Introduction to AWS Step Functions
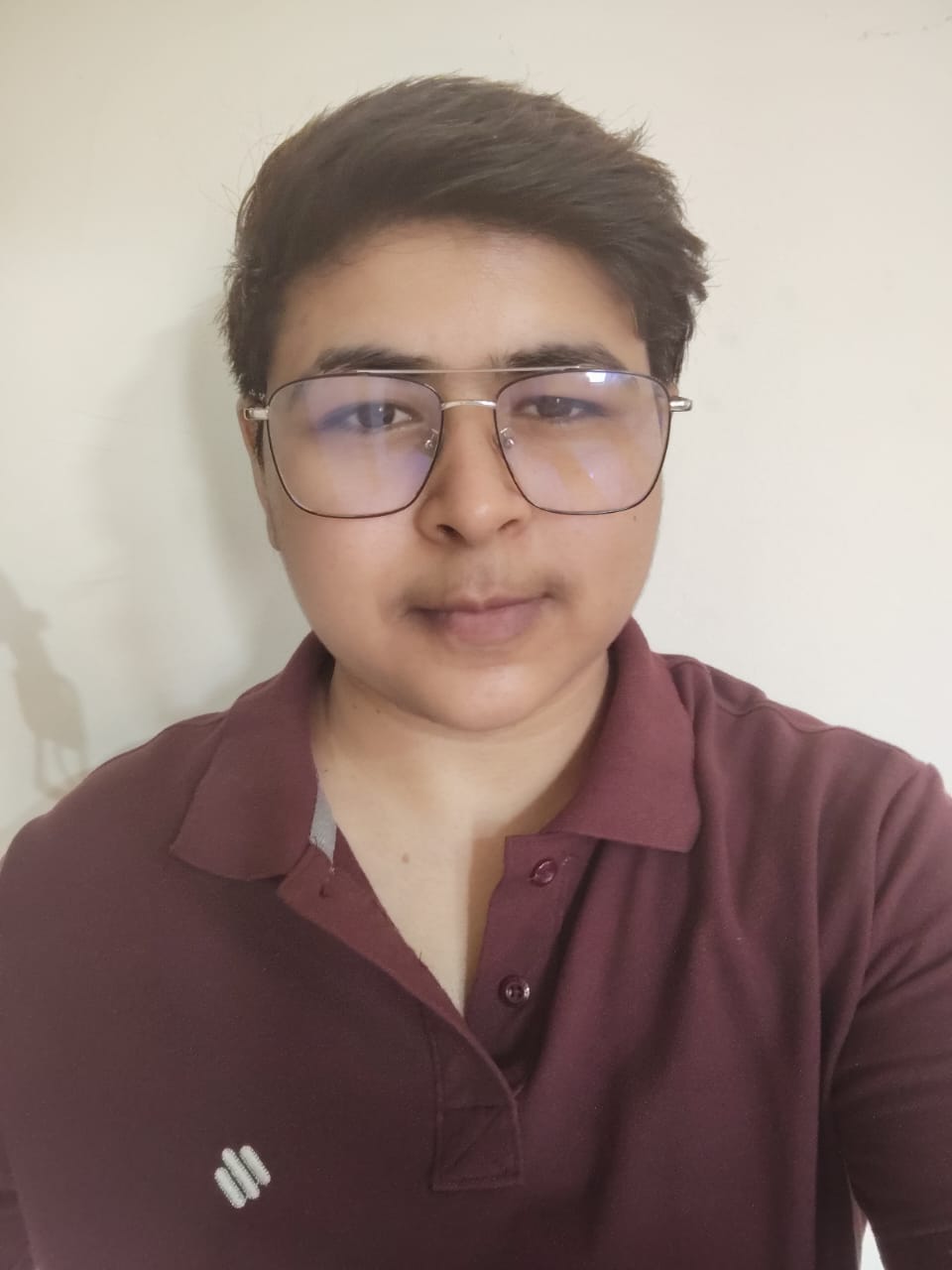
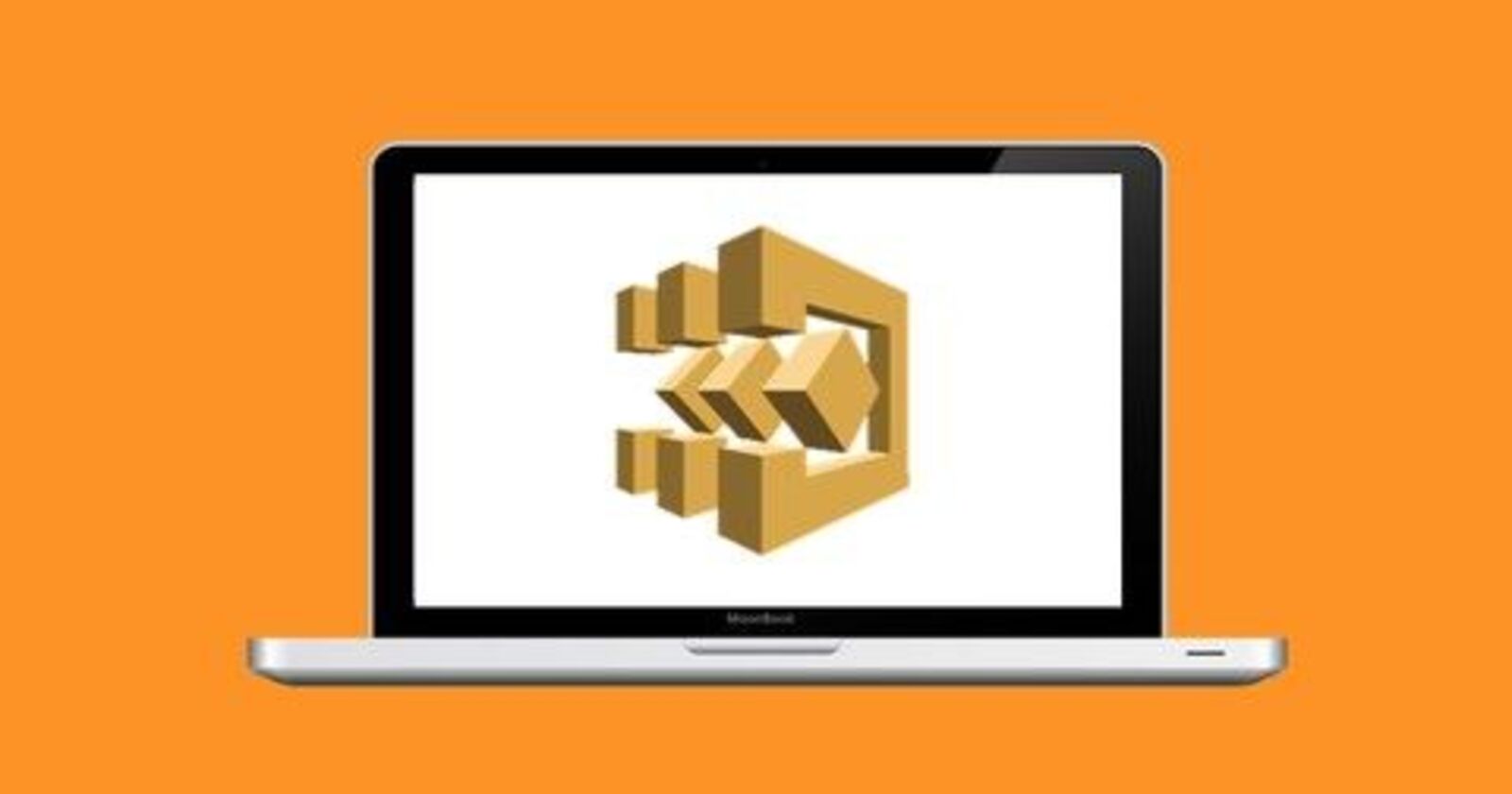
Introduction
AWS Step Functions is a powerful orchestration service that enables you to coordinate multiple AWS services into serverless workflows. It provides a visual interface to build and run a series of steps each representing a single unit of work that is either an AWS Lambda function an AWS service or your custom application. Step Functions make it easy to design and implement complex workflows, manage state, handle errors and ensure that your applications run smoothly.
In this blog we will dive into the concepts of AWS Step Functions, understand how to create state machines, explore common use cases and provide examples to help you get started with orchestrating serverless workflows.
Understanding AWS Step Functions
What Are AWS Step Functions?
AWS Step Functions is a fully managed service that allows you to orchestrate workflows using state machines. A state machine is a collection of states each performing a specific task or waiting for a certain condition to be met before transitioning to the next state. Step Functions ensure that your workflows are executed in a reliable, scalable and cost-effective manner.
Key Features of AWS Step Functions
Visual Workflow Design :- Step Functions provide a graphical console to design, visualize and monitor your workflows.
Integration with AWS Services :- Step Functions integrate with a wide range of AWS services including Lambda, EC2, ECS, SNS, SQS, DynamoDB and more.
Error Handling :- Built-in retry mechanisms and error handling capabilities ensure robust workflow execution.
State Management :- Automatically manages the state of each step simplifying the development of complex workflows.
Scalability :- Automatically scales to handle the execution of workflows without requiring manual intervention.
Creating State Machines
Components of a State Machine
States :- The building blocks of a state machine representing individual tasks, choices, waits or parallel executions.
Transitions :- Define the flow of execution from one state to another.
Start State :- The initial state of the state machine.
End State :- The final state indicating the completion of the workflow.
Types of States
Task State :- Executes a single unit of work such as invoking a Lambda function or an API call.
Choice State :- Branches the execution path based on the evaluation of conditions.
Wait State :- Pauses the execution for a specified duration.
Parallel State :- Executes multiple branches of states concurrently.
Pass State :- Passes input to the next state without performing any work.
Fail State :- Stops the execution and marks the workflow as failed.
Succeed State :- Marks the successful completion of the workflow.
Example :- Creating a Simple State Machine
Let's create a simple state machine that invokes a Lambda function to process data and then waits for 5 seconds before completing the workflow.
- Step 1 :- Create a Lambda Function
Create a Lambda function that processes data. For this example we'll use a simple Python function.
import json
def lambda_handler(event, context):
print("Processing data...")
# Your processing logic here
return {
'statusCode': 200,
'body': json.dumps('Data processed successfully!')
}
- Step 2 :- Define the State Machine
Use the AWS Management Console or AWS CLI to define the state machine. Here's the JSON definition for our state machine :-
{
"Comment": "A simple state machine example",
"StartAt": "ProcessData",
"States": {
"ProcessData": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:ProcessDataFunction",
"End": true
}
}
}
- Step 3 :- Create the State Machine
In the AWS Management Console navigate to the Step Functions service and create a new state machine using the above JSON definition.Alternatively use the AWS CLI :
aws stepfunctions create-state-machine --name SimpleStateMachine --definition file://state-machine.json --role-arn arn:aws:iam::123456789012:role/service-role/StepFunctions-SimpleStateMachine
Use Cases for AWS Step Functions
1. Data Processing Pipelines
Step Functions can orchestrate complex data processing pipelines involving multiple stages such as data extraction, transformation and loading (ETL). Each stage can be implemented as a separate Lambda function or an AWS service integration.
Example :-
{
"Comment": "Data processing pipeline",
"StartAt": "ExtractData",
"States": {
"ExtractData": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:ExtractDataFunction",
"Next": "TransformData"
},
"TransformData": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:TransformDataFunction",
"Next": "LoadData"
},
"LoadData": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:LoadDataFunction",
"End": true
}
}
}
2. Microservices Orchestration
Step Functions can coordinate the interactions between multiple microservices ensuring that the overall workflow is executed reliably and efficiently. Each microservice can be represented as a task state, and the state machine can handle error recovery and retries.
Example :-
{
"Comment": "Microservices orchestration",
"StartAt": "ServiceA",
"States": {
"ServiceA": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:ServiceAFunction",
"Next": "ServiceB"
},
"ServiceB": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:ServiceBFunction",
"Next": "ServiceC"
},
"ServiceC": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:ServiceCFunction",
"End": true
}
}
}
3. Human Approval Workflows
Step Functions can be used to build workflows that require human approval. For example a workflow can pause execution at a specific state and wait for a human operator to provide input before proceeding.
Example :-
{
"Comment": "Human approval workflow",
"StartAt": "SubmitRequest",
"States": {
"SubmitRequest": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:SubmitRequestFunction",
"Next": "WaitForApproval"
},
"WaitForApproval": {
"Type": "Wait",
"Seconds": 3600,
"Next": "CheckApproval"
},
"CheckApproval": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:CheckApprovalFunction",
"End": true
}
}
}
4. Batch Processing
Step Functions can orchestrate batch processing jobs where each job consists of multiple tasks that need to be executed sequentially or in parallel. This can include data validation, processing and aggregation tasks.
Example :-
{
"Comment": "Batch processing workflow",
"StartAt": "ValidateData",
"States": {
"ValidateData": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:ValidateDataFunction",
"Next": "ProcessData"
},
"ProcessData": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:ProcessDataFunction",
"Next": "AggregateResults"
},
"AggregateResults": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:AggregateResultsFunction",
"End": true
}
}
}
Best Practices for Using AWS Step Functions
1. Modular Design
Design your workflows in a modular manner where each state performs a single well-defined task. This makes it easier to manage, test and reuse individual components of your workflow.
2. Error Handling and Retries
Implement robust error handling and retry mechanisms to ensure that your workflows can recover from failures. Use Catch and Retry fields to handle exceptions and control retry behavior.
Example :-
{
"StartAt": "ProcessData",
"States": {
"ProcessData": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:ProcessDataFunction",
"Retry": [
{
"ErrorEquals": ["Lambda.ServiceException", "Lambda.AWSLambdaException"],
"IntervalSeconds": 2,
"MaxAttempts": 6,
"BackoffRate": 2.0
}
],
"Catch": [
{
"ErrorEquals": ["States.ALL"],
"Next": "HandleError"
}
],
"End": true
},
"HandleError": {
"Type": "Task",
"Resource": "arn:aws:lambda:us-west-2:123456789012:function:HandleErrorFunction",
"End": true
}
}
}
3. Logging and Monitoring
Enable logging and monitoring for your state machines to track execution history, identify issues and analyze performance. Use CloudWatch Logs and CloudWatch Metrics to gain insights into your workflows.
4. Testing and Validation
Thoroughly test your state machines to ensure they behave as expected. Use Step Functions built-in validation features to check for syntax errors and logical inconsistencies.
Conclusion
AWS Step Functions is a versatile and powerful service for orchestrating serverless workflows. By understanding its key features, components and use cases you can design and implement complex workflows that integrate seamlessly with other AWS services. Whether you're building data processing pipelines, microservices orchestration, human approval workflows or batch processing jobs. Step Functions provides the tools you need to ensure reliable and efficient execution.
Stay tuned for more insights in our upcoming blog posts.
Let's connect and grow on LinkedIn :Click Here
Let's connect and grow on Twitter :Click Here
Happy Cloud Computing!!!
Happy Reading!!!
Sudha Yadav
Subscribe to my newsletter
Read articles from Sudha Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
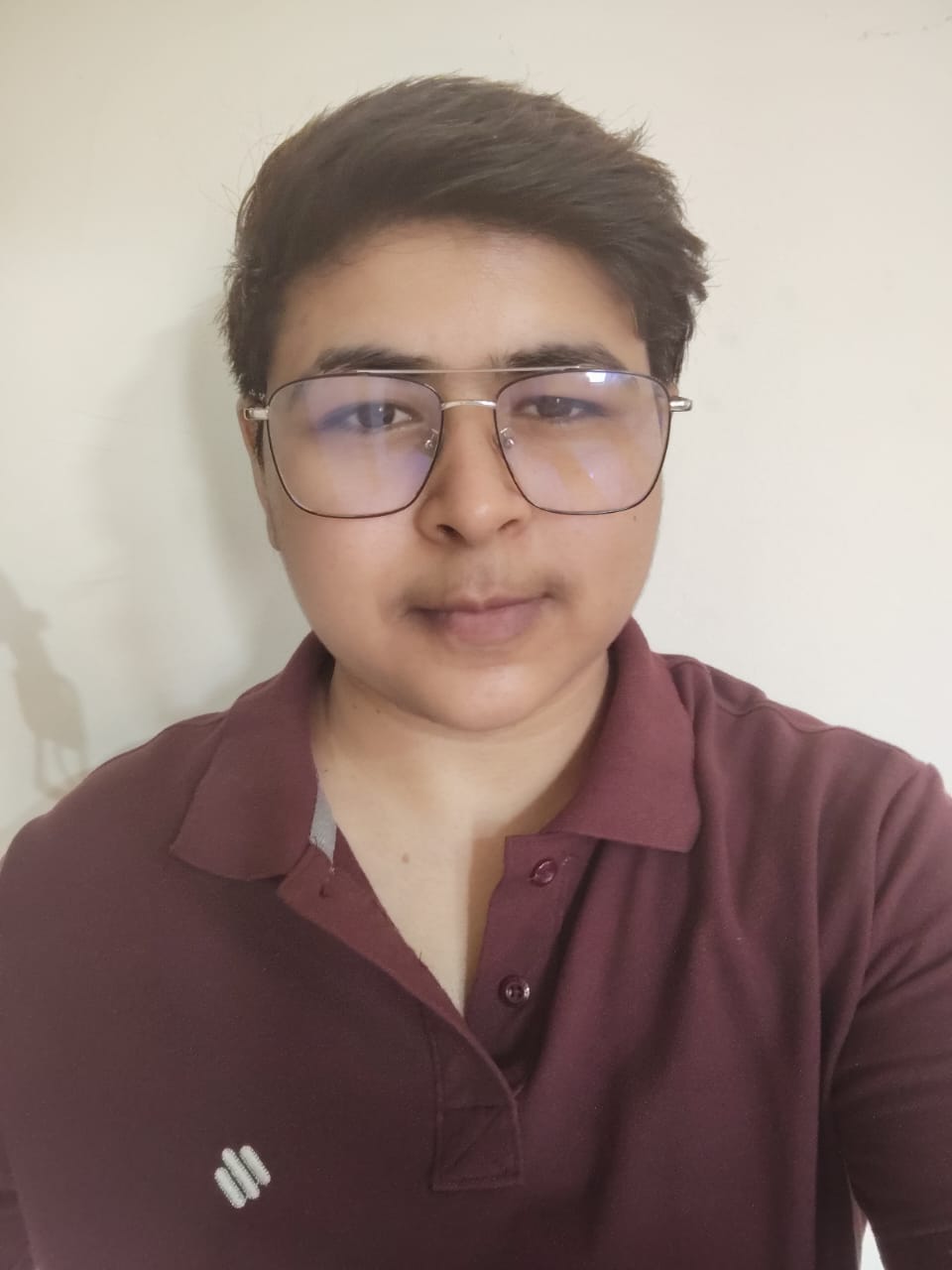
Sudha Yadav
Sudha Yadav
๐ Hi everyone! I'm Sudha Yadav, a DevOps engineer with a big passion for all things DevOps. I've knowledge and worked on some cool projects and I can't wait to share what I'm learning with you all! ๐ ๏ธ Here's what's in my toolbox: Linux Github Docker Kubernetes Jenkins AWS Python Prometheus Grafana Ansible Terraform Join me as we explore AWS DevOps together. Let's learn and grow together in this ever-changing field! ๐ค Feel free to connect with me for: Sharing experiences Friendly chats Learning together Follow my journey on Twitter and LinkedIn for daily updates. Let's dive into the world of DevOps together! ๐ #DevOps #AWS #DevOpsJourney #90DaysOfDevOps