Passing Data in Android: A Guide for Developers

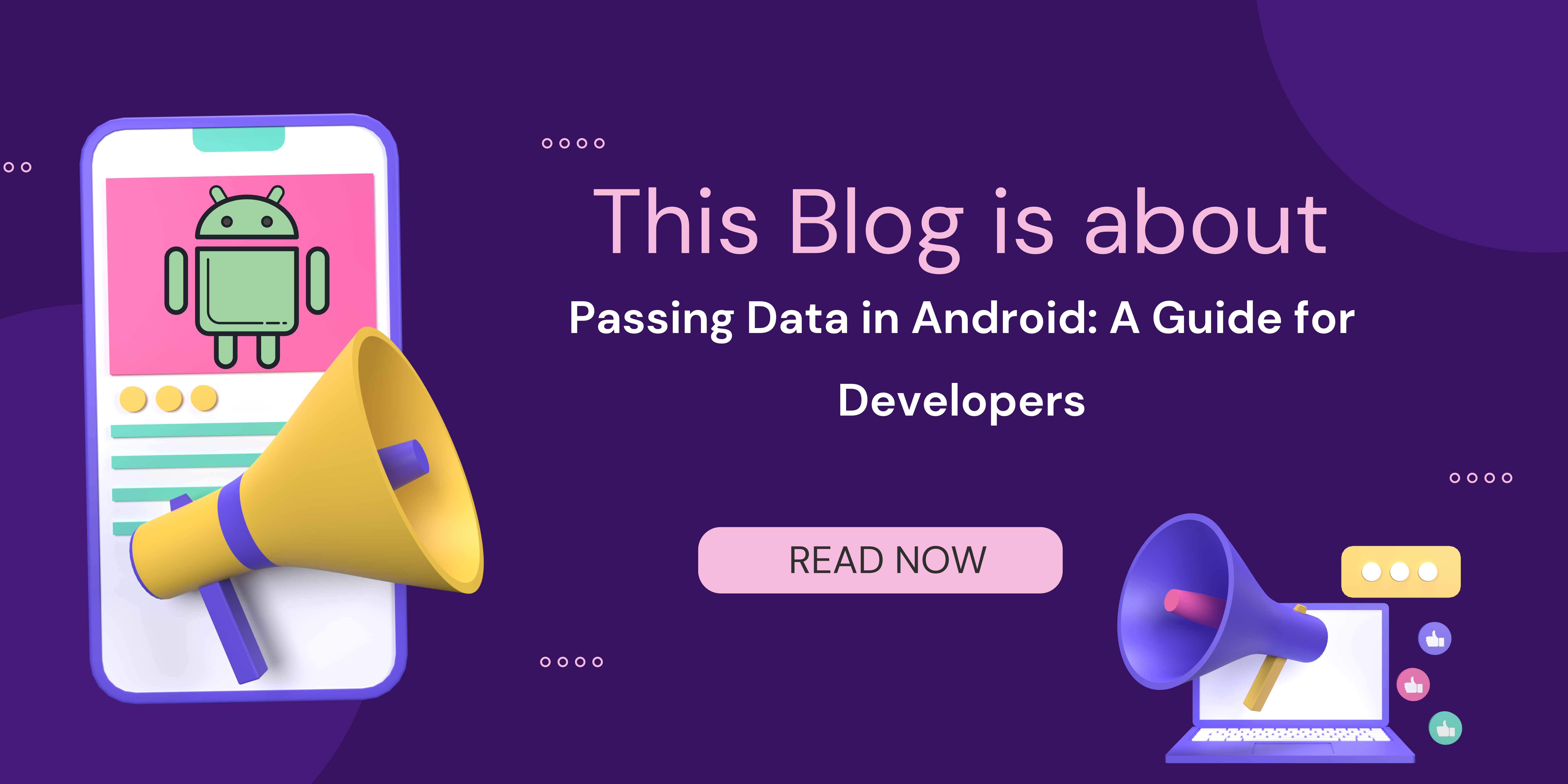
Hello devs, in today's article, we will explore different ways to pass data in the Android Component. Mastering data transfer between various parts of an application is crucial. This guide covers methods to pass data between activities, fragments, and their combinations. Each section includes best practices, code examples, and common mistakes.
Passing Data from
Activity to Activity
Activity to Fragment
Activity2 to Activity1
Fragment to Fragment
Fragment to Activity
Fragment2 to Fragment1
Let's start the journey.
Passing Data from Activity to Activity
When navigating between activities, we often need to pass data. The Intent
class provides a seamless way to achieve this.
// In Activity1
val intent = Intent(this, Activity2::class.java).apply {
putExtra("key", "value")
}
startActivity(intent)
// In Activity2
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity2)
val value = intent.getStringExtra("key")
}
Key Points:
Use
putExtra()
to add data to theIntent
.Retrieve the data in the destination activity with
getStringExtra()
or similar methods for other data types.
Passing Data from Activity to Fragment
To pass data from an activity to a fragment, you can use the Bundle
class.
// In Activity
val bundle = Bundle().apply {
putString("key", "value")
}
val fragment = YourFragment().apply {
arguments = bundle
}
supportFragmentManager.beginTransaction()
.replace(R.id.fragment_container, fragment)
.commit()
// In YourFragment
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
arguments?.let {
val value = it.getString("key")
}
}
Key Points:
Use
arguments
property to pass aBundle
to the fragment.Retrieve the data in the fragment using
arguments
.
Passing Data from Activity2 to Activity1
When passing data back from Activity2 to Activity1, startActivityForResult()
is useful.
// In Activity1
private lateinit var resultLauncher: ActivityResultLauncher<Intent>
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity1)
resultLauncher = registerForActivityResult(ActivityResultContracts.StartActivityForResult()) { result ->
if (result.resultCode == Activity.RESULT_OK) {
val data: Intent? = result.data
val value = data?.getStringExtra("key")
// Handle the received data
}
}
val intent = Intent(this, Activity2::class.java)
resultLauncher.launch(intent)
}
}
// In Activity2
val returnIntent = Intent().apply {
putExtra("key", "value")
}
setResult(Activity.RESULT_OK, returnIntent)
finish()
Key Points:
ActivityResultLauncher: Registers a callback to handle the result.
ActivityResultContracts.StartActivityForResult: Built-in contract for starting an activity and receiving a result.
setResult(): Sets the result data and finishes the activity.
Passing Data from Fragment to Fragment
Fragments within the same activity can communicate through their parent activity.
// In Fragment1
val fragment = Fragment2().apply {
arguments = Bundle().apply {
putString("key", "value")
}
}
parentFragmentManager.beginTransaction()
.replace(R.id.fragment_container, fragment)
.commit()
// In Fragment2
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
arguments?.let {
val value = it.getString("key")
}
}
Key Points:
Fragments should use
parentFragmentManager
to replace or add other fragments.Data is passed using
Bundle
as in activity-to-fragment communication.
Passing Data from Fragment to Activity
Fragments can communicate with their parent activity using interfaces.
// In Fragment
interface OnFragmentInteractionListener {
fun onFragmentInteraction(data: String)
}
private var listener: OnFragmentInteractionListener? = null
override fun onAttach(context: Context) {
super.onAttach(context)
if (context is OnFragmentInteractionListener) {
listener = context
} else {
throw RuntimeException("$context must implement OnFragmentInteractionListener")
}
}
fun someMethod() {
listener?.onFragmentInteraction("value")
}
// In Activity
class MainActivity : AppCompatActivity(), Fragment.OnFragmentInteractionListener {
override fun onFragmentInteraction(data: String) {
// Handle the data
}
}
Key Points:
Define an interface in the fragment.
Implement the interface in the parent activity to handle the data.
Passing Data from Fragment2 to Fragment1
To pass data between fragments, the parent activity can be used as a mediator.
// In Fragment2
(activity as? MainActivity)?.sendDataToFragment1("value")
// In MainActivity
fun sendDataToFragment1(data: String) {
val fragment = supportFragmentManager.findFragmentById(R.id.fragment1) as? Fragment1
fragment?.receiveData(data)
}
// In Fragment1
fun receiveData(data: String) {
// Handle the data
}
Key Points:
Use the parent activity to facilitate communication between fragments.
Ensure the receiving fragment has a method to handle incoming data.
Okay devs, SO it's time to wrap up the blog. I hope you understand data transfer between different components in Android. See you on the next Blog Devs.
Connect with Me:
Hey there! If you enjoyed reading this blog and found it informative, why not connect with me on LinkedIn? ๐ You can also follow my Instagram page for more mobile development-related content. ๐ฒ๐จโ๐ป Letโs stay connected, share knowledge and have some fun in the exciting world of app development! ๐
Subscribe to my newsletter
Read articles from Mayursinh Parmar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mayursinh Parmar
Mayursinh Parmar
๐ฑMobile App Developer | Android & Flutter ๐๐ก Passionate about creating intuitive and engaging apps ๐ญโจ Letโs shape the future of mobile technology!