Mastering Linked Lists: Types, Operations, and Use Cases - Hiike
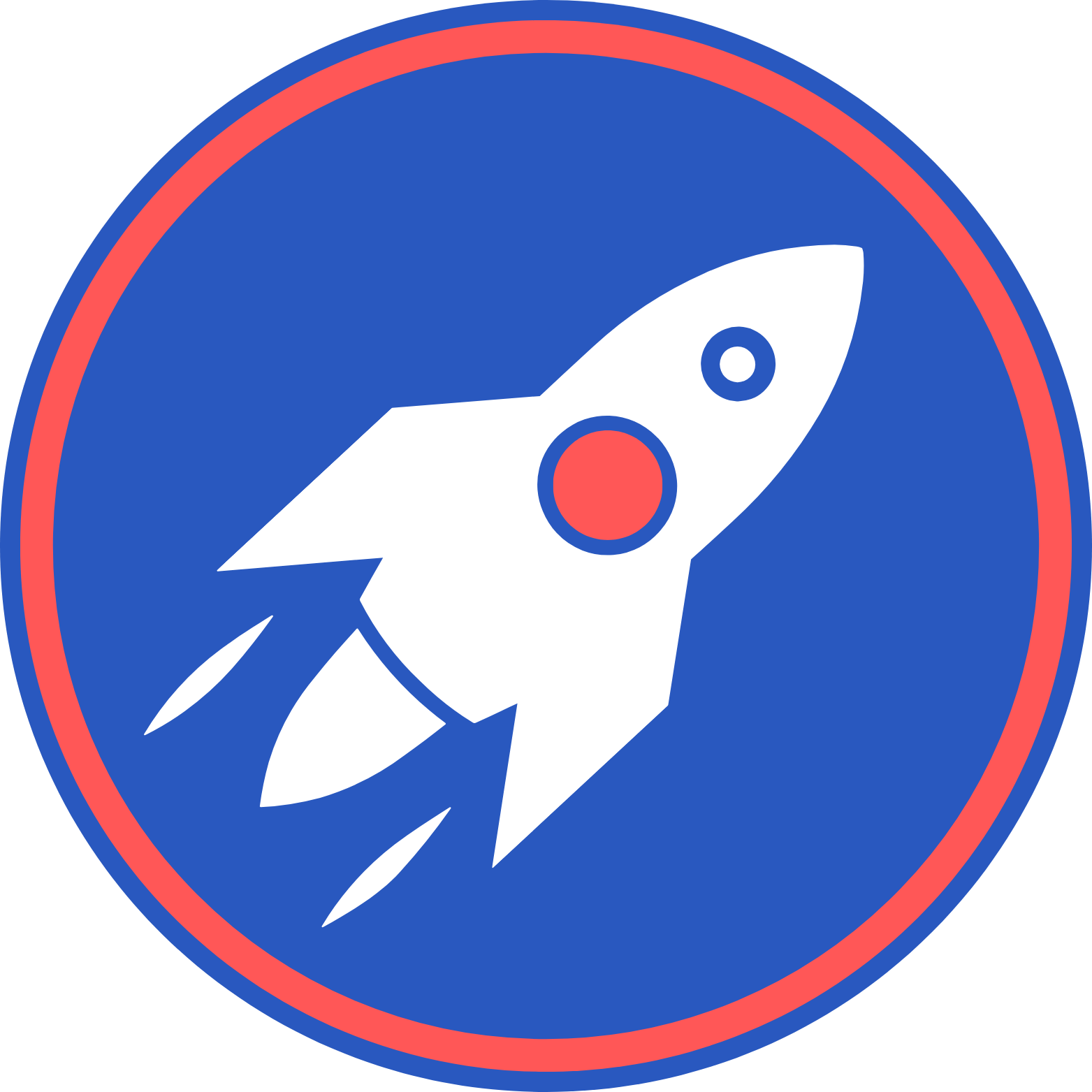
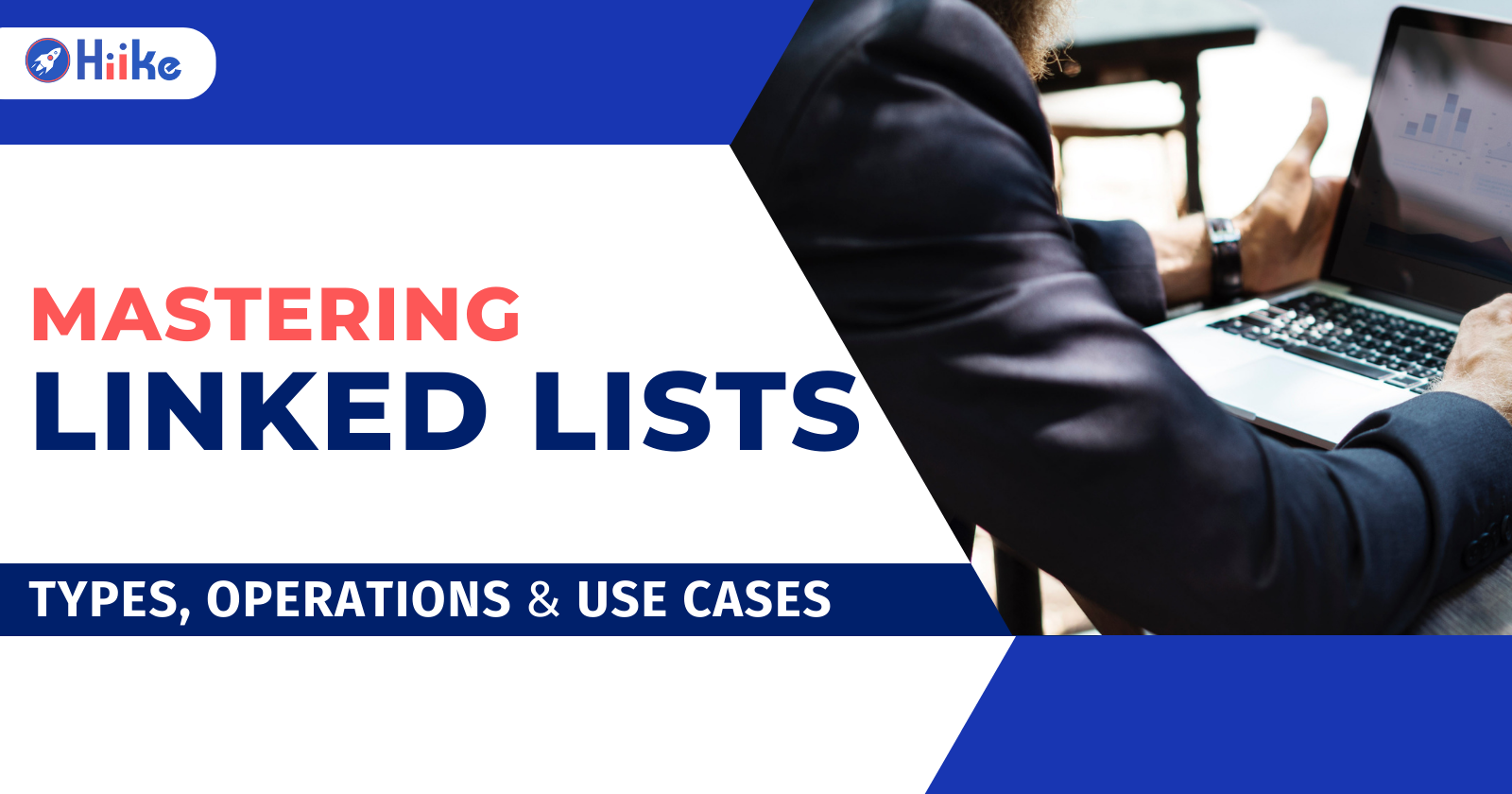
Linked lists are one of the foundational data structures in computer science, prized for their simplicity and flexibility. Whether you're just starting out in programming or looking to deepen your understanding, this guide will walk you through everything you need to know about linked lists: the different types, essential operations, and practical use cases. Let's dive in!
Types of Linked Lists
Linked lists come in a few flavors, each with its own strengths. Let's explore the three main types: singly linked lists, doubly linked lists, and circular linked lists.
Singly Linked Lists
Singly linked lists are the simplest type. Each node contains two things: the data and a pointer to the next node. This structure lets you traverse the list in one direction, from the head to the end.
Key Points:
Data and Next Pointer: Each node has data and a next pointer.
Single Direction: You can only move forward through the list.
Efficiency: Great for situations where you only need forward traversal.
Doubly Linked Lists
Doubly linked lists add a bit more complexity. Each node includes data, a pointer to the next node, and a pointer to the previous node. This allows traversal in both directions, offering more flexibility.
Key Points:
Data, Next, and Prev Pointers: Each node has pointers for both the next and previous nodes.
Bidirectional Traversal: Move forward or backward through the list.
Memory Overhead: Requires extra memory for the additional pointer.
Circular Linked Lists
Circular linked lists take things a step further. Here, the last node points back to the first node, forming a loop. This can be particularly useful for applications that require cyclic traversal.
Key Points:
Circular Structure: The last node points to the first node.
Endless Loop: Suitable for scenarios where continuous traversal is needed.
Flexible Forms: Can be either singly or doubly linked.
Linked List Operations
Linked lists are powerful because they support several fundamental operations: insertion, deletion, searching, and traversal. Let’s break these down.
Insertion
Inserting a node into a linked list is pretty straightforward. You can add a node at the beginning, end, or any specific position by adjusting the pointers accordingly.
Types of Insertion:
At the Beginning: Update the new node’s next pointer to the current head, then update the head to the new node.
At the End: Traverse to the last node, then update its next pointer to the new node.
At a Specific Position: Traverse to the desired position, update the new node’s next pointer to the next node, and the previous node’s next pointer to the new node.
Deletion
Deleting a node involves removing it and adjusting the pointers to maintain the list structure. This can also be done at the beginning, end, or any specific position.
Types of Deletion:
At the Beginning: Update the head to the next node.
At the End: Traverse to the second-to-last node and set its next pointer to null.
At a Specific Position: Update the previous node’s next pointer to skip the node being deleted.
Searching
Searching through a linked list means starting at the head and moving through each node until you find the one you’re looking for. This has a linear time complexity of O(n).
Steps:
Start at the head node.
Traverse each node until the desired element is found or the end of the list is reached.
Return the position of the element or null if not found.
Traversal
Traversal involves visiting each node in the list to perform operations like displaying data or modifying nodes. You can use a temporary pointer to iterate through the list.
Steps:
Initialize a temporary pointer to the head node.
Move the pointer to the next node while performing the required operation.
Continue until you reach the end of the list.
Use Cases of Linked Lists
Linked lists are incredibly versatile and can be used in a variety of scenarios. Here are some of the most common and practical uses:
Implementing Stacks and Queues
Linked lists are ideal for implementing abstract data types like stacks and queues. Stacks operate on a Last In, First Out (LIFO) basis, while queues follow a First In, First Out (FIFO) principle.
Stacks:
Efficiently implement push and pop operations.
Dynamic resizing capabilities.
Queues:
Simplifies enqueue and dequeue operations.
Perfect for dynamic data handling.
Reversing a Linked List
Reversing a linked list requires altering the pointers' directions. This operation is frequently used in various algorithms and can be accomplished through iterative or recursive methods.
Steps:
Initialize three pointers: previous, current, and next.
Traverse the list and reverse the pointers one by one.
Modify the head pointer to reference the last node.
Implementing Undo/Redo Functionality
Many applications, like text editors, use linked lists to manage undo and redo operations. Each action is stored as a node, allowing easy navigation through the history of actions.
Representing Polynomials
Polynomials can be efficiently represented using linked lists, where each node stores a term of the polynomial. This makes it easy to manipulate terms and perform polynomial arithmetic.
Implementing Hash Tables
Linked lists help resolve collisions in hash tables through separate chaining. Each bucket in the hash table points to a linked list of entries that hash to the same index.
Graph Algorithms
Linked lists are used to represent adjacency lists in graph algorithms. They provide an efficient way to store and traverse graph edges, essential for algorithms like Breadth-First Search (BFS) and Depth-First Search (DFS).
Conclusion
Linked lists are a cornerstone of data structures, offering a combination of simplicity and power. Understanding the different types of linked lists, their operations, and practical use cases will significantly enhance your programming skills.
By practicing these concepts, you’ll be able to implement, manipulate, and utilize linked lists with confidence. Whether you're dealing with stacks, queues, undo/redo functionality, or graph algorithms, linked lists provide a robust and flexible solution.
I hope this guide has given you a clear understanding of linked lists. If you have any questions or need further clarification, feel free to reach out. Happy coding.
Author Bio
Pranjal Jain is the co-founder of Hiike, an innovative edtech startup dedicated to upskilling software engineers. A gold medalist from IIT Kanpur, Pranjal has a distinguished career with previous roles at Samsung and Microsoft. He personally teaches Data Structures, Algorithms, and System Design, demonstrating his deep passion and dedication to education. Pranjal’s commitment to teaching ensures that complex technical concepts are accessible and engaging for learners. He is also an active voice in the tech education community, sharing valuable insights and resources through Hiike’s YouTube channel and LinkedIn profile. Connect with him on LinkedIn — https://www.linkedin.com/in/techpranjal/ .
Subscribe to my newsletter
Read articles from Hiike directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
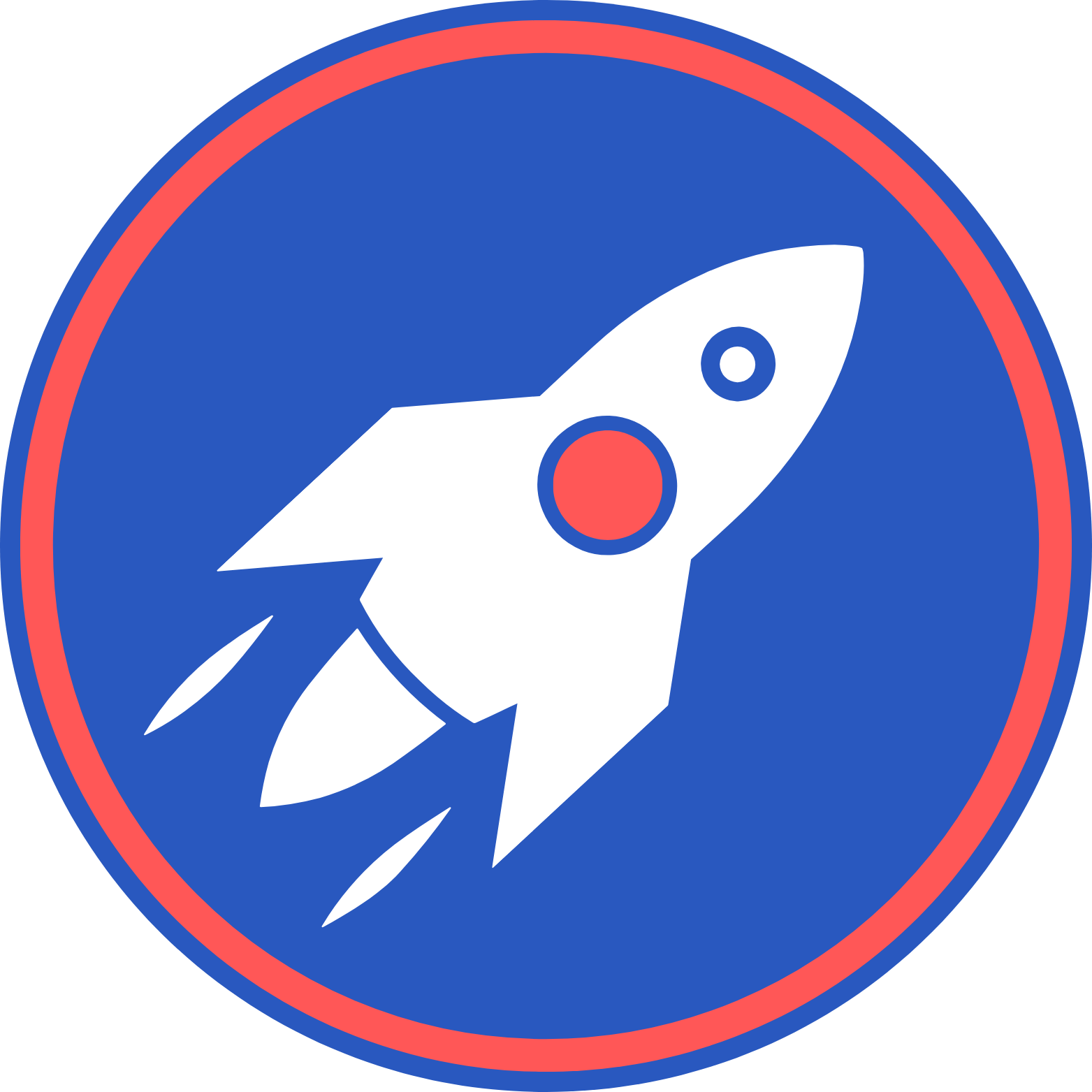
Hiike
Hiike
🌟𝐇𝐢𝐢𝐤𝐞 - Crafting Your Tech Odyssey! 🚀 Join Hiike for immersive learning, expert mentorship, and accelerated career growth. Our IITian-led courses and vibrant community ensure you excel in Data Structures, Algorithms, and System Design. Transform your tech career with Hiike today!