Image Optimization in Next.js: Improving Performance and User Experience
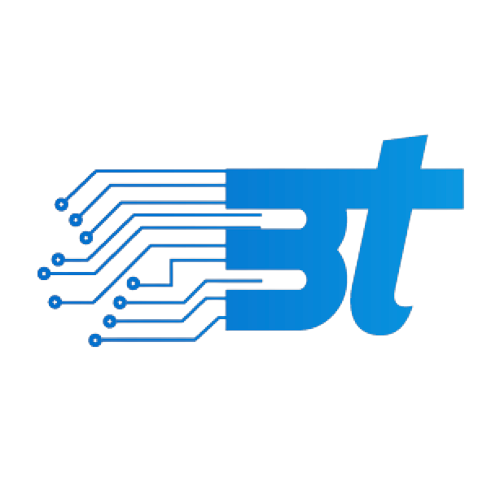
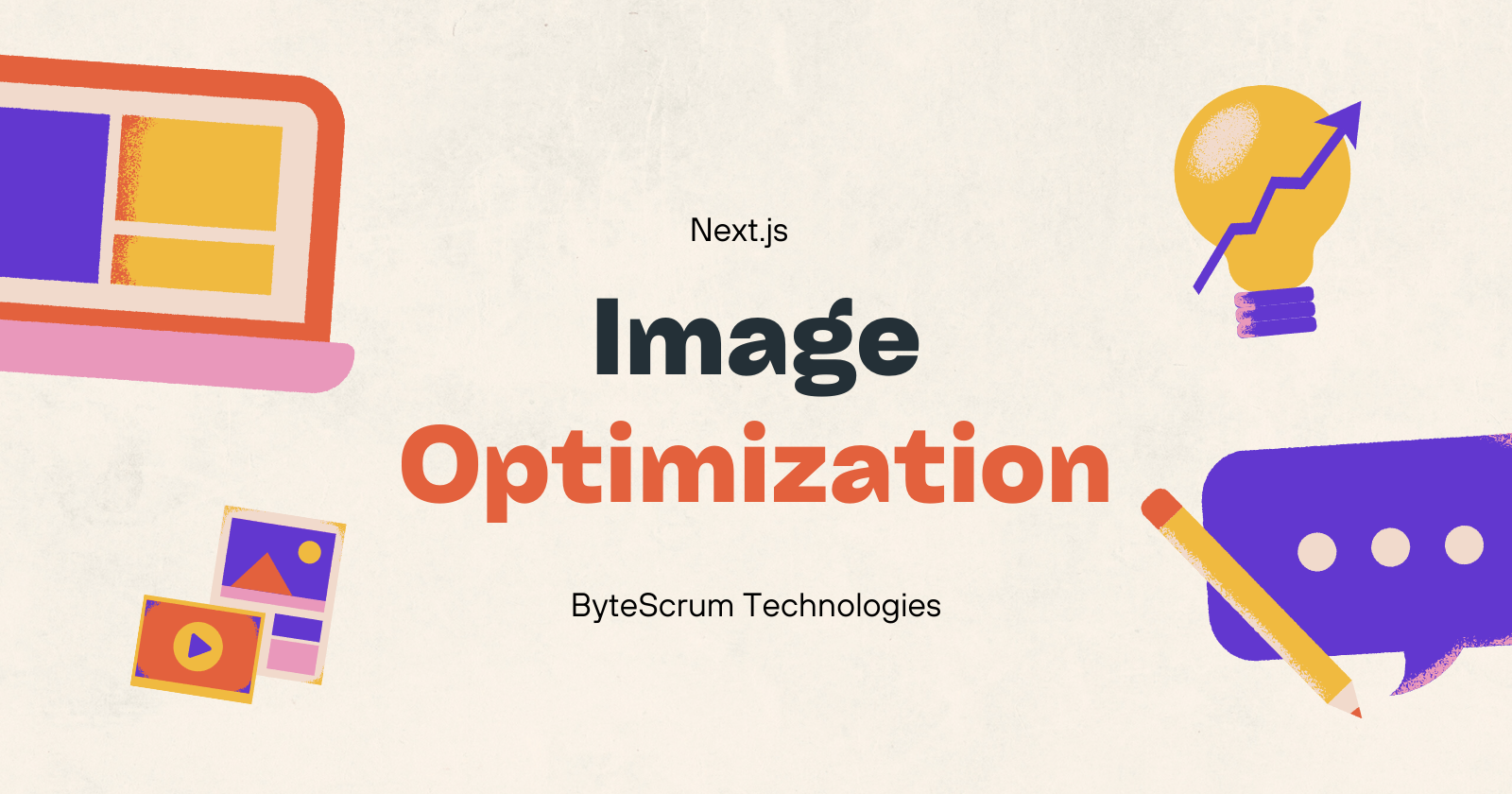
In today's fast-paced digital world, website performance is crucial for maintaining user engagement and improving SEO rankings. One of the most effective ways to enhance your website's performance is through image optimization. Next.js, a powerful React framework, offers built-in support for optimizing images, making it easier than ever to deliver fast and visually appealing websites. In this blog post, we'll explore how to optimize images in Next.js to improve performance and user experience.
Why Image Optimization Matters
Images are often the largest assets on a webpage, significantly impacting load times and overall performance. Optimizing images can:
Reduce Load Times: Smaller image sizes lead to faster page loads, which is crucial for retaining users and reducing bounce rates.
Improve SEO: Search engines favor fast-loading websites, which can improve your rankings.
Enhance User Experience: Faster load times result in a smoother browsing experience, increasing user satisfaction and engagement.
Next.js Image Component
Next.js provides an Image
component that automatically optimizes images. It includes features like responsive loading, lazy loading, and support for modern image formats (e.g., WebP). Here's how to use it:
Installation
First, ensure you have Next.js installed in your project:
npm install next react react-dom
Using the Image Component
Here's an example of how to use the Image
component in a Next.js project:
import Image from 'next/image'
export default function HomePage() {
return (
<div>
<h1>Welcome to My Next.js Site</h1>
<Image
src="/images/my-image.jpg"
alt="A beautiful scenery"
width={800}
height={600}
priority
/>
</div>
)
}
Key Features of the Image Component
Responsive Loading:
The
Image
component automatically adjusts the image size based on the device's screen size, ensuring optimal resolution and performance.Lazy Loading:
Images are loaded only when they enter the viewport, reducing initial page load time.
Modern Image Formats:
The
Image
component supports modern formats like WebP, which provide better compression without sacrificing quality.
Advanced Image Optimization Techniques
Next.js offers additional features to further enhance image optimization:
1. Image Optimization API:
Next.js provides an API to automatically optimize images on-demand. This API resizes, optimizes, and serves images in modern formats. No additional configuration is needed; it works out of the box with the Image
component.
2. Custom Loaders:
You can define custom loaders to fetch images from various sources (e.g., a CMS or cloud storage). Here's an example using a custom loader:
import Image from 'next/image'
const myLoader = ({ src, width, quality }) => {
return `https://example.com/${src}?w=${width}&q=${quality || 75}`
}
export default function HomePage() {
return (
<div>
<h1>Welcome to My Next.js Site</h1>
<Image
loader={myLoader}
src="my-image.jpg"
alt="A beautiful scenery"
width={800}
height={600}
/>
</div>
)
}
3. Responsive and Art-Direction Support:
You can define different image sizes for various screen sizes, ensuring optimal display across devices. This technique is known as art direction.
import Image from 'next/image'
export default function HomePage() {
return (
<div>
<h1>Welcome to My Next.js Site</h1>
<Image
src="/images/my-image.jpg"
alt="A beautiful scenery"
sizes="(max-width: 800px) 100vw, 800px"
width={800}
height={600}
priority
/>
</div>
)
}
4. Placeholder Images:
To improve user experience, you can use placeholder images while the main image is loading. Next.js supports blur and empty placeholders:
import Image from 'next/image'
export default function HomePage() {
return (
<div>
<h1>Welcome to My Next.js Site</h1>
<Image
src="/images/my-image.jpg"
alt="A beautiful scenery"
width={800}
height={600}
placeholder="blur"
blurDataURL="/images/placeholder.jpg"
/>
</div>
)
}
Conclusion
Image
component and built-in optimization features. By leveraging these tools, you can ensure that your website loads quickly, looks great, and provides an exceptional user experience. Implement these techniques in your Next.js projects to boost performance and stay ahead in the competitive digital landscape.Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
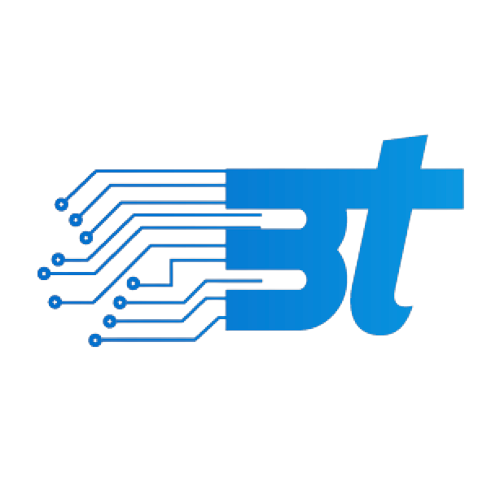
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.