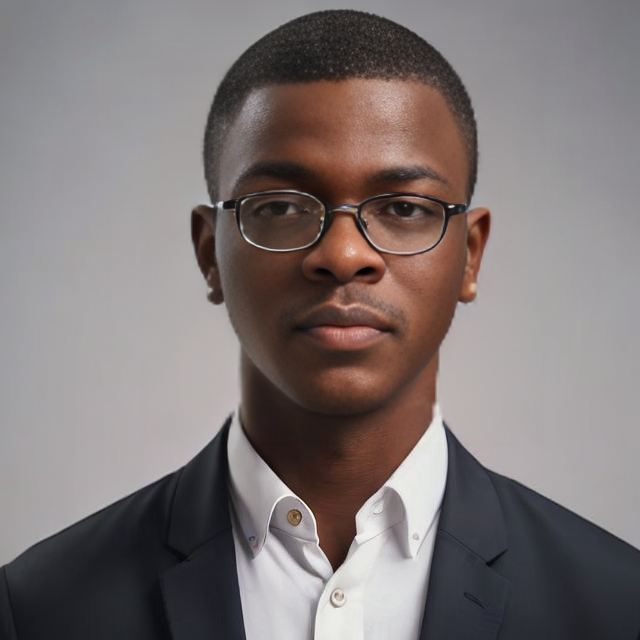
Shakir Bakare
Shakir Bakare
I'm a passionate frontend web developer with a love for creating beautiful and responsive web applications. With a strong foundation in HTML, CSS, JavaScript, and frameworks like React and Vue.js, I strive to bring innovative ideas to life. When I'm not coding, I enjoy sharing my knowledge through technical writing. I believe in the power of clear and concise documentation, and I write articles and tutorials to help others on their coding journey. Let's connect and create something amazing together!